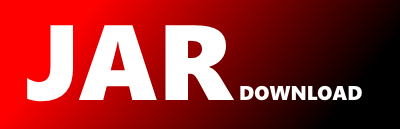
org.tinystruct.system.AnnotationProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tinystruct Show documentation
Show all versions of tinystruct Show documentation
A simple framework for Java development. Simple is hard, Complex is easy. Better thinking, better design.
package org.tinystruct.system;
import org.tinystruct.Application;
import org.tinystruct.application.ActionRegistry;
import org.tinystruct.system.annotation.Action;
import org.tinystruct.system.annotation.Argument;
import org.tinystruct.system.cli.CommandArgument;
import org.tinystruct.system.cli.CommandLine;
import org.tinystruct.system.cli.CommandOption;
import java.util.*;
public class AnnotationProcessor {
private final Application app;
private final ActionRegistry actionRegistry = ActionRegistry.getInstance();
public AnnotationProcessor(Application app) {
this.app = app;
}
public void processActionAnnotations() {
Action annotation = this.app.getClass().getAnnotation(Action.class);
if (annotation != null) {
Argument[] optionAnnotations = annotation.options();
List options = new ArrayList<>();
CommandLine commandLine = new CommandLine(this.app, annotation.value(), annotation.description());
this.app.getCommandLines().put(annotation.value(), commandLine);
for (Argument optionAnnotation : optionAnnotations) {
String key = optionAnnotation.key();
String optionDescription = optionAnnotation.description();
CommandOption option = new CommandOption(key, null, optionDescription);
options.add(option);
}
this.app.getCommandLines().get(annotation.value()).setOptions(options);
}
for (java.lang.reflect.Method method : this.app.getClass().getDeclaredMethods()) {
Action actionAnnotation = method.getAnnotation(Action.class);
if (actionAnnotation != null) {
String commandName = actionAnnotation.value();
String description = actionAnnotation.description();
String example = actionAnnotation.example();
String path = actionAnnotation.value();
org.tinystruct.application.Action.Mode mode = actionAnnotation.mode();
CommandLine commandLine = new CommandLine(this.app, commandName, description);
this.app.getCommandLines().put(commandName, commandLine);
Set> arguments = getCommandArguments(actionAnnotation);
this.app.getCommandLines().get(commandName).setArguments(arguments);
Argument[] optionAnnotations = actionAnnotation.options();
List options = new ArrayList<>();
for (Argument optionAnnotation : optionAnnotations) {
String key = optionAnnotation.key();
String optionDescription = optionAnnotation.description();
CommandOption option = new CommandOption(key, null, optionDescription);
options.add(option);
}
this.app.getCommandLines().get(commandName).setOptions(options);
if (!example.isEmpty())
this.app.getCommandLines().get(commandName).setExample(example);
// Register the action handler method
// Set the action in the action registry
if (mode != org.tinystruct.application.Action.Mode.All) {
this.actionRegistry.set(this.app, path, method.getName(), mode);
} else {
this.actionRegistry.set(this.app, path, method.getName());
}
// Exclude the command starting with '-'
if (path.indexOf("-") != 0)
this.app.createLinkVariable(path);
}
}
}
private static Set> getCommandArguments(Action actionAnnotation) {
Argument[] argumentAnnotations = actionAnnotation.arguments();
Set> arguments = new HashSet<>();
for (Argument argumentAnnotation : argumentAnnotations) {
String key = argumentAnnotation.key();
String argDescription = argumentAnnotation.description();
CommandArgument argument = new CommandArgument<>(key, null, argDescription);
arguments.add(argument);
}
return arguments;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy