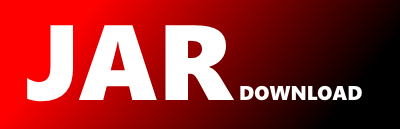
org.thymeleaf.standard.inliner.StandardTextInliner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thymeleaf Show documentation
Show all versions of thymeleaf Show documentation
Modern server-side Java template engine for both web and standalone environments
/*
* =============================================================================
*
* Copyright (c) 2011, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.standard.inliner;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.thymeleaf.Arguments;
import org.thymeleaf.TemplateEngine;
import org.thymeleaf.inliner.ITextInliner;
import org.thymeleaf.standard.processor.value.IStandardValueProcessor;
import org.thymeleaf.standard.syntax.StandardSyntax;
import org.thymeleaf.standard.syntax.StandardSyntax.Value;
import org.thymeleaf.templateresolver.TemplateResolution;
import org.thymeleaf.util.JavaScriptUtils;
import org.w3c.dom.Text;
/**
*
* @author Daniel Fernández
*
* @since 1.0
*
*/
public class StandardTextInliner implements ITextInliner {
private static final Logger logger = LoggerFactory.getLogger(StandardTextInliner.class);
public enum InlineType { TEXT, JAVASCRIPT, NONE }
public static final String TEXT_INLINE_EVAL = "\\[\\[(.*?)\\]\\]";
public static final Pattern TEXT_INLINE_EVAL_PATTERN = Pattern.compile(TEXT_INLINE_EVAL, Pattern.DOTALL);
public static final String JAVASCRIPT_ADD_INLINE_EVAL = "\\/\\*\\[\\+(.*?)\\+\\]\\*\\/";
public static final Pattern JAVASCRIPT_ADD_INLINE_EVAL_PATTERN = Pattern.compile(JAVASCRIPT_ADD_INLINE_EVAL, Pattern.DOTALL);
public static final String JAVASCRIPT_REMOVE_INLINE_EVAL = "\\/\\*\\[\\-(.*?)\\-\\]\\*\\/";
public static final Pattern JAVASCRIPT_REMOVE_INLINE_EVAL_PATTERN = Pattern.compile(JAVASCRIPT_REMOVE_INLINE_EVAL, Pattern.DOTALL);
public static final String JAVASCRIPT_VARIABLE_EXPRESSION_INLINE_EVAL = "\\/\\*(\\[\\[(.*?)\\]\\])\\*\\/([^\n]*?)\n";
public static final Pattern JAVASCRIPT_VARIABLE_EXPRESSION_INLINE_EVAL_PATTERN = Pattern.compile(JAVASCRIPT_VARIABLE_EXPRESSION_INLINE_EVAL, Pattern.DOTALL);
public static final String JAVASCRIPT_INLINE_EVAL = "\\[\\[(.*?)\\]\\]";
public static final Pattern JAVASCRIPT_INLINE_EVAL_PATTERN = Pattern.compile(JAVASCRIPT_INLINE_EVAL, Pattern.DOTALL);
private final InlineType inlineType;
private final IStandardValueProcessor valueProcessor;
public StandardTextInliner(final InlineType inlineType, final IStandardValueProcessor valueProcessor) {
super();
this.inlineType = inlineType;
this.valueProcessor = valueProcessor;
}
public void inline(final Arguments arguments, final TemplateResolution templateResolution, final Text text) {
final String content = text.getTextContent();
switch (this.inlineType) {
case TEXT:
final String textContent =
processTextInline(content, this.valueProcessor, arguments, templateResolution);
text.setTextContent(textContent);
break;
case JAVASCRIPT:
final String javascriptContent =
processJavascriptInline(content, this.valueProcessor, arguments, templateResolution);
text.setTextContent(javascriptContent);
break;
case NONE:
break;
}
}
private static String processTextInline(
final String input,
final IStandardValueProcessor valueProcessor,
final Arguments arguments, final TemplateResolution templateResolution) {
final Matcher matcher = TEXT_INLINE_EVAL_PATTERN.matcher(input);
if (matcher.find()) {
final StringBuilder strBuilder = new StringBuilder();
int curr = 0;
do {
strBuilder.append(input.substring(curr,matcher.start(0)));
final String match = matcher.group(1);
if (logger.isTraceEnabled()) {
logger.trace("[THYMELEAF][{}] Applying text inline evaluation on \"{}\"", TemplateEngine.threadIndex(), match);
}
final Value value =
StandardSyntax.parseValue(match, valueProcessor, arguments, templateResolution);
if (value == null) {
strBuilder.append(match);
} else {
final Object result =
valueProcessor.getValue(arguments, templateResolution, value);
strBuilder.append(result);
}
curr = matcher.end(0);
} while (matcher.find());
strBuilder.append(input.substring(curr));
return strBuilder.toString();
}
return input;
}
private static String processJavascriptInline(
final String input,
final IStandardValueProcessor valueProcessor,
final Arguments arguments, final TemplateResolution templateResolution) {
return
processJavascriptVariableInline(
processJavascriptVariableExpressionInline(
processJavascriptAddInline(
processJavascriptRemoveInline(
input)
)
),
valueProcessor, arguments, templateResolution);
}
private static String processJavascriptAddInline(final String input) {
final Matcher matcher = JAVASCRIPT_ADD_INLINE_EVAL_PATTERN.matcher(input);
if (matcher.find()) {
final StringBuilder strBuilder = new StringBuilder();
int curr = 0;
do {
strBuilder.append(input.substring(curr,matcher.start(0)));
final String match = matcher.group(1);
if (logger.isTraceEnabled()) {
logger.trace("[THYMELEAF][{}] Adding inlined javascript text \"{}\"", TemplateEngine.threadIndex(), match);
}
strBuilder.append(match);
curr = matcher.end(0);
} while (matcher.find());
strBuilder.append(input.substring(curr));
return strBuilder.toString();
}
return input;
}
private static String processJavascriptRemoveInline(final String input) {
final Matcher matcher = JAVASCRIPT_REMOVE_INLINE_EVAL_PATTERN.matcher(input);
if (matcher.find()) {
final StringBuilder strBuilder = new StringBuilder();
int curr = 0;
do {
strBuilder.append(input.substring(curr,matcher.start(0)));
final String match = matcher.group(1);
if (logger.isTraceEnabled()) {
logger.trace("[THYMELEAF][{}] Removing inlined javascript text \"{}\"", TemplateEngine.threadIndex(), match);
}
curr = matcher.end(0);
} while (matcher.find());
strBuilder.append(input.substring(curr));
return strBuilder.toString();
}
return input;
}
private static String processJavascriptVariableExpressionInline(final String input) {
final Matcher matcher = JAVASCRIPT_VARIABLE_EXPRESSION_INLINE_EVAL_PATTERN.matcher(input);
if (matcher.find()) {
final StringBuilder strBuilder = new StringBuilder();
int curr = 0;
do {
strBuilder.append(input.substring(curr,matcher.start(0)));
strBuilder.append(matcher.group(1));
strBuilder.append(';');
curr = matcher.end(0);
} while (matcher.find());
strBuilder.append(input.substring(curr));
return strBuilder.toString();
}
return input;
}
private static String processJavascriptVariableInline(
final String input,
final IStandardValueProcessor valueProcessor,
final Arguments arguments, final TemplateResolution templateResolution) {
final Matcher matcher = JAVASCRIPT_INLINE_EVAL_PATTERN.matcher(input);
if (matcher.find()) {
final StringBuilder strBuilder = new StringBuilder();
int curr = 0;
do {
strBuilder.append(input.substring(curr,matcher.start(0)));
final String match = matcher.group(1);
if (logger.isTraceEnabled()) {
logger.trace("[THYMELEAF][{}] Applying javascript variable inline evaluation on \"{}\"", TemplateEngine.threadIndex(), match);
}
final Value value =
StandardSyntax.parseValue(match, valueProcessor, arguments, templateResolution);
if (value == null) {
strBuilder.append(match);
} else {
final Object result =
valueProcessor.getValue(arguments, templateResolution, value);
strBuilder.append(JavaScriptUtils.print(result));
}
curr = matcher.end(0);
} while (matcher.find());
strBuilder.append(input.substring(curr));
return strBuilder.toString();
}
return input;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy