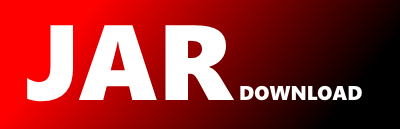
org.thymeleaf.messageresolver.StandardMessageResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thymeleaf Show documentation
Show all versions of thymeleaf Show documentation
Modern server-side Java template engine for both web and standalone environments
/*
* =============================================================================
*
* Copyright (c) 2011, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.messageresolver;
import java.util.Properties;
import org.thymeleaf.Arguments;
import org.thymeleaf.standard.StandardMessageResolutionUtils;
import org.thymeleaf.templateresolver.TemplateResolution;
import org.thymeleaf.util.Validate;
/**
*
* Standard implementation of {@link IMessageResolver}.
*
*
* A message in template /WEB-INF/templates/home.html for locale
* ll_CC-vv ("ll" = language, "CC" = country, "vv" = variant) would be looked for
* in .properties files in the following sequence:
*
*
* - /WEB-INF/templates/home_ll_CC-vv.properties
* - /WEB-INF/templates/home_ll_CC.properties
* - /WEB-INF/templates/home_ll.properties
* - /WEB-INF/templates/home.properties
* - (default messages, if they exist)
*
*
* @author Daniel Fernández
*
* @since 1.0
*
*/
public class StandardMessageResolver
extends AbstractMessageResolver {
private final Properties defaultMessages;
public StandardMessageResolver() {
super();
this.defaultMessages = new Properties();
}
/**
*
* Returns the default messages. These messages will be used
* if no other messages can be found.
*
*
* @return the default messages
*/
public synchronized Properties getDefaultMessages() {
final Properties properties = new Properties();
properties.putAll(this.defaultMessages);
return properties;
}
/**
*
* Unsynchronized method meant only for use by subclasses.
*
*
* @return the default messages
*/
protected Properties unsafeGetDefaultMessages() {
return this.defaultMessages;
}
/**
*
* Sets the default messages. These messages will be used
* if no other messages can be found.
*
*
* @param defaultMessages the new default messages
*/
public synchronized void setDefaultMessages(final Properties defaultMessages) {
checkNotInitialized();
if (defaultMessages != null) {
this.defaultMessages.putAll(defaultMessages);
}
}
/**
*
* Adds a new message to the set of default messages.
*
*
* @param key the message key
* @param value the message value (text)
*/
public synchronized void addDefaultMessage(final String key, final String value) {
checkNotInitialized();
Validate.notNull(key, "Key for default message cannot be null");
Validate.notNull(value, "Value for default message cannot be null");
this.defaultMessages.put(key, value);
}
/**
*
* Clears the set of default messages.
*
*/
public synchronized void clearDefaultMessages() {
checkNotInitialized();
this.defaultMessages.clear();
}
public MessageResolution resolveMessage(
final Arguments arguments, final TemplateResolution templateResolution,
final String key, final Object[] messageParameters) {
// This method can be overriden
checkInitialized();
return
new MessageResolution(
StandardMessageResolutionUtils.resolveMessageForTemplate(
arguments, templateResolution, key, messageParameters,
unsafeGetDefaultMessages()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy