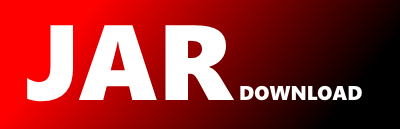
org.thymeleaf.templateresolver.AbstractConfigurableTemplateResolver Maven / Gradle / Ivy
Show all versions of thymeleaf Show documentation
/*
* =============================================================================
*
* Copyright (c) 2011-2016, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.templateresolver;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.thymeleaf.IEngineConfiguration;
import org.thymeleaf.cache.AlwaysValidCacheEntryValidity;
import org.thymeleaf.cache.ICacheEntryValidity;
import org.thymeleaf.cache.NonCacheableCacheEntryValidity;
import org.thymeleaf.cache.TTLCacheEntryValidity;
import org.thymeleaf.templatemode.TemplateMode;
import org.thymeleaf.templateresource.ITemplateResource;
import org.thymeleaf.util.ContentTypeUtils;
import org.thymeleaf.util.PatternSpec;
import org.thymeleaf.util.StringUtils;
import org.thymeleaf.util.Validate;
/**
*
* Abstract implementation of {@link ITemplateResolver} extending {@link AbstractTemplateResolver}
* and providing a large set of methods for configuring resource name (from template name), template mode,
* cache validity and character encoding.
*
*
* Unless overriden, this class will always apply the following validity to
* template resolutions:
*
*
* - If not cacheable: {@link NonCacheableCacheEntryValidity}.
* - If cacheable and TTL not set: {@link AlwaysValidCacheEntryValidity}.
* - If cacheable and TTL set: {@link TTLCacheEntryValidity}.
*
*
* @author Daniel Fernández
*
* @since 3.0.0
*
*/
public abstract class AbstractConfigurableTemplateResolver extends AbstractTemplateResolver {
/**
*
* Default template mode: {@link TemplateMode#HTML}
*
*/
public static final TemplateMode DEFAULT_TEMPLATE_MODE = TemplateMode.HTML;
/**
*
* Default value for the cacheable flag: {@value}.
*
*/
public static final boolean DEFAULT_CACHEABLE = true;
/**
*
* Default value for the cache TTL: null. This means the parsed template will live in
* cache until removed by LRU (because of being the oldest entry).
*
*/
public static final Long DEFAULT_CACHE_TTL_MS = null;
private String prefix = null;
private String suffix = null;
private boolean forceSuffix = false;
private String characterEncoding = null;
private TemplateMode templateMode = DEFAULT_TEMPLATE_MODE;
private boolean forceTemplateMode = false;
private boolean cacheable = DEFAULT_CACHEABLE;
private Long cacheTTLMs = DEFAULT_CACHE_TTL_MS;
private final HashMap templateAliases = new HashMap(8);
private final PatternSpec xmlTemplateModePatternSpec = new PatternSpec();
private final PatternSpec htmlTemplateModePatternSpec = new PatternSpec();
private final PatternSpec textTemplateModePatternSpec = new PatternSpec();
private final PatternSpec javaScriptTemplateModePatternSpec = new PatternSpec();
private final PatternSpec cssTemplateModePatternSpec = new PatternSpec();
private final PatternSpec rawTemplateModePatternSpec = new PatternSpec();
private final PatternSpec cacheablePatternSpec = new PatternSpec();
private final PatternSpec nonCacheablePatternSpec = new PatternSpec();
public AbstractConfigurableTemplateResolver() {
super();
}
/**
*
* Returns the (optional) prefix to be added to all template names in order
* to convert template names into resource names.
*
*
* @return the prefix.
*/
public final String getPrefix() {
return this.prefix;
}
/**
*
* Sets a new (optional) prefix to be added to all template names in order
* to convert template names into resource names.
*
*
* @param prefix the prefix to be set.
*/
public final void setPrefix(final String prefix) {
this.prefix = prefix;
}
/**
*
* Returns the (optional) suffix to be added to all template names in order
* to convert template names into resource names.
*
*
* Note that this suffix may not be applied to the template name if the template name
* already ends in a known file name suffix: .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt. If this behaviour needs to be overridden so
* that suffix is always applied, the {@link #setForceSuffix(boolean)} will need to be set.
*
*
* @return the suffix.
*/
public final String getSuffix() {
return this.suffix;
}
/**
*
* Sets a new (optional) suffix to be added to all template names in order
* to convert template names into resource names.
*
*
* Note that this suffix may not be applied to the template name if the template name
* already ends in a known file name suffix: .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt. If this behaviour needs to be overridden so
* that suffix is always applied, the {@link #setForceSuffix(boolean)} will need to be set.
*
*
* @param suffix the suffix to be set.
*/
public final void setSuffix(final String suffix) {
this.suffix = suffix;
}
/**
*
* Returns whether the application of the suffix should be forced on the template
* name.
*
*
* When forced, suffix will be appended to the template name even if the template
* name ends in a known suffix: .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt.
*
* Default value is false
.
*
* @return whether the suffix will be forced or not.
* @since 3.0.6
*/
public final boolean getForceSuffix() {
return this.forceSuffix;
}
/**
*
* Sets whether the application of the suffix should be forced on the template
* name.
*
*
* When forced, suffix will be appended to the template name even if the template
* name ends in a known suffix: .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt.
*
* Default value is false
.
*
* @param forceSuffix whether the suffix should be forced or not.
* @since 3.0.6
*/
public final void setForceSuffix(final boolean forceSuffix) {
this.forceSuffix = forceSuffix;
}
/**
*
* Returns the character encoding to be used for reading template resources
* resolved by this template resolver.
*
*
* @return the character encoding.
*/
public final String getCharacterEncoding() {
return this.characterEncoding;
}
/**
*
* Sets a new character encoding for reading template resources.
*
*
* @param characterEncoding the character encoding to be used.
*/
public final void setCharacterEncoding(final String characterEncoding) {
this.characterEncoding = characterEncoding;
}
/**
*
* Returns the template mode to be applied to templates resolved by
* this template resolver.
*
*
* If template mode patterns (see {@link #setXhtmlTemplateModePatterns(Set)},
* {@link #setHtml5TemplateModePatterns(Set)}, etc.) are also set, they have higher
* priority than the template mode set here (this would act as a default).
*
*
* Note that this template mode also may not be applied if the template resource name
* ends in a known file name suffix: .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt. If this behaviour needs to be overridden so
* that template name is always applied, the {@link #setForceTemplateMode(boolean)} will need to be set.
*
*
* @return the template mode to be used.
*/
public final TemplateMode getTemplateMode() {
return this.templateMode;
}
/**
*
* Sets the template mode to be applied to templates resolved by this resolver.
*
*
* If template mode patterns (see {@link #setXhtmlTemplateModePatterns(Set)},
* {@link #setHtml5TemplateModePatterns(Set)}, etc.) are also set, they have higher
* priority than the template mode set here (this would act as a default).
*
*
* Note that this template mode also may not be applied if the template resource name
* ends in a known file name suffix: .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt. If this behaviour needs to be overridden so
* that template name is always applied, the {@link #setForceTemplateMode(boolean)} will need to be set.
*
*
* @param templateMode the template mode.
*/
public final void setTemplateMode(final TemplateMode templateMode) {
Validate.notNull(templateMode, "Cannot set a null template mode value");
// We re-parse the specified template mode so that we make sure we get rid of deprecated values
this.templateMode = TemplateMode.parse(templateMode.toString());
}
/**
*
* Sets the template mode to be applied to templates resolved by this resolver.
*
*
* Allowed templates modes are defined by the {@link TemplateMode} class.
*
*
* If template mode patterns (see {@link #setXhtmlTemplateModePatterns(Set)},
* {@link #setHtml5TemplateModePatterns(Set)}, etc.) are also set, they have higher
* priority than the template mode set here (this would act as a default).
*
*
* Note that this template mode also may not be applied if the template resource name
* ends in a known file name suffix: .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt. If this behaviour needs to be overridden so
* that template name is always applied, the {@link #setForceTemplateMode(boolean)} will need to be set.
*
*
* @param templateMode the template mode.
*/
public final void setTemplateMode(final String templateMode) {
// Setter overload actually goes against the JavaBeans spec, but having this one is good for legacy
// compatibility reasons. Besides, given the getter returns TemplateMode, intelligent frameworks like
// Spring will recognized the property as TemplateMode-typed and simply ignore this setter.
Validate.notNull(templateMode, "Cannot set a null template mode value");
this.templateMode = TemplateMode.parse(templateMode);
}
/**
*
* Returns whether the configured template mode should be forced instead of attempting
* a smart template mode resolution based on template resource name.
*
*
* When forced, the configured template mode ({@link #setTemplateMode(TemplateMode)} will
* be applied even if the template resource name ends in a known suffix:
* .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt.
*
* Default value is false
.
*
* @return whether the suffix will be forced or not.
* @since 3.0.6
*/
public final boolean getForceTemplateMode() {
return this.forceTemplateMode;
}
/**
*
* Sets whether the configured template mode should be forced instead of attempting
* a smart template mode resolution based on template resource name.
*
*
* When forced, the configured template mode ({@link #setTemplateMode(TemplateMode)} will
* be applied even if the template resource name ends in a known suffix:
* .html, .htm, .xhtml,
* .xml, .js, .json,
* .css, .rss, .atom, .txt.
*
* Default value is false
.
*
* @param forceTemplateMode whether the configured template mode should be forced or not.
* @since 3.0.6
*/
public final void setForceTemplateMode(final boolean forceTemplateMode) {
this.forceTemplateMode = forceTemplateMode;
}
/**
*
* Returns whether templates resolved by this resolver have to be considered
* cacheable or not.
*
*
* If cacheable patterns (see {@link #setCacheablePatterns(Set)})
* are also set, they have higher priority than the value set here (this
* would act as a default).
*
*
* @return whether templates resolved are cacheable or not.
*/
public final boolean isCacheable() {
return this.cacheable;
}
/**
*
* Sets a new value for the cacheable flag.
*
*
* If cacheable patterns (see {@link #setCacheablePatterns(Set)})
* are also set, they have higher priority than the value set here (this
* would act as a default).
*
*
* @param cacheable whether resolved patterns should be considered cacheable or not.
*/
public final void setCacheable(final boolean cacheable) {
this.cacheable = cacheable;
}
/**
*
* Returns the TTL (Time To Live) in cache of templates resolved by this
* resolver.
*
*
* If a template is resolved as cacheable but cache TTL is null,
* this means the template will live in cache until evicted by LRU
* (Least Recently Used) algorithm for being the oldest entry in cache.
*
*
* @return the cache TTL for resolved templates.
*/
public final Long getCacheTTLMs() {
return this.cacheTTLMs;
}
/**
*
* Sets a new value for the cache TTL for resolved templates.
*
*
* If a template is resolved as cacheable but cache TTL is null,
* this means the template will live in cache until evicted by LRU
* (Least Recently Used) algorithm for being the oldest entry in cache.
*
*
* @param cacheTTLMs the new cache TTL, or null for using natural LRU eviction.
*/
public final void setCacheTTLMs(final Long cacheTTLMs) {
this.cacheTTLMs = cacheTTLMs;
}
/**
*
* Returns the currently configured template aliases.
*
*
* Template aliases allow the use of several (and probably shorter)
* names for templates.
*
*
* Aliases are applied to template names before prefix/suffix.
*
*
* @return the map of template aliases.
*/
public final Map getTemplateAliases() {
return Collections.unmodifiableMap(this.templateAliases);
}
/**
*
* Sets all the new template aliases to be used.
*
*
* Template aliases allow the use of several (and probably shorter)
* names for templates.
*
*
* Aliases are applied to template names before prefix/suffix.
*
*
* @param templateAliases the new template aliases.
*/
public final void setTemplateAliases(final Map templateAliases) {
if (templateAliases != null) {
this.templateAliases.putAll(templateAliases);
}
}
/**
*
* Adds a new template alias to the currently configured ones.
*
*
* @param alias the new alias name
* @param templateName the name of the template the alias will be applied to
*/
public final void addTemplateAlias(final String alias, final String templateName) {
Validate.notNull(alias, "Alias cannot be null");
Validate.notNull(templateName, "Template name cannot be null");
this.templateAliases.put(alias, templateName);
}
/**
*
* Removes all currently configured template aliases.
*
*/
public final void clearTemplateAliases() {
this.templateAliases.clear();
}
/**
*
* Returns the pattern spec specified for establishing the {@link TemplateMode#XML}
* template mode to resolved templates.
*
*
* @return the pattern spec
*/
public final PatternSpec getXmlTemplateModePatternSpec() {
return this.xmlTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the {@link TemplateMode#XML}
* template mode to resolved templates.
*
*
* This is a convenience method equivalent to {@link #getXmlTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
*/
public final Set getXmlTemplateModePatterns() {
return this.xmlTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the {@link TemplateMode#XML}
* template mode as Strings.
*
*
* This is a convenience method equivalent to {@link #getXmlTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newXmlTemplateModePatterns the new patterns
*/
public final void setXmlTemplateModePatterns(final Set newXmlTemplateModePatterns) {
this.xmlTemplateModePatternSpec.setPatterns(newXmlTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the {@link TemplateMode#HTML}
* template mode to resolved templates.
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final PatternSpec getHtmlTemplateModePatternSpec() {
return this.htmlTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the {@link TemplateMode#HTML}
* template mode to resolved templates.
*
*
* This is a convenience method equivalent to {@link #getHtmlTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final Set getHtmlTemplateModePatterns() {
return this.htmlTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the {@link TemplateMode#HTML}
* template mode as Strings.
*
*
* This is a convenience method equivalent to {@link #getHtmlTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newHtmlTemplateModePatterns the new patterns
* @since 3.0.0
*/
public final void setHtmlTemplateModePatterns(final Set newHtmlTemplateModePatterns) {
this.htmlTemplateModePatternSpec.setPatterns(newHtmlTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the {@link TemplateMode#JAVASCRIPT}
* template mode to resolved templates.
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final PatternSpec getJavaScriptTemplateModePatternSpec() {
return this.javaScriptTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the {@link TemplateMode#JAVASCRIPT}
* template mode to resolved templates.
*
*
* This is a convenience method equivalent to {@link #getJavaScriptTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final Set getJavaScriptTemplateModePatterns() {
return this.javaScriptTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the {@link TemplateMode#JAVASCRIPT}
* template mode as Strings.
*
*
* This is a convenience method equivalent to {@link #getJavaScriptTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newJavaScriptTemplateModePatterns the new patterns
* @since 3.0.0
*/
public final void setJavaScriptTemplateModePatterns(final Set newJavaScriptTemplateModePatterns) {
this.javaScriptTemplateModePatternSpec.setPatterns(newJavaScriptTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the {@link TemplateMode#CSS}
* template mode to resolved templates.
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final PatternSpec getCSSTemplateModePatternSpec() {
return this.cssTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the {@link TemplateMode#CSS}
* template mode to resolved templates.
*
*
* This is a convenience method equivalent to {@link #getCSSTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final Set getCSSTemplateModePatterns() {
return this.cssTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the {@link TemplateMode#CSS}
* template mode as Strings.
*
*
* This is a convenience method equivalent to {@link #getCSSTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newCSSTemplateModePatterns the new patterns
* @since 3.0.0
*/
public final void setCSSTemplateModePatterns(final Set newCSSTemplateModePatterns) {
this.cssTemplateModePatternSpec.setPatterns(newCSSTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the {@link TemplateMode#RAW}
* template mode to resolved templates.
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final PatternSpec getRawTemplateModePatternSpec() {
return this.rawTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the {@link TemplateMode#RAW}
* template mode to resolved templates.
*
*
* This is a convenience method equivalent to {@link #getRawTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final Set getRawTemplateModePatterns() {
return this.rawTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the {@link TemplateMode#RAW}
* template mode as Strings.
*
*
* This is a convenience method equivalent to {@link #getRawTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newRawTemplateModePatterns the new patterns
* @since 3.0.0
*/
public final void setRawTemplateModePatterns(final Set newRawTemplateModePatterns) {
this.rawTemplateModePatternSpec.setPatterns(newRawTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the {@link TemplateMode#TEXT}
* template mode to resolved templates.
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final PatternSpec getTextTemplateModePatternSpec() {
return this.textTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the {@link TemplateMode#TEXT}
* template mode to resolved templates.
*
*
* This is a convenience method equivalent to {@link #getTextTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @since 3.0.0
*/
public final Set getTextTemplateModePatterns() {
return this.textTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the {@link TemplateMode#TEXT}
* template mode as Strings.
*
*
* This is a convenience method equivalent to {@link #getTextTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newTextTemplateModePatterns the new patterns
* @since 3.0.0
*/
public final void setTextTemplateModePatterns(final Set newTextTemplateModePatterns) {
this.textTemplateModePatternSpec.setPatterns(newTextTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the deprecated VALIDXML (validated XML)
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#XML} template mode instead.
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final PatternSpec getValidXmlTemplateModePatternSpec() {
return this.xmlTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the deprecated VALIDXML (validated XML)
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#XML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getValidXmlTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final Set getValidXmlTemplateModePatterns() {
return this.xmlTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the deprecated VALIDXML (validated XML)
* template mode as Strings. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#XML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getValidXmlTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newValidXmlTemplateModePatterns the new patterns
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final void setValidXmlTemplateModePatterns(final Set newValidXmlTemplateModePatterns) {
this.xmlTemplateModePatternSpec.setPatterns(newValidXmlTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the deprecated XHTML
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final PatternSpec getXhtmlTemplateModePatternSpec() {
return this.htmlTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the deprecated XHTML
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getXhtmlTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final Set getXhtmlTemplateModePatterns() {
return this.htmlTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the deprecated XHTML
* template mode as Strings. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getXhtmlTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newXhtmlTemplateModePatterns the new patterns
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final void setXhtmlTemplateModePatterns(final Set newXhtmlTemplateModePatterns) {
this.htmlTemplateModePatternSpec.setPatterns(newXhtmlTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the deprecated VALIDXHTML (validated XHTML)
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final PatternSpec getValidXhtmlTemplateModePatternSpec() {
return this.htmlTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the deprecated VALIDXHTML (validated XHTML)
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getValidXhtmlTemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final Set getValidXhtmlTemplateModePatterns() {
return this.htmlTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the deprecated VALIDXHTML (validated XHTML)
* template mode as Strings. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getValidXhtmlTemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newValidXhtmlTemplateModePatterns the new patterns
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final void setValidXhtmlTemplateModePatterns(final Set newValidXhtmlTemplateModePatterns) {
this.htmlTemplateModePatternSpec.setPatterns(newValidXhtmlTemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the deprecated LEGACYHTML5 (non-XML-formed HTML5 that needs HTML-to-XML conversion)
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final PatternSpec getLegacyHtml5TemplateModePatternSpec() {
return this.htmlTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the deprecated LEGACYHTML5 (non-XML-formed HTML5 that needs HTML-to-XML conversion)
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getLegacyHtml5TemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final Set getLegacyHtml5TemplateModePatterns() {
return this.htmlTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the deprecated LEGACYHTML5 (non-XML-formed HTML5 that needs HTML-to-XML conversion)
* template mode as Strings. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getLegacyHtml5TemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newLegacyHtml5TemplateModePatterns the new patterns
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final void setLegacyHtml5TemplateModePatterns(final Set newLegacyHtml5TemplateModePatterns) {
this.htmlTemplateModePatternSpec.setPatterns(newLegacyHtml5TemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing the deprecated HTML5 (correct, XML-formed HTML5)
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final PatternSpec getHtml5TemplateModePatternSpec() {
return this.htmlTemplateModePatternSpec;
}
/**
*
* Returns the patterns specified for establishing the deprecated HTML5 (correct, XML-formed HTML5)
* template mode to resolved templates. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getHtml5TemplateModePatternSpec()}.getPatterns()
*
*
* @return the pattern spec
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final Set getHtml5TemplateModePatterns() {
return this.htmlTemplateModePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing the deprecated HTML5 (correct, XML-formed HTML5)
* template mode as Strings. Note that, due to the deprecation of this template mode, these patterns
* will be applied to the {@link TemplateMode#HTML} template mode instead.
*
*
* This is a convenience method equivalent to {@link #getHtml5TemplateModePatternSpec()}.setPatterns(Set<String>)
*
*
* @param newHtml5TemplateModePatterns the new patterns
* @deprecated Deprecated in 3.0.0. Use the methods for the {@link TemplateMode#XML} template mode instead.
* Will be removed in 3.1
*/
@Deprecated
public final void setHtml5TemplateModePatterns(final Set newHtml5TemplateModePatterns) {
this.htmlTemplateModePatternSpec.setPatterns(newHtml5TemplateModePatterns);
}
/**
*
* Returns the pattern spec specified for establishing which
* templates have to be considered cacheable.
*
*
* These patterns have higher precedence than the cacheable
* flag (see {@link #setCacheable(boolean)}). Such flag can be considered
* a default value after cacheable patterns and
* non-cacheable patterns have been applied.
*
*
* @return the pattern spec
*/
public final PatternSpec getCacheablePatternSpec() {
return this.cacheablePatternSpec;
}
/**
*
* Returns the patterns (as String) specified for establishing which
* templates have to be considered cacheable.
*
*
* These patterns have higher precedence than the cacheable
* flag (see {@link #setCacheable(boolean)}). Such flag can be considered
* a default value after cacheable patterns and
* non-cacheable patterns have been applied.
*
*
* This is a convenience method equivalent to {@link #getCacheablePatternSpec()}.getPatterns()
*
*
* @return the patterns
*/
public final Set getCacheablePatterns() {
return this.cacheablePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing which
* templates have to be considered cacheable
*
*
* These patterns have higher precedence than the cacheable
* flag (see {@link #setCacheable(boolean)}). Such flag can be considered
* a default value after cacheable patterns and
* non-cacheable patterns have been applied.
*
*
* This is a convenience method equivalent to {@link #getCacheablePatternSpec()}.setPatterns(Set<String>)
*
*
* @param cacheablePatterns the new patterns
*/
public final void setCacheablePatterns(final Set cacheablePatterns) {
this.cacheablePatternSpec.setPatterns(cacheablePatterns);
}
/**
*
* Returns the pattern spec specified for establishing which
* templates have to be considered non cacheable.
*
*
* These patterns have higher precedence than the cacheable
* flag (see {@link #setCacheable(boolean)}). Such flag can be considered
* a default value after cacheable patterns and
* non-cacheable patterns have been applied.
*
*
* @return the pattern spec
*/
public final PatternSpec getNonCacheablePatternSpec() {
return this.nonCacheablePatternSpec;
}
/**
*
* Returns the patterns (as String) specified for establishing which
* templates have to be considered non cacheable.
*
*
* These patterns have higher precedence than the cacheable
* flag (see {@link #setCacheable(boolean)}). Such flag can be considered
* a default value after cacheable patterns and
* non-cacheable patterns have been applied.
*
*
* This is a convenience method equivalent to {@link #getNonCacheablePatternSpec()}.getPatterns()
*
*
* @return the patterns
*/
public final Set getNonCacheablePatterns() {
return this.nonCacheablePatternSpec.getPatterns();
}
/**
*
* Sets the new patterns to be applied for establishing which
* templates have to be considered non cacheable
*
*
* These patterns have higher precedence than the cacheable
* flag (see {@link #setCacheable(boolean)}). Such flag can be considered
* a default value after cacheable patterns and
* non-cacheable patterns have been applied.
*
*
* This is a convenience method equivalent to {@link #getNonCacheablePatternSpec()}.setPatterns(Set<String>)
*
*
* @param nonCacheablePatterns the new patterns
*/
public final void setNonCacheablePatterns(final Set nonCacheablePatterns) {
this.nonCacheablePatternSpec.setPatterns(nonCacheablePatterns);
}
/**
*
* Computes the resource name that will be used for resolving, from the template name and other
* parameters configured at this configurable resolver.
*
*
* This method can be overridden by subclasses that need to modify the standard way in which the
* name of the template resource is computed by default before passing it to the real resource
* resolution mechanism (in method {@link #computeTemplateResource(IEngineConfiguration, String, String, String, String, Map)}
*
*
* By default, the resource name will be created by first applying the template aliases, and then
* adding prefix and suffix to the specified template (template name).
*
*
* @param configuration the engine configuration in use.
* @param ownerTemplate the owner template, if the resource being computed is a fragment. Might be null.
* @param template the template (normally the template name, except for String templates).
* @param prefix the prefix to be applied.
* @param suffix the suffix to be applied.
* @param templateAliases the template aliases map.
* @param templateResolutionAttributes the template resolution attributes, if any. Might be null.
* @return the resource name that should be used for resolving
* @deprecated in 3.0.6. Use {@link #computeResourceName(IEngineConfiguration, String, String, String, String, boolean, Map, Map)} instead.
* Will be removed in Thymeleaf 3.2.
*/
@Deprecated
protected String computeResourceName(
final IEngineConfiguration configuration, final String ownerTemplate, final String template,
final String prefix, final String suffix, final Map templateAliases,
final Map templateResolutionAttributes) {
return computeResourceName(
configuration, ownerTemplate, template, prefix, suffix, false, templateAliases,
templateResolutionAttributes);
}
/**
*
* Computes the resource name that will be used for resolving, from the template name and other
* parameters configured at this configurable resolver.
*
*
* This method can be overridden by subclasses that need to modify the standard way in which the
* name of the template resource is computed by default before passing it to the real resource
* resolution mechanism (in method {@link #computeTemplateResource(IEngineConfiguration, String, String, String, String, Map)}
*
*
* By default, the resource name will be created by first applying the template aliases, and then
* adding prefix and suffix to the specified template (template name).
*
*
* @param configuration the engine configuration in use.
* @param ownerTemplate the owner template, if the resource being computed is a fragment. Might be null.
* @param template the template (normally the template name, except for String templates).
* @param prefix the prefix to be applied.
* @param suffix the suffix to be applied.
* @param forceSuffix whether the suffix should be forced or not.
* @param templateAliases the template aliases map.
* @param templateResolutionAttributes the template resolution attributes, if any. Might be null.
* @return the resource name that should be used for resolving
* @since 3.0.6
*/
protected String computeResourceName(
final IEngineConfiguration configuration, final String ownerTemplate, final String template,
final String prefix, final String suffix, final boolean forceSuffix,
final Map templateAliases, final Map templateResolutionAttributes) {
Validate.notNull(template, "Template name cannot be null");
String unaliasedName = templateAliases.get(template);
if (unaliasedName == null) {
unaliasedName = template;
}
final boolean hasPrefix = !StringUtils.isEmptyOrWhitespace(prefix);
final boolean hasSuffix = !StringUtils.isEmptyOrWhitespace(suffix);
final boolean shouldApplySuffix =
hasSuffix && (forceSuffix || !ContentTypeUtils.hasRecognizedFileExtension(unaliasedName));
if (!hasPrefix && !shouldApplySuffix){
return unaliasedName;
}
if (!hasPrefix) { // shouldApplySuffix
return unaliasedName + suffix;
}
if (!shouldApplySuffix) { // hasPrefix
return prefix + unaliasedName;
}
// hasPrefix && shouldApplySuffix
return prefix + unaliasedName + suffix;
}
@Override
protected TemplateMode computeTemplateMode(
final IEngineConfiguration configuration, final String ownerTemplate,
final String template, final Map templateResolutionAttributes) {
if (this.xmlTemplateModePatternSpec.matches(template)) {
return TemplateMode.XML;
}
if (this.htmlTemplateModePatternSpec.matches(template)) {
return TemplateMode.HTML;
}
if (this.textTemplateModePatternSpec.matches(template)) {
return TemplateMode.TEXT;
}
if (this.javaScriptTemplateModePatternSpec.matches(template)) {
return TemplateMode.JAVASCRIPT;
}
if (this.cssTemplateModePatternSpec.matches(template)) {
return TemplateMode.CSS;
}
if (this.rawTemplateModePatternSpec.matches(template)) {
return TemplateMode.RAW;
}
if (!this.forceTemplateMode) {
final String templateResourceName =
computeResourceName(
configuration, ownerTemplate, template,
this.prefix, this.suffix, this.forceSuffix, this.templateAliases,
templateResolutionAttributes);
final TemplateMode autoResolvedTemplateMode =
ContentTypeUtils.computeTemplateModeForTemplateName(templateResourceName);
if (autoResolvedTemplateMode != null) {
return autoResolvedTemplateMode;
}
}
return getTemplateMode();
}
@Override
protected ICacheEntryValidity computeValidity(final IEngineConfiguration configuration, final String ownerTemplate, final String template, final Map templateResolutionAttributes) {
if (this.cacheablePatternSpec.matches(template)) {
if (this.cacheTTLMs != null) {
return new TTLCacheEntryValidity(this.cacheTTLMs.longValue());
}
return AlwaysValidCacheEntryValidity.INSTANCE;
}
if (this.nonCacheablePatternSpec.matches(template)) {
return NonCacheableCacheEntryValidity.INSTANCE;
}
if (isCacheable()) {
if (this.cacheTTLMs != null) {
return new TTLCacheEntryValidity(this.cacheTTLMs.longValue());
}
return AlwaysValidCacheEntryValidity.INSTANCE;
}
return NonCacheableCacheEntryValidity.INSTANCE;
}
@Override
protected final ITemplateResource computeTemplateResource(final IEngineConfiguration configuration, final String ownerTemplate, final String template, final Map templateResolutionAttributes) {
final String resourceName =
computeResourceName(configuration, ownerTemplate, template, this.prefix, this.suffix, this.forceSuffix, this.templateAliases, templateResolutionAttributes);
return computeTemplateResource(configuration, ownerTemplate, template, resourceName, this.characterEncoding, templateResolutionAttributes);
}
/**
*
* Compute the real resource, once the resource name has been computed using prefix, suffix, and other
* configured artifacts.
*
*
* @param configuration the engine configuration in use.
* @param ownerTemplate the owner template, if the resource being computed is a fragment. Might be null.
* @param template the template (normally the template name, except for String templates).
* @param resourceName the resource name, complete with prefix, suffix, aliases, etc.
* @param characterEncoding the character encoding to be used for reading the resource.
* @param templateResolutionAttributes the template resolution attributes, if any. Might be null.
* @return the template resource
*/
protected abstract ITemplateResource computeTemplateResource(
final IEngineConfiguration configuration, final String ownerTemplate, final String template, final String resourceName, final String characterEncoding, final Map templateResolutionAttributes);
}