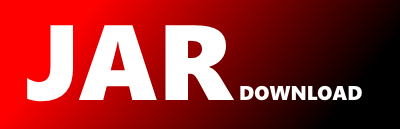
org.togglz.testing.vary.VariationSetBuilder Maven / Gradle / Ivy
package org.togglz.testing.vary;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Set;
import org.togglz.core.Feature;
import org.togglz.core.util.Validate;
/**
* Default implementation of {@link VariationSet} that allows to build the set dynamically.
*
* @param The feature class
* @author Christian Kaltepoth
*/
public class VariationSetBuilder implements VariationSet {
public static VariationSetBuilder create(Class featureClass) {
return new VariationSetBuilder<>(featureClass);
}
private final Class featureClass;
private final Set featuresToVary = new HashSet();
private final Set featuresToEnable = new HashSet();
private final Set featuresToDisable = new HashSet();
protected VariationSetBuilder(Class featureEnum) {
Validate.notNull(featureEnum, "The featureEnum argument is required");
Validate.isTrue(featureEnum.isEnum(), "This class only works with feature enums");
this.featureClass = featureEnum;
}
/**
* Vary this feature in the variation set.
*/
public VariationSetBuilder vary(F f) {
featuresToVary.add(f);
featuresToEnable.remove(f);
featuresToDisable.remove(f);
return this;
}
/**
* Enable this feature in the variation set.
*/
public VariationSetBuilder enable(F f) {
featuresToVary.remove(f);
featuresToEnable.add(f);
featuresToDisable.remove(f);
return this;
}
/**
* Disable this feature in the variation set.
*/
public VariationSetBuilder disable(F f) {
featuresToVary.remove(f);
featuresToEnable.remove(f);
featuresToDisable.add(f);
return this;
}
/**
* Enable all features in the variation set.
*/
public VariationSetBuilder enableAll() {
for (F f : featureClass.getEnumConstants()) {
enable(f);
}
return this;
}
/**
* Disable all features in the variation set.
*/
public VariationSetBuilder disableAll() {
for (F f : featureClass.getEnumConstants()) {
disable(f);
}
return this;
}
/*
* (non-Javadoc)
*
* @see org.togglz.junit.vary.VariationSet#getVariants()
*/
@Override
public Set> getVariants() {
// start with a single variant with all feature disabled
Set> variantSet = new LinkedHashSet<>();
variantSet.add(new HashSet());
for (F feature : featureClass.getEnumConstants()) {
// enable the feature in all variants
if (featuresToEnable.contains(feature)) {
for (Set variant : variantSet) {
variant.add(feature);
}
}
// disable the feature in all variants
else if (featuresToDisable.contains(feature)) {
for (Set variant : variantSet) {
variant.remove(feature);
}
}
// copy the existing variants, enable the feature in the copy, and merge them
else if (featuresToVary.contains(feature)) {
Set> copy = deepCopy(variantSet);
for (Set variant : copy) {
variant.add(feature);
}
variantSet.addAll(copy);
}
}
return variantSet;
}
private Set> deepCopy(Set> src) {
Set> copy = new LinkedHashSet>();
for (Set variant : src) {
copy.add(new HashSet(variant));
}
return copy;
}
@Override
public Class getFeatureClass() {
return featureClass;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy