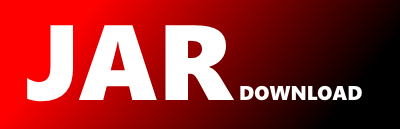
org.tinystruct.http.MemorySession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tinystruct Show documentation
Show all versions of tinystruct Show documentation
A simple framework for Java development. Simple is hard, Complex is easy. Better thinking, better design.
package org.tinystruct.http;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class MemorySession implements Session {
private final Map storage = new ConcurrentHashMap<>();
private final String sessionId;
private long expiry;
public MemorySession(String sessionId) {
this.sessionId = sessionId;
this.expiry = System.currentTimeMillis() + 1800000L;
}
@Override
public String getId() {
return this.sessionId;
}
@Override
public void setAttribute(String key, Object value) {
storage.put(key, value);
}
@Override
public Object getAttribute(String key) {
this.expiry = System.currentTimeMillis() + 1800000L;
return storage.get(key);
}
@Override
public void removeAttribute(String key) {
storage.remove(key);
}
@Override
public boolean isExpired() {
return System.currentTimeMillis() > expiry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy