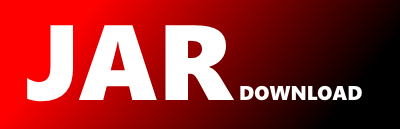
org.tinymediamanager.scraper.entities.CountryCode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api-scraper Show documentation
Show all versions of api-scraper Show documentation
API for tinyMediaManager scrapers
/*
* Copyright 2012 - 2019 Manuel Laggner
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.tinymediamanager.scraper.entities;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
/**
* ISO 3166-1 country code.
*
*
* Enum names of this enum themselves are represented by ISO 3166-1 alpha-2 codes. There
* are instance methods to get the country name ({@link #getName()} ), the ISO 3166-1
* alpha-3 code ({@link #getAlpha3()}) and the ISO 3166-1 numeric code (
* {@link #getNumeric()}). In addition, there are static methods to get a CountryCode instance that corresponds to a given alpha-2/alpha-3/numeric
* code ({@link #getByCode(String)}, {@link #getByCode(int)}).
*
*
*
* // EXAMPLE
*
* CountryCode cc = CountryCode.{@link #getByCode(String) getByCode}("JP");
*
* // Country name
* System.out.println("Country name = " + cc.{@link #getName()}); // "Japan"
*
* // ISO 3166-1 alpha-2 code
* System.out.println("ISO 3166-1 alpha-2 code = " + cc.{@link #getAlpha2()}); // "JP"
*
* // ISO 3166-1 alpha-3 code
* System.out.println("ISO 3166-1 alpha-3 code = " + cc.{@link #getAlpha3()}); // "JPN"
*
* // ISO 3166-1 numeric code
* System.out.println("ISO 3166-1 numeric code = " + cc.{@link #getNumeric()}); // 392
*
*
* @author Takahiko Kawasaki
* @since 1.0
*/
public enum CountryCode {
// @formatter:off
// /** Andorra */
// AD("Andorra", "AND", 16),
//
// /**
// * United Arab
// * Emirates
// */
// AE("United Arab Emirates", "ARE", 784),
//
// /** Afghanistan */
// AF("Afghanistan", "AFG", 4),
//
// /**
// * Antigua and
// * Barbuda
// */
// AG("Antigua and Barbuda", "ATG", 28),
//
// /** Anguilla */
// AI("Anguilla", "AIA", 660),
//
// /** Albania */
// AL("Albania", "ALB", 8),
//
// /** Armenia */
// AM("Armenia", "ARM", 51),
//
// /**
// * Netherlands
// * Antilles
// */
// AN("Netherlands Antilles", "ANT", 530),
//
// /** Angola */
// AO("Angola", "AGO", 24),
//
// /** Antarctica */
// AQ("Antarctica", "ATA", 10),
//
// /** Argentina */
// AR("Argentina", "ARG", 32),
//
// /** American
// Samoa */
// AS("American Samoa", "ASM", 16),
//
// /** Austria */
// AT("Austria", "AUT", 40),
//
/** Australia */
AU("Australia", "AUS", 36),
//
// /** Aruba */
// AW("Aruba", "ABW", 533),
//
// /**
// * Åland
// * Islands
// */
// AX("\u212Bland Islands", "ALA", 248),
//
// /** Azerbaijan */
// AZ("Azerbaijan", "AZE", 31),
//
// /**
// * Bosnia and
// * Herzegovina
// */
// BA("Bosnia and Herzegovina", "BIH", 70),
//
// /** Barbados */
// BB("Barbados", "BRB", 52),
//
// /** Bangladesh */
// BD("Bangladesh", "BGD", 50),
//
// /** Belgium */
// BE("Belgium", "BEL", 56),
//
// /** Burkina Faso */
// BF("Burkina Faso", "BFA", 854),
//
// /** Bulgaria */
// BG("Bulgaria", "BGR", 100),
//
// /** Bahrain */
// BH("Bahrain", "BHR", 48),
//
// /** Burundi */
// BI("Burundi", "BDI", 108),
//
// /** Benin */
// BJ("Benin", "BEN", 204),
//
// /**
// * Saint
// * Barthélemy
// */
// BL("Saint Barth\u00E9lemy", "BLM", 652),
//
// /** Bermuda */
// BM("Bermuda", "BMU", 60),
//
// /** Brunei Darussalam */
// BN("Brunei Darussalam", "BRN", 96),
//
// /**
// * Plurinational State of
// * Bolivia
// */
// BO("Plurinational State of Bolivia", "BOL", 68),
//
// /**
// * Bonaire,
// Sint
// * Eustatius and Saba
// */
// BQ("Bonaire, Sint Eustatius and Saba", "BES", 535),
//
// /** Brazil */
// BR("Brazil", "BRA", 76),
//
// /** Bahamas */
// BS("Bahamas", "BHS", 44),
//
// /** Bhutan */
// BT("Bhutan", "BTN", 64),
//
// /** Bouvet Island
// */
// BV("Bouvet Island", "BVT", 74),
//
// /** Botswana */
// BW("Botswana", "BWA", 72),
//
// /** Belarus */
// BY("Belarus", "BLR", 112),
//
// /** Belize */
// BZ("Belize", "BLZ", 84),
//
/** Canada */
CA("Canada", "CAN", 124),
//
// /**
// * Cocos
// * (Keeling) Islands
// */
// CC("Cocos Islands", "CCK", 166),
//
// /**
// * The
// * Democratic Republic of the Congo
// */
// CD("The Democratic Republic of the Congo", "COD", 180),
//
// /**
// * Central
// * African Republic
// */
// CF("Central African Republic", "CAF", 140),
//
// /** Congo
// */
// CG("Congo", "COG", 178),
//
/** Switzerland */
CH("Switzerland", "CHE", 756),
//
// /**
// * Côte
// * d'Ivoire
// */
// CI("C\u00F4te d'Ivoire", "CIV", 384),
//
// /** Cook Islands */
// CK("Cook Islands", "COK", 184),
//
// /** Chile */
// CL("Chile", "CHL", 152),
//
// /** Cameroon */
// CM("Cameroon", "CMR", 120),
//
// /** China */
// CN("China", "CHN", 156),
//
// /** Colombia */
// CO("Colombia", "COL", 170),
//
// /** Costa Rica */
// CR("Costa Rica", "CRI", 188),
//
// /** Cuba */
// CU("Cuba", "CUB", 192),
//
// /** Cape Verde */
// CV("Cape Verde", "CPV", 132),
//
// /** Curaçao
// */
// CW("Cura/u00E7ao", "CUW", 531),
//
// /**
// * Christmas
// * Island
// */
// CX("Christmas Island", "CXR", 162),
//
// /** Cyprus */
// CY("Cyprus", "CYP", 196),
//
/**
* Czech Republic
*/
CZ("Czech Republic", "CZE", 203),
/** Germany */
DE("Germany", "DEU", 276),
// /** Djibouti */
// DJ("Djibouti", "DJI", 262),
//
/** Denmark */
DK("Denmark", "DNK", 208),
//
// /** Dominica */
// DM("Dominica", "DMA", 212),
//
// /**
// * Dominican
// * Republic
// */
// DO("Dominican Republic", "DOM", 214),
//
// /** Algeria */
// DZ("Algeria", "DZA", 12),
//
// /** Ecuador */
// EC("Ecuador", "ECU", 218),
//
/** Estonia */
EE("Estonia", "EST", 233),
//
// /** Egypt */
// EG("Egypt", "EGY", 818),
//
// /** Western
// Sahara */
// EH("Western Sahara", "ESH", 732),
//
// /** Eritrea */
// ER("Eritrea", "ERI", 232),
//
/** Spain */
ES("Spain", "ESP", 724),
//
// /** Ethiopia */
// ET("Ethiopia", "ETH", 231),
//
/** Finland */
FI("Finland", "FIN", 246),
//
// /** Fiji */
// FJ("Fiji", "FJI", 242),
//
// /**
// * Falkland Islands
// * (Malvinas)
// */
// FK("Falkland Islands", "FLK", 238),
//
// /**
// *
// * Federated States of Micronesia
// */
// FM("Federated States of Micronesia", "FSM", 583),
//
// /** Faroe Islands
// */
// FO("Faroe Islands", "FRO", 234),
//
/** France */
FR("France", "FRA", 250),
//
// /** Gabon */
// GA("Gabon", "GAB", 266),
//
/**
* United Kingdom
*/
GB("United Kingdom", "GBR", 826),
//
// /** Grenada */
// GD("Grenada", "GRD", 308),
//
// /** Georgia */
// GE("Georgia", "GEO", 268),
//
// /** French Guiana
// */
// GF("French Guiana", "GUF", 254),
//
// /** Guemsey */
// GG("Guemsey", "GGY", 831),
//
// /** Ghana */
// GH("Ghana", "GHA", 288),
//
// /** Gibraltar */
// GI("Gibraltar", "GIB", 292),
//
// /** Greenland */
// GL("Greenland", "GRL", 304),
//
// /** Gambia */
// GM("Gambia", "GMB", 270),
//
// /** Guinea */
// GN("Guinea", "GIN", 324),
//
// /** Guadeloupe */
// GP("Guadeloupe", "GLP", 312),
//
// /**
// * Equatorial
// * Guinea
// */
// GQ("Equatorial Guinea", "GNQ", 226),
//
/** Greece */
GR("Greece", "GRC", 300),
//
// /**
// * South Georgia and the South Sandwich Islands
// */
// GS("South Georgia and the South Sandwich Islands", "SGS", 239),
//
// /** Guatemala */
// GT("Guatemala", "GTM", 320),
//
// /** Guam */
// GU("Guam", "GUM", 316),
//
// /** Guinea-Bissau
// */
// GW("Guinea-Bissau", "GNB", 624),
//
// /** Guyana */
// GY("Guyana", "GUY", 328),
//
// /** Hong Kong */
// HK("Hong Kong", "HKG", 344),
//
// /**
// *
// * Heard Island and McDonald Islands
// */
// HM("Heard Island and McDonald Islands", "HMD", 334),
//
// /** Honduras */
// HN("Honduras", "HND", 340),
//
// /** Croatia */
// HR("Croatia", "HRV", 191),
//
// /** Haiti */
// HT("Haiti", "HTI", 332),
//
/** Hungary */
HU("Hungary", "HUN", 348),
//
// /** Indonesia */
// ID("Indonesia", "IDN", 360),
//
/** Ireland */
IE("Ireland", "IRL", 372),
//
// /** Israel */
// IL("Israel", "ISR", 376),
//
// /** Isle of Man */
// IM("Isle of Man", "IMN", 833),
//
/** India */
IN("India", "IND", 356),
//
// /**
// *
// * British Indian Ocean Territory
// */
// IO("British Indian Ocean Territory", "IOT", 86),
//
// /** Iraq */
// IQ("Iraq", "IRQ", 368),
//
// /** Islamic Republic of
// Iran */
// IR("Islamic Republic of Iran", "IRN", 364),
//
/** Iceland */
IS("Iceland", "ISL", 352),
//
/** Italy */
IT("Italy", "ITA", 380),
//
// /** Jersey */
// JE("Jersey", "JEY", 832),
//
// /** Jamaica */
// JM("Jamaica", "JAM", 388),
//
// /** Jordan */
// JO("Jordan", "JOR", 400),
//
/** Japan */
JP("Japan", "JPN", 392),
//
// /** Kenya */
// KE("Kenya", "KEN", 404),
//
// /** Kyrgyzstan */
// KG("Kyrgyzstan", "KGZ", 417),
//
// /** Cambodia */
// KH("Cambodia", "KHM", 116),
//
// /** Kiribati */
// KI("Kiribati", "KIR", 296),
//
// /** Comoros */
// KM("Comoros", "COM", 174),
//
// /**
// * Saint Kitts
// * and Nevis
// */
// KN("Saint Kitts and Nevis", "KNA", 659),
//
// /**
// * Democratic People's
// * Republic of Korea
// */
// KP("Democratic People's Republic of Korea", "PRK", 408),
//
// /** Republic of
// Korea */
// KR("Republic of Korea", "KOR", 410),
//
// /** Kuwait */
// KW("Kuwait", "KWT", 414),
//
// /** Cayman
// Islands */
// KY("Cayman Islands", "CYM", 136),
//
// /** Kazakhstan */
// KZ("Kazakhstan", "KAZ", 398),
//
// /**
// * Lao People's Democratic
// * Republic
// */
// LA("Lao People's Democratic Republic", "LAO", 418),
//
// /** Lebanon */
// LB("Lebanon", "LBN", 422),
//
// /** Saint Lucia */
// LC("Saint Lucia", "LCA", 662),
//
// /** Liechtenstein
// */
// LI("Liechtenstein", "LIE", 438),
//
// /** Sri Lanka */
// LK("Sri Lanka", "LKA", 144),
//
// /** Liberia */
// LR("Liberia", "LBR", 430),
//
// /** Lesotho */
// LS("Lesotho", "LSO", 426),
//
// /** Lithuania */
// LT("Lithuania", "LTU", 440),
//
// /** Luxembourg */
// LU("Luxembourg", "LUX", 442),
//
// /** Latvia */
// LV("Latvia", "LVA", 428),
//
// /** Libya */
// LY("Libya", "LBY", 434),
//
// /** Morocco */
// MA("Morocco", "MAR", 504),
//
// /** Monaco */
// MC("Monaco", "MCO", 492),
//
// /** Republic of Moldova
// */
// MD("Republic of Moldova", "MDA", 498),
//
// /** Montenegro */
// ME("Montenegro", "MNE", 499),
//
// /**
// * Saint
// * Martin (French part)
// */
// MF("Saint Martin", "MAF", 663),
//
// /** Madagascar */
// MG("Madagascar", "MDG", 450),
//
// /**
// * Marshall
// * Islands
// */
// MH("Marshall Islands", "MHL", 584),
//
// /**
// * The former
// * Yugoslav Republic of Macedonia
// */
// MK("The former Yugoslav Republic of Macedonia", "MKD", 807),
//
// /** Mali */
// ML("Mali", "MLI", 466),
//
// /** Myanmar */
// MM("Myanmar", "MMR", 104),
//
// /** Mongolia */
// MN("Mongolia", "MNG", 496),
//
// /** Macao */
// MO("Macao", "MCO", 492),
//
// /**
// * Northern
// * Mariana Islands
// */
// MP("Northern Mariana Islands", "MNP", 580),
//
// /** Martinique */
// MQ("Martinique", "MTQ", 474),
//
// /** Mauritania */
// MR("Mauritania", "MRT", 478),
//
// /** Montserrat */
// MS("Montserrat", "MSR", 500),
//
// /** Malta */
// MT("Malta", "MLT", 470),
//
// /** Mauritius */
// MU("Mauritius", "MUS", 480),
//
// /** Maldives */
// MV("Maldives", "MDV", 462),
//
// /** Malawi */
// MW("Malawi", "MWI", 454),
//
// /** Mexico */
// MX("Mexico", "MEX", 484),
//
// /** Malaysia */
// MY("Malaysia", "MYS", 458),
//
// /** Mozambique */
// MZ("Mozambique", "MOZ", 508),
//
// /** Namibia */
// NA("Namibia", "NAM", 516),
//
// /** New Caledonia
// */
// NC("New Caledonia", "NCL", 540),
//
// /** Niger */
// NE("Niger", "NER", 562),
//
// /** Norfolk
// Island */
// NF("Norfolk Island", "NFK", 574),
//
// /** Nigeria */
// NG("Nigeria", "NGA", 566),
//
// /** Nicaragua */
// NI("Nicaragua", "NIC", 558),
//
/** Netherlands */
NL("Netherlands", "NLD", 528),
//
/** Norway */
NO("Norway", "NOR", 578),
//
// /** Nepal */
// NP("Nepal", "NPL", 524),
//
// /** Nauru */
// NR("Nauru", "NRU", 520),
//
// /** Niue */
// NU("Niue", "NIU", 570),
//
/** New Zealand */
NZ("New Zealand", "NZL", 554),
//
// /** Oman */
// OM("Oman", "OMN", 512),
//
// /** Panama */
// PA("Panama", "PAN", 591),
//
// /** Peru */
// PE("Peru", "PER", 604),
//
// /**
// * French
// * Polynesia
// */
// PF("French Polynesia", "PYF", 258),
//
// /**
// * Papua New
// * Guinea
// */
// PG("Papua New Guinea", "PNG", 598),
//
// /** Philippines */
// PH("Philippines", "PHL", 608),
//
// /** Pakistan */
// PK("Pakistan", "PAK", 586),
//
/** Poland */
PL("Poland", "POL", 616),
//
// /**
// * Saint
// * Pierre and Miquelon
// */
// PM("Saint Pierre and Miquelon", "SPM", 666),
//
// /** Pitcairn */
// PN("Pitcairn", "PCN", 612),
//
// /** Puerto Rico */
// PR("Puerto Rico", "PRI", 630),
//
// /**
// * Occupied
// * Palestinian Territory
// */
// PS("Occupied Palestinian Territory", "PSE", 275),
//
/** Portugal */
PT("Portugal", "PRT", 620),
//
// /** Palau */
// PW("Palau", "PLW", 585),
//
// /** Paraguay */
// PY("Paraguay", "PRY", 600),
//
// /** Qatar */
// QA("Qatar", "QAT", 634),
//
// /** Réunion
// */
// RE("R\u00E9union", "REU", 638),
//
/** Romania */
RO("Romania", "ROU", 642),
//
// /** Serbia */
// RS("Serbia", "SRB", 688),
//
/** Russian Federation */
RU("Russian Federation", "RUS", 643),
//
// /** Rwanda */
// RW("Rwanda", "RWA", 646),
//
// /** Saudi Arabia */
// SA("Saudi Arabia", "SAU", 682),
//
// /** Solomon
// Islands */
// SB("Solomon Islands", "SLB", 90),
//
// /** Seychelles */
// SC("Seychelles", "SYC", 690),
//
// /** Sudan */
// SD("Sudan", "SDN", 729),
//
/** Sweden */
SE("Sweden", "SWE", 752),
//
// /** Singapore */
// SG("Singapore", "SGP", 702),
//
// /**
// * Saint Helena, Ascension and Tristan da Cunha
// */
// SH("Saint Helena, Ascension and Tristan da Cunha", "SHN", 654),
//
// /** Slovenia */
// SI("Slovenia", "SVN", 705),
//
// /**
// * Svalbard
// and
// * Jan Mayen
// */
// SJ("Svalbard and Jan Mayen", "SJM", 744),
//
// /** Slovakia */
// SK("Slovakia", "SVK", 703),
//
// /** Sierra Leone */
// SL("Sierra Leone", "SLE", 694),
//
// /** San Marino */
// SM("San Marino", "SMR", 674),
//
// /** Senegal */
// SN("Senegal", "SEN", 686),
//
// /** Somalia */
// SO("Somalia", "SOM", 706),
//
// /** Suriname */
// SR("Suriname", "SUR", 740),
//
// /** South Sudan */
// SS("South Sudan", "SSD", 728),
//
// /**
// *
// * Sao Tome and Principe
// */
// ST("Sao Tome and Principe", "STP", 678),
//
// /** El Salvador */
// SV("El Salvador", "SLV", 222),
//
// /**
// * Sint Maarten (Dutch
// * part)
// */
// SX("Sint Maarten", "SXM", 534),
//
// /** Syrian Arab Republic
// */
// SY("Syrian Arab Republic", "SYR", 760),
//
// /** Swaziland */
// SZ("Swaziland", "SWZ", 748),
//
// /**
// * Turks and
// * Caicos Islands
// */
// TC("Turks and Caicos Islands", "TCA", 796),
//
// /** Chad */
// TD("Chad", "TCD", 148),
//
// /**
// *
// * French Southern Territories
// */
// TF("French Southern Territories", "ATF", 260),
//
// /** Togo */
// TG("Togo", "TGO", 768),
//
/** Thailand */
TH("Thailand", "THA", 764),
//
// /** Tajikistan */
// TJ("Tajikistan", "TJK", 762),
//
// /** Tokelau */
// TK("Tokelau", "TKL", 772),
//
// /** Timor-Leste */
// TL("Timor-Leste", "TLS", 626),
//
// /** Turkmenistan */
// TM("Turkmenistan", "TKM", 795),
//
// /** Tunisia */
// TN("Tunisia", "TUN", 788),
//
// /** Tonga */
// TO("Tonga", "TON", 776),
//
// /** Turkey */
// TR("Turkey", "TUR", 792),
//
// /**
// * Trinidad and
// * Tobago
// */
// TT("Trinidad and Tobago", "TTO", 780),
//
// /** Tuvalu */
// TV("Tuvalu", "TUV", 798),
//
// /**
// * Taiwan, Province of
// China
// */
// TW("Taiwan, Province of China", "TWN", 158),
//
// /**
// * United Republic of
// * Tanzania
// */
// TZ("United Republic of Tanzania", "TZA", 834),
//
// /** Ukraine */
// UA("Ukraine", "UKR", 804),
//
// /** Uganda */
// UG("Uganda", "UGA", 800),
//
// /**
// *
// * United States Minor Outlying Islands
// */
// UM("United States Minor Outlying Islands", "UMI", 581),
/** United States */
US("United States", "USA", 840),
// /** Uruguay */
// UY("Uruguay", "URY", 858),
//
// /** Uzbekistan */
// UZ("Uzbekistan", "UZB", 860),
//
// /**
// * Holy See (Vatican
// City
// * State)
// */
// VA("Holy See", "VAT", 336),
//
// /**
// *
// * Saint Vincent and the Grenadines
// */
// VC("Saint Vincent and the Grenadines", "VCT", 670),
//
// /**
// * Bolivarian Republic of
// * Venezuela
// */
// VE("Bolivarian Republic of Venezuela", "VEN", 862),
//
// /**
// * British
// * Virgin Islands
// */
// VG("British Virgin Islands", "VGB", 92),
//
// /**
// * Virgin
// * Islands, U.S.
// */
// VI("Virgin Islands, U.S.", "VIR", 850),
//
// /** Viet Nam */
// VN("Viet Nam", "VNM", 704),
//
// /** Vanuatu */
// VU("Vanuatu", "VUT", 548),
//
// /**
// * Wallis and
// * Futuna
// */
// WF("Wallis and Futuna", "WLF", 876),
//
// /** Samoa */
// WS("Samoa", "WSM", 882),
//
// /** Yemen */
// YE("Yemen", "YEM", 887),
//
// /** Mayotte */
// YT("Mayotte", "MYT", 175),
//
// /** South Africa */
// ZA("South Africa", "ZAF", 710),
//
// /** Zambia */
// ZM("Zambia", "ZMB", 894),
//
// /** Zimbabwe */
// ZW("Zimbabwe", "ZWE", 716), ; //NOSONAR
// @formatter:on
;
private static final Map alpha3Map = new HashMap<>();
private static final Map numericMap = new HashMap<>();
static {
for (CountryCode cc : values()) {
alpha3Map.put(cc.getAlpha3(), cc);
numericMap.put(cc.getNumeric(), cc);
}
}
private final String name;
private final String alpha3;
private final int numeric;
private CountryCode(String name, String alpha3, int numeric) {
this.name = name;
this.alpha3 = alpha3;
this.numeric = numeric;
}
/**
* Get the country name.
*
* @return The country name.
*/
public String getName() {
return name;
}
/**
* Get the ISO 3166-1 alpha-2 code.
*
* @return The ISO 3166-1 alpha-2 code.
*/
public String getAlpha2() {
return name();
}
/**
* Get the ISO 3166-1 alpha-3 code.
*
* @return The ISO 3166-1 alpha-3 code.
*/
public String getAlpha3() {
return alpha3;
}
/**
* Get the ISO 3166-1 numeric code.
*
* @return The ISO 3166-1 numeric code.
*/
public int getNumeric() {
return numeric;
}
/**
* Get a CountryCode that corresponds to a given ISO 3166-1 alpha-2 or
* alpha-3 code.
*
* @param code
* An ISO 3166-1 alpha-2 or
* alpha-3 code.
*
* @return A CountryCode instance, or null if not found.
*/
public static CountryCode getByCode(String code) {
if (code == null) {
return null;
}
switch (code.length()) {
case 2:
return getByAlpha2Code(code);
case 3:
return getByAlpha3Code(code);
default:
return null;
}
}
private static CountryCode getByAlpha2Code(String code) {
try {
return Enum.valueOf(CountryCode.class, code);
}
catch (IllegalArgumentException e) {
return null;
}
}
private static CountryCode getByAlpha3Code(String code) {
return alpha3Map.get(code);
}
/**
* Get a CountryCode that corresponds to a given ISO 3166-1 numeric code.
*
* @param code
* An ISO 3166-1 numeric code.
*
* @return A CountryCode instance, or null if not found.
*/
public static CountryCode getByCode(int code) {
return numericMap.get(code);
}
/**
* Get the CountryCode for the systems default locale or CountryCode.US
*
* @return the default CountryCode
*/
public static CountryCode getDefault() {
Locale defaultLocale = Locale.getDefault();
CountryCode countryCode = getByCode(defaultLocale.getCountry());
if (countryCode == null) {
countryCode = US;
}
return countryCode;
}
@Override
public String toString() {
return getName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy