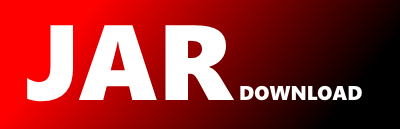
org.tomitribe.jamira.cli.cache.CachedMetadataRestClient Maven / Gradle / Ivy
/*
* Copyright 2021 Tomitribe and community
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.tomitribe.jamira.cli.cache;
import com.atlassian.jira.rest.client.api.MetadataRestClient;
import com.atlassian.jira.rest.client.api.domain.Field;
import com.atlassian.jira.rest.client.api.domain.IssueType;
import com.atlassian.jira.rest.client.api.domain.IssuelinksType;
import com.atlassian.jira.rest.client.api.domain.Priority;
import com.atlassian.jira.rest.client.api.domain.Resolution;
import com.atlassian.jira.rest.client.api.domain.ServerInfo;
import com.atlassian.jira.rest.client.api.domain.Status;
import io.atlassian.util.concurrent.Promise;
import org.tomitribe.jamira.cli.Cache;
import java.net.URI;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import static org.tomitribe.jamira.cli.Client.stream;
public class CachedMetadataRestClient implements MetadataRestClient {
private final MetadataRestClient client;
private final Cache cache;
public CachedMetadataRestClient(final MetadataRestClient client, final Cache cache) {
this.client = client;
this.cache = cache;
}
@Override
public Promise getIssueType(final URI uri) {
final Optional match = stream(getIssueTypes())
.filter(issueType -> issueType.getSelf().equals(uri))
.findFirst();
if (match.isPresent()) {
return new CompletedPromise<>(match.get());
}
return client.getIssueType(uri);
}
@Override
public Promise> getIssueTypes() {
final Cache.Entry entry = new Cache.Entry<>(cache.issueTypesJson(), CachedIssueType[].class);
if (entry.isFresh()) {
final CachedIssueType[] cachedIssueTypes = entry.read();
final List issueTypes = Stream.of(cachedIssueTypes)
.map(CachedIssueType::toIssueType)
.collect(Collectors.toList());
return new CompletedPromise<>(issueTypes);
}
final Iterable issueTypes = get(client.getIssueTypes());
{ // Cache the values
final List cachedIssueTypes = stream(issueTypes)
.map(CachedIssueType::fromIssueType)
.collect(Collectors.toList());
entry.write(cachedIssueTypes);
}
return new CompletedPromise<>(issueTypes);
}
private Iterable get(final Promise> issueTypes) {
try {
return issueTypes.get();
} catch (final Exception e) {
throw new IllegalStateException(e);
}
}
@Override
public Promise> getIssueLinkTypes() {
return client.getIssueLinkTypes();
}
@Override
public Promise getStatus(final URI uri) {
return client.getStatus(uri);
}
@Override
public Promise> getStatuses() {
final Cache.Entry entry = new Cache.Entry<>(cache.statusesJson(), CachedStatus[].class);
if (entry.isFresh()) {
final CachedStatus[] cachedStatuses = entry.read();
final List statuses = Stream.of(cachedStatuses)
.map(CachedStatus::toStatus)
.collect(Collectors.toList());
return new CompletedPromise<>(statuses);
}
final Iterable statuses = get(client.getStatuses());
{ // Cache the values
final List cachedStatuses = stream(statuses)
.map(CachedStatus::fromStatus)
.collect(Collectors.toList());
entry.write(cachedStatuses);
}
return new CompletedPromise<>(statuses);
}
@Override
public Promise getPriority(final URI uri) {
return client.getPriority(uri);
}
@Override
public Promise> getPriorities() {
final Cache.Entry entry = new Cache.Entry<>(cache.prioritiesJson(), CachedPriority[].class);
if (entry.isFresh()) {
final CachedPriority[] cachedPriorities = entry.read();
final List priorities = Stream.of(cachedPriorities)
.map(CachedPriority::toPriority)
.collect(Collectors.toList());
return new CompletedPromise<>(priorities);
}
final Iterable priorities = get(client.getPriorities());
{ // Cache the values
final List cachedPriorities = stream(priorities)
.map(CachedPriority::fromPriority)
.collect(Collectors.toList());
entry.write(cachedPriorities);
}
return new CompletedPromise<>(priorities);
}
@Override
public Promise getResolution(final URI uri) {
return client.getResolution(uri);
}
@Override
public Promise> getResolutions() {
final Cache.Entry entry = new Cache.Entry<>(cache.resolutionsJson(), CachedResolution[].class);
if (entry.isFresh()) {
final CachedResolution[] cachedResolutions = entry.read();
final List resolutions = Stream.of(cachedResolutions)
.map(CachedResolution::toResolution)
.collect(Collectors.toList());
return new CompletedPromise<>(resolutions);
}
final Iterable resolutions = get(client.getResolutions());
{ // Cache the values
final List cachedResolutions = stream(resolutions)
.map(CachedResolution::fromResolution)
.collect(Collectors.toList());
entry.write(cachedResolutions);
}
return new CompletedPromise<>(resolutions);
}
@Override
public Promise getServerInfo() {
return client.getServerInfo();
}
@Override
public Promise> getFields() {
return client.getFields();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy