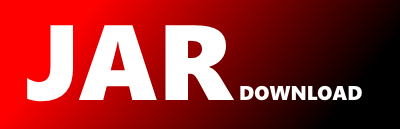
org.thymeleaf.model.IAttribute Maven / Gradle / Ivy
Show all versions of thymeleaf Show documentation
/*
* =============================================================================
*
* Copyright (c) 2011-2016, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.model;
import java.io.IOException;
import java.io.Writer;
import org.thymeleaf.engine.AttributeDefinition;
/**
*
* Interface defining an attribute contained in a tag.
*
*
* Container tags are implementations of {@link IProcessableElementTag}.
*
*
* Note that any implementations of this interface should be immutable.
*
*
* @author Daniel Fernández
* @since 3.0.0
*
*/
public interface IAttribute {
/**
*
* Returns the complete name of the attribute, exactly as it was written in the original template (if it
* did appear there).
*
*
* @return the complete name.
*/
public String getAttributeCompleteName();
/**
*
* Returns the {@link AttributeDefinition} corresponding to this attribute.
*
*
* The attribute definition contains several metadata related to the attribute. For example, if the
* template mode is {@link org.thymeleaf.templatemode.TemplateMode#HTML}, an attribute definition could
* specify whether the attribute is boolean (represents a true/false value by appearing or not appearing
* at a specific tag).
*
*
* @return the attribute definition.
*/
public AttributeDefinition getAttributeDefinition();
/**
*
* Returns the operator specified for this attribute.
*
*
* The operator itself, if present, is always an equals sign (=), but the reason this is specified as a separate
* field is that it could be surrounded by white space, which should be respected in output when present at
* the input template.
*
*
* If the attribute is specified without a value at all (and therefore no operator either), this method will
* return null.
*
*
* @return the attribute operator (might be null if no value specified).
*/
public String getOperator();
/**
*
* Returns the value of this attribute, or null if it has none.
*
*
* A null-valued attribute is an attribute of which only the name has been specified (only allowed in HTML mode).
*
*
* @return the value of this attribute, or null if it has none.
*/
public String getValue();
/**
*
* Returns the type of quotes surrounding the attribute value.
*
*
* @return the {@link AttributeValueQuotes} value representing the attribute value quotes (might be null).
*/
public AttributeValueQuotes getValueQuotes();
/**
*
* Checks whether this attribute contains location information (template name, line and column).
*
*
* Only attributes that are generated during the parsing of templates contain location info, locating them
* in their original template. All attributes generated during template processing and not originally present
* at the template do not contain this location data.
*
*
* @return whether the attribute contains location data or not.
*/
public boolean hasLocation();
/**
*
* Returns the name of the template from which parsing this attribute was originally created.
*
*
* @return the name of the template
*/
public String getTemplateName();
/**
*
* Returns the line at which this attribute can be found in the template specified by {@link #getTemplateName()}.
*
*
* @return the line number, starting in 1.
*/
public int getLine();
/**
*
* Returns the column at which this attribute can be found in the template specified by {@link #getTemplateName()}.
*
*
* @return the column number, starting in 1.
*/
public int getCol();
/**
*
* Writes this attribute to the specified {@link Writer}.
*
*
* This is usually called as a part of {@link ITemplateEvent#write(Writer)}.
*
*
* @param writer the writer this attribute should be written to.
* @throws IOException if an input/output exception occurs.
*/
public void write(final Writer writer) throws IOException;
}