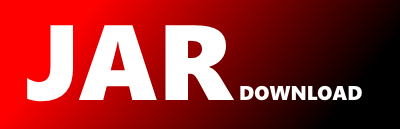
org.thymeleaf.util.EvaluationUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thymeleaf Show documentation
Show all versions of thymeleaf Show documentation
Modern server-side Java template engine for both web and standalone environments
/*
* =============================================================================
*
* Copyright (c) 2011-2018, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.util;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import org.thymeleaf.standard.expression.LiteralValue;
/**
*
* @author Daniel Fernández
*
* @since 3.0.0 (renamed from org.thymeleaf.util.EvaluationUtil)
*
*/
public final class EvaluationUtils {
public static boolean evaluateAsBoolean(final Object condition) {
boolean result = true;
if (condition == null) {
result = false;
} else {
if (condition instanceof Boolean) {
result = ((Boolean)condition).booleanValue();
} else if (condition instanceof Number) {
if (condition instanceof BigDecimal) {
result = (((BigDecimal) condition).compareTo(BigDecimal.ZERO) != 0);
} else if (condition instanceof BigInteger) {
result = !condition.equals(BigInteger.ZERO);
} else {
result = ((Number)condition).doubleValue() != 0.0;
}
} else if (condition instanceof Character) {
result = ((Character) condition).charValue() != 0;
} else if (condition instanceof String) {
final String condStr = ((String)condition).trim().toLowerCase();
result = !("false".equals(condStr) || "off".equals(condStr) || "no".equals(condStr));
} else if (condition instanceof LiteralValue) {
final String condStr = ((LiteralValue)condition).getValue().trim().toLowerCase();
result = !("false".equals(condStr) || "off".equals(condStr) || "no".equals(condStr));
} else {
result = true;
}
}
return result;
}
public static BigDecimal evaluateAsNumber(final Object object) {
if (object == null) {
return null;
}
if (object instanceof Number) {
if (object instanceof BigDecimal) {
return (BigDecimal)object;
} else if (object instanceof BigInteger) {
return new BigDecimal((BigInteger)object);
} else if (object instanceof Byte) {
return new BigDecimal(((Byte)object).intValue());
} else if (object instanceof Short) {
return new BigDecimal(((Short)object).intValue());
} else if (object instanceof Integer) {
return new BigDecimal(((Integer)object).intValue());
} else if (object instanceof Long) {
return new BigDecimal(((Long)object).longValue());
} else if (object instanceof Float) {
//noinspection UnpredictableBigDecimalConstructorCall
return new BigDecimal(((Float)object).doubleValue());
} else if (object instanceof Double) {
//noinspection UnpredictableBigDecimalConstructorCall
return new BigDecimal(((Double)object).doubleValue());
}
} else if (object instanceof String && ((String)object).length() > 0) {
final char c0 = ((String)object).charAt(0);
// This test will avoid trying to create the BigDecimal most of the times, which
// will improve performance by avoiding lots of NumberFormatExceptions
if ((c0 >= '0' && c0 <= '9') || c0 == '+' || c0 == '-') {
try {
return new BigDecimal(((String)object).trim());
} catch (final NumberFormatException ignored) {
return null;
}
}
}
return null;
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy