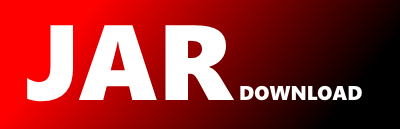
org.thymeleaf.standard.fragment.StandardFragmentSignatureNodeReferenceChecker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thymeleaf Show documentation
Show all versions of thymeleaf Show documentation
Modern server-side Java template engine for both web and standalone environments
/*
* =============================================================================
*
* Copyright (c) 2011-2014, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.standard.fragment;
import org.thymeleaf.Configuration;
import org.thymeleaf.dom.Attribute;
import org.thymeleaf.dom.DOMSelector;
import org.thymeleaf.dom.NestableAttributeHolderNode;
import org.thymeleaf.dom.Node;
import org.thymeleaf.standard.expression.FragmentSignature;
import org.thymeleaf.standard.expression.FragmentSignatureUtils;
import org.thymeleaf.util.Validate;
/**
*
* Implementation of the {@link org.thymeleaf.dom.DOMSelector.INodeReferenceChecker} interface used for looking
* for standard fragment signature attributes in nodes, and consider the names of the fragments to be
* reference values.
*
*
* For example, if the standard fragment signature attribute is th:fragment (which is the default), objects
* of this class will consider that DOM Selector expressions like myfrag or %myfrag will match
* th:fragment="myfrag(param1, param2)".
*
*
* @author Daniel Fernández
*
* @since 2.1.0
*
*/
public final class StandardFragmentSignatureNodeReferenceChecker extends DOMSelector.AbstractNodeReferenceChecker {
private final Configuration configuration;
private final String dialectPrefix;
private final String fragmentAttributeName;
public StandardFragmentSignatureNodeReferenceChecker(
final Configuration configuration, final String dialectPrefix, final String fragmentAttributeName) {
super();
Validate.notNull(configuration, "Configuration cannot be null");
Validate.notNull(fragmentAttributeName, "Fragment attribute name cannot be null");
this.configuration = configuration;
this.dialectPrefix = dialectPrefix;
this.fragmentAttributeName = Attribute.normalizeAttributeName(fragmentAttributeName);
}
public Configuration getConfiguration() {
return this.configuration;
}
public String getDialectPrefix() {
return this.dialectPrefix;
}
public String getFragmentAttributeName() {
return this.fragmentAttributeName;
}
public boolean checkReference(final Node node, final String referenceValue) {
if (referenceValue == null) {
return true;
}
if (node instanceof NestableAttributeHolderNode) {
final NestableAttributeHolderNode attributeHolderNode = (NestableAttributeHolderNode) node;
if (attributeHolderNode.hasNormalizedAttribute(this.dialectPrefix, this.fragmentAttributeName)) {
final String elementAttrValue =
attributeHolderNode.getAttributeValueFromNormalizedName(this.dialectPrefix, this.fragmentAttributeName);
if (elementAttrValue != null) {
final FragmentSignature fragmentSignature =
FragmentSignatureUtils.parseFragmentSignature(this.configuration, elementAttrValue);
if (fragmentSignature != null) {
final String signatureFragmentName = fragmentSignature.getFragmentName();
if (referenceValue.equals(signatureFragmentName)) {
return true;
}
}
}
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy