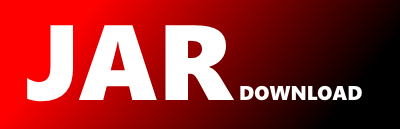
org.tinymediamanager.jsonrpc.api.call.JSONRPC Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kodi-json-rpc Show documentation
Show all versions of kodi-json-rpc Show documentation
This library is the result of freezy's Kodi JSON introspection, merged with dereulenspiegel's adoption
without android, and patched to Kodi 16 Jarvis.
The newest version!
/*
* Copyright (C) 2005-2013 Team XBMC
* http://xbmc.org
*
* This Program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2, or (at your option)
* any later version.
*
* This Program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with XBMC Remote; see the file license. If not, write to
* the Free Software Foundation, 675 Mass Ave, Cambridge, MA 02139, USA.
* http://www.gnu.org/copyleft/gpl.html
*
*/
package org.tinymediamanager.jsonrpc.api.call;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.codehaus.jackson.JsonNode;
import org.codehaus.jackson.node.ArrayNode;
import org.codehaus.jackson.node.ObjectNode;
import org.tinymediamanager.jsonrpc.api.AbstractCall;
import org.tinymediamanager.jsonrpc.api.AbstractModel;
public final class JSONRPC {
/**
* Notify all other connected clients.
*
* This class represents the API method JSONRPC.NotifyAll
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class NotifyAll extends AbstractCall {
public final static String API_TYPE = "JSONRPC.NotifyAll";
/**
* Notify all other connected clients.
*
* @param sender
* @param message
* @param data
*/
public NotifyAll(String sender, String message, String data) {
super();
addParameter("sender", sender);
addParameter("message", message);
addParameter("data", data);
}
/**
* Notify all other connected clients.
*
* @param sender
* @param message
*/
public NotifyAll(String sender, String message) {
super();
addParameter("sender", sender);
addParameter("message", message);
}
@Override
protected String parseOne(JsonNode node) {
return node.getTextValue();
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
}
/**
* Retrieve the clients permissions.
*
* This class represents the API method JSONRPC.Permission
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class Permission extends AbstractCall {
public final static String API_TYPE = "JSONRPC.Permission";
/**
* Retrieve the clients permissions.
*/
public Permission() {
super();
}
@Override
protected PermissionResult parseOne(JsonNode node) {
return new PermissionResult(node);
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
/**
* Note: This class is used as result only.
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class PermissionResult extends AbstractModel {
// field names
public static final String CONTROLGUI = "controlgui";
public static final String CONTROLNOTIFY = "controlnotify";
public static final String CONTROLPLAYBACK = "controlplayback";
public static final String CONTROLPOWER = "controlpower";
public static final String CONTROLPVR = "controlpvr";
public static final String CONTROLSYSTEM = "controlsystem";
public static final String EXECUTEADDON = "executeaddon";
public static final String MANAGEADDON = "manageaddon";
public static final String NAVIGATE = "navigate";
public static final String READDATA = "readdata";
public static final String REMOVEDATA = "removedata";
public static final String UPDATEDATA = "updatedata";
public static final String WRITEFILE = "writefile";
// class members
public final Boolean controlgui;
public final Boolean controlnotify;
public final Boolean controlplayback;
public final Boolean controlpower;
public final Boolean controlpvr;
public final Boolean controlsystem;
public final Boolean executeaddon;
public final Boolean manageaddon;
public final Boolean navigate;
public final Boolean readdata;
public final Boolean removedata;
public final Boolean updatedata;
public final Boolean writefile;
/**
* @param controlgui
* @param controlnotify
* @param controlplayback
* @param controlpower
* @param controlpvr
* @param controlsystem
* @param executeaddon
* @param manageaddon
* @param navigate
* @param readdata
* @param removedata
* @param updatedata
* @param writefile
*/
public PermissionResult(Boolean controlgui, Boolean controlnotify, Boolean controlplayback, Boolean controlpower, Boolean controlpvr,
Boolean controlsystem, Boolean executeaddon, Boolean manageaddon, Boolean navigate, Boolean readdata, Boolean removedata,
Boolean updatedata, Boolean writefile) {
this.controlgui = controlgui;
this.controlnotify = controlnotify;
this.controlplayback = controlplayback;
this.controlpower = controlpower;
this.controlpvr = controlpvr;
this.controlsystem = controlsystem;
this.executeaddon = executeaddon;
this.manageaddon = manageaddon;
this.navigate = navigate;
this.readdata = readdata;
this.removedata = removedata;
this.updatedata = updatedata;
this.writefile = writefile;
}
/**
* Construct from JSON object.
*
* @param node
* JSON object representing a PermissionResult object
*/
public PermissionResult(JsonNode node) {
controlgui = node.get(CONTROLGUI).getBooleanValue(); // required value
controlnotify = node.get(CONTROLNOTIFY).getBooleanValue(); // required value
controlplayback = node.get(CONTROLPLAYBACK).getBooleanValue(); // required value
controlpower = node.get(CONTROLPOWER).getBooleanValue(); // required value
controlpvr = node.get(CONTROLPVR).getBooleanValue(); // required value
controlsystem = node.get(CONTROLSYSTEM).getBooleanValue(); // required value
executeaddon = node.get(EXECUTEADDON).getBooleanValue(); // required value
manageaddon = node.get(MANAGEADDON).getBooleanValue(); // required value
navigate = node.get(NAVIGATE).getBooleanValue(); // required value
readdata = node.get(READDATA).getBooleanValue(); // required value
removedata = node.get(REMOVEDATA).getBooleanValue(); // required value
updatedata = node.get(UPDATEDATA).getBooleanValue(); // required value
writefile = node.get(WRITEFILE).getBooleanValue(); // required value
}
@Override
public JsonNode toJsonNode() {
final ObjectNode node = OM.createObjectNode();
node.put(CONTROLGUI, controlgui);
node.put(CONTROLNOTIFY, controlnotify);
node.put(CONTROLPLAYBACK, controlplayback);
node.put(CONTROLPOWER, controlpower);
node.put(CONTROLPVR, controlpvr);
node.put(CONTROLSYSTEM, controlsystem);
node.put(EXECUTEADDON, executeaddon);
node.put(MANAGEADDON, manageaddon);
node.put(NAVIGATE, navigate);
node.put(READDATA, readdata);
node.put(REMOVEDATA, removedata);
node.put(UPDATEDATA, updatedata);
node.put(WRITEFILE, writefile);
return node;
}
/**
* Extracts a list of {@link PermissionResult} objects from a JSON array.
*
* @param node
* ObjectNode containing the list of objects.
* @param key
* Key pointing to the node where the list is stored.
*/
static List getJSONRPCPermissionResultList(JsonNode node, String key) {
if (node.has(key)) {
final ArrayNode a = (ArrayNode) node.get(key);
final List l = new ArrayList(a.size());
for (int i = 0; i < a.size(); i++) {
l.add(new PermissionResult((JsonNode) a.get(i)));
}
return l;
}
return new ArrayList(0);
}
}
}
/**
* Ping responder.
*
* This class represents the API method JSONRPC.Ping
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class Ping extends AbstractCall {
public final static String API_TYPE = "JSONRPC.Ping";
/**
* Ping responder.
*/
public Ping() {
super();
}
@Override
protected String parseOne(JsonNode node) {
return node.getTextValue();
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
}
/**
* Retrieve the JSON-RPC protocol version.
*
* This class represents the API method JSONRPC.Version
*
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class Version extends AbstractCall {
public final static String API_TYPE = "JSONRPC.Version";
/**
* Retrieve the JSON-RPC protocol version.
*/
public Version() {
super();
}
@Override
protected VersionResult parseOne(JsonNode node) {
return new VersionResult(node);
}
@Override
public String getName() {
return API_TYPE;
}
@Override
protected boolean returnsList() {
return false;
}
/**
* Note: This class is used as result only.
* This class was generated automatically from XBMC's JSON-RPC introspect.
*/
public static class VersionResult extends AbstractModel {
// field names
public static final String MAJOR = "major";
public static final String MINOR = "minor";
public static final String PATCH = "patch";
public static final HashMap CODENAME;
static {
// https://kodi.wiki/?title=JSON-RPC_API#API_versions
CODENAME = new HashMap();
CODENAME.put("8.3.0", "Kodi 18 (Leia)");
CODENAME.put("8.0.0", "Kodi 17 (Krypton)");
CODENAME.put("6.32.5", "Kodi 16.1 (Jarvis)");
CODENAME.put("6.32.4", "Kodi 16.0 (Jarvis)");
CODENAME.put("6.25.2", "Kodi 15 (Isengard)");
CODENAME.put("6.21.2", "Kodi 14 (Helix)");
CODENAME.put("6.14.3", "XBMC 13 (Gotham)");
// don't care about the rest...
}
// class members
public final Integer major;
public final Integer minor;
public final Integer patch;
/**
* @param major
* @param minor
* @param patch
*/
public VersionResult(Integer major, Integer minor, Integer patch) {
this.major = major;
this.minor = minor;
this.patch = patch;
}
@Override
public String toString() {
return major + "." + minor + "." + patch;
}
public String getKodiVersion() {
String cn = CODENAME.get(toString());
if (cn == null) {
// https://github.com/xbmc/xbmc/blob/master/xbmc/interfaces/json-rpc/schema/version.txt
if (major == 8) {
cn = "Kodi 17 (Krypton)";
if (minor > 3) {
cn = "Kodi 18 (Leia)";
}
}
if (major >= 9) {
cn = "Kodi 18 (Leia)";
}
// STILL null?
if (cn == null) {
cn = "Unknown";
}
}
return cn;
}
/**
* Returns a comparable integer by multiplying and adding the version parts.
* Example: 2.19.625 becomes 20190625
*
* @return
*/
public int toInt() {
return patch + minor * 10000 + major * 10000000;
}
/**
* Construct from JSON object.
*
* @param node
* JSON object representing a VersionResult object
*/
public VersionResult(JsonNode node) {
major = node.findValue(MAJOR).getIntValue(); // required value
minor = node.findValue(MINOR).getIntValue(); // required value
patch = node.findValue(PATCH).getIntValue(); // required value
}
@Override
public JsonNode toJsonNode() {
final ObjectNode node = OM.createObjectNode();
node.put(MAJOR, major);
node.put(MINOR, minor);
node.put(PATCH, patch);
return node;
}
/**
* Extracts a list of {@link VersionResult} objects from a JSON array.
*
* @param node
* ObjectNode containing the list of objects.
* @param key
* Key pointing to the node where the list is stored.
*/
static List getJSONRPCVersionResultList(JsonNode node, String key) {
if (node.has(key)) {
final ArrayNode a = (ArrayNode) node.get(key);
final List l = new ArrayList(a.size());
for (int i = 0; i < a.size(); i++) {
l.add(new VersionResult((JsonNode) a.get(i)));
}
return l;
}
return new ArrayList(0);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy