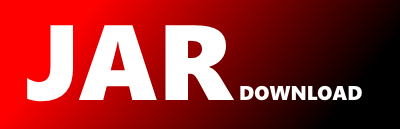
org.thymeleaf.expression.Strings Maven / Gradle / Ivy
Show all versions of thymeleaf Show documentation
/*
* =============================================================================
*
* Copyright (c) 2011-2016, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.expression;
import java.util.ArrayList;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Locale;
import java.util.Set;
import org.thymeleaf.util.StringUtils;
import org.thymeleaf.util.Validate;
/**
*
* Expression Object for performing String-related operations inside Thymeleaf Standard Expressions.
*
*
* An object of this class is usually available in variable evaluation expressions with the name
* #strings.
*
*
* @author Daniel Fernández
* @author Bernard Le Roux
*
* @since 1.0
*
*/
public final class Strings {
private final Locale locale;
public Strings(final Locale locale) {
super();
this.locale = locale;
}
/**
*
* Performs a null-safe toString() operation.
*
*
* @param target the object on which toString will be executed
* @return the result of calling target.toString() if target is not null,
* null if target is null.
* @since 2.0.12
*/
public String toString(final Object target) {
if (target == null) {
return null;
}
return StringUtils.toString(target);
}
/**
*
* Performs a null-safe toString() operation on each
* element of the array.
*
*
* @param target the array of objects on which toString will be executed
* @return for each element: the result of calling target.toString()
* if target is not null, null if target is null.
* @since 2.0.12
*/
public String[] arrayToString(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = toString(target[i]);
}
return result;
}
/**
*
* Performs a null-safe toString() operation on each
* element of the list.
*
*
* @param target the list of objects on which toString will be executed
* @return for each element: the result of calling target.toString()
* if target is not null, null if target is null.
* @since 2.0.12
*/
public List listToString(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(toString(element));
}
return result;
}
/**
*
* Performs a null-safe toString() operation on each
* element of the set.
*
*
* @param target the set of objects on which toString will be executed
* @return for each element: the result of calling target.toString()
* if target is not null, null if target is null.
* @since 2.0.12
*/
public Set setToString(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(toString(element));
}
return result;
}
public String abbreviate(final Object target, final int maxSize) {
if (target == null) {
return null;
}
return StringUtils.abbreviate(target, maxSize);
}
public String[] arrayAbbreviate(final Object[] target, final int maxSize) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = abbreviate(target[i], maxSize);
}
return result;
}
public List listAbbreviate(final List> target, final int maxSize) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(abbreviate(element, maxSize));
}
return result;
}
public Set setAbbreviate(final Set> target, final int maxSize) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(abbreviate(element, maxSize));
}
return result;
}
/**
*
* @param first first
* @param second second
* @return the result
* @since 2.0.16
*/
public Boolean equals(final Object first, final Object second) {
return StringUtils.equals(first, second);
}
/**
*
* @param first first
* @param second second
* @return the result
* @since 2.0.16
*/
public Boolean equalsIgnoreCase(final Object first, final Object second) {
return StringUtils.equalsIgnoreCase(first, second);
}
public Boolean contains(final Object target, final String fragment) {
return StringUtils.contains(target, fragment);
}
public Boolean[] arrayContains(final Object[] target, final String fragment) {
if (target == null) {
return null;
}
final Boolean[] result = new Boolean[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = contains(target[i], fragment);
}
return result;
}
public List listContains(final List> target, final String fragment) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(contains(element, fragment));
}
return result;
}
public Set setContains(final Set> target, final String fragment) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(contains(element, fragment));
}
return result;
}
public Boolean containsIgnoreCase(final Object target, final String fragment) {
return StringUtils.containsIgnoreCase(target, fragment, this.locale);
}
public Boolean[] arrayContainsIgnoreCase(final Object[] target, final String fragment) {
if (target == null) {
return null;
}
final Boolean[] result = new Boolean[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = containsIgnoreCase(target[i], fragment);
}
return result;
}
public List listContainsIgnoreCase(final List> target, final String fragment) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(containsIgnoreCase(element, fragment));
}
return result;
}
public Set setContainsIgnoreCase(final Set> target, final String fragment) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(containsIgnoreCase(element, fragment));
}
return result;
}
public Boolean startsWith(final Object target, final String prefix) {
return StringUtils.startsWith(target, prefix);
}
public Boolean[] arrayStartsWith(final Object[] target, final String prefix) {
if (target == null) {
return null;
}
final Boolean[] result = new Boolean[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = startsWith(target[i], prefix);
}
return result;
}
public List listStartsWith(final List> target, final String prefix) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(startsWith(element, prefix));
}
return result;
}
public Set setStartsWith(final Set> target, final String prefix) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(startsWith(element, prefix));
}
return result;
}
public Boolean endsWith(final Object target, final String suffix) {
return StringUtils.endsWith(target, suffix);
}
public Boolean[] arrayEndsWith(final Object[] target, final String suffix) {
if (target == null) {
return null;
}
final Boolean[] result = new Boolean[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = endsWith(target[i], suffix);
}
return result;
}
public List listEndsWith(final List> target, final String suffix) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(endsWith(element, suffix));
}
return result;
}
public Set setEndsWith(final Set> target, final String suffix) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(endsWith(element, suffix));
}
return result;
}
public String substring(final Object target, final int start, final int end) {
if (target == null) {
return null;
}
return StringUtils.substring(target, start, end);
}
public String[] arraySubstring(final Object[] target, final int start, final int end) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = substring(target[i], start, end);
}
return result;
}
public List listSubstring(final List> target, final int start, final int end) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(substring(element, start, end));
}
return result;
}
public Set setSubstring(final Set> target, final int start, final int end) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(substring(element, start, end));
}
return result;
}
/**
*
* copy a part of target start beginIndex to the end of target.
* If non-String object, toString() will be called.
*
* @param target source of the copy.
* @param start index where the copy start.
*
* @return part of target, or null if target is null.
*
* @since 1.1.2
*
*/
public String substring(final Object target, final int start) {
if (target == null) {
return null;
}
return StringUtils.substring(target, start);
}
/**
*
* copy a part of target start beginIndex to the end of target
* for all the elements in the target array.
* If non-String object, toString() will be called.
*
* @param target source of the copy.
* @param start index where the copy start.
*
* @return part of target, or null if target is null.
*
* @since 1.1.2
*
*/
public String[] arraySubstring(final Object[] target, final int start) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = substring(target[i], start);
}
return result;
}
/**
*
* copy a part of target start beginIndex to the end of target
* for all the elements in the target list.
* If non-String object, toString() will be called.
*
* @param target source of the copy.
* @param start index where the copy start.
*
* @return part of target, or null if target is null.
*
* @since 1.1.2
*
*/
public List listSubstring(final List> target, final int start) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(substring(element, start));
}
return result;
}
/**
*
* copy a part of target start beginIndex to the end of target
* for all the elements in the target set.
* If non-String object, toString() will be called.
*
* @param target source of the copy.
* @param start index where the copy start.
*
* @return part of target, or null if target is null.
*
* @since 1.1.2
*
*/
public Set setSubstring(final Set> target, final int start) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(substring(element, start));
}
return result;
}
public String substringAfter(final Object target, final String substr) {
if (target == null) {
return null;
}
return StringUtils.substringAfter(target, substr);
}
public String[] arraySubstringAfter(final Object[] target, final String substr) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = substringAfter(target[i], substr);
}
return result;
}
public List listSubstringAfter(final List> target, final String substr) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(substringAfter(element, substr));
}
return result;
}
public Set setSubstringAfter(final Set> target, final String substr) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(substringAfter(element, substr));
}
return result;
}
public String substringBefore(final Object target, final String substr) {
if (target == null) {
return null;
}
return StringUtils.substringBefore(target, substr);
}
public String[] arraySubstringBefore(final Object[] target, final String substr) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = substringBefore(target[i], substr);
}
return result;
}
public List listSubstringBefore(final List> target, final String substr) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(substringBefore(element, substr));
}
return result;
}
public Set setSubstringBefore(final Set> target, final String substr) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(substringBefore(element, substr));
}
return result;
}
public String prepend(final Object target, final String prefix) {
if (target == null) {
return null;
}
return StringUtils.prepend(target, prefix);
}
public String[] arrayPrepend(final Object[] target, final String prefix) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = prepend(target[i], prefix);
}
return result;
}
public List listPrepend(final List> target, final String prefix) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(prepend(element, prefix));
}
return result;
}
public Set setPrepend(final Set> target, final String prefix) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(prepend(element, prefix));
}
return result;
}
/**
*
* @param target target
* @param times times
* @return the result
* @since 2.1.0
*/
public String repeat(final Object target, final int times) {
if (target == null) {
return null;
}
return StringUtils.repeat(target, times);
}
public String append(final Object target, final String suffix) {
if (target == null) {
return null;
}
return StringUtils.append(target, suffix);
}
/**
*
* @param values values
* @return the result
* @since 2.0.16
*/
public String concat(final Object ... values) {
return StringUtils.concat(values);
}
/**
*
* @param nullValue nullValue
* @param values values
* @return the result
* @since 2.0.16
*/
public String concatReplaceNulls(final String nullValue, final Object ... values) {
return StringUtils.concatReplaceNulls(nullValue, values);
}
public String[] arrayAppend(final Object[] target, final String suffix) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = append(target[i], suffix);
}
return result;
}
public List listAppend(final List> target, final String suffix) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(append(element, suffix));
}
return result;
}
public Set setAppend(final Set> target, final String suffix) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(append(element, suffix));
}
return result;
}
public Integer indexOf(final Object target, final String fragment) {
return StringUtils.indexOf(target, fragment);
}
public Integer[] arrayIndexOf(final Object[] target, final String fragment) {
if (target == null) {
return null;
}
final Integer[] result = new Integer[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = indexOf(target[i], fragment);
}
return result;
}
public List listIndexOf(final List> target, final String fragment) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(indexOf(element, fragment));
}
return result;
}
public Set setIndexOf(final Set> target, final String fragment) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(indexOf(element, fragment));
}
return result;
}
public Boolean isEmpty(final Object target) {
return Boolean.valueOf(target == null || StringUtils.isEmptyOrWhitespace(target.toString()));
}
public Boolean[] arrayIsEmpty(final Object[] target) {
if (target == null) {
return null;
}
final Boolean[] result = new Boolean[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = isEmpty(target[i]);
}
return result;
}
public List listIsEmpty(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(isEmpty(element));
}
return result;
}
public Set setIsEmpty(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(isEmpty(element));
}
return result;
}
public String arrayJoin(final Object[] stringArray, final String separator) {
if (stringArray == null) {
return null;
}
return StringUtils.join(stringArray, separator);
}
public String listJoin(final List> stringIter, final String separator) {
if (stringIter == null) {
return null;
}
return StringUtils.join(stringIter, separator);
}
public String setJoin(final Set> stringIter, final String separator) {
if (stringIter == null) {
return null;
}
return StringUtils.join(stringIter, separator);
}
public String[] arraySplit(final Object target, final String separator) {
if (target == null) {
return null;
}
return StringUtils.split(target, separator);
}
public List listSplit(final Object target, final String separator) {
if (target == null) {
return null;
}
return new ArrayList(java.util.Arrays.asList(StringUtils.split(target, separator)));
}
public Set setSplit(final Object target, final String separator) {
if (target == null) {
return null;
}
return new LinkedHashSet(java.util.Arrays.asList(StringUtils.split(target, separator)));
}
public Integer length(final Object target) {
return StringUtils.length(target);
}
public Integer[] arrayLength(final Object[] target) {
if (target == null) {
return null;
}
final Integer[] result = new Integer[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = length(target[i]);
}
return result;
}
public List listLength(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(length(element));
}
return result;
}
public Set setLength(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(length(element));
}
return result;
}
public String replace(final Object target, final String before, final String after) {
if (target == null) {
return null;
}
return StringUtils.replace(target, before, after);
}
public String[] arrayReplace(final Object[] target, final String before, final String after) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = replace(target[i], before, after);
}
return result;
}
public List listReplace(final List> target, final String before, final String after) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(replace(element, before, after));
}
return result;
}
public Set setReplace(final Set> target, final String before, final String after) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(replace(element, before, after));
}
return result;
}
public String multipleReplace(final Object target,
final String[] before, final String[] after) {
Validate.notNull(before, "Array of 'before' values cannot be null");
Validate.notNull(after, "Array of 'after' values cannot be null");
Validate.isTrue(before.length == after.length,
"Arrays of 'before' and 'after' values must have the same length");
if (target == null) {
return null;
}
String ret = target.toString();
for (int i = 0; i < before.length; i++) {
ret = StringUtils.replace(ret, before[i], after[i]);
}
return ret;
}
public String[] arrayMultipleReplace(final Object[] target,
final String[] before, final String[] after) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = multipleReplace(target[i], before, after);
}
return result;
}
public List listMultipleReplace(final List> target,
final String[] before, final String[] after) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(multipleReplace(element, before, after));
}
return result;
}
public Set setMultipleReplace(final Set> target, final String[] before,
final String[] after) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(multipleReplace(element, before, after));
}
return result;
}
public String toUpperCase(final Object target) {
if (target == null) {
return null;
}
return StringUtils.toUpperCase(target, this.locale);
}
public String[] arrayToUpperCase(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = toUpperCase(target[i]);
}
return result;
}
public List listToUpperCase(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(toUpperCase(element));
}
return result;
}
public Set setToUpperCase(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(toUpperCase(element));
}
return result;
}
public String toLowerCase(final Object target) {
if (target == null) {
return null;
}
return StringUtils.toLowerCase(target, this.locale);
}
public String[] arrayToLowerCase(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = toLowerCase(target[i]);
}
return result;
}
public List listToLowerCase(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(toLowerCase(element));
}
return result;
}
public Set setToLowerCase(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(toLowerCase(element));
}
return result;
}
public String trim(final Object target) {
if (target == null) {
return null;
}
return StringUtils.trim(target);
}
public String[] arrayTrim(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = trim(target[i]);
}
return result;
}
public List listTrim(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(trim(element));
}
return result;
}
public Set setTrim(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(trim(element));
}
return result;
}
/**
*
* Convert the first letter of target to uppercase (title-case, in fact).
*
*
* @param target the String to be capitalized.
* If non-String object, toString() will be called.
* @return String the result of capitalizing the target.
*
* @since 1.1.2
*
*/
public String capitalize(final Object target) {
if (target == null) {
return null;
}
return StringUtils.capitalize(target);
}
/**
*
* Convert the first letter into uppercase (title-case, in fact) for
* all the elements in the target array.
*
*
* @param target the array of Strings to be capitalized.
* If non-String objects, toString() will be called.
* @return a String[] with the result of capitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public String[] arrayCapitalize(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = capitalize(target[i]);
}
return result;
}
/**
*
* Convert the first letter into uppercase (title-case, in fact) for
* all the elements in the target list.
*
*
* @param target the list of Strings to be capitalized.
* If non-String objects, toString() will be called.
* @return a List with the result of capitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public List listCapitalize(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(capitalize(element));
}
return result;
}
/**
*
* Convert the first letter into uppercase (title-case, in fact) for
* all the elements in the target set.
*
*
* @param target the set of Strings to be capitalized.
* If non-String objects, toString() will be called.
* @return a Set with the result of capitalizing each element of the target.
*
* @since 1.1.2
*
*/
public Set setCapitalize(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(capitalize(element));
}
return result;
}
/**
*
* Convert the first letter of target to lowercase.
*
*
* @param target the String to be uncapitalized.
* If non-String object, toString() will be called.
*
* @return String the result of uncapitalizing the target.
*
* @since 1.1.2
*
*/
public String unCapitalize(final Object target) {
if (target == null) {
return null;
}
return StringUtils.unCapitalize(target);
}
/**
*
* Convert the first letter into lowercase for
* all the elements in the target array.
*
*
* @param target the array of Strings to be uncapitalized.
* If non-String objects, toString() will be called.
*
* @return a String[] with the result of uncapitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public String[] arrayUnCapitalize(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = unCapitalize(target[i]);
}
return result;
}
/**
*
* Convert the first letter into lowercase for
* all the elements in the target list.
*
*
* @param target the list of Strings to be uncapitalized.
* If non-String objects, toString() will be called.
*
* @return a List with the result of uncapitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public List listUnCapitalize(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(unCapitalize(element));
}
return result;
}
/**
*
* Convert the first letter into lowercase for
* all the elements in the target set.
*
*
* @param target the set of Strings to be uncapitalized.
* If non-String objects, toString() will be called.
*
* @return a Set with the result of uncapitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public Set setUnCapitalize(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(unCapitalize(element));
}
return result;
}
/**
*
* Convert the first letter of each words of target
* to uppercase (title-case, in fact).
* The default delimiter characters between the words are
* the whitespace characters
* (see Characters.IsWhiteSpace method in the Java documentation).
*
*
* @param target the String to be capitalized.
* If non-String object, toString() will be called.
*
* @return String the result of capitalizing the target.
*
* @since 1.1.2
*
*/
public String capitalizeWords(final Object target) {
if (target == null) {
return null;
}
return StringUtils.capitalizeWords(target);
}
/**
*
* Convert the first letter of each words of target
* to uppercase (title-case, in fact) for
* all the elements in the target array.
* The default delimiter characters between the words are
* the whitespace characters
* (see Characters.IsWhiteSpace method in the Java documentation).
*
*
* @param target the array of Strings to be capitalized.
* If non-String objects, toString() will be called.
*
* @return a String[] with the result of capitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public String[] arrayCapitalizeWords(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = capitalizeWords(target[i]);
}
return result;
}
/**
*
* Convert the first letter of each words of target
* to uppercase (title-case, in fact) for
* all the elements in the target list.
* The default delimiter characters between the words are
* the whitespace characters
* (see Characters.IsWhiteSpace method in the Java documentation).
*
*
* @param target the list of Strings to be capitalized.
* If non-String objects, toString() will be called.
*
* @return a List with the result of capitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public List listCapitalizeWords(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(capitalizeWords(element));
}
return result;
}
/**
*
* Convert the first letter of each words of target
* to uppercase (title-case, in fact)
* for all the elements in the target set.
* The default delimiter characters between the words are
* the whitespace characters
* (see Characters.IsWhiteSpace method in the Java documentation).
*
*
* @param target the set of Strings to be capitalized.
* If non-String objects, toString() will be called.
*
* @return a Set with the result of capitalizing each element of the target.
*
* @since 1.1.2
*
*/
public Set setCapitalizeWords(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(capitalizeWords(element));
}
return result;
}
/**
*
* Convert the first letter of each words of target to uppercase
* (title-case, in fact), using the specified delimiter chars for determining
* word ends/starts.
*
*
* @param target the String to be capitalized.
* If non-String object, toString() will be called.
* @param delimiters the delimiters of the words.
* If non-String object, toString() will be called.
*
* @return String the result of capitalizing the target.
*
* @since 1.1.2
*
*/
public String capitalizeWords(final Object target,
final Object delimiters) {
if (target == null) {
return null;
}
return StringUtils.capitalizeWords(target, delimiters);
}
/**
*
* Convert the first letter of each words of target
* to uppercase (title-case, in fact) for
* all the elements in the target array.
* The specified delimiter chars will be used for determining
* word ends/starts.
*
*
* @param target the array of Strings to be capitalized.
* If non-String objects, toString() will be called.
* @param delimiters the delimiters of the words.
* If non-String object, toString() will be called.
*
* @return a String[] with the result of capitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public String[] arrayCapitalizeWords(final Object[] target,
final Object delimiters) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = capitalizeWords(target[i], delimiters);
}
return result;
}
/**
*
* Convert the first letter of each words of target
* to uppercase (title-case, in fact) for
* all the elements in the target list.
* The specified delimiter chars will be used for determining
* word ends/starts.
*
*
* @param target the list of Strings to be capitalized.
* If non-String objects, toString() will be called.
* @param delimiters the delimiters of the words.
* If non-String object, toString() will be called.
*
* @return a List with the result of capitalizing
* each element of the target.
*
* @since 1.1.2
*
*/
public List listCapitalizeWords(final List> target,
final Object delimiters) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(capitalizeWords(element, delimiters));
}
return result;
}
/**
*
* Convert the first letter of each words of target
* to uppercase (title-case, in fact) for
* all the elements in the target set.
* The specified delimiter chars will be used for determining
* word ends/starts.
*
*
* @param target the set of Strings to be capitalized.
* If non-String objects, toString() will be called.
* @param delimiters the delimiters of the words.
* If non-String object, toString()
*
* @return a Set with the result of capitalizing each element of the target.
*
* @since 1.1.2
*
*/
public Set setCapitalizeWords(final Set> target,
final Object delimiters) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(capitalizeWords(element, delimiters));
}
return result;
}
/**
*
* XML-escapes the specified text.
*
*
* @param target the text to be escaped
* @return the escaped text.
*
* @since 2.0.9
*/
public String escapeXml(final Object target) {
if (target == null) {
return null;
}
return StringUtils.escapeXml(target);
}
/**
*
* XML-escapes all the elements in the target array.
*
*
* @param target the array of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a String[] with the result of each
* each element of the target.
*
* @since 2.0.9
*
*/
public String[] arrayEscapeXml(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = escapeXml(target[i]);
}
return result;
}
/**
*
* XML-escapes all the elements in the target list.
*
*
* @param target the list of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a List with the result of each
* each element of the target.
*
* @since 2.0.9
*
*/
public List listEscapeXml(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(escapeXml(element));
}
return result;
}
/**
*
* XML-escapes all the elements in the target set.
*
*
* @param target the list of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a Set with the result of each
* each element of the target.
*
* @since 2.0.9
*
*/
public Set setEscapeXml(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(escapeXml(element));
}
return result;
}
/**
*
* JavaScript-escapes the specified text.
*
*
* @param target the text to be escaped
* @return the escaped text.
*
* @since 2.0.11
*/
public String escapeJavaScript(final Object target) {
if (target == null) {
return null;
}
return StringUtils.escapeJavaScript(target);
}
/**
*
* JavaScript-escapes all the elements in the target array.
*
*
* @param target the array of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a String[] with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public String[] arrayEscapeJavaScript(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = escapeJavaScript(target[i]);
}
return result;
}
/**
*
* JavaScript-escapes all the elements in the target list.
*
*
* @param target the list of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a List with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public List listEscapeJavaScript(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(escapeJavaScript(element));
}
return result;
}
/**
*
* JavaScript-escapes all the elements in the target set.
*
*
* @param target the list of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a Set with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public Set setEscapeJavaScript(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(escapeJavaScript(element));
}
return result;
}
/**
*
* JavaScript-unescapes the specified text.
*
*
* @param target the text to be unescaped
* @return the unescaped text.
*
* @since 2.0.11
*/
public String unescapeJavaScript(final Object target) {
if (target == null) {
return null;
}
return StringUtils.unescapeJavaScript(target);
}
/**
*
* JavaScript-unescapes all the elements in the target array.
*
*
* @param target the array of Strings to be unescaped.
* If non-String objects, toString() will be called.
*
* @return a String[] with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public String[] arrayUnescapeJavaScript(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = unescapeJavaScript(target[i]);
}
return result;
}
/**
*
* JavaScript-unescapes all the elements in the target list.
*
*
* @param target the list of Strings to be unescaped.
* If non-String objects, toString() will be called.
*
* @return a List with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public List listUnescapeJavaScript(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(unescapeJavaScript(element));
}
return result;
}
/**
*
* JavaScript-unescapes all the elements in the target set.
*
*
* @param target the list of Strings to be unescaped.
* If non-String objects, toString() will be called.
*
* @return a Set with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public Set setUnescapeJavaScript(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(unescapeJavaScript(element));
}
return result;
}
/**
*
* Java-escapes the specified text.
*
*
* @param target the text to be escaped
* @return the escaped text.
*
* @since 2.0.11
*/
public String escapeJava(final Object target) {
if (target == null) {
return null;
}
return StringUtils.escapeJava(target);
}
/**
*
* Java-escapes all the elements in the target array.
*
*
* @param target the array of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a String[] with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public String[] arrayEscapeJava(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = escapeJava(target[i]);
}
return result;
}
/**
*
* Java-escapes all the elements in the target list.
*
*
* @param target the list of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a List with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public List listEscapeJava(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(escapeJava(element));
}
return result;
}
/**
*
* Java-escapes all the elements in the target set.
*
*
* @param target the list of Strings to be escaped.
* If non-String objects, toString() will be called.
*
* @return a Set with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public Set setEscapeJava(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(escapeJava(element));
}
return result;
}
/**
*
* Java-unescapes the specified text.
*
*
* @param target the text to be unescaped
* @return the unescaped text.
*
* @since 2.0.11
*/
public String unescapeJava(final Object target) {
if (target == null) {
return null;
}
return StringUtils.unescapeJava(target);
}
/**
*
* Java-unescapes all the elements in the target array.
*
*
* @param target the array of Strings to be unescaped.
* If non-String objects, toString() will be called.
*
* @return a String[] with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public String[] arrayUnescapeJava(final Object[] target) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = unescapeJava(target[i]);
}
return result;
}
/**
*
* Java-unescapes all the elements in the target list.
*
*
* @param target the list of Strings to be unescaped.
* If non-String objects, toString() will be called.
*
* @return a List with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public List listUnescapeJava(final List> target) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(unescapeJava(element));
}
return result;
}
/**
*
* Java-unescapes all the elements in the target set.
*
*
* @param target the list of Strings to be unescaped.
* If non-String objects, toString() will be called.
*
* @return a Set with the result of each
* each element of the target.
*
* @since 2.0.11
*
*/
public Set setUnescapeJava(final Set> target) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(unescapeJava(element));
}
return result;
}
/**
*
* Builds a random String using characters 0..9 and A..Z.
*
*
* @param count length of the generated String
*
* @return a random String
*
* @since 2.1.0
*
*/
public String randomAlphanumeric(final int count) {
return StringUtils.randomAlphanumeric(count);
}
/**
*
* Checks if target text is empty and uses either target,
* or if the target is empty uses {@code defaultValue}.
*
*
* @param target value that to be checked if is null or empty
* If non-String objects, toString() will be called.
* @param defaultValue value to use if target is empty
* If non-String objects, toString() will be called.
*
* @return either target, or if the target is empty {@code defaultValue}
*
* @since 2.1.3
*/
public String defaultString(final Object target, final Object defaultValue) {
if (target == null) {
if (defaultValue == null) {
return "null";
}
return defaultValue.toString();
}
String targetString = target.toString();
if (StringUtils.isEmptyOrWhitespace(targetString)) {
if (defaultValue == null) {
return "null";
}
return defaultValue.toString();
}
return targetString;
}
/**
*
* Checks if each target element is empty and uses either target element,
* or if the target element is empty uses {@code defaultValue}.
*
*
* @param target the array of values that to be checked if is null or empty
* If non-String objects, toString() will be called.
* @param defaultValue value to return if target is empty
* If non-String objects, toString() will be called.
*
* @return a String[] with the result of {@link #defaultString(Object, Object)}
* for each element of the target.
*
* @since 2.1.3
*/
public String[] arrayDefaultString(final Object[] target, final Object defaultValue) {
if (target == null) {
return null;
}
final String[] result = new String[target.length];
for (int i = 0; i < target.length; i++) {
result[i] = defaultString(target[i], defaultValue);
}
return result;
}
/**
*
* Checks if each target element is empty and uses either target element,
* or if the target element is empty uses {@code defaultValue}.
*
*
* @param target the list of values that to be checked if is null or empty
* If non-String objects, toString() will be called.
* @param defaultValue value to return if target is empty
* If non-String objects, toString() will be called.
*
* @return a {@code List} with the result of
* {@link #defaultString(Object, Object)} for each element of the target.
*
* @since 2.1.3
*/
public List listDefaultString(final List> target, final Object defaultValue) {
if (target == null) {
return null;
}
final List result = new ArrayList(target.size() + 2);
for (final Object element : target) {
result.add(defaultString(element, defaultValue));
}
return result;
}
/**
*
* Checks if each target element is empty and uses either target element,
* or if the target element is empty uses {@code defaultValue}.
*
*
* @param target the set of values that to be checked if is null or empty
* If non-String objects, toString() will be called.
* @param defaultValue value to return if target is empty
* If non-String objects, toString() will be called.
*
* @return a {@code Set} with the result of
* {@link #defaultString(Object, Object)} for each element of the target.
*
* @since 2.1.3
*/
public Set setDefaultString(final Set> target, final Object defaultValue) {
if (target == null) {
return null;
}
final Set result = new LinkedHashSet(target.size() + 2);
for (final Object element : target) {
result.add(defaultString(element, defaultValue));
}
return result;
}
}