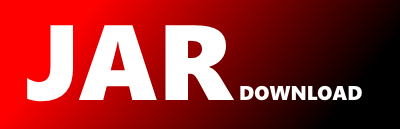
org.tp23.xgen.MutableNodelList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of generation-x Show documentation
Show all versions of generation-x Show documentation
Utility for generating XML using XPath like statements
package org.tp23.xgen;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
/**
* org.w3c.dom.NodeList implementation that accepts changes and implements Iterable.
*
* I wonder why all NodeList don't implement Iterable.
*
* @author teknopaul
*
*/
public class MutableNodelList implements NodeList, Iterable {
private List list = new ArrayList();
public MutableNodelList() {
}
public MutableNodelList(NodeList nodeList) {
addElements(nodeList);
}
@Override
public Node item(int index) {
return list.get(index);
}
@Override
public int getLength() {
return list.size();
}
public void add(Node node) {
list.add(node);
}
public void addElements(NodeList nodeList) {
for (int i = 0 ; i < nodeList.getLength() ; i++) {
list.add(nodeList.item(i));
}
}
@Override
public Iterator iterator() {
return new Iterator() {
int pos = 0;
@Override
public boolean hasNext() {
return getLength() > pos;
}
@Override
public Node next() {
return item(pos++);
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy