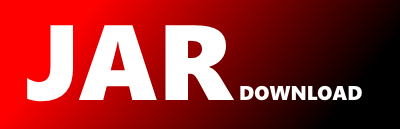
org.tinymediamanager.jsonrpc.api.call.GUI Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kodi-json-rpc Show documentation
Show all versions of kodi-json-rpc Show documentation
This library is the result of freezy's Kodi JSON introspection, merged with dereulenspiegel's adoption
without android, and patched to Kodi 16 Jarvis.
The newest version!
/*
* Copyright (C) 2005-2013 Team XBMC
* http://xbmc.org
*
* This Program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2, or (at your option)
* any later version.
*
* This Program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with XBMC Remote; see the file license. If not, write to
* the Free Software Foundation, 675 Mass Ave, Cambridge, MA 02139, USA.
* http://www.gnu.org/copyleft/gpl.html
*
*/
package org.tinymediamanager.jsonrpc.api.call;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
import org.codehaus.jackson.JsonNode;
import org.codehaus.jackson.node.ArrayNode;
import org.codehaus.jackson.node.ObjectNode;
import org.tinymediamanager.jsonrpc.api.AbstractCall;
import org.tinymediamanager.jsonrpc.api.model.GUIModel;
import org.tinymediamanager.jsonrpc.api.model.GlobalModel;
public final class GUI {
/**
* Activates the given window.
*
* This class represents the API method GUI.ActivateWindow
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class ActivateWindow extends AbstractCall {
public final static String API_TYPE = "GUI.ActivateWindow";
/**
* Activates the given window.
*
* @param window
* One of: home, programs, pictures, filemanager, files, settings, music,
* video, videos, pvr, tvchannels, tvrecordings, tvguide, tvtimers,
* tvsearch, radiochannels, radiorecordings, radioguide, radiotimers, radiosearch,
* pvrguideinfo, pvrrecordinginfo, pvrradiordsinfo, pvrtimersetting, pvrgroupmanager,
* pvrchannelmanager, pvrguidesearch, pvrchannelscan, pvrupdateprogress, pvrosdchannels,
* pvrosdguide, pvrosdteletext, systeminfo, testpattern, screencalibration,
* guicalibration, picturessettings, programssettings, weathersettings, musicsettings,
* systemsettings, videossettings, networksettings, servicesettings, appearancesettings,
* pvrsettings, tvsettings, scripts, videofiles, videolibrary, videoplaylist,
* loginscreen, profiles, skinsettings, addonbrowser, yesnodialog, progressdialog,
* virtualkeyboard, volumebar, submenu, favourites, contextmenu, infodialog,
* numericinput, gamepadinput, shutdownmenu, mutebug, playercontrols, seekbar,
* musicosd, addonsettings, visualisationsettings, visualisationpresetlist, osdvideosettings
* , osdaudiosettings, audiodspmanager, osdaudiodspsettings, videobookmarks, filebrowser,
* networksetup, mediasource, profilesettings, locksettings, contentsettings,
* songinformation, smartplaylisteditor, smartplaylistrule, busydialog, pictureinfo,
* accesspoints, fullscreeninfo, sliderdialog, addoninformation, subtitlesearch,
* musicplaylist, musicfiles, musiclibrary, musicplaylisteditor, teletext,
* selectdialog, musicinformation, okdialog, movieinformation, textviewer,
* fullscreenvideo, fullscreenlivetv, fullscreenradio, visualisation, slideshow,
* weather, screensaver, videoosd, videomenu, videotimeseek, startwindow,
* startup, peripheralsettings, extendedprogressdialog, mediafilter, addon,
* eventlog. See constants at {@link GUIModel.Window}.
* @param parameters
*/
public ActivateWindow(String window, String... parameters) {
super();
addParameter("window", window);
addParameter("parameters", parameters);
}
/**
* Activates the given window.
*
* @param window
* One of: home, programs, pictures, filemanager, files, settings, music,
* video, videos, pvr, tvchannels, tvrecordings, tvguide, tvtimers,
* tvsearch, radiochannels, radiorecordings, radioguide, radiotimers, radiosearch,
* pvrguideinfo, pvrrecordinginfo, pvrradiordsinfo, pvrtimersetting, pvrgroupmanager,
* pvrchannelmanager, pvrguidesearch, pvrchannelscan, pvrupdateprogress, pvrosdchannels,
* pvrosdguide, pvrosdteletext, systeminfo, testpattern, screencalibration,
* guicalibration, picturessettings, programssettings, weathersettings, musicsettings,
* systemsettings, videossettings, networksettings, servicesettings, appearancesettings,
* pvrsettings, tvsettings, scripts, videofiles, videolibrary, videoplaylist,
* loginscreen, profiles, skinsettings, addonbrowser, yesnodialog, progressdialog,
* virtualkeyboard, volumebar, submenu, favourites, contextmenu, infodialog,
* numericinput, gamepadinput, shutdownmenu, mutebug, playercontrols, seekbar,
* musicosd, addonsettings, visualisationsettings, visualisationpresetlist, osdvideosettings
* , osdaudiosettings, audiodspmanager, osdaudiodspsettings, videobookmarks, filebrowser,
* networksetup, mediasource, profilesettings, locksettings, contentsettings,
* songinformation, smartplaylisteditor, smartplaylistrule, busydialog, pictureinfo,
* accesspoints, fullscreeninfo, sliderdialog, addoninformation, subtitlesearch,
* musicplaylist, musicfiles, musiclibrary, musicplaylisteditor, teletext,
* selectdialog, musicinformation, okdialog, movieinformation, textviewer,
* fullscreenvideo, fullscreenlivetv, fullscreenradio, visualisation, slideshow,
* weather, screensaver, videoosd, videomenu, videotimeseek, startwindow,
* startup, peripheralsettings, extendedprogressdialog, mediafilter, addon,
* eventlog. See constants at {@link GUIModel.Window}.
*/
public ActivateWindow(String window) {
super();
addParameter("window", window);
}
@Override
protected String parseOne(JsonNode node) {
return node.getTextValue();
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
}
/**
* Retrieves the values of the given properties.
*
* This class represents the API method GUI.GetProperties
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class GetProperties extends AbstractCall {
public final static String API_TYPE = "GUI.GetProperties";
/**
* Retrieves the values of the given properties.
*
* @param properties
* One or more of: currentwindow, currentcontrol, skin, fullscreen, stereoscopicmode. See
* constants at {@link GUIModel.PropertyName}.
*/
public GetProperties(String... properties) {
super();
addParameter("properties", properties);
}
@Override
protected GUIModel.PropertyValue parseOne(JsonNode node) {
return new GUIModel.PropertyValue(node);
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
}
/**
* Returns the supported stereoscopic modes of the GUI.
*
* This class represents the API method GUI.GetStereoscopicModes
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class GetStereoscopicModes extends AbstractCall {
public final static String API_TYPE = "GUI.GetStereoscopicModes";
public final static String RESULT = "stereoscopicmodes";
/**
* Returns the supported stereoscopic modes of the GUI.
*/
public GetStereoscopicModes() {
super();
}
@Override
protected ArrayList parseMany(JsonNode node) {
final ArrayNode stereoscopicmodes = parseResults(node, RESULT);
if (stereoscopicmodes != null) {
final ArrayList ret = new ArrayList(stereoscopicmodes.size());
for (int i = 0; i < stereoscopicmodes.size(); i++) {
final ObjectNode item = (ObjectNode) stereoscopicmodes.get(i);
ret.add(new GUIModel.StereoscopyMode(item));
}
return ret;
}
else {
return new ArrayList(0);
}
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return true;
}
}
/**
* Toggle fullscreen/GUI.
*
* This class represents the API method GUI.SetFullscreen
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class SetFullscreen extends AbstractCall {
public final static String API_TYPE = "GUI.SetFullscreen";
/**
* Toggle fullscreen/GUI.
*
* @param fullscreen
*/
public SetFullscreen(GlobalModel.Toggle fullscreen) {
super();
addParameter("fullscreen", fullscreen);
}
@Override
protected Boolean parseOne(JsonNode node) {
return node.getBooleanValue();
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
}
/**
* Sets the stereoscopic mode of the GUI to the given mode.
*
* This class represents the API method GUI.SetStereoscopicMode
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class SetStereoscopicMode extends AbstractCall {
public final static String API_TYPE = "GUI.SetStereoscopicMode";
/**
* Sets the stereoscopic mode of the GUI to the given mode.
*
* @param mode
* One of: toggle, tomono, next, previous, select, off, split_vertical,
* split_horizontal, row_interleaved, hardware_based, anaglyph_cyan_red,
* anaglyph_green_magenta, monoscopic. See constants at {@link GUI.SetStereoscopicMode.Mode}.
*/
public SetStereoscopicMode(String mode) {
super();
addParameter("mode", mode);
}
@Override
protected String parseOne(JsonNode node) {
return node.getTextValue();
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
/**
* API Name: mode
*/
public interface Mode {
public final String TOGGLE = "toggle";
public final String TOMONO = "tomono";
public final String NEXT = "next";
public final String PREVIOUS = "previous";
public final String SELECT = "select";
public final String OFF = "off";
public final String SPLIT_VERTICAL = "split_vertical";
public final String SPLIT_HORIZONTAL = "split_horizontal";
public final String ROW_INTERLEAVED = "row_interleaved";
public final String HARDWARE_BASED = "hardware_based";
public final String ANAGLYPH_CYAN_RED = "anaglyph_cyan_red";
public final String ANAGLYPH_GREEN_MAGENTA = "anaglyph_green_magenta";
public final String MONOSCOPIC = "monoscopic";
public final static Set values = new HashSet(Arrays.asList(TOGGLE, TOMONO, NEXT, PREVIOUS, SELECT, OFF,
SPLIT_VERTICAL, SPLIT_HORIZONTAL, ROW_INTERLEAVED, HARDWARE_BASED, ANAGLYPH_CYAN_RED, ANAGLYPH_GREEN_MAGENTA, MONOSCOPIC));
}
}
/**
* Shows a GUI notification.
*
* This class represents the API method GUI.ShowNotification
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class ShowNotification extends AbstractCall {
public final static String API_TYPE = "GUI.ShowNotification";
/**
* Shows a GUI notification.
*
* @param title
* @param message
* @param image
* @param displaytime
* The time in milliseconds the notification will be visible.
*/
public ShowNotification(String title, String message, String image, Integer displaytime) {
super();
addParameter("title", title);
addParameter("message", message);
addParameter("image", image);
addParameter("displaytime", displaytime);
}
/**
* Shows a GUI notification.
*
* @param title
* @param message
*/
public ShowNotification(String title, String message) {
super();
addParameter("title", title);
addParameter("message", message);
}
/**
* Shows a GUI notification.
*
* @param title
* @param message
* @param image
*/
public ShowNotification(String title, String message, String image) {
super();
addParameter("title", title);
addParameter("message", message);
addParameter("image", image);
}
/**
* Shows a GUI notification.
*
* @param title
* @param message
* @param displaytime
* The time in milliseconds the notification will be visible.
*/
public ShowNotification(String title, String message, Integer displaytime) {
super();
addParameter("title", title);
addParameter("message", message);
addParameter("displaytime", displaytime);
}
@Override
protected String parseOne(JsonNode node) {
return node.getTextValue();
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy