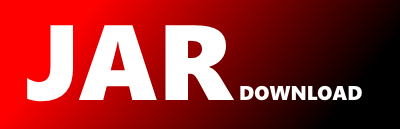
org.thymeleaf.templateparser.html.AbstractHtmlTemplateParser Maven / Gradle / Ivy
package org.thymeleaf.templateparser.html;
import java.io.Reader;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.List;
import org.thymeleaf.Configuration;
import org.thymeleaf.dom.Document;
import org.thymeleaf.dom.Node;
import org.thymeleaf.exceptions.ConfigurationException;
import org.thymeleaf.exceptions.TemplateInputException;
import org.thymeleaf.exceptions.TemplateProcessingException;
import org.thymeleaf.templateparser.EntityResolver;
import org.thymeleaf.templateparser.EntitySubstitutionTemplateReader;
import org.thymeleaf.templateparser.ErrorHandler;
import org.thymeleaf.templateparser.ITemplateParser;
import org.thymeleaf.util.ResourcePool;
import org.thymeleaf.util.StandardDOMTranslator;
import org.xml.sax.InputSource;
/**
*
* Document parser implementation for non-XML HTML documents.
*
*
* @since 2.0.0
*
* @author Daniel Fernández
*/
public abstract class AbstractHtmlTemplateParser implements ITemplateParser {
private final String templateModeName;
private final boolean nekoInClasspath;
private final NekoBasedHtmlParser parser;
public AbstractHtmlTemplateParser(final String templateModeName, int poolSize) {
super();
boolean nekoFound = true;
try {
Thread.currentThread().getContextClassLoader().loadClass("org.cyberneko.html.parsers.DOMParser");
} catch (final ClassNotFoundException e) {
nekoFound = false;
}
this.nekoInClasspath = nekoFound;
this.templateModeName = templateModeName;
if (this.nekoInClasspath) {
this.parser = new NekoBasedHtmlParser(poolSize);
} else {
this.parser = null;
}
}
public final Document parseTemplate(final Configuration configuration, final String documentName, final Reader reader) {
if (!this.nekoInClasspath) {
throw new ConfigurationException(
"Cannot perform conversion to XML from legacy HTML: The nekoHTML library " +
"is not in classpath. nekoHTML 1.9.15 or newer is required for processing templates in " +
"\"" + this.templateModeName + "\" mode [http://nekohtml.sourceforge.net]. Maven spec: " +
"\"net.sourceforge.nekohtml::nekohtml::1.9.15\". IMPORTANT: DO NOT use versions of " +
"nekoHTML older than 1.9.15.");
}
return this.parser.parseTemplate(configuration, documentName, reader);
}
public final List parseFragment(final Configuration configuration, final String fragment) {
final String wrappedFragment = wrapFragment(fragment);
final Document document =
parseTemplate(
configuration,
null, // documentName
new StringReader(wrappedFragment));
return unwrapFragment(document);
}
protected abstract String wrapFragment(final String fragment);
protected abstract List unwrapFragment(final Document document);
/*
* This is defined in a class apart so that the classloader does not always try to load
* neko and xerces classes that might not be in the classpath.
*/
private static class NekoBasedHtmlParser {
// The org.apache.xerces.parsers.DOMParser is not used here as a type
// parameter to avoid the class loader to try to load this xerces class
// (and fail) before we control the error at the constructor.
private ResourcePool
© 2015 - 2025 Weber Informatics LLC | Privacy Policy