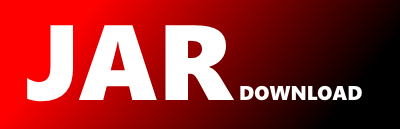
timeBench.action.analytical.IndexingAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of timebench Show documentation
Show all versions of timebench Show documentation
TimeBench, a flexible, easy-to-use, and reusable software library written in Java that provides foundational data structures and algorithms for time- oriented data in Visual Analytics.
The newest version!
package timeBench.action.analytical;
import java.util.Map;
import java.util.TreeMap;
import prefuse.action.ItemAction;
import prefuse.visual.VisualItem;
public abstract class IndexingAction extends ItemAction {
protected Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy