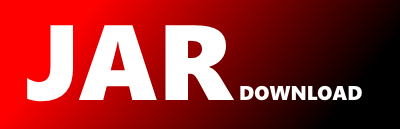
org.thymeleaf.util.ResourcePool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thymeleaf Show documentation
Show all versions of thymeleaf Show documentation
Modern server-side Java template engine for both web and standalone environments
package org.thymeleaf.util;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.Set;
import java.util.concurrent.Semaphore;
/**
*
* Represents a limited set or pool of resources, which needs to be used with
* exclusive ownership.
*
*
*
* Should be used in a similar fashion to the following so that resources
* are always released properly:
*
*
*
* MyResource resource = myLimitedResource.allocate();
* try {
* // actual code using the resource
* } finally {
* myLimitedResource.release(resource);
* }
*
*
* @since 2.0.0
*
* @author Guven Demir
*
*/
public final class ResourcePool {
private final LinkedList pool;
private final Set allocated;
private final Semaphore semaphore;
public ResourcePool(final Collection resources) {
super();
this.pool = new LinkedList(resources);
this.allocated = new HashSet(this.pool.size() + 1, 1.0f);
this.semaphore = new Semaphore(this.pool.size());
}
/**
*
* Allocates and returns a resource from the pool.
*
*
*
* Blocks until a resource is available when a resource is not
* available immediately.
*
*
* @return the allocated resource, heving been removed from the allocation pool.
*/
public T allocate() {
try {
this.semaphore.acquire();
} catch(InterruptedException e) {
throw new RuntimeException(e);
}
synchronized(this) {
final T object = this.pool.removeFirst();
this.allocated.add(object);
return object;
}
}
/**
*
* Releases a previously allocated resource.
*
*
*
* Might also be used to introduce new resources, e.g. in place of
* a broken resource.
*
*
*/
public void release(final T resource) {
synchronized(this) {
if (this.allocated.contains(resource)) {
this.pool.addLast(resource);
this.allocated.remove(resource);
}
}
this.semaphore.release();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy