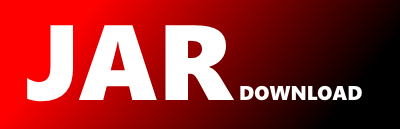
org.thymeleaf.model.IModelFactory Maven / Gradle / Ivy
Show all versions of thymeleaf Show documentation
/*
* =============================================================================
*
* Copyright (c) 2011-2018, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.model;
import java.util.Map;
import org.thymeleaf.context.ITemplateContext;
import org.thymeleaf.engine.AttributeName;
import org.thymeleaf.engine.TemplateData;
/**
*
* Interface defining model factories.
*
*
* A Model Factory can be obtained at custom processor artifacts by means of
* {@link ITemplateContext#getModelFactory()}, and then used for creating and modifying models
* ({@link IModel}) and events ({@link ITemplateEvent}.
*
*
* The {@link org.thymeleaf.engine.StandardModelFactory} implementation will be used by default.
*
*
* @see IModel
* @see ITemplateEvent
* @see org.thymeleaf.engine.StandardModelFactory
* @author Daniel Fernández
* @since 3.0.0
*
*/
public interface IModelFactory {
/**
*
* Creates a new, empty model.
*
*
* @return the new model.
*/
public IModel createModel();
/**
*
* Creates a new model containing only one event (initially).
*
*
* @param event the event to be put into the newly created model.
* @return the new model.
*/
public IModel createModel(final ITemplateEvent event);
/**
*
* Parse the template specified as String and return the result as a model.
*
*
* Note the {@code template} argument specified here is the template content itself, not a name to be resolved
* by a template resolver. The {@code ownerTemplate} argument is mandatory, and specifies the template that
* is being processed and as a part of which process the String template is being parsed.
*
*
* @param ownerTemplate the template being processed, for which the String template is being parsed
* into a model object.
* @param template the String containing the contents of the template to be parsed.
* @return the {@link IModel} representing the parsed template.
*/
public IModel parse(final TemplateData ownerTemplate, final String template);
/**
*
* Create a new CDATA Section event, containing the specified contents.
*
*
* @param content the content (i.e. without prefix or suffix) of the new CDATA Section.
* @return the new CDATA Section.
*/
public ICDATASection createCDATASection(final CharSequence content);
/**
*
* Create a new Comment event, containing the specified contents.
*
*
* @param content the content (i.e. without prefix or suffix) of the new Comment.
* @return the new Comment.
*/
public IComment createComment(final CharSequence content);
/**
*
* Create a DOCTYPE clause event for HTML5 (no type, no public or system id).
*
*
* @return the new DOCTYPE, corresponding with an HTML5 DOCTYPE clause.
*/
public IDocType createHTML5DocType();
/**
*
* Create a DOCTYPE clause event with the specified public ID and system ID.
*
*
* @param publicId the public ID to be applied (might be null).
* @param systemId the system ID to be applied (might be null if public ID is also null).
* @return the new DOCTPYE.
*/
public IDocType createDocType(final String publicId, final String systemId);
/**
*
* Create a DOCTYPE clause event, specifying all its components.
*
*
* @param keyword the keyword value (should be {@code DOCTYPE}, but case might vary).
* @param elementName the root element name.
* @param publicId the public ID (might be null).
* @param systemId the system ID (might be null).
* @param internalSubset the internal subset (might be null).
* @return the new DOCTYPE.
*/
public IDocType createDocType(
final String keyword,
final String elementName,
final String publicId,
final String systemId,
final String internalSubset);
/**
*
* Create a new Processing Instruction event, specifying its target and content.
*
*
* @param target the target value.
* @param content the content value.
* @return the new Processing Instruction.
*/
public IProcessingInstruction createProcessingInstruction(final String target, final String content);
/**
*
* Create a new Text event, specifying its contents.
*
*
* @param text the text contents.
* @return the new Text.
*/
public IText createText(final CharSequence text);
/**
*
* Create a new XML Declaration event, specifying values for all its attributes.
*
*
* @param version the version value (might be null).
* @param encoding the encoding value (might be null).
* @param standalone the standalone value (might be null).
* @return the new XML Declaration.
*/
public IXMLDeclaration createXMLDeclaration(final String version, final String encoding, final String standalone);
/**
*
* Create a new standalone element tag, non synthetic and minimized.
*
*
* This is equivalent to calling {@link #createStandaloneElementTag(String, boolean, boolean)} with
* {@code false} as a value for {@code synthetic} and {@code true} as a value for {@code minimized}.
*
*
* @param elementName the element name.
* @return the standalone tag.
*/
public IStandaloneElementTag createStandaloneElementTag(final String elementName);
/**
*
* Create a new standalone element tag, non synthetic and minimized, specifying one attribute.
*
*
* This is equivalent to calling {@link #createStandaloneElementTag(String, String, String, boolean, boolean)} with
* {@code false} as a value for {@code synthetic} and {@code true} as a value for {@code minimized}.
*
*
* @param elementName the element name.
* @param attributeName the name of the attribute to be added to the tag.
* @param attributeValue the value of the attribute to be added to the tag.
* @return the standalone tag.
*/
public IStandaloneElementTag createStandaloneElementTag(final String elementName, final String attributeName, final String attributeValue);
/**
*
* Create a new standalone element tag.
*
*
* @param elementName the element name.
* @param synthetic whether the tag is synthetic or not. Synthetic tags are used for balancing of markup and
* will not appear on output.
* @param minimized whether the tag is minimized or not, i.e. whether it ends in {@code />} or simply
* {@code >}.
* @return the standalone tag.
*/
public IStandaloneElementTag createStandaloneElementTag(final String elementName, final boolean synthetic, final boolean minimized);
/**
*
* Create a new standalone element tag, specifying one attribute.
*
*
* @param elementName the element name.
* @param attributeName the name of the attribute to be added to the tag.
* @param attributeValue the value of the attribute to be added to the tag.
* @param synthetic whether the tag is synthetic or not. Synthetic tags are used for balancing of markup and
* will not appear on output.
* @param minimized whether the tag is minimized or not, i.e. whether it ends in {@code />} or simply
* {@code >}.
* @return the standalone tag.
*/
public IStandaloneElementTag createStandaloneElementTag(final String elementName, final String attributeName, final String attributeValue, final boolean synthetic, final boolean minimized);
/**
*
* Create a new standalone element tag, specifying several attributes.
*
*
* @param elementName the element name.
* @param attributes the map of attribute names and values.
* @param attributeValueQuotes the type of quotes to be used for representing the attribute values.
* @param synthetic whether the tag is synthetic or not. Synthetic tags are used for balancing of markup and
* will not appear on output.
* @param minimized whether the tag is minimized or not, i.e. whether it ends in {@code />} or simply
* {@code >}.
* @return the standalone tag.
*/
public IStandaloneElementTag createStandaloneElementTag(final String elementName, final Map attributes, final AttributeValueQuotes attributeValueQuotes, final boolean synthetic, final boolean minimized);
/**
*
* Create a new open element tag, non-synthetic.
*
*
* This is equivalent to calling {@link #createOpenElementTag(String, boolean)} with
* {@code false} as a value for {@code synthetic}.
*
*
* @param elementName the element name.
* @return the open tag.
*/
public IOpenElementTag createOpenElementTag(final String elementName);
/**
*
* Create a new open element tag, non-synthetic, specifying one attribute.
*
*
* This is equivalent to calling {@link #createOpenElementTag(String, String, String, boolean)} with
* {@code false} as a value for {@code synthetic}.
*
*
* @param elementName the element name.
* @param attributeName the name of the attribute to be added to the tag.
* @param attributeValue the value of the attribute to be added to the tag.
* @return the open tag.
*/
public IOpenElementTag createOpenElementTag(final String elementName, final String attributeName, final String attributeValue);
/**
*
* Create a new open element tag.
*
*
* @param elementName the element name.
* @param synthetic whether the tag is synthetic or not. Synthetic tags are used for balancing of markup and
* will not appear on output.
* @return the open tag.
*/
public IOpenElementTag createOpenElementTag(final String elementName, final boolean synthetic);
/**
*
* Create a new open element tag, specifying one attribute.
*
*
* @param elementName the element name.
* @param attributeName the name of the attribute to be added to the tag.
* @param attributeValue the value of the attribute to be added to the tag.
* @param synthetic whether the tag is synthetic or not. Synthetic tags are used for balancing of markup and
* will not appear on output.
* @return the open tag.
*/
public IOpenElementTag createOpenElementTag(final String elementName, final String attributeName, final String attributeValue, final boolean synthetic);
/**
*
* Create a new open element tag, specifying several attributes.
*
*
* @param elementName the element name.
* @param attributes the map of attribute names and values.
* @param attributeValueQuotes the type of quotes to be used for representing the attribute values.
* @param synthetic whether the tag is synthetic or not. Synthetic tags are used for balancing of markup and
* will not appear on output.
* @return the open tag.
*/
public IOpenElementTag createOpenElementTag(final String elementName, final Map attributes, final AttributeValueQuotes attributeValueQuotes, final boolean synthetic);
/**
*
* Create a new close tag, non-synthetic and non-unmatched.
*
*
* This is equivalent to calling {@link #createCloseElementTag(String, boolean, boolean)} with
* {@code false} as a value for {@code synthetic} and also {@code false} as a value for {@code unmatched}.
*
*
* @param elementName the element name.
* @return the close tag.
*/
public ICloseElementTag createCloseElementTag(final String elementName);
/**
*
* Create a new close tag.
*
*
* @param elementName the element name.
* @param synthetic whether the tag is synthetic or not. Synthetic tags are used for balancing of markup and
* will not appear on output.
* @param unmatched whether this tag should be considered unmatched, i.e. there is no corresponding
* previous open tag for it.
* @return the close tag.
*/
public ICloseElementTag createCloseElementTag(final String elementName, final boolean synthetic, final boolean unmatched);
/**
*
* Create a new tag object that adds a new attribute to the existing ones in a specified tag.
*
*
* Note that this method should create a new object because {@link ITemplateEvent} implementations are immutable.
* Also, the created tag will be of the same type (i.e. standalone or open) as the specified as argument.
*
*
* @param tag the original tag.
* @param attributeName the name of the attribute to be added.
* @param attributeValue the value of the attribute to be added.
* @param the type of the original and new tags.
* @return the new tag.
*/
public T setAttribute(final T tag, final String attributeName, final String attributeValue);
/**
*
* Create a new tag object that adds a new attribute to the existing ones in a specified tag, also specifying the
* type of quotes to be used for representing the attribute value.
*
*
* Note that this method should create a new object because {@link ITemplateEvent} implementations are immutable.
* Also, the created tag will be of the same type (i.e. standalone or open) as the specified as argument.
*
*
* @param tag the original tag.
* @param attributeName the name of the attribute to be added.
* @param attributeValue the value of the attribute to be added.
* @param attributeValueQuotes the type of quotes to be used for representing the attribute value.
* @param the type of the original and new tags.
* @return the new tag.
*/
public T setAttribute(final T tag, final String attributeName, final String attributeValue, final AttributeValueQuotes attributeValueQuotes);
/**
*
* Create a new tag object replacing an attribute in the original tag with another one.
*
*
* Note that this method should create a new object because {@link ITemplateEvent} implementations are immutable.
* Also, the created tag will be of the same type (i.e. standalone or open) as the specified as argument.
*
*
* If the attribute being replaced does not exist, the new one will be created as if
* {@link #setAttribute(IProcessableElementTag, String, String)} was called instead. If the old attribute does
* exist, its position in the tag as well as its quote type will be used.
*
*
* @param tag the original tag.
* @param oldAttributeName the name of the attribute to be replaced.
* @param attributeName the name of the attribute to be added.
* @param attributeValue the value of the attribute to be added.
* @param the type of the original and new tags.
* @return the new tag.
*/
public T replaceAttribute(final T tag, final AttributeName oldAttributeName, final String attributeName, final String attributeValue);
/**
*
* Create a new tag object replacing an attribute in the original tag with another one, also specifying the
* type of quotes to be used for representing the attribute value.
*
*
* Note that this method should create a new object because {@link ITemplateEvent} implementations are immutable.
* Also, the created tag will be of the same type (i.e. standalone or open) as the specified as argument.
*
*
* If the attribute being replaced does not exist, the new one will be created as if
* {@link #setAttribute(IProcessableElementTag, String, String)} was called instead. If the old attribute does
* exist, its position in the tag will be used.
*
*
* @param tag the original tag.
* @param oldAttributeName the name of the attribute to be replaced.
* @param attributeName the name of the attribute to be added.
* @param attributeValue the value of the attribute to be added.
* @param attributeValueQuotes the type of quotes to be used for representing the attribute value.
* @param the type of the original and new tags.
* @return the new tag.
*/
public T replaceAttribute(final T tag, final AttributeName oldAttributeName, final String attributeName, final String attributeValue, final AttributeValueQuotes attributeValueQuotes);
/**
*
* Create a new tag removing an existing attribute.
*
*
* Note that this method should create a new object because {@link ITemplateEvent} implementations are immutable.
* Also, the created tag will be of the same type (i.e. standalone or open) as the specified as argument.
*
*
* If the attribute being removed does not exist, nothing will be done and the same tag object will be returned.
*
*
* @param tag the original tag.
* @param attributeName the name of the attribute to be removed.
* @param the type of the original and new tags.
* @return the new tag.
*/
public T removeAttribute(final T tag, final String attributeName);
/**
*
* Create a new tag removing an existing attribute.
*
*
* Note that this method should create a new object because {@link ITemplateEvent} implementations are immutable.
* Also, the created tag will be of the same type (i.e. standalone or open) as the specified as argument.
*
*
* If the attribute being removed does not exist, nothing will be done and the same tag object will be returned.
*
*
* @param tag the original tag.
* @param prefix the prefix of the attribute (might be null).
* @param name the name of the attribute.
* @param the type of the original and new tags.
* @return the new tag.
*/
public T removeAttribute(final T tag, final String prefix, final String name);
/**
*
* Create a new tag removing an existing attribute.
*
*
* Note that this method should create a new object because {@link ITemplateEvent} implementations are immutable.
* Also, the created tag will be of the same type (i.e. standalone or open) as the specified as argument.
*
*
* If the attribute being removed does not exist, nothing will be done and the same tag object will be returned.
*
*
* @param tag the original tag.
* @param attributeName the attribute name.
* @param the type of the original and new tags.
* @return the new tag.
*/
public T removeAttribute(final T tag, final AttributeName attributeName);
}