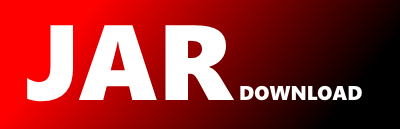
org.tinymediamanager.ui.components.tree.TmmTreeDataProvider Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 - 2019 Manuel Laggner
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.tinymediamanager.ui.components.tree;
import java.util.Comparator;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
import org.tinymediamanager.core.AbstractModelObject;
/**
* The class TmmTreeDataProvider is the base class for all data providers used with the TmmTree
*
* @author Manuel Laggner
*
* @param
*/
public abstract class TmmTreeDataProvider extends AbstractModelObject {
public final static String NODE_INSERTED = "nodeInserted";
public final static String NODE_CHANGED = "nodeChanged";
public final static String NODE_REMOVED = "nodeRemoved";
public final static String NODE_STRUCTURE_CHANGED = "nodeStructureChanged";
protected Set> treeFilters;
protected Comparator treeComparator = null;
protected final ReadWriteLock readWriteLock = new ReentrantReadWriteLock();
protected final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy