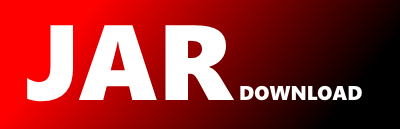
org.fife.ui.rsyntaxtextarea.modes.HTMLTokenMaker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rsyntaxtextarea Show documentation
Show all versions of rsyntaxtextarea Show documentation
A Logo interpreter written in Java.
/* The following code was generated by JFlex 1.4.1 on 12/30/16 12:26 AM */
/*
* 01/24/2005
*
* HTMLTokenMaker.java - Generates tokens for HTML syntax highlighting.
*
* This library is distributed under a modified BSD license. See the included
* RSyntaxTextArea.License.txt file for details.
*/
package org.fife.ui.rsyntaxtextarea.modes;
import java.io.IOException;
import java.util.Stack;
import javax.swing.text.Segment;
import org.fife.ui.rsyntaxtextarea.HtmlOccurrenceMarker;
import org.fife.ui.rsyntaxtextarea.OccurrenceMarker;
import org.fife.ui.rsyntaxtextarea.RSyntaxUtilities;
import org.fife.ui.rsyntaxtextarea.Token;
import org.fife.ui.rsyntaxtextarea.TokenImpl;
/**
* Scanner for HTML 5 files.
*
* This implementation was created using
* JFlex 1.4.1; however, the generated file
* was modified for performance. Memory allocation needs to be almost
* completely removed to be competitive with the handwritten lexers (subclasses
* of AbstractTokenMaker
, so this class has been modified so that
* Strings are never allocated (via yytext()), and the scanner never has to
* worry about refilling its buffer (needlessly copying chars around).
* We can achieve this because RText always scans exactly 1 line of tokens at a
* time, and hands the scanner this line as an array of characters (a Segment
* really). Since tokens contain pointers to char arrays instead of Strings
* holding their contents, there is no need for allocating new memory for
* Strings.
*
* The actual algorithm generated for scanning has, of course, not been
* modified.
*
* If you wish to regenerate this file yourself, keep in mind the following:
*
* - The generated
HTMLTokenMaker.java
file will contain two
* definitions of both zzRefill
and yyreset
.
* You should hand-delete the second of each definition (the ones
* generated by the lexer), as these generated methods modify the input
* buffer, which we'll never have to do.
* - You should also change the declaration/definition of zzBuffer to NOT
* be initialized. This is a needless memory allocation for us since we
* will be pointing the array somewhere else anyway.
* - You should NOT call
yylex()
on the generated scanner
* directly; rather, you should use getTokenList
as you would
* with any other TokenMaker
instance.
*
*
* @author Robert Futrell
* @version 0.9
*/
public class HTMLTokenMaker extends AbstractMarkupTokenMaker {
/** This character denotes the end of file */
public static final int YYEOF = -1;
/** lexical states */
public static final int INATTR_SINGLE_SCRIPT = 10;
public static final int JS_CHAR = 16;
public static final int CSS_STRING = 22;
public static final int JS_MLC = 17;
public static final int CSS_CHAR_LITERAL = 23;
public static final int INTAG_SCRIPT = 8;
public static final int JS_TEMPLATE_LITERAL_EXPR = 26;
public static final int CSS_PROPERTY = 20;
public static final int CSS_C_STYLE_COMMENT = 24;
public static final int CSS = 19;
public static final int CSS_VALUE = 21;
public static final int COMMENT = 1;
public static final int INATTR_DOUBLE_SCRIPT = 9;
public static final int PI = 2;
public static final int JAVASCRIPT = 14;
public static final int INTAG = 4;
public static final int INTAG_CHECK_TAG_NAME = 5;
public static final int INATTR_SINGLE_STYLE = 13;
public static final int DTD = 3;
public static final int JS_EOL_COMMENT = 18;
public static final int INATTR_DOUBLE_STYLE = 12;
public static final int INATTR_SINGLE = 7;
public static final int JS_TEMPLATE_LITERAL = 25;
public static final int YYINITIAL = 0;
public static final int INATTR_DOUBLE = 6;
public static final int JS_STRING = 15;
public static final int INTAG_STYLE = 11;
/**
* Translates characters to character classes
*/
private static final String ZZ_CMAP_PACKED =
"\11\0\1\4\1\2\1\0\1\1\1\33\22\0\1\4\1\51\1\7"+
"\1\34\1\36\1\50\1\5\1\110\1\105\1\104\1\37\1\42\1\45"+
"\1\31\1\43\1\10\1\25\6\125\1\27\2\24\1\53\1\6\1\3"+
"\1\46\1\17\1\52\1\103\1\112\1\26\1\12\1\116\1\22\1\41"+
"\1\120\1\124\1\14\1\126\1\121\1\21\1\115\1\114\1\113\1\15"+
"\1\122\1\13\1\11\1\16\1\117\1\123\1\23\1\40\1\20\1\23"+
"\1\107\1\35\1\107\1\47\1\30\1\130\1\71\1\102\1\66\1\70"+
"\1\76\1\73\1\57\1\65\1\56\1\126\1\100\1\67\1\77\1\64"+
"\1\62\1\75\1\122\1\61\1\72\1\63\1\32\1\101\1\111\1\106"+
"\1\74\1\127\1\60\1\55\1\44\1\54\uff81\0";
/**
* Translates characters to character classes
*/
private static final char [] ZZ_CMAP = zzUnpackCMap(ZZ_CMAP_PACKED);
/**
* Translates DFA states to action switch labels.
*/
private static final int [] ZZ_ACTION = zzUnpackAction();
private static final String ZZ_ACTION_PACKED_0 =
"\6\0\2\1\1\0\2\1\1\0\2\1\7\0\1\2"+
"\5\0\2\2\1\3\1\4\1\5\1\6\1\1\1\7"+
"\5\1\1\10\2\1\1\11\1\12\2\13\1\14\1\15"+
"\1\16\1\17\1\20\1\21\1\22\1\23\2\21\2\23"+
"\3\21\2\23\5\21\1\23\3\21\1\23\1\1\1\24"+
"\1\1\1\25\1\15\1\26\1\27\1\30\1\31\1\32"+
"\1\33\1\34\1\35\1\36\2\17\1\2\1\37\1\17"+
"\2\2\1\17\2\40\1\17\1\2\1\35\2\17\1\2"+
"\1\41\1\35\17\2\1\42\2\2\1\43\1\1\1\44"+
"\1\45\1\46\1\1\1\47\1\50\1\51\1\1\1\52"+
"\6\1\1\53\4\1\1\54\1\55\1\54\1\56\1\54"+
"\1\57\1\54\1\60\1\61\1\54\1\62\1\63\1\64"+
"\2\63\1\65\1\63\1\66\1\67\1\70\1\71\1\70"+
"\1\72\2\2\1\40\1\2\2\70\1\73\1\74\1\75"+
"\1\76\1\77\1\100\1\101\1\1\1\102\2\1\1\103"+
"\1\104\1\105\1\106\1\1\1\107\1\1\1\110\1\4"+
"\2\111\1\112\1\113\1\6\5\0\1\114\31\21\1\23"+
"\1\21\1\23\2\21\1\23\44\21\1\115\3\0\1\116"+
"\1\0\1\117\1\17\1\35\1\2\1\17\1\120\1\40"+
"\1\120\2\121\1\120\1\122\1\120\1\2\1\65\1\2"+
"\1\65\17\2\1\65\34\2\1\123\1\124\1\125\1\0"+
"\1\126\12\0\1\127\1\130\15\0\1\131\1\40\5\0"+
"\1\40\1\0\1\77\1\132\1\133\1\134\1\135\2\111"+
"\1\0\1\136\4\0\13\21\1\23\64\21\1\0\1\137"+
"\1\0\1\35\1\2\1\121\1\0\2\122\1\2\1\57"+
"\23\2\1\140\40\2\41\0\2\111\1\141\2\0\1\142"+
"\22\21\1\23\5\21\1\23\12\21\1\0\1\143\1\35"+
"\10\2\1\144\6\2\1\57\16\2\1\145\1\2\1\146"+
"\4\2\1\0\1\1\3\0\1\147\3\0\1\150\6\0"+
"\1\65\15\0\2\111\2\0\11\21\1\23\12\21\1\0"+
"\1\35\7\2\1\65\10\2\1\65\6\2\24\0\1\111"+
"\1\151\12\21\1\0\1\35\5\2\1\152\12\2\14\0"+
"\1\153\3\21\1\0\10\2\10\0\1\21\1\0\3\2"+
"\2\0\1\154\4\0\1\21\1\155\1\2\1\156\1\157"+
"\6\0\1\160";
private static int [] zzUnpackAction() {
int [] result = new int[819];
int offset = 0;
offset = zzUnpackAction(ZZ_ACTION_PACKED_0, offset, result);
return result;
}
private static int zzUnpackAction(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/**
* Translates a state to a row index in the transition table
*/
private static final int [] ZZ_ROWMAP = zzUnpackRowMap();
private static final String ZZ_ROWMAP_PACKED_0 =
"\0\0\0\131\0\262\0\u010b\0\u0164\0\u01bd\0\u0216\0\u026f"+
"\0\u02c8\0\u0321\0\u037a\0\u03d3\0\u042c\0\u0485\0\u04de\0\u0537"+
"\0\u0590\0\u05e9\0\u0642\0\u069b\0\u06f4\0\u074d\0\u07a6\0\u07ff"+
"\0\u0858\0\u08b1\0\u090a\0\u0963\0\u09bc\0\u0a15\0\u0a6e\0\u0ac7"+
"\0\u0b20\0\u0b79\0\u0a15\0\u0bd2\0\u0c2b\0\u0c84\0\u0cdd\0\u0d36"+
"\0\u0a15\0\u0d8f\0\u0de8\0\u0a15\0\u0a15\0\u0e41\0\u0e9a\0\u0a15"+
"\0\u0ef3\0\u0a15\0\u0a15\0\u0a15\0\u0f4c\0\u0a15\0\u0fa5\0\u0ffe"+
"\0\u1057\0\u10b0\0\u1109\0\u1162\0\u11bb\0\u1214\0\u126d\0\u12c6"+
"\0\u131f\0\u1378\0\u13d1\0\u142a\0\u1483\0\u14dc\0\u1535\0\u158e"+
"\0\u15e7\0\u0f4c\0\u1640\0\u0a15\0\u1699\0\u0a15\0\u16f2\0\u0a15"+
"\0\u0a15\0\u0a15\0\u0a15\0\u0a15\0\u0a15\0\u0a15\0\u174b\0\u0a15"+
"\0\u17a4\0\u17fd\0\u0a15\0\u0a15\0\u1856\0\u18af\0\u1908\0\u1961"+
"\0\u19ba\0\u1a13\0\u1a6c\0\u1ac5\0\u0a15\0\u1b1e\0\u1b77\0\u1bd0"+
"\0\u0a15\0\u1c29\0\u1c82\0\u1cdb\0\u1d34\0\u1d8d\0\u1de6\0\u1e3f"+
"\0\u1e98\0\u1ef1\0\u1f4a\0\u1fa3\0\u1ffc\0\u2055\0\u20ae\0\u2107"+
"\0\u2160\0\u0a15\0\u21b9\0\u2212\0\u0a15\0\u226b\0\u0a15\0\u0a15"+
"\0\u22c4\0\u231d\0\u0a15\0\u22c4\0\u0a15\0\u2376\0\u0a15\0\u23cf"+
"\0\u2428\0\u2481\0\u24da\0\u2533\0\u258c\0\u0a15\0\u25e5\0\u263e"+
"\0\u2697\0\u26f0\0\u0a15\0\u0a15\0\u2749\0\u0a15\0\u27a2\0\u27fb"+
"\0\u2854\0\u28ad\0\u0a15\0\u2906\0\u0a15\0\u0a15\0\u0a15\0\u2749"+
"\0\u27a2\0\u295f\0\u29b8\0\u0a15\0\u0a15\0\u0a15\0\u0a15\0\u2749"+
"\0\u0a15\0\u2a11\0\u2a6a\0\u2ac3\0\u2b1c\0\u2b75\0\u2bce\0\u0a15"+
"\0\u0a15\0\u0a15\0\u0a15\0\u2c27\0\u0a15\0\u0a15\0\u2c80\0\u0a15"+
"\0\u2cd9\0\u2d32\0\u0a15\0\u22c4\0\u2d8b\0\u0a15\0\u2de4\0\u0a15"+
"\0\u2e3d\0\u0a15\0\u2e96\0\u2eef\0\u2f48\0\u2fa1\0\u0a15\0\u0a15"+
"\0\u2ffa\0\u3053\0\u30ac\0\u3105\0\u315e\0\u0a15\0\u31b7\0\u3210"+
"\0\u3269\0\u32c2\0\u331b\0\u3374\0\u33cd\0\u3426\0\u347f\0\u34d8"+
"\0\u3531\0\u358a\0\u35e3\0\u363c\0\u3695\0\u36ee\0\u3747\0\u37a0"+
"\0\u37f9\0\u3852\0\u38ab\0\u3904\0\u395d\0\u39b6\0\u3a0f\0\u3a68"+
"\0\u3ac1\0\u3b1a\0\u3b73\0\u3bcc\0\u3c25\0\u3c7e\0\u3cd7\0\u3d30"+
"\0\u3d89\0\u3de2\0\u3e3b\0\u3e94\0\u3eed\0\u3f46\0\u3f9f\0\u3ff8"+
"\0\u4051\0\u40aa\0\u4103\0\u415c\0\u41b5\0\u420e\0\u4267\0\u42c0"+
"\0\u4319\0\u4372\0\u43cb\0\u4424\0\u447d\0\u44d6\0\u452f\0\u4588"+
"\0\u45e1\0\u463a\0\u4693\0\u46ec\0\u4745\0\u479e\0\u47f7\0\u4850"+
"\0\u48a9\0\u0a15\0\u1b1e\0\u4902\0\u495b\0\u0a15\0\u49b4\0\u4a0d"+
"\0\u495b\0\u4a66\0\u4abf\0\u4b18\0\u4b71\0\u4b71\0\u4bca\0\u4b71"+
"\0\u4c23\0\u4c7c\0\u4cd5\0\u4d2e\0\u4d87\0\u4de0\0\u4e39\0\u18af"+
"\0\u4e92\0\u4eeb\0\u4f44\0\u4f9d\0\u4ff6\0\u504f\0\u50a8\0\u5101"+
"\0\u515a\0\u51b3\0\u520c\0\u5265\0\u52be\0\u5317\0\u5370\0\u53c9"+
"\0\u5422\0\u547b\0\u54d4\0\u552d\0\u5586\0\u55df\0\u5638\0\u5691"+
"\0\u56ea\0\u5743\0\u579c\0\u57f5\0\u584e\0\u58a7\0\u5900\0\u5959"+
"\0\u59b2\0\u5a0b\0\u5a64\0\u5abd\0\u5b16\0\u5b6f\0\u5bc8\0\u5c21"+
"\0\u5c7a\0\u5cd3\0\u5d2c\0\u5d85\0\u0a15\0\u5dde\0\u5e37\0\u5e90"+
"\0\u0a15\0\u5ee9\0\u5f42\0\u5f9b\0\u5ff4\0\u604d\0\u60a6\0\u60ff"+
"\0\u6158\0\u61b1\0\u620a\0\u0a15\0\u6263\0\u62bc\0\u6315\0\u636e"+
"\0\u63c7\0\u6420\0\u6479\0\u64d2\0\u652b\0\u6584\0\u65dd\0\u6636"+
"\0\u668f\0\u66e8\0\u6741\0\u0a15\0\u679a\0\u67f3\0\u684c\0\u68a5"+
"\0\u68fe\0\u2b75\0\u6957\0\u0a15\0\u0a15\0\u0a15\0\u0a15\0\u2e96"+
"\0\u69b0\0\u6a09\0\u6a62\0\u0a15\0\u6abb\0\u6b14\0\u6b6d\0\u6bc6"+
"\0\u6c1f\0\u6c78\0\u6cd1\0\u6d2a\0\u6d83\0\u6ddc\0\u6e35\0\u6e8e"+
"\0\u6ee7\0\u6f40\0\u6f99\0\u6ff2\0\u704b\0\u70a4\0\u70fd\0\u7156"+
"\0\u71af\0\u7208\0\u7261\0\u72ba\0\u7313\0\u736c\0\u73c5\0\u741e"+
"\0\u7477\0\u74d0\0\u7529\0\u7582\0\u75db\0\u7634\0\u3b1a\0\u768d"+
"\0\u76e6\0\u773f\0\u7798\0\u77f1\0\u784a\0\u78a3\0\u78fc\0\u7955"+
"\0\u79ae\0\u7a07\0\u7a60\0\u7ab9\0\u7b12\0\u7b6b\0\u12c6\0\u7bc4"+
"\0\u7c1d\0\u7c76\0\u7ccf\0\u7d28\0\u7d81\0\u7dda\0\u7e33\0\u7e8c"+
"\0\u7ee5\0\u7f3e\0\u7f97\0\u7ff0\0\u8049\0\u80a2\0\u80fb\0\u8154"+
"\0\u81ad\0\u8206\0\u825f\0\u82b8\0\u8311\0\u836a\0\u83c3\0\u4b71"+
"\0\u841c\0\u8475\0\u84ce\0\u8527\0\u8580\0\u85d9\0\u8632\0\u868b"+
"\0\u86e4\0\u873d\0\u8796\0\u87ef\0\u8848\0\u88a1\0\u88fa\0\u8953"+
"\0\u89ac\0\u8a05\0\u8a5e\0\u8ab7\0\u8b10\0\u8b69\0\u18af\0\u8bc2"+
"\0\u8c1b\0\u8c74\0\u8ccd\0\u8d26\0\u8d7f\0\u8dd8\0\u8e31\0\u8e8a"+
"\0\u8ee3\0\u8f3c\0\u8f95\0\u8fee\0\u9047\0\u90a0\0\u90f9\0\u9152"+
"\0\u91ab\0\u9204\0\u925d\0\u92b6\0\u930f\0\u9368\0\u93c1\0\u941a"+
"\0\u9473\0\u94cc\0\u9525\0\u957e\0\u95d7\0\u9630\0\u9689\0\u96e2"+
"\0\u973b\0\u9794\0\u97ed\0\u9846\0\u989f\0\u98f8\0\u9951\0\u99aa"+
"\0\u9a03\0\u9a5c\0\u9ab5\0\u9b0e\0\u9b67\0\u9bc0\0\u9c19\0\u9c72"+
"\0\u9ccb\0\u9d24\0\u9d7d\0\u9dd6\0\u9e2f\0\u9e88\0\u9ee1\0\u9f3a"+
"\0\u9f93\0\u9fec\0\ua045\0\ua09e\0\ua0f7\0\ua150\0\ua1a9\0\ua202"+
"\0\ua25b\0\ua2b4\0\u0a15\0\ua30d\0\ua366\0\ua3bf\0\ua418\0\ua471"+
"\0\ua4ca\0\ua523\0\ua57c\0\ua5d5\0\ua62e\0\ua687\0\ua6e0\0\ua739"+
"\0\ua792\0\ua7eb\0\ua844\0\ua89d\0\ua8f6\0\ua94f\0\ua9a8\0\uaa01"+
"\0\uaa5a\0\uaab3\0\uab0c\0\uab65\0\u3c25\0\uabbe\0\u7529\0\uac17"+
"\0\uac70\0\uacc9\0\uad22\0\uad7b\0\uadd4\0\uae2d\0\uae86\0\uaedf"+
"\0\uaf38\0\uaf91\0\u0a15\0\uafea\0\ub043\0\ub09c\0\ub0f5\0\ub14e"+
"\0\ub1a7\0\ub200\0\ub259\0\ub2b2\0\u18af\0\ub30b\0\ub364\0\ub3bd"+
"\0\ub416\0\ub46f\0\ub4c8\0\u18af\0\ub521\0\ub57a\0\ub5d3\0\ub62c"+
"\0\ub685\0\ub6de\0\ub737\0\ub790\0\ub7e9\0\ub842\0\ub89b\0\ub8f4"+
"\0\ub94d\0\ub9a6\0\u18af\0\ub9ff\0\u18af\0\uba58\0\ubab1\0\ubb0a"+
"\0\ubb63\0\u5e37\0\u0a15\0\ubbbc\0\ubc15\0\ubc6e\0\ubcc7\0\ubd20"+
"\0\ubd79\0\ubdd2\0\ube2b\0\ube84\0\ubedd\0\ubf36\0\ubf8f\0\ubfe8"+
"\0\uc041\0\u0a15\0\uc09a\0\uc0f3\0\uc14c\0\uc1a5\0\uc1fe\0\uc257"+
"\0\uc2b0\0\uc309\0\uc362\0\uc3bb\0\uc414\0\uc46d\0\uc4c6\0\uc51f"+
"\0\uc578\0\uc5d1\0\ua3bf\0\uc62a\0\uc683\0\uc6dc\0\uc735\0\uc78e"+
"\0\uc7e7\0\uc840\0\uc899\0\uc8f2\0\uc94b\0\uc94b\0\uc9a4\0\uc9fd"+
"\0\uca56\0\ucaaf\0\ucb08\0\ucb61\0\ucbba\0\ucc13\0\ucc6c\0\uccc5"+
"\0\ucd1e\0\ucd77\0\ucdd0\0\uce29\0\uce82\0\ucedb\0\ucf34\0\ucf8d"+
"\0\u87ef\0\ucfe6\0\ud03f\0\ud098\0\ud0f1\0\ud14a\0\ud1a3\0\ud1fc"+
"\0\ud255\0\ud2ae\0\ud307\0\ud360\0\ud3b9\0\ud412\0\ud46b\0\ud4c4"+
"\0\ud51d\0\ud576\0\ubcc7\0\ud5cf\0\ud628\0\ube2b\0\ud681\0\ud6da"+
"\0\ud733\0\ud78c\0\ud7e5\0\ud83e\0\ud897\0\ud8f0\0\ud949\0\ud9a2"+
"\0\ud9fb\0\uda54\0\udaad\0\udb06\0\udb5f\0\u2f48\0\udbb8\0\udc11"+
"\0\udc6a\0\udcc3\0\udd1c\0\udd75\0\uddce\0\ude27\0\ude80\0\uded9"+
"\0\udf32\0\udf8b\0\udfe4\0\ue03d\0\ue096\0\ue0ef\0\ue148\0\u18af"+
"\0\ue1a1\0\ue1fa\0\ue253\0\ue2ac\0\ue305\0\ue35e\0\ue3b7\0\ue410"+
"\0\ue469\0\ue4c2\0\ue51b\0\ue574\0\ue5cd\0\ue626\0\ue67f\0\ue6d8"+
"\0\ue731\0\ue78a\0\ue7e3\0\ue83c\0\ue895\0\ue8ee\0\u2f48\0\ue947"+
"\0\ue9a0\0\ue9f9\0\uea52\0\ueaab\0\ueb04\0\ueb5d\0\uebb6\0\uec0f"+
"\0\uec68\0\uecc1\0\ued1a\0\ued73\0\uedcc\0\uee25\0\uee7e\0\ueed7"+
"\0\uef30\0\uef89\0\uefe2\0\uf03b\0\uf094\0\uf0ed\0\uf146\0\uf19f"+
"\0\uf1f8\0\uf251\0\u0a15\0\uf2aa\0\uf303\0\uf35c\0\uf3b5\0\uf40e"+
"\0\u0a15\0\uf467\0\u0a15\0\u0a15\0\uf4c0\0\uf519\0\uf572\0\uf5cb"+
"\0\uf624\0\uf67d\0\u0a15";
private static int [] zzUnpackRowMap() {
int [] result = new int[819];
int offset = 0;
offset = zzUnpackRowMap(ZZ_ROWMAP_PACKED_0, offset, result);
return result;
}
private static int zzUnpackRowMap(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int high = packed.charAt(i++) << 16;
result[j++] = high | packed.charAt(i++);
}
return j;
}
/**
* The transition table of the DFA
*/
private static final int [] ZZ_TRANS = zzUnpackTrans();
private static final String ZZ_TRANS_PACKED_0 =
"\1\34\1\35\1\36\1\37\1\40\1\41\123\34\2\42"+
"\1\43\26\42\1\44\33\42\1\45\5\42\1\46\15\42"+
"\1\47\17\42\2\50\1\51\47\50\1\52\56\50\2\53"+
"\1\54\14\53\1\55\111\53\1\56\1\57\1\0\1\56"+
"\1\40\2\56\1\60\1\61\6\56\1\62\26\56\1\63"+
"\41\56\1\64\20\56\2\65\1\0\1\65\1\66\2\65"+
"\2\66\1\67\1\70\1\71\1\72\1\73\1\74\1\66"+
"\1\65\1\75\1\76\3\65\1\77\3\65\1\100\6\65"+
"\1\101\4\65\1\66\7\65\1\72\2\65\1\71\1\102"+
"\1\74\1\103\1\104\1\70\1\75\1\105\1\106\1\67"+
"\1\101\1\65\1\73\1\76\1\107\1\110\1\111\1\77"+
"\5\65\1\66\1\65\1\106\1\102\1\103\1\107\1\105"+
"\1\100\1\65\1\110\1\112\1\111\1\104\4\65\7\113"+
"\1\114\121\113\110\115\1\114\20\115\1\56\1\57\1\0"+
"\1\56\1\40\2\56\1\116\1\117\6\56\1\120\26\56"+
"\1\63\41\56\1\121\20\56\7\113\1\122\121\113\110\115"+
"\1\122\20\115\1\56\1\57\1\0\1\56\1\40\2\56"+
"\1\123\1\117\6\56\1\124\26\56\1\63\41\56\1\125"+
"\20\56\7\113\1\126\121\113\110\115\1\126\20\115\1\127"+
"\1\40\1\130\1\131\1\40\1\132\1\133\1\134\1\135"+
"\3\136\1\137\2\136\1\140\4\136\1\141\1\142\1\136"+
"\1\141\1\136\1\143\1\144\1\145\2\127\1\136\1\146"+
"\2\136\1\147\1\150\1\151\1\133\4\146\3\63\1\152"+
"\1\153\1\154\1\151\1\155\1\136\1\156\1\157\1\136"+
"\1\160\1\161\1\162\1\163\1\164\1\165\1\136\1\166"+
"\1\167\2\136\1\170\1\171\1\127\2\151\1\136\1\151"+
"\1\172\1\173\2\136\1\174\10\136\1\141\2\136\1\175"+
"\2\176\1\177\4\176\1\200\25\176\1\201\73\176\2\202"+
"\1\203\32\202\1\204\52\202\1\205\20\202\2\206\1\207"+
"\1\210\33\206\1\211\25\206\1\212\5\206\1\213\15\206"+
"\1\214\17\206\2\215\1\216\1\217\61\215\1\220\5\215"+
"\1\221\15\215\1\222\17\215\1\223\1\40\1\224\1\225"+
"\1\40\1\223\1\151\1\226\1\227\6\230\1\63\4\230"+
"\2\223\1\230\1\223\3\230\1\223\1\231\1\223\1\63"+
"\3\230\1\63\1\230\1\223\1\133\2\63\3\223\1\232"+
"\2\63\2\230\1\233\22\230\1\234\2\151\1\230\1\151"+
"\1\235\14\230\1\223\2\230\1\223\1\236\1\40\1\237"+
"\1\240\1\40\3\236\1\241\6\242\1\236\4\242\2\236"+
"\1\242\1\236\3\242\4\236\1\243\2\242\2\236\1\244"+
"\6\236\1\245\2\236\2\242\1\236\22\242\3\236\1\242"+
"\2\236\14\242\1\236\2\242\1\236\1\246\1\40\1\247"+
"\1\250\1\40\1\246\1\251\1\226\1\252\6\253\1\246"+
"\4\253\2\254\1\253\1\254\1\253\1\255\1\253\1\246"+
"\1\256\1\253\2\246\2\253\1\246\1\133\1\244\1\133"+
"\3\246\1\257\4\246\2\253\1\246\22\253\1\246\1\260"+
"\1\261\1\253\1\246\1\235\14\253\1\254\2\253\1\246"+
"\2\176\1\262\4\176\1\263\25\176\1\264\73\176\2\202"+
"\1\265\32\202\1\264\52\202\1\266\20\202\2\267\1\270"+
"\34\267\1\271\25\267\1\212\5\267\1\213\15\267\1\214"+
"\17\267\2\272\1\273\32\272\1\274\1\275\71\272\1\276"+
"\2\277\1\300\33\277\1\301\5\277\1\302\64\277\2\34"+
"\4\0\124\34\1\35\2\0\1\40\1\0\123\34\141\0"+
"\1\303\1\304\5\305\1\0\10\305\2\0\1\305\5\0"+
"\2\305\7\0\1\306\1\307\3\0\2\305\1\0\11\305"+
"\1\304\10\305\3\0\1\305\2\0\17\305\2\0\1\40"+
"\2\0\1\40\124\0\4\41\1\0\1\41\1\310\122\41"+
"\2\42\1\0\26\42\1\0\33\42\1\0\5\42\1\0"+
"\15\42\1\0\17\42\31\0\1\311\162\0\1\312\123\0"+
"\1\313\4\0\1\314\156\0\1\315\17\0\2\50\1\0"+
"\47\50\1\0\56\50\17\0\1\316\111\0\2\53\1\0"+
"\14\53\1\0\111\53\2\56\1\0\1\56\1\0\2\56"+
"\2\0\6\56\1\0\26\56\1\0\41\56\1\0\21\56"+
"\1\57\1\0\1\56\1\40\2\56\2\0\6\56\1\0"+
"\26\56\1\0\41\56\1\0\20\56\17\0\1\62\111\0"+
"\2\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\41\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\1\65\1\317\2\65\1\320\1\321\1\0"+
"\2\65\1\322\7\65\1\323\13\65\1\0\13\65\1\324"+
"\1\321\2\65\1\317\2\65\1\325\3\65\1\320\1\322"+
"\1\326\10\65\1\0\1\65\1\325\1\324\1\65\1\326"+
"\1\65\1\323\13\65\1\0\1\65\1\0\2\65\2\0"+
"\3\65\1\327\2\65\1\0\2\65\1\330\23\65\1\0"+
"\7\65\1\327\3\65\1\331\6\65\1\332\4\65\1\330"+
"\11\65\1\0\1\65\1\332\1\331\17\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\12\65\1\333\13\65"+
"\1\0\41\65\1\0\6\65\1\333\13\65\1\0\1\65"+
"\1\0\2\65\2\0\1\334\5\65\1\0\1\65\1\335"+
"\17\65\1\336\4\65\1\0\15\65\1\337\2\65\1\335"+
"\2\65\1\334\1\336\3\65\1\340\10\65\1\0\3\65"+
"\1\337\1\340\15\65\1\0\1\65\1\0\2\65\2\0"+
"\2\65\1\341\3\65\1\0\1\65\1\342\24\65\1\0"+
"\12\65\1\341\5\65\1\342\1\65\1\343\16\65\1\0"+
"\1\65\1\343\20\65\1\0\1\65\1\0\2\65\2\0"+
"\2\65\1\112\1\344\1\65\1\112\1\0\2\65\1\345"+
"\3\65\1\346\12\65\1\347\4\65\1\0\7\65\1\344"+
"\2\65\1\112\1\65\1\112\1\65\1\350\2\65\1\112"+
"\1\351\1\65\1\347\2\65\1\345\3\65\1\346\5\65"+
"\1\0\1\65\1\351\3\65\1\112\5\65\1\350\6\65"+
"\1\0\1\65\1\0\2\65\2\0\3\65\1\352\2\65"+
"\1\0\2\65\1\353\23\65\1\0\7\65\1\352\12\65"+
"\1\354\4\65\1\353\11\65\1\0\1\65\1\354\20\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\30\65\1\355\1\65\1\356\6\65\1\0\4\65"+
"\1\355\5\65\1\356\7\65\1\0\1\65\1\0\2\65"+
"\2\0\2\65\1\112\1\340\2\65\1\0\1\65\1\357"+
"\10\65\1\360\13\65\1\0\7\65\1\340\1\361\1\65"+
"\1\112\1\362\4\65\1\357\1\363\1\364\16\65\1\0"+
"\1\65\1\364\1\362\2\65\1\363\1\360\1\361\12\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\1\65"+
"\1\112\24\65\1\0\20\65\1\112\20\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\2\65\1\365\1\366"+
"\2\65\1\0\26\65\1\0\7\65\1\366\2\65\1\365"+
"\1\367\25\65\1\0\2\65\1\367\17\65\1\0\1\65"+
"\1\0\2\65\2\0\4\65\1\370\1\65\1\0\1\65"+
"\1\112\4\65\1\371\3\65\1\372\13\65\1\0\20\65"+
"\1\112\5\65\1\370\4\65\1\371\5\65\1\0\6\65"+
"\1\372\13\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\2\65\1\373\23\65\1\0\13\65\1\374\6\65"+
"\1\375\4\65\1\373\11\65\1\0\1\65\1\375\1\374"+
"\17\65\1\0\1\65\1\0\2\65\2\0\2\65\1\112"+
"\2\65\1\376\1\0\2\65\1\377\23\65\1\0\12\65"+
"\1\112\1\65\1\376\12\65\1\377\11\65\1\0\14\65"+
"\1\112\5\65\1\0\1\65\1\0\2\65\2\0\3\65"+
"\1\u0100\1\65\1\112\1\0\1\65\1\112\1\u0101\16\65"+
"\1\u0102\4\65\1\0\7\65\1\u0100\4\65\1\112\3\65"+
"\2\112\1\u0103\1\65\1\u0102\2\65\1\u0101\11\65\1\0"+
"\1\65\1\u0103\3\65\1\112\14\65\1\0\1\65\1\0"+
"\2\65\2\0\1\u0104\1\u0105\1\u0106\1\65\1\u0107\1\65"+
"\1\0\6\65\1\u0108\3\65\1\u0109\13\65\1\0\12\65"+
"\1\u0106\4\65\1\u0105\1\65\1\u010a\1\65\1\u0104\2\65"+
"\1\u0107\4\65\1\u0108\5\65\1\0\5\65\1\u010a\1\u0109"+
"\13\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\2\65\1\u010b\7\65\1\u010c\13\65\1\0\22\65\1\u010d"+
"\4\65\1\u010b\11\65\1\0\1\65\1\u010d\4\65\1\u010c"+
"\13\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\2\65\1\u010e\3\65\1\u010f\17\65\1\0\27\65\1\u010e"+
"\3\65\1\u010f\5\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\3\65\1\u0110\2\65\1\0\26\65\1\0"+
"\7\65\1\u0110\12\65\1\u0111\16\65\1\0\1\65\1\u0111"+
"\16\65\7\113\1\0\121\113\110\115\1\0\20\115\17\0"+
"\1\u0112\111\0\1\127\10\0\6\127\1\0\11\127\1\0"+
"\1\127\1\0\3\127\1\0\2\127\14\0\2\127\1\0"+
"\23\127\2\0\1\127\2\0\17\127\4\0\1\u0113\4\0"+
"\1\u0114\35\0\1\63\67\0\1\63\40\0\1\63\62\0"+
"\10\u0115\1\u0116\24\u0115\1\u0117\1\u0115\1\u0118\6\u0115\1\u0119"+
"\62\u0115\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\22\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\3\136\1\u011b\16\136\1\127\2\0\1\136\2\0\17\136"+
"\20\0\1\u011c\26\0\1\63\62\0\1\u011d\10\0\6\u011d"+
"\1\0\1\u011d\1\u011e\1\u011f\1\u011d\2\141\1\u011d\1\141"+
"\1\u011d\1\0\1\u011d\1\0\3\u011d\1\0\1\u011d\1\u0120"+
"\1\0\1\u0121\12\0\2\u011d\1\0\6\u011d\1\u011e\1\u0120"+
"\2\u011d\1\u0120\2\u011d\1\u011f\5\u011d\2\0\1\u011d\2\0"+
"\5\u011d\1\u0120\6\u011d\1\141\2\u011d\1\0\1\u011d\10\0"+
"\6\u011d\1\0\1\u011d\1\u011e\1\u011f\1\u011d\1\u0122\1\u0123"+
"\1\u011d\1\u0123\1\u011d\1\0\1\u011d\1\0\3\u011d\1\0"+
"\1\u0124\1\u0120\1\0\1\u0121\12\0\2\u011d\1\0\6\u011d"+
"\1\u011e\1\u0120\2\u011d\1\u0120\2\u011d\1\u011f\5\u011d\2\0"+
"\1\u0124\2\0\5\u011d\1\u0120\6\u011d\1\u0123\2\u011d\32\0"+
"\1\63\14\0\1\63\62\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\3\136\1\u0125\16\136\1\127"+
"\2\0\1\136\2\0\17\136\47\0\1\63\124\0\1\63"+
"\3\0\1\63\106\0\2\u0121\1\0\1\u0121\75\0\1\u0121"+
"\51\0\1\63\6\0\1\63\53\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\3\136\1\u0126\5\136"+
"\1\u0127\1\u0128\3\136\1\u0129\3\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\1\136\1\u012a\20\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\15\136\1\u012b\4\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\1\u012c\3\136\1\u012d"+
"\6\136\1\u012e\6\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\u012f"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\10\136\1\u0130\4\136\1\u0131\4\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\1\136\1\u0132\2\136\1\u0133"+
"\1\136\1\u0134\1\136\1\u0135\11\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\1\136\1\u0136\13\136\1\u0137\4\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\1\136\1\u0138"+
"\13\136\1\u0139\4\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\21\136\1\u013a\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\u013b"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\2\136\1\u013c\1\136\1\u013d\6\136\1\u013e\6\136"+
"\1\127\2\0\1\136\2\0\1\u013f\16\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\u0140\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\1\u0141\1\136\1\0"+
"\1\136\1\u0142\4\136\1\u0143\1\136\1\u0144\11\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\u0145\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\1\u0146\7\136\1\u0147"+
"\11\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\3\136"+
"\1\u0148\2\136\1\u0149\1\136\1\u014a\1\u014b\6\136\1\u014c"+
"\1\136\1\127\2\0\1\u014d\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\1\136"+
"\1\u014e\6\136\1\u0142\11\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\1\u014f\1\u0150\11\136\1\u0151\6\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\1\u0152\1\136\1\0\4\136\1\u0153"+
"\15\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\10\136"+
"\1\u0154\11\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\2\176\1\0\4\176\1\0\25\176\1\0\73\176\2\u0155"+
"\1\0\27\u0155\1\u0156\53\u0155\1\u0157\22\u0155\2\202\1\0"+
"\32\202\1\0\52\202\1\0\20\202\2\206\2\0\33\206"+
"\1\0\25\206\1\0\5\206\1\0\15\206\1\0\17\206"+
"\10\0\1\u0158\130\0\1\u0159\203\0\1\u015a\123\0\1\u015b"+
"\4\0\1\u015c\156\0\1\u015d\17\0\2\215\2\0\61\215"+
"\1\0\5\215\1\0\15\215\1\0\17\215\10\0\1\u015e"+
"\203\0\1\u015f\123\0\1\u0160\4\0\1\u0161\156\0\1\u0162"+
"\27\0\1\u0163\157\0\1\u0164\102\0\6\230\1\0\13\230"+
"\5\0\2\230\1\0\1\230\12\0\2\230\1\0\22\230"+
"\3\0\1\230\2\0\17\230\12\0\6\u0165\1\0\4\u0165"+
"\2\0\1\u0165\1\0\3\u0165\4\0\3\u0165\1\0\1\u0165"+
"\12\0\2\u0165\1\0\22\u0165\3\0\1\u0165\2\0\14\u0165"+
"\1\0\2\u0165\54\0\1\u0166\5\0\1\u0167\1\u0168\1\u0169"+
"\1\u016a\1\u016b\1\u016c\1\u016d\1\u016e\1\u016f\1\0\1\u0170"+
"\2\0\1\u0171\2\0\1\u0172\40\0\6\u0173\1\0\4\u0173"+
"\2\0\1\u0173\1\0\3\u0173\4\0\3\u0173\1\0\1\u0173"+
"\12\0\2\u0173\1\0\22\u0173\3\0\1\u0173\2\0\14\u0173"+
"\1\0\2\u0173\12\0\6\242\1\0\13\242\5\0\2\242"+
"\14\0\2\242\1\0\22\242\3\0\1\242\2\0\17\242"+
"\12\0\6\242\1\0\4\242\2\0\1\242\1\0\3\242"+
"\5\0\2\242\14\0\2\242\1\0\22\242\3\0\1\242"+
"\2\0\14\242\1\0\2\242\11\0\7\253\1\0\4\253"+
"\2\0\1\253\1\0\3\253\2\0\1\253\1\0\1\u0164"+
"\2\253\14\0\2\253\1\0\22\253\2\0\1\261\1\253"+
"\2\0\14\253\1\0\2\253\11\0\7\253\1\0\4\253"+
"\2\0\1\253\1\0\3\253\2\0\1\253\2\0\2\253"+
"\14\0\2\253\1\0\22\253\2\0\1\261\1\253\2\0"+
"\14\253\1\0\2\253\25\0\2\254\1\0\1\254\13\0"+
"\1\254\4\0\1\u0174\5\0\1\u0175\7\0\1\u0176\3\0"+
"\1\u0174\2\0\1\u0177\1\u0178\1\u0179\25\0\1\254\13\0"+
"\7\253\1\0\4\253\2\254\1\253\1\254\3\253\2\0"+
"\1\253\2\0\2\253\14\0\2\253\1\0\22\253\2\0"+
"\1\261\1\253\2\0\14\253\1\254\2\253\13\0\1\u017a"+
"\7\0\1\u017a\1\0\4\u017a\11\0\1\u017a\24\0\1\u017a"+
"\1\0\2\u017a\1\0\1\u017a\2\0\1\u017a\3\0\1\u017a"+
"\7\0\1\u017a\3\0\1\u017a\6\0\1\u017a\61\0\1\u017b"+
"\52\0\2\u017c\1\0\126\u017c\2\267\1\0\34\267\1\0"+
"\25\267\1\0\5\267\1\0\15\267\1\0\17\267\10\0"+
"\1\u017d\120\0\2\272\1\0\32\272\2\0\71\272\61\0"+
"\1\u017e\50\0\2\277\1\0\33\277\1\0\5\277\1\0"+
"\64\277\60\0\1\u017f\61\0\6\u0180\1\0\10\u0180\2\0"+
"\1\u0180\5\0\2\u0180\14\0\2\u0180\1\0\22\u0180\3\0"+
"\1\u0180\2\0\17\u0180\12\0\1\305\1\u0181\3\305\1\u0182"+
"\1\0\10\305\2\0\1\305\5\0\2\305\14\0\2\305"+
"\1\0\2\305\1\u0182\2\305\1\u0181\14\305\3\0\1\305"+
"\2\0\17\305\12\0\6\305\1\0\10\305\2\0\1\305"+
"\5\0\2\305\14\0\2\305\1\0\22\305\3\0\1\305"+
"\2\0\17\305\32\0\1\u0183\116\0\1\u0184\174\0\1\u0185"+
"\134\0\1\u0186\136\0\1\u0187\144\0\1\u0188\17\0\2\65"+
"\1\0\1\65\1\0\2\65\2\0\2\65\1\u0189\3\65"+
"\1\0\26\65\1\0\12\65\1\u0189\26\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\22\65\1\u018a\16\65\1\0\1\65\1\u018a\20\65"+
"\1\0\1\65\1\0\2\65\2\0\2\65\1\u018b\3\65"+
"\1\0\1\333\25\65\1\0\12\65\1\u018b\12\65\1\333"+
"\13\65\1\0\22\65\1\0\1\65\1\0\2\65\2\0"+
"\1\65\1\u018c\1\u018d\3\65\1\0\1\65\1\u018e\24\65"+
"\1\0\12\65\1\u018d\4\65\1\u018c\1\u018e\20\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\4\65\1\112"+
"\1\65\1\0\6\65\1\112\17\65\1\0\26\65\1\112"+
"\4\65\1\112\5\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\12\65\1\u018f\13\65\1\0"+
"\41\65\1\0\6\65\1\u018f\13\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\30\65\1\u0190"+
"\10\65\1\0\4\65\1\u0190\15\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\22\65\1\u0191"+
"\16\65\1\0\1\65\1\u0191\20\65\1\0\1\65\1\0"+
"\2\65\2\0\5\65\1\u0192\1\0\26\65\1\0\14\65"+
"\1\u0192\24\65\1\0\22\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\26\65\1\0\15\65\1\u0193\23\65"+
"\1\0\3\65\1\u0193\16\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\1\65\1\u0194\24\65\1\0\20\65"+
"\1\u0194\1\u0192\6\65\1\u0195\10\65\1\0\4\65\1\u0195"+
"\1\u0192\14\65\1\0\1\65\1\0\2\65\2\0\4\65"+
"\1\u018c\1\65\1\0\26\65\1\0\15\65\1\u0196\10\65"+
"\1\u018c\12\65\1\0\3\65\1\u0196\16\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\1\65\1\u0192\24\65"+
"\1\0\20\65\1\u0192\20\65\1\0\22\65\1\0\1\65"+
"\1\0\2\65\2\0\3\65\1\u0197\2\65\1\0\26\65"+
"\1\0\7\65\1\u0197\31\65\1\0\22\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\22\65"+
"\1\u0198\16\65\1\0\1\65\1\u0198\20\65\1\0\1\65"+
"\1\0\2\65\2\0\2\65\1\u0199\3\65\1\0\26\65"+
"\1\0\12\65\1\u0199\26\65\1\0\22\65\1\0\1\65"+
"\1\0\2\65\2\0\1\112\3\65\1\u019a\1\65\1\0"+
"\26\65\1\0\23\65\1\112\2\65\1\u019a\12\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\10\65\1\112\30\65\1\0\7\65\1\112"+
"\12\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\2\65\1\112\23\65\1\0\13\65\1\u019b\13\65\1\112"+
"\11\65\1\0\2\65\1\u019b\17\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\22\65\1\u019c"+
"\16\65\1\0\1\65\1\u019c\20\65\1\0\1\65\1\0"+
"\2\65\2\0\2\65\1\u019d\3\65\1\0\26\65\1\0"+
"\12\65\1\u019d\26\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\5\65\1\333\1\0\26\65\1\0\14\65"+
"\1\333\13\65\1\u0192\10\65\1\0\4\65\1\u0192\15\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\20\65"+
"\1\u019e\5\65\1\0\37\65\1\u019e\1\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\13\65\1\362\25\65\1\0\2\65\1\362\17\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\13\65\1\u019f\25\65\1\0\2\65\1\u019f\17\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\2\65"+
"\1\u01a0\23\65\1\0\27\65\1\u01a0\11\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\6\65"+
"\1\333\17\65\1\0\33\65\1\333\5\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\15\65\1\u01a1\23\65\1\0\3\65\1\u01a1\16\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\10\65\1\u01a2\30\65\1\0\7\65\1\u01a2\12\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\1\u01a3"+
"\5\65\1\u01a4\17\65\1\0\25\65\1\u01a3\5\65\1\u01a4"+
"\5\65\1\0\22\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\6\65\1\u01a5\17\65\1\0\33\65\1\u01a5"+
"\5\65\1\0\22\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\2\65\1\u01a6\23\65\1\0\27\65\1\u01a6"+
"\11\65\1\0\22\65\1\0\1\65\1\0\2\65\2\0"+
"\3\65\1\u01a7\2\65\1\0\26\65\1\0\7\65\1\u01a7"+
"\3\65\1\u01a8\25\65\1\0\2\65\1\u01a8\17\65\1\0"+
"\1\65\1\0\2\65\2\0\5\65\1\u01a9\1\0\26\65"+
"\1\0\14\65\1\u01a9\24\65\1\0\22\65\1\0\1\65"+
"\1\0\2\65\2\0\1\u01aa\5\65\1\0\26\65\1\0"+
"\23\65\1\u01aa\15\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\21\65\1\u01ab"+
"\17\65\1\0\5\65\1\u01ab\14\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\13\65\1\112"+
"\25\65\1\0\2\65\1\112\17\65\1\0\1\65\1\0"+
"\2\65\2\0\1\u01ac\5\65\1\0\26\65\1\0\23\65"+
"\1\u01ac\15\65\1\0\22\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\26\65\1\0\22\65\1\u01ad\16\65"+
"\1\0\1\65\1\u01ad\20\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\2\65\1\u01ae\23\65\1\0\10\65"+
"\1\u01af\16\65\1\u01ae\11\65\1\0\7\65\1\u01af\12\65"+
"\1\0\1\65\1\0\2\65\2\0\2\65\1\u01b0\3\65"+
"\1\0\26\65\1\0\12\65\1\u01b0\1\u0193\1\65\1\u01b1"+
"\23\65\1\0\2\65\1\u0193\1\u01b1\16\65\1\0\1\65"+
"\1\0\2\65\2\0\5\65\1\u01b2\1\0\26\65\1\0"+
"\14\65\1\u01b2\24\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\41\65\1\0"+
"\15\65\1\u018e\4\65\1\0\1\65\1\0\2\65\2\0"+
"\5\65\1\u01b3\1\0\26\65\1\0\14\65\1\u01b3\24\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\1\u01b1"+
"\5\65\1\0\26\65\1\0\23\65\1\u01b1\15\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\1\u01b4\5\65"+
"\1\0\1\65\1\335\1\u01b5\3\65\1\u0111\12\65\1\u01b6"+
"\4\65\1\0\20\65\1\335\2\65\1\u01b4\1\u01b6\2\65"+
"\1\u01b5\3\65\1\u0111\5\65\1\0\22\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\32\65"+
"\1\112\6\65\1\0\12\65\1\112\7\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\30\65"+
"\1\u01b7\10\65\1\0\4\65\1\u01b7\15\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\22\65"+
"\1\u01b8\16\65\1\0\1\65\1\u01b8\20\65\1\0\1\65"+
"\1\0\2\65\2\0\2\65\1\112\3\65\1\0\26\65"+
"\1\0\12\65\1\112\7\65\1\u01b9\7\65\1\112\6\65"+
"\1\0\1\65\1\u01b9\10\65\1\112\7\65\1\0\1\65"+
"\1\0\2\65\2\0\5\65\1\u01ba\1\0\1\65\1\112"+
"\24\65\1\0\14\65\1\u01ba\3\65\1\112\20\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\15\65\1\112\23\65\1\0\3\65\1\112"+
"\16\65\1\0\1\65\1\0\2\65\2\0\5\65\1\u01bb"+
"\1\0\26\65\1\0\14\65\1\u01bb\24\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\3\65\1\u01bc\2\65"+
"\1\0\26\65\1\0\7\65\1\u01bc\31\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\2\65\1\u01bd\3\65"+
"\1\0\26\65\1\0\12\65\1\u01bd\26\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\5\65\1\u01be\1\0"+
"\2\65\1\u01bf\23\65\1\0\14\65\1\u01be\12\65\1\u01bf"+
"\11\65\1\0\22\65\1\0\1\65\1\0\2\65\2\0"+
"\4\65\1\u01c0\1\65\1\0\26\65\1\0\26\65\1\u01c0"+
"\12\65\1\0\22\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\6\65\1\u0111\17\65\1\0\33\65\1\u0111"+
"\5\65\1\0\22\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\26\65\1\0\21\65\1\u01c1\17\65\1\0"+
"\5\65\1\u01c1\14\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\26\65\1\0\21\65\1\u01c2\17\65\1\0"+
"\5\65\1\u01c2\14\65\1\0\1\65\1\0\2\65\2\0"+
"\5\65\1\u01c3\1\0\26\65\1\0\14\65\1\u01c3\1\u01c4"+
"\23\65\1\0\3\65\1\u01c4\16\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\1\65\1\u01c5\24\65\1\0"+
"\20\65\1\u01c5\20\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\2\65\1\u01c6\1\65\1\112\1\65\1\0"+
"\26\65\1\0\12\65\1\u01c6\13\65\1\112\12\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\1\u01c7\25\65\1\0\25\65\1\u01c7\13\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\21\65\1\112\17\65\1\0\5\65\1\112\14\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\21\65\1\u01c8\17\65\1\0\5\65\1\u01c8\14\65"+
"\1\0\1\65\1\0\2\65\2\0\2\65\1\112\3\65"+
"\1\0\26\65\1\0\12\65\1\112\26\65\1\0\20\65"+
"\11\0\1\u01c9\60\0\1\u01c9\36\0\10\u0115\1\u01ca\24\u0115"+
"\1\u0117\75\u0115\1\0\126\u0115\37\0\1\u01cb\71\0\1\127"+
"\10\0\6\127\1\0\11\127\1\0\1\u01cc\1\0\3\127"+
"\1\0\2\127\14\0\2\127\1\0\23\127\2\0\1\127"+
"\2\0\17\127\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\12\136\1\u01cd\7\136\1\127\2\0"+
"\1\136\2\0\17\136\20\0\1\146\26\0\1\63\62\0"+
"\1\u011d\10\0\6\u011d\1\0\11\u011d\1\0\1\u011d\1\0"+
"\3\u011d\1\0\2\u011d\14\0\2\u011d\1\0\23\u011d\2\0"+
"\1\u011d\2\0\17\u011d\1\0\1\u011d\10\0\6\u011d\1\0"+
"\4\u011d\2\u01ce\1\u011d\1\u01ce\1\u011d\1\u01cf\1\u011d\1\0"+
"\3\u011d\1\0\2\u011d\1\u01cf\13\0\2\u011d\1\0\23\u011d"+
"\2\0\1\u011d\2\0\14\u011d\1\u01ce\2\u011d\1\0\1\u011d"+
"\10\0\6\u011d\1\0\2\u011d\1\u011f\1\u011d\2\u0121\1\u011d"+
"\1\u0121\1\u011d\1\0\1\u011d\1\0\3\u011d\1\0\1\u011d"+
"\1\u0120\14\0\2\u011d\1\0\7\u011d\1\u0120\2\u011d\1\u0120"+
"\2\u011d\1\u011f\5\u011d\2\0\1\u011d\2\0\5\u011d\1\u0120"+
"\6\u011d\1\u0121\2\u011d\1\0\1\u011d\10\0\6\u011d\1\0"+
"\2\u011d\1\u011f\1\u011d\2\u0122\1\u011d\1\u0122\1\u011d\1\0"+
"\1\u011d\1\0\3\u011d\1\0\1\u011d\1\u0120\1\0\1\u0121"+
"\12\0\2\u011d\1\0\7\u011d\1\u0120\2\u011d\1\u0120\2\u011d"+
"\1\u011f\5\u011d\2\0\1\u011d\2\0\5\u011d\1\u0120\6\u011d"+
"\1\u0122\2\u011d\1\0\1\u011d\10\0\6\u011d\1\0\1\u011d"+
"\1\u01d0\1\u011f\1\u011d\1\u0122\1\u0123\1\u011d\1\u0123\1\u011d"+
"\1\0\1\u011d\1\0\3\u011d\1\0\1\u011d\1\u0120\1\0"+
"\1\u0121\12\0\2\u011d\1\0\6\u011d\1\u01d0\1\u0120\2\u011d"+
"\1\u0120\2\u011d\1\u011f\5\u011d\2\0\1\u011d\2\0\5\u011d"+
"\1\u0120\6\u011d\1\u0123\2\u011d\1\0\1\u011d\10\0\1\u011d"+
"\1\u01d1\4\u011d\1\0\2\u011d\1\u01d1\1\u011d\4\u01d1\1\u011d"+
"\1\0\1\u011d\1\0\3\u011d\1\0\1\u011d\1\u01d1\14\0"+
"\2\u011d\1\0\5\u011d\1\u01d1\1\u011d\2\u01d1\1\u011d\1\u01d1"+
"\2\u011d\1\u01d1\3\u011d\1\u01d1\1\u011d\2\0\1\u011d\2\0"+
"\1\u011d\1\u01d1\3\u011d\1\u01d1\6\u011d\1\u01d1\2\u011d\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\15\136\1\u01d2\4\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\2\136\1\u01d3\6\136\1\u01d4\10\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\1\136\1\u01d5\14\0\2\136\1\0\22\136\1\127\2\0"+
"\1\136\2\0\3\136\1\u01d6\13\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\14\136\1\u01d7"+
"\5\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\2\136"+
"\1\u01d8\17\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\2\136\1\u01d9\17\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\u01da"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\10\136\1\u01db\2\136\1\u0128\6\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\1\u01dc\1\136\1\0\1\u01dd\21\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\14\136\1\u01de\5\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\6\136\1\u01df"+
"\13\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\2\136"+
"\1\u01e0\17\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\22\136\1\127\2\0\1\136\2\0\1\u0128\16\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\3\136\1\u01e1\16\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\10\136\1\u01e2\11\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\10\136\1\u01e3\11\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\2\136\1\u01e4\6\136\1\u01e5\10\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\3\136\1\u01e6"+
"\16\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\2\136"+
"\1\u01e7\17\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\u01e8\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\22\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\6\136"+
"\1\u01e9\3\136\1\u01ea\6\136\1\u01eb\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\11\136\1\u01ec\10\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\14\136\1\u01ed\5\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\10\136\1\u01ee\11\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\1\136\1\u01ef"+
"\20\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\3\136"+
"\1\u01f0\16\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\1\u01f1\1\136"+
"\1\0\22\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\3\136\1\u01f2\16\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\3\136\1\u01f3\16\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\1\u0128\21\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\1\136\1\u01f4\20\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\6\136\1\u01f5\13\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\21\136\1\u01f6\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\1\u01f7\1\136\1\0\1\136\1\u01f8\20\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\1\u01f9\4\136"+
"\1\u01fa\14\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\u01fb\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\22\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\11\136"+
"\1\u01e5\10\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\5\136\1\u01fc\14\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\5\136\1\u01fd\14\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\10\136\1\u01fe\11\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\2\136\1\u01ff\11\136\1\u0200\5\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\1\u0201\1\136\1\0\6\136"+
"\1\u0202\13\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\15\136\1\u0203\4\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\1\136\1\u0204\20\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\2\136\1\u0205\17\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\2\136\1\u0206\17\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\1\u0207\1\136\1\0\22\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\22\136\1\127\2\0\1\136"+
"\2\0\3\136\1\u0128\13\136\13\0\1\u0208\7\0\1\u0208"+
"\1\0\4\u0208\11\0\1\u0208\24\0\1\u0208\1\0\2\u0208"+
"\1\0\1\u0208\2\0\1\u0208\3\0\1\u0208\7\0\1\u0208"+
"\3\0\1\u0208\6\0\1\u0208\15\0\1\u0209\7\0\1\u0209"+
"\1\0\4\u0209\11\0\1\u0209\24\0\1\u0209\1\0\2\u0209"+
"\1\0\1\u0209\2\0\1\u0209\3\0\1\u0209\7\0\1\u0209"+
"\3\0\1\u0209\6\0\1\u0209\14\0\1\u020a\60\0\1\u020a"+
"\121\0\1\u020b\134\0\1\u020c\136\0\1\u020d\144\0\1\u020e"+
"\30\0\1\u020f\60\0\1\u020f\121\0\1\u0210\134\0\1\u0211"+
"\136\0\1\u0212\144\0\1\u0213\30\0\1\u0214\60\0\1\u0214"+
"\47\0\6\u0165\1\0\13\u0165\5\0\2\u0165\1\0\1\u0165"+
"\12\0\2\u0165\1\0\22\u0165\3\0\1\u0165\2\0\17\u0165"+
"\72\0\1\u0215\1\0\1\u0216\6\0\1\u0217\110\0\1\u0218"+
"\132\0\1\u0219\135\0\1\u021a\121\0\1\u021b\1\u021c\127\0"+
"\1\u021d\133\0\1\u021e\121\0\1\u021f\12\0\1\u0220\115\0"+
"\1\u0221\140\0\1\u0222\120\0\1\u0223\3\0\1\u0224\132\0"+
"\1\u0225\12\0\1\u0226\107\0\1\u0227\63\0\6\u0173\1\0"+
"\13\u0173\5\0\2\u0173\1\0\1\u0173\12\0\2\u0173\1\0"+
"\22\u0173\3\0\1\u0173\2\0\17\u0173\65\0\1\u0174\143\0"+
"\1\u0174\114\0\1\u0174\2\0\1\u0174\17\0\1\u0174\121\0"+
"\1\u0174\6\0\1\u0174\114\0\1\u0174\4\0\1\u0174\130\0"+
"\1\u0228\42\0\2\305\1\u0229\3\305\1\0\10\305\2\0"+
"\1\305\5\0\2\305\14\0\2\305\1\0\1\u0229\21\305"+
"\3\0\1\305\2\0\17\305\12\0\6\305\1\0\1\u022a"+
"\7\305\2\0\1\305\5\0\2\305\14\0\2\305\1\0"+
"\13\305\1\u022a\6\305\3\0\1\305\2\0\17\305\32\0"+
"\1\u022b\174\0\1\u022c\131\0\1\u0187\105\0\1\u022d\120\0"+
"\1\u022e\65\0\2\65\1\0\1\65\1\0\2\65\2\0"+
"\3\65\1\u022f\2\65\1\0\26\65\1\0\7\65\1\u022f"+
"\31\65\1\0\22\65\1\0\1\65\1\0\2\65\2\0"+
"\1\65\1\u01a3\4\65\1\0\26\65\1\0\15\65\1\112"+
"\1\65\1\u01a3\21\65\1\0\3\65\1\112\16\65\1\0"+
"\1\65\1\0\2\65\2\0\3\65\1\u0230\2\65\1\0"+
"\26\65\1\0\7\65\1\u0230\3\65\1\u0231\25\65\1\0"+
"\2\65\1\u0231\17\65\1\0\1\65\1\0\2\65\2\0"+
"\5\65\1\u0232\1\0\26\65\1\0\14\65\1\u0232\24\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\26\65\1\0\32\65\1\u01a3\6\65\1\0\12\65"+
"\1\u01a3\7\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\2\65\1\u0233\23\65\1\0\27\65\1\u0233\11\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\2\65"+
"\1\u0234\3\65\1\0\26\65\1\0\12\65\1\u0234\26\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\4\65"+
"\1\112\1\65\1\0\26\65\1\0\26\65\1\112\12\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\1\65\1\u01b7\24\65\1\0\20\65\1\u01b7\20\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\2\65\1\112\23\65\1\0\27\65\1\112\11\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\5\65"+
"\1\u01a3\1\0\26\65\1\0\14\65\1\u01a3\24\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\10\65\1\u0235\30\65\1\0\7\65\1\u0235"+
"\12\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\30\65\1\u0236\10\65\1\0\4\65\1\u0236"+
"\15\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\32\65\1\u0237\6\65\1\0\12\65\1\u0237"+
"\7\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\15\65\1\u0238\23\65\1\0\3\65\1\u0238"+
"\16\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\1\u01a3\25\65\1\0\25\65\1\u01a3\13\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\22\65\1\u0239\16\65\1\0\1\65\1\u0239\20\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\12\65"+
"\1\u01b1\13\65\1\0\41\65\1\0\6\65\1\u01b1\13\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\10\65\1\u01c2\30\65\1\0\7\65\1\u01c2\12\65"+
"\1\0\1\65\1\0\2\65\2\0\3\65\1\u023a\2\65"+
"\1\0\26\65\1\0\7\65\1\u023a\31\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\22\65\1\u01b0\16\65\1\0\1\65\1\u01b0\20\65"+
"\1\0\1\65\1\0\2\65\2\0\5\65\1\u023b\1\0"+
"\26\65\1\0\14\65\1\u023b\24\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\13\65\1\u01b1\25\65\1\0\2\65\1\u01b1\17\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\22\65\1\u010f\16\65\1\0\1\65\1\u010f\20\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\31\65\1\112\7\65\1\0\10\65\1\112\11\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\2\65\1\u023c"+
"\23\65\1\0\27\65\1\u023c\11\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\2\65\1\u0111"+
"\23\65\1\0\27\65\1\u0111\11\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\2\65\1\u01b7"+
"\23\65\1\0\27\65\1\u01b7\11\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\2\65\1\u010f"+
"\23\65\1\0\27\65\1\u010f\11\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\15\65\1\u023d\23\65\1\0\3\65\1\u023d\16\65\1\0"+
"\1\65\1\0\2\65\2\0\1\65\1\u023e\4\65\1\0"+
"\26\65\1\0\17\65\1\u023e\21\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\5\65\1\u023f\1\0\26\65"+
"\1\0\14\65\1\u023f\24\65\1\0\22\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\13\65"+
"\1\u0240\25\65\1\0\2\65\1\u0240\17\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\1\112\25\65\1\0"+
"\25\65\1\112\13\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\2\65\1\u0241\23\65\1\0"+
"\27\65\1\u0241\11\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\30\65\1\u0242"+
"\10\65\1\0\4\65\1\u0242\15\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\1\65\1\u0243\24\65\1\0"+
"\20\65\1\u0243\20\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\12\65\1\u0244\13\65\1\0"+
"\41\65\1\0\6\65\1\u0244\13\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\30\65\1\112"+
"\10\65\1\0\4\65\1\112\15\65\1\0\1\65\1\0"+
"\2\65\2\0\5\65\1\112\1\0\26\65\1\0\14\65"+
"\1\112\24\65\1\0\22\65\1\0\1\65\1\0\2\65"+
"\2\0\3\65\1\u023f\2\65\1\0\26\65\1\0\7\65"+
"\1\u023f\1\u0235\30\65\1\0\7\65\1\u0235\12\65\1\0"+
"\1\65\1\0\2\65\2\0\4\65\1\u019a\1\65\1\0"+
"\26\65\1\0\26\65\1\u019a\12\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\1\65\1\317\4\65\1\0"+
"\26\65\1\0\17\65\1\317\21\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\30\65\1\u0245\10\65\1\0\4\65\1\u0245\15\65\1\0"+
"\1\65\1\0\2\65\2\0\2\65\1\u0246\3\65\1\0"+
"\26\65\1\0\12\65\1\u0246\26\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\21\65\1\u0247\17\65\1\0\5\65\1\u0247\14\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\1\65\1\u0248"+
"\24\65\1\0\20\65\1\u0248\20\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\22\65\1\u0249\16\65\1\0\1\65\1\u0249\20\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\22\65\1\u024a\16\65\1\0\1\65\1\u024a\20\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\21\65\1\u0192\17\65\1\0\5\65\1\u0192\14\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\13\65\1\u024b\25\65\1\0\2\65\1\u024b\17\65\1\0"+
"\1\65\1\0\2\65\2\0\3\65\1\u024c\2\65\1\0"+
"\26\65\1\0\7\65\1\u024c\31\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\22\65\1\112\16\65\1\0\1\65\1\112\20\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\1\65\1\u024d"+
"\24\65\1\0\20\65\1\u024d\20\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\3\65\1\363\2\65\1\0"+
"\26\65\1\0\7\65\1\363\31\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\2\65\1\u024e\3\65\1\0"+
"\26\65\1\0\12\65\1\u024e\26\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\2\65\1\u0111"+
"\23\65\1\0\22\65\1\112\4\65\1\u0111\11\65\1\0"+
"\1\65\1\112\20\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\12\65\1\112\13\65\1\0\41\65\1\0"+
"\6\65\1\112\13\65\1\0\1\65\1\0\2\65\2\0"+
"\5\65\1\u024f\1\0\26\65\1\0\14\65\1\u024f\24\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\26\65\1\0\31\65\1\112\7\65\1\0\10\65"+
"\1\112\1\u0250\10\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\26\65\1\0\10\65\1\u0251\30\65\1\0"+
"\7\65\1\u0251\12\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\2\65\1\363\23\65\1\0\27\65\1\363"+
"\11\65\1\0\20\65\12\0\1\u0252\53\0\1\u0252\120\0"+
"\2\u01ca\17\0\1\u01ca\41\0\1\u0253\120\0\1\127\10\0"+
"\1\127\1\u0254\4\127\1\0\2\127\1\u0254\1\127\4\u0254"+
"\1\127\1\0\1\127\1\0\3\127\1\0\1\127\1\u0254"+
"\14\0\2\127\1\0\5\127\1\u0254\1\127\2\u0254\1\127"+
"\1\u0254\2\127\1\u0254\3\127\1\u0254\1\127\2\0\1\127"+
"\2\0\1\127\1\u0254\3\127\1\u0254\6\127\1\u0254\2\127"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\1\u0255"+
"\1\136\1\0\22\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\u011d\10\0\6\u011d\1\0\4\u011d\2\u01ce\1\u011d"+
"\1\u01ce\1\u011d\1\0\1\u011d\1\0\3\u011d\1\0\1\u011d"+
"\1\u0120\14\0\2\u011d\1\0\7\u011d\1\u0120\2\u011d\1\u0120"+
"\10\u011d\2\0\1\u011d\2\0\5\u011d\1\u0120\6\u011d\1\u01ce"+
"\2\u011d\25\0\2\u01ce\1\0\1\u01ce\75\0\1\u01ce\3\0"+
"\1\u011d\10\0\1\u011d\1\u01d1\4\u011d\1\0\1\u011d\1\u01d0"+
"\1\u01d1\1\u011d\4\u01d1\1\u011d\1\0\1\u011d\1\0\3\u011d"+
"\1\0\1\u011d\1\u01d1\14\0\2\u011d\1\0\5\u011d\1\u01d1"+
"\1\u01d0\2\u01d1\1\u011d\1\u01d1\2\u011d\1\u01d1\3\u011d\1\u01d1"+
"\1\u011d\2\0\1\u011d\2\0\1\u011d\1\u01d1\3\u011d\1\u01d1"+
"\6\u011d\1\u01d1\2\u011d\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\11\136\1\u014b\10\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\15\136\1\u0256\4\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\2\136\1\u0257"+
"\17\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\1\u0258\1\136\1\0"+
"\22\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\10\136"+
"\1\u0259\11\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\1\136\1\u025a\4\136\1\u025b\13\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\1\136\1\u0128\20\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\u025c\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\22\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\15\136\1\u025d\4\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\3\136\1\u025e\16\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\11\136\1\u0128\10\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\1\136\1\u025f"+
"\20\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\15\136"+
"\1\u0260\4\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\6\136\1\u0128\13\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\1\u0261"+
"\1\136\1\0\22\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\2\136\1\u0262\6\136\1\u0263\10\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\1\u0264\21\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\11\136\1\u01dc\10\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\5\136\1\u0206\14\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\15\136\1\u0128"+
"\4\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\1\136\1\u0264\1\0"+
"\22\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\21\136"+
"\1\u0265\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\15\136"+
"\1\u0266\4\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\10\136\1\u0267\11\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\u0268"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\22\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\2\136\1\u0269\17\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\15\136\1\u0142\4\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\2\136\1\u026a\17\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\1\u026b\21\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\5\136\1\u026c\14\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\2\136\1\u01e4\17\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\5\136\1\u026d\14\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\10\136\1\u026e"+
"\11\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\10\136"+
"\1\u026b\11\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\11\136\1\u01da\10\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\6\136\1\u026a\13\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\20\136\1\u026f\1\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\2\136\1\u0270\17\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\11\136\1\u0271\10\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\17\136\1\u0272\2\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\16\136\1\u0128"+
"\3\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\4\136"+
"\1\u0273\15\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\10\136\1\u0274\11\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\6\136\1\u0275\13\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\15\136\1\u0276\4\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\1\136\1\u025a\20\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\7\136\1\u0128\12\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\10\136\1\u0277\11\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\10\136\1\u0278"+
"\11\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\6\136"+
"\1\u0279\13\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\15\136\1\u0264\4\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\4\136\1\u0128\15\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\6\136\1\u01e5\13\136\1\127\2\0\1\136"+
"\2\0\17\136\13\0\1\u027a\7\0\1\u027a\1\0\4\u027a"+
"\11\0\1\u027a\24\0\1\u027a\1\0\2\u027a\1\0\1\u027a"+
"\2\0\1\u027a\3\0\1\u027a\7\0\1\u027a\3\0\1\u027a"+
"\6\0\1\u027a\15\0\1\u027b\7\0\1\u027b\1\0\4\u027b"+
"\11\0\1\u027b\24\0\1\u027b\1\0\2\u027b\1\0\1\u027b"+
"\2\0\1\u027b\3\0\1\u027b\7\0\1\u027b\3\0\1\u027b"+
"\6\0\1\u027b\15\0\1\u027c\53\0\1\u027c\137\0\1\u027d"+
"\131\0\1\u020d\105\0\1\u027e\120\0\1\u027f\77\0\1\u0280"+
"\53\0\1\u0280\137\0\1\u0281\131\0\1\u0212\105\0\1\u0282"+
"\120\0\1\u0283\103\0\1\u0284\44\0\1\u0284\140\0\1\u0285"+
"\113\0\1\u0286\150\0\1\u0287\114\0\1\u021b\135\0\1\u0288"+
"\122\0\1\u0289\132\0\1\u028a\132\0\1\u028b\144\0\1\u028c"+
"\125\0\1\u028d\116\0\1\u028e\130\0\1\u028f\5\0\1\u0290"+
"\130\0\1\u0225\121\0\1\u0291\126\0\1\u0292\135\0\1\u0293"+
"\133\0\1\u0294\134\0\1\u0295\125\0\1\u0296\133\0\1\u0297"+
"\44\0\3\305\1\u0298\2\305\1\0\10\305\2\0\1\305"+
"\5\0\2\305\14\0\1\u0298\1\305\1\0\22\305\3\0"+
"\1\305\2\0\17\305\12\0\6\305\1\0\1\305\1\u0299"+
"\6\305\2\0\1\305\5\0\2\305\14\0\2\305\1\0"+
"\6\305\1\u0299\13\305\3\0\1\305\2\0\17\305\54\0"+
"\1\u022d\16\0\1\u0187\46\0\1\u029a\125\0\2\u029b\1\0"+
"\7\u022e\1\0\10\u022e\2\u029b\1\u022e\1\0\1\u029b\1\0"+
"\1\u022e\1\u029b\2\u022e\2\u029b\1\0\2\u029b\1\0\5\u029b"+
"\1\0\2\u022e\1\0\22\u022e\3\u029b\1\u022e\2\u029b\17\u022e"+
"\1\0\2\65\1\0\1\65\1\0\2\65\2\0\4\65"+
"\1\u01b1\1\65\1\0\26\65\1\0\26\65\1\u01b1\12\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\26\65\1\0\31\65\1\u0192\7\65\1\0\10\65"+
"\1\u0192\11\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\26\65\1\0\15\65\1\340\23\65\1\0\3\65"+
"\1\340\16\65\1\0\1\65\1\0\2\65\2\0\3\65"+
"\1\u023f\2\65\1\0\26\65\1\0\7\65\1\u023f\31\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\1\65"+
"\1\u01b1\4\65\1\0\26\65\1\0\17\65\1\u01b1\21\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\1\65"+
"\1\u0192\4\65\1\0\26\65\1\0\17\65\1\u0192\21\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\2\65"+
"\1\u029c\3\65\1\0\26\65\1\0\12\65\1\u029c\26\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\2\65\1\u029d\23\65\1\0\22\65\1\u023c\4\65"+
"\1\u029d\11\65\1\0\1\65\1\u023c\20\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\22\65"+
"\1\u029e\16\65\1\0\1\65\1\u029e\20\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\21\65"+
"\1\u029f\17\65\1\0\5\65\1\u029f\14\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\30\65"+
"\1\u0192\10\65\1\0\4\65\1\u0192\15\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\15\65"+
"\1\u02a0\23\65\1\0\3\65\1\u02a0\16\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\22\65"+
"\1\u02a1\16\65\1\0\1\65\1\u02a1\20\65\1\0\1\65"+
"\1\0\2\65\2\0\6\65\1\0\26\65\1\0\15\65"+
"\1\u010f\23\65\1\0\3\65\1\u010f\16\65\1\0\1\65"+
"\1\0\2\65\2\0\5\65\1\u02a2\1\0\26\65\1\0"+
"\14\65\1\u02a2\24\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\31\65\1\u02a3"+
"\7\65\1\0\10\65\1\u02a3\11\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\13\65\1\u0102"+
"\25\65\1\0\2\65\1\u0102\17\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\12\65\1\u023c\13\65\1\0"+
"\41\65\1\0\6\65\1\u023c\13\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\21\65\1\u02a4\4\65\1\0"+
"\24\65\1\u02a4\14\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\2\65\1\u02a5\23\65\1\0"+
"\27\65\1\u02a5\11\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\21\65\1\u02a6"+
"\17\65\1\0\5\65\1\u02a6\14\65\1\0\1\65\1\0"+
"\2\65\2\0\2\65\1\u0192\3\65\1\0\26\65\1\0"+
"\12\65\1\u0192\26\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\22\65\1\u02a7"+
"\16\65\1\0\1\65\1\u02a7\20\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\13\65\1\340"+
"\25\65\1\0\2\65\1\340\17\65\1\0\1\65\1\0"+
"\2\65\2\0\3\65\1\u02a8\2\65\1\0\26\65\1\0"+
"\7\65\1\u02a8\31\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\5\65\1\u02a9\1\0\1\65\1\u02aa\24\65"+
"\1\0\10\65\1\u02ab\3\65\1\u02a9\3\65\1\u02aa\20\65"+
"\1\0\7\65\1\u02ab\12\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\26\65\1\0\15\65\1\u02ac\23\65"+
"\1\0\3\65\1\u02ac\16\65\1\0\1\65\1\0\2\65"+
"\2\0\1\65\1\333\4\65\1\0\26\65\1\0\17\65"+
"\1\333\21\65\1\0\22\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\2\65\1\u01b1\23\65\1\0\27\65"+
"\1\u01b1\11\65\1\0\22\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\2\65\1\u02ad\23\65\1\0\27\65"+
"\1\u02ad\11\65\1\0\22\65\1\0\1\65\1\0\2\65"+
"\2\0\3\65\1\u02ae\2\65\1\0\26\65\1\0\7\65"+
"\1\u02ae\31\65\1\0\22\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\12\65\1\u02af\13\65\1\0\41\65"+
"\1\0\6\65\1\u02af\13\65\1\0\1\65\1\0\2\65"+
"\2\0\6\65\1\0\2\65\1\u0102\23\65\1\0\27\65"+
"\1\u0102\11\65\1\0\20\65\13\0\1\u02b0\45\0\1\u02b0"+
"\47\0\1\127\10\0\1\127\1\u02b1\4\127\1\0\2\127"+
"\1\u02b1\1\127\4\u02b1\1\127\1\0\1\127\1\0\3\127"+
"\1\0\1\127\1\u02b1\14\0\2\127\1\0\5\127\1\u02b1"+
"\1\127\2\u02b1\1\127\1\u02b1\2\127\1\u02b1\3\127\1\u02b1"+
"\1\127\2\0\1\127\2\0\1\127\1\u02b1\3\127\1\u02b1"+
"\6\127\1\u02b1\2\127\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\3\136\1\u02b2\16\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\1\u02b3\21\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\10\136\1\u02b4\11\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\3\136\1\u02b5"+
"\16\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\22\136"+
"\1\127\2\0\1\136\2\0\3\136\1\u0275\13\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\1\u0263\21\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\15\136\1\u02b6\4\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\1\u02b7\21\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\11\136\1\u02b8\10\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\22\136\1\127\2\0\1\136\2\0\1\u02b9"+
"\16\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\1\136\1\u02ba\20\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\20\136\1\u01e5\1\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\1\u02bb\1\136\1\0\22\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\2\136\1\u0128\17\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\6\136\1\u0205\13\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\2\136\1\u01e5"+
"\17\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\u02bc\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\22\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\1\136\1\u02bd\1\0\22\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\1\u02be\21\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\1\u02bf\1\136\1\0\22\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\2\136\1\u0264"+
"\17\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\4\136"+
"\1\u02c0\15\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\2\136\1\u02c1\17\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\6\136\1\u02c2\13\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\10\136\1\u0266\11\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\15\136\1\u02c3\4\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\15\136\1\u02c4\4\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\10\136\1\u02c5\11\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\14\136\1\u02c6"+
"\5\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\3\136"+
"\1\u02c7\16\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\2\136\1\u0153\17\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\17\136\1\u0128\2\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\15\136\1\u02c8\4\136\1\127\2\0\1\136"+
"\2\0\17\136\14\0\1\u02c9\45\0\1\u02c9\122\0\1\u027e"+
"\16\0\1\u020d\46\0\1\u02ca\125\0\2\u02cb\1\0\7\u027f"+
"\1\0\10\u027f\2\u02cb\1\u027f\1\0\1\u02cb\1\0\1\u027f"+
"\1\u02cb\2\u027f\2\u02cb\1\0\2\u02cb\1\0\5\u02cb\1\0"+
"\2\u027f\1\0\22\u027f\3\u02cb\1\u027f\2\u02cb\17\u027f\14\0"+
"\1\u02cc\45\0\1\u02cc\122\0\1\u0282\16\0\1\u0212\46\0"+
"\1\u02cd\125\0\2\u02ce\1\0\7\u0283\1\0\10\u0283\2\u02ce"+
"\1\u0283\1\0\1\u02ce\1\0\1\u0283\1\u02ce\2\u0283\2\u02ce"+
"\1\0\2\u02ce\1\0\5\u02ce\1\0\2\u0283\1\0\22\u0283"+
"\3\u02ce\1\u0283\2\u02ce\17\u0283\21\0\1\u02cf\53\0\1\u02cf"+
"\117\0\1\u028c\126\0\1\u02d0\142\0\1\u02d1\131\0\1\u02d2"+
"\113\0\1\u02d3\102\0\1\u02d4\175\0\1\u02d5\120\0\1\u02d6"+
"\142\0\1\u028a\107\0\1\u028a\134\0\1\u02d2\123\0\1\u02d7"+
"\144\0\1\u0290\70\0\1\u02d8\200\0\1\u02d9\111\0\1\u02da"+
"\123\0\1\u02db\134\0\1\u02dc\57\0\4\305\1\u02dd\1\305"+
"\1\0\10\305\2\0\1\305\5\0\2\305\14\0\2\305"+
"\1\0\14\305\1\u02dd\5\305\3\0\1\305\2\0\17\305"+
"\12\0\6\305\1\0\2\305\1\u02de\5\305\2\0\1\305"+
"\5\0\2\305\14\0\2\305\1\0\15\305\1\u02de\4\305"+
"\3\0\1\305\2\0\17\305\11\0\1\u022e\120\0\2\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\13\65\1\u02df\25\65\1\0\2\65\1\u02df\17\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\26\65"+
"\1\0\15\65\1\u01b1\23\65\1\0\3\65\1\u01b1\16\65"+
"\1\0\1\65\1\0\2\65\2\0\1\112\5\65\1\0"+
"\26\65\1\0\23\65\1\112\15\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\2\65\1\u02e0"+
"\23\65\1\0\27\65\1\u02e0\11\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\5\65\1\u02e1\1\0\26\65"+
"\1\0\14\65\1\u02e1\24\65\1\0\22\65\1\0\1\65"+
"\1\0\2\65\2\0\2\65\1\u02e2\3\65\1\0\26\65"+
"\1\0\12\65\1\u02e2\26\65\1\0\22\65\1\0\1\65"+
"\1\0\2\65\2\0\1\u02e3\5\65\1\0\26\65\1\0"+
"\23\65\1\u02e3\15\65\1\0\22\65\1\0\1\65\1\0"+
"\2\65\2\0\6\65\1\0\26\65\1\0\41\65\1\0"+
"\11\65\1\u02e4\10\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\26\65\1\0\13\65\1\u029d\25\65\1\0"+
"\2\65\1\u029d\17\65\1\0\1\65\1\0\2\65\2\0"+
"\1\u024d\5\65\1\0\26\65\1\0\23\65\1\u024d\15\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\26\65\1\0\30\65\1\u02e5\10\65\1\0\4\65"+
"\1\u02e5\15\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\1\65\1\u029e\24\65\1\0\20\65\1\u029e\20\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\2\65\1\u02e6\23\65\1\0\27\65\1\u02e6\11\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\3\65"+
"\1\373\2\65\1\0\26\65\1\0\7\65\1\373\31\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\2\65"+
"\1\u02e7\3\65\1\0\26\65\1\0\12\65\1\u02e7\26\65"+
"\1\0\22\65\1\0\1\65\1\0\2\65\2\0\6\65"+
"\1\0\1\u01b0\25\65\1\0\25\65\1\u01b0\13\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\1\u029e\5\65"+
"\1\0\26\65\1\0\23\65\1\u029e\15\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\1\65\1\u02e8\4\65"+
"\1\0\26\65\1\0\17\65\1\u02e8\21\65\1\0\22\65"+
"\1\0\1\65\1\0\2\65\2\0\6\65\1\0\2\65"+
"\1\u0192\23\65\1\0\27\65\1\u0192\11\65\1\0\20\65"+
"\14\0\1\u02e9\41\0\1\u02e9\52\0\1\127\10\0\1\127"+
"\1\u02ea\4\127\1\0\2\127\1\u02ea\1\127\4\u02ea\1\127"+
"\1\0\1\127\1\0\3\127\1\0\1\127\1\u02ea\14\0"+
"\2\127\1\0\5\127\1\u02ea\1\127\2\u02ea\1\127\1\u02ea"+
"\2\127\1\u02ea\3\127\1\u02ea\1\127\2\0\1\127\2\0"+
"\1\127\1\u02ea\3\127\1\u02ea\6\127\1\u02ea\2\127\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\1\u02eb\1\136"+
"\1\0\22\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\12\136\1\u02ec\7\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\3\136\1\u02ed\16\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\1\u02ee\1\136\1\0\22\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\16\136\1\u02ef\3\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\3\136\1\u02f0\16\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\1\u02f1\1\136\1\0\22\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\12\136\1\u0128\7\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\3\136\1\u02f2\16\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\6\136\1\u0263"+
"\13\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\1\136\1\u01ed\1\0"+
"\22\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\10\136"+
"\1\u02f3\11\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\5\136\1\u0128\14\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\1\u02f4\21\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\1\u02f5"+
"\1\136\1\0\22\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\6\136\1\u02f6\13\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\5\136\1\u02f7\14\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\3\136\1\u02f8\2\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\1\136\1\u02f9\14\0\2\136\1\0\22\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\1\136\1\u01e5\1\0\22\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\15\136\1\u0275\4\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\7\136\1\u01dc"+
"\12\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\10\136"+
"\1\u02fa\11\136\1\127\2\0\1\136\2\0\17\136\15\0"+
"\1\u02fb\41\0\1\u02fb\62\0\1\u027f\134\0\1\u02fc\41\0"+
"\1\u02fc\62\0\1\u0283\141\0\1\u02fd\45\0\1\u02fd\133\0"+
"\1\u02fe\120\0\1\u02ff\77\0\1\u0300\175\0\1\u021b\114\0"+
"\1\u0301\3\0\1\u0302\1\u0303\122\0\1\u028a\147\0\1\u0304"+
"\131\0\1\u0305\121\0\1\u028a\125\0\1\u0304\135\0\1\u028a"+
"\117\0\1\u0304\126\0\1\u0306\60\0\5\305\1\u0307\1\0"+
"\10\305\2\0\1\305\5\0\2\305\14\0\2\305\1\0"+
"\2\305\1\u0307\17\305\3\0\1\305\2\0\17\305\1\0"+
"\2\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\12\65\1\u0190\13\65\1\0\41\65\1\0\6\65\1\u0190"+
"\13\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\20\65\1\112\5\65\1\0\37\65\1\112\1\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\2\65\1\u0308\23\65\1\0\27\65\1\u0308\11\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\2\65\1\u01bf\23\65\1\0\27\65\1\u01bf\11\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\13\65\1\324\25\65\1\0\2\65\1\324"+
"\17\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\12\65\1\u0309\13\65\1\0\41\65\1\0\6\65\1\u0309"+
"\13\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\2\65\1\u029e\23\65\1\0\27\65\1\u029e\11\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\30\65\1\u030a\10\65\1\0\4\65\1\u030a"+
"\15\65\1\0\1\65\1\0\2\65\2\0\3\65\1\u010f"+
"\2\65\1\0\26\65\1\0\7\65\1\u010f\31\65\1\0"+
"\22\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\26\65\1\0\13\65\1\u01b7\25\65\1\0\2\65\1\u01b7"+
"\15\65\15\0\1\u030b\57\0\1\u030b\33\0\1\127\10\0"+
"\1\127\1\136\4\127\1\0\2\127\1\136\1\127\4\136"+
"\1\127\1\0\1\127\1\0\3\127\1\0\1\127\1\136"+
"\14\0\2\127\1\0\5\127\1\136\1\127\2\136\1\127"+
"\1\136\2\127\1\136\3\127\1\136\1\127\2\0\1\127"+
"\2\0\1\127\1\136\3\127\1\136\6\127\1\136\2\127"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\2\136\1\u02f6\17\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\10\136\1\u030c\11\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\5\136\1\u01de\14\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\2\136\1\u02c6\17\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\15\136\1\u030d\4\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\15\136\1\u030e"+
"\4\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\u01e5\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\22\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\5\136\1\u0263"+
"\14\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\1\136"+
"\1\u030f\20\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\1\136\1\u0310\20\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\13\136\1\u0128\6\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\2\136\1\u0311\17\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\3\136\1\u0312\16\136\1\127\2\0"+
"\1\136\2\0\17\136\1\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\6\136\1\u0313\13\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\3\136\1\u0264\16\136"+
"\1\127\2\0\1\136\2\0\17\136\16\0\1\u0314\57\0"+
"\1\u0314\50\0\1\u0315\57\0\1\u0315\55\0\1\u0316\53\0"+
"\1\u0316\115\0\1\u0317\126\0\1\u0305\131\0\1\u0301\3\0"+
"\1\u0302\135\0\1\u0318\122\0\1\u0319\134\0\1\u0292\135\0"+
"\1\u031a\130\0\1\u028a\115\0\1\u031b\45\0\2\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\20\65\1\u01b1"+
"\5\65\1\0\37\65\1\u01b1\1\65\1\0\22\65\1\0"+
"\1\65\1\0\2\65\2\0\6\65\1\0\26\65\1\0"+
"\13\65\1\327\25\65\1\0\2\65\1\327\17\65\1\0"+
"\1\65\1\0\2\65\2\0\4\65\1\u031c\1\65\1\0"+
"\26\65\1\0\26\65\1\u031c\12\65\1\0\20\65\16\0"+
"\1\u031d\44\0\1\u031d\45\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\5\136\1\u01e5\14\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\3\136\1\u031e\16\136"+
"\1\127\2\0\1\136\2\0\17\136\1\0\1\127\10\0"+
"\6\136\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a"+
"\1\136\1\0\2\136\14\0\2\136\1\0\3\136\1\u0263"+
"\16\136\1\127\2\0\1\136\2\0\17\136\1\0\1\127"+
"\10\0\6\136\1\0\11\136\1\0\1\136\1\0\1\127"+
"\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0\3\136"+
"\1\u031f\16\136\1\127\2\0\1\136\2\0\17\136\1\0"+
"\1\127\10\0\6\136\1\0\11\136\1\0\1\136\1\0"+
"\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136\1\0"+
"\3\136\1\u0128\16\136\1\127\2\0\1\136\2\0\17\136"+
"\1\0\1\127\10\0\6\136\1\0\11\136\1\0\1\136"+
"\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0\2\136"+
"\1\0\15\136\1\u0201\4\136\1\127\2\0\1\136\2\0"+
"\17\136\1\0\1\127\10\0\6\136\1\0\11\136\1\0"+
"\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136\14\0"+
"\2\136\1\0\2\136\1\u0275\17\136\1\127\2\0\1\136"+
"\2\0\17\136\1\0\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\1\136\1\u0320\20\136\1\127\2\0"+
"\1\136\2\0\17\136\17\0\1\u0321\44\0\1\u0321\63\0"+
"\1\u0322\44\0\1\u0322\64\0\1\u0323\142\0\1\u0324\130\0"+
"\1\u0325\155\0\1\u0326\142\0\1\u028a\131\0\1\u0327\37\0"+
"\2\65\1\0\1\65\1\0\2\65\2\0\6\65\1\0"+
"\1\65\1\u0328\24\65\1\0\20\65\1\u0328\20\65\1\0"+
"\20\65\17\0\1\u0329\111\0\1\127\10\0\6\136\1\0"+
"\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0"+
"\2\136\14\0\2\136\1\0\2\136\1\u01dc\17\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\1\u032a\1\136\1\0\22\136\1\127"+
"\2\0\1\136\2\0\17\136\1\0\1\127\10\0\6\136"+
"\1\0\11\136\1\0\1\136\1\0\1\127\1\u011a\1\136"+
"\1\0\2\136\14\0\2\136\1\0\10\136\1\u0312\11\136"+
"\1\127\2\0\1\136\2\0\17\136\20\0\1\u032b\130\0"+
"\1\u032c\200\0\1\u032d\124\0\1\u032e\134\0\1\u031a\125\0"+
"\1\u032f\44\0\2\65\1\0\1\65\1\0\2\65\2\0"+
"\6\65\1\0\26\65\1\0\22\65\1\327\16\65\1\0"+
"\1\65\1\327\16\65\1\127\10\0\6\136\1\0\11\136"+
"\1\0\1\136\1\0\1\127\1\u011a\1\136\1\0\2\136"+
"\14\0\2\136\1\0\22\136\1\127\2\0\1\136\2\0"+
"\16\136\1\u0311\57\0\1\u0330\17\0\1\u0331\126\0\1\u0332"+
"\117\0\1\u0333\131\0\1\u0305\127\0\1\u0285\142\0\1\u0305"+
"\33\0";
private static int [] zzUnpackTrans() {
int [] result = new int[63190];
int offset = 0;
offset = zzUnpackTrans(ZZ_TRANS_PACKED_0, offset, result);
return result;
}
private static int zzUnpackTrans(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
value--;
do result[j++] = value; while (--count > 0);
}
return j;
}
/* error codes */
private static final int ZZ_UNKNOWN_ERROR = 0;
private static final int ZZ_NO_MATCH = 1;
private static final int ZZ_PUSHBACK_2BIG = 2;
/* error messages for the codes above */
private static final String ZZ_ERROR_MSG[] = {
"Unkown internal scanner error",
"Error: could not match input",
"Error: pushback value was too large"
};
/**
* ZZ_ATTRIBUTE[aState] contains the attributes of state aState
*/
private static final int [] ZZ_ATTRIBUTE = zzUnpackAttribute();
private static final String ZZ_ATTRIBUTE_PACKED_0 =
"\6\0\2\1\1\0\2\1\1\0\2\1\7\0\1\1"+
"\5\0\2\1\1\11\4\1\1\11\5\1\1\11\2\1"+
"\2\11\2\1\1\11\1\1\3\11\1\1\1\11\25\1"+
"\1\11\1\1\1\11\1\1\7\11\1\1\1\11\2\1"+
"\2\11\10\1\1\11\3\1\1\11\20\1\1\11\2\1"+
"\1\11\1\1\2\11\2\1\1\11\1\1\1\11\1\1"+
"\1\11\6\1\1\11\4\1\2\11\1\1\1\11\4\1"+
"\1\11\1\1\3\11\4\1\4\11\1\1\1\11\6\1"+
"\4\11\1\1\2\11\1\1\1\11\2\1\1\11\2\1"+
"\1\11\1\1\1\11\1\1\1\11\4\1\2\11\5\0"+
"\1\11\103\1\1\11\3\0\1\11\1\0\75\1\1\11"+
"\2\1\1\0\1\11\12\0\1\11\1\1\15\0\1\1"+
"\1\11\5\0\1\1\1\0\4\11\3\1\1\0\1\11"+
"\4\0\100\1\1\0\1\1\1\0\3\1\1\0\70\1"+
"\41\0\2\1\1\11\2\0\44\1\1\0\1\11\46\1"+
"\1\0\1\11\3\0\1\1\3\0\1\1\6\0\1\11"+
"\15\0\2\1\2\0\24\1\1\0\30\1\24\0\14\1"+
"\1\0\21\1\14\0\4\1\1\0\10\1\10\0\1\1"+
"\1\0\3\1\2\0\1\11\4\0\1\1\1\11\1\1"+
"\2\11\6\0\1\11";
private static int [] zzUnpackAttribute() {
int [] result = new int[819];
int offset = 0;
offset = zzUnpackAttribute(ZZ_ATTRIBUTE_PACKED_0, offset, result);
return result;
}
private static int zzUnpackAttribute(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/** the input device */
private java.io.Reader zzReader;
/** the current state of the DFA */
private int zzState;
/** the current lexical state */
private int zzLexicalState = YYINITIAL;
/** this buffer contains the current text to be matched and is
the source of the yytext() string */
private char zzBuffer[];
/** the textposition at the last accepting state */
private int zzMarkedPos;
/** the current text position in the buffer */
private int zzCurrentPos;
/** startRead marks the beginning of the yytext() string in the buffer */
private int zzStartRead;
/** endRead marks the last character in the buffer, that has been read
from input */
private int zzEndRead;
/** zzAtEOF == true <=> the scanner is at the EOF */
private boolean zzAtEOF;
/* user code: */
/**
* Type specific to XMLTokenMaker denoting a line ending with an unclosed
* double-quote attribute.
*/
public static final int INTERNAL_ATTR_DOUBLE = -1;
/**
* Type specific to XMLTokenMaker denoting a line ending with an unclosed
* single-quote attribute.
*/
public static final int INTERNAL_ATTR_SINGLE = -2;
/**
* Token type specific to HTMLTokenMaker; this signals that the user has
* ended a line with an unclosed HTML tag; thus a new line is beginning
* still inside of the tag.
*/
public static final int INTERNAL_INTAG = -3;
/**
* Token type specific to HTMLTokenMaker; this signals that the user has
* ended a line with an unclosed <script>
tag.
*/
public static final int INTERNAL_INTAG_SCRIPT = -4;
/**
* Token type specifying we're in a double-qouted attribute in a
* script tag.
*/
public static final int INTERNAL_ATTR_DOUBLE_QUOTE_SCRIPT = -5;
/**
* Token type specifying we're in a single-qouted attribute in a
* script tag.
*/
public static final int INTERNAL_ATTR_SINGLE_QUOTE_SCRIPT = -6;
/**
* Token type specific to HTMLTokenMaker; this signals that the user has
* ended a line with an unclosed <style>
tag.
*/
public static final int INTERNAL_INTAG_STYLE = -7;
/**
* Token type specifying we're in a double-qouted attribute in a
* style tag.
*/
public static final int INTERNAL_ATTR_DOUBLE_QUOTE_STYLE = -8;
/**
* Token type specifying we're in a single-qouted attribute in a
* style tag.
*/
public static final int INTERNAL_ATTR_SINGLE_QUOTE_STYLE = -9;
/**
* Token type specifying we're in JavaScript.
*/
public static final int INTERNAL_IN_JS = -10;
/**
* Token type specifying we're in a JavaScript multiline comment.
*/
public static final int INTERNAL_IN_JS_MLC = -11;
/**
* Token type specifying we're in an invalid multi-line JS string.
*/
public static final int INTERNAL_IN_JS_STRING_INVALID = -12;
/**
* Token type specifying we're in a valid multi-line JS string.
*/
public static final int INTERNAL_IN_JS_STRING_VALID = -13;
/**
* Token type specifying we're in an invalid multi-line JS single-quoted string.
*/
public static final int INTERNAL_IN_JS_CHAR_INVALID = -14;
/**
* Token type specifying we're in a valid multi-line JS single-quoted string.
*/
public static final int INTERNAL_IN_JS_CHAR_VALID = -15;
/**
* Internal type denoting a line ending in CSS.
*/
public static final int INTERNAL_CSS = -16;
/**
* Internal type denoting a line ending in a CSS property.
*/
public static final int INTERNAL_CSS_PROPERTY = -17;
/**
* Internal type denoting a line ending in a CSS property value.
*/
public static final int INTERNAL_CSS_VALUE = -18;
/**
* Token type specifying we're in a valid multi-line template literal.
*/
private static final int INTERNAL_IN_JS_TEMPLATE_LITERAL_VALID = -23;
/**
* Token type specifying we're in an invalid multi-line template literal.
*/
private static final int INTERNAL_IN_JS_TEMPLATE_LITERAL_INVALID = -24;
/**
* Internal type denoting line ending in a CSS double-quote string.
* The state to return to is embedded in the actual end token type.
*/
public static final int INTERNAL_CSS_STRING = -(1<<11);
/**
* Internal type denoting line ending in a CSS single-quote string.
* The state to return to is embedded in the actual end token type.
*/
public static final int INTERNAL_CSS_CHAR = -(2<<11);
/**
* Internal type denoting line ending in a CSS multi-line comment.
* The state to return to is embedded in the actual end token type.
*/
public static final int INTERNAL_CSS_MLC = -(3<<11);
/**
* The state previous CSS-related state we were in before going into a CSS
* string, multi-line comment, etc.
*/
private int cssPrevState;
/**
* Whether closing markup tags are automatically completed for HTML.
*/
private static boolean completeCloseTags;
/**
* When in the JS_STRING state, whether the current string is valid.
*/
private boolean validJSString;
/**
* Language state set on HTML tokens. Must be 0.
*/
private static final int LANG_INDEX_DEFAULT = 0;
/**
* Language state set on JavaScript tokens.
*/
private static final int LANG_INDEX_JS = 1;
/**
* Language state set on CSS tokens.
*/
private static final int LANG_INDEX_CSS = 2;
private Stack varDepths;
/**
* Constructor. This must be here because JFlex does not generate a
* no-parameter constructor.
*/
public HTMLTokenMaker() {
super();
}
/**
* Adds the token specified to the current linked list of tokens as an
* "end token;" that is, at zzMarkedPos
.
*
* @param tokenType The token's type.
*/
private void addEndToken(int tokenType) {
addToken(zzMarkedPos,zzMarkedPos, tokenType);
}
/**
* Adds the token specified to the current linked list of tokens.
*
* @param tokenType The token's type.
* @see #addToken(int, int, int)
*/
private void addHyperlinkToken(int start, int end, int tokenType) {
int so = start + offsetShift;
addToken(zzBuffer, start,end, tokenType, so, true);
}
/**
* Adds the token specified to the current linked list of tokens.
*
* @param tokenType The token's type.
*/
private void addToken(int tokenType) {
addToken(zzStartRead, zzMarkedPos-1, tokenType);
}
/**
* Adds the token specified to the current linked list of tokens.
*
* @param tokenType The token's type.
*/
private void addToken(int start, int end, int tokenType) {
int so = start + offsetShift;
addToken(zzBuffer, start,end, tokenType, so);
}
/**
* Adds the token specified to the current linked list of tokens.
*
* @param array The character array.
* @param start The starting offset in the array.
* @param end The ending offset in the array.
* @param tokenType The token's type.
* @param startOffset The offset in the document at which this token
* occurs.
*/
@Override
public void addToken(char[] array, int start, int end, int tokenType, int startOffset) {
super.addToken(array, start,end, tokenType, startOffset);
zzStartRead = zzMarkedPos;
}
/**
* {@inheritDoc}
*/
@Override
protected OccurrenceMarker createOccurrenceMarker() {
return new HtmlOccurrenceMarker();
}
/**
* Sets whether markup close tags should be completed. You might not want
* this to be the case, since some tags in standard HTML aren't usually
* closed.
*
* @return Whether closing markup tags are completed.
* @see #setCompleteCloseTags(boolean)
*/
@Override
public boolean getCompleteCloseTags() {
return completeCloseTags;
}
@Override
public boolean getCurlyBracesDenoteCodeBlocks(int languageIndex) {
return languageIndex==LANG_INDEX_CSS || languageIndex==LANG_INDEX_JS;
}
/**
* {@inheritDoc}
*/
@Override
public String[] getLineCommentStartAndEnd(int languageIndex) {
switch (languageIndex) {
case LANG_INDEX_JS:
return new String[] { "//", null };
case LANG_INDEX_CSS:
return new String[] { "/*", "*/" };
default:
return new String[] { "" };
}
}
/**
* Returns Token.MARKUP_TAG_NAME
.
*
* @param type The token type.
* @return Whether tokens of this type should have "mark occurrences"
* enabled.
*/
@Override
public boolean getMarkOccurrencesOfTokenType(int type) {
return type==Token.MARKUP_TAG_NAME;
}
/**
* Overridden to handle newlines in JS and CSS differently than those in
* markup.
*/
@Override
public boolean getShouldIndentNextLineAfter(Token token) {
int languageIndex = token==null ? 0 : token.getLanguageIndex();
if (getCurlyBracesDenoteCodeBlocks(languageIndex)) {
if (token!=null && token.length()==1) {
char ch = token.charAt(0);
return ch=='{' || ch=='(';
}
}
return false;
}
/**
* Returns the first token in the linked list of tokens generated
* from text
. This method must be implemented by
* subclasses so they can correctly implement syntax highlighting.
*
* @param text The text from which to get tokens.
* @param initialTokenType The token type we should start with.
* @param startOffset The offset into the document at which
* text
starts.
* @return The first Token
in a linked list representing
* the syntax highlighted text.
*/
@Override
public Token getTokenList(Segment text, int initialTokenType, int startOffset) {
resetTokenList();
this.offsetShift = -text.offset + startOffset;
cssPrevState = CSS; // Shouldn't be necessary
int languageIndex = 0;
// Start off in the proper state.
int state = Token.NULL;
switch (initialTokenType) {
case Token.MARKUP_COMMENT:
state = COMMENT;
break;
case Token.PREPROCESSOR:
state = PI;
break;
case Token.VARIABLE:
state = DTD;
break;
case INTERNAL_INTAG:
state = INTAG;
break;
case INTERNAL_INTAG_SCRIPT:
state = INTAG_SCRIPT;
break;
case INTERNAL_INTAG_STYLE:
state = INTAG_STYLE;
break;
case INTERNAL_ATTR_DOUBLE:
state = INATTR_DOUBLE;
break;
case INTERNAL_ATTR_SINGLE:
state = INATTR_SINGLE;
break;
case INTERNAL_ATTR_DOUBLE_QUOTE_SCRIPT:
state = INATTR_DOUBLE_SCRIPT;
break;
case INTERNAL_ATTR_SINGLE_QUOTE_SCRIPT:
state = INATTR_SINGLE_SCRIPT;
break;
case INTERNAL_ATTR_DOUBLE_QUOTE_STYLE:
state = INATTR_DOUBLE_STYLE;
break;
case INTERNAL_ATTR_SINGLE_QUOTE_STYLE:
state = INATTR_SINGLE_STYLE;
break;
case INTERNAL_IN_JS:
state = JAVASCRIPT;
languageIndex = LANG_INDEX_JS;
break;
case INTERNAL_IN_JS_MLC:
state = JS_MLC;
languageIndex = LANG_INDEX_JS;
break;
case INTERNAL_IN_JS_STRING_INVALID:
state = JS_STRING;
validJSString = false;
languageIndex = LANG_INDEX_JS;
break;
case INTERNAL_IN_JS_STRING_VALID:
state = JS_STRING;
validJSString = true;
languageIndex = LANG_INDEX_JS;
break;
case INTERNAL_IN_JS_CHAR_INVALID:
state = JS_CHAR;
validJSString = false;
languageIndex = LANG_INDEX_JS;
break;
case INTERNAL_IN_JS_CHAR_VALID:
state = JS_CHAR;
validJSString = true;
languageIndex = LANG_INDEX_JS;
break;
case INTERNAL_CSS:
state = CSS;
languageIndex = LANG_INDEX_CSS;
break;
case INTERNAL_CSS_PROPERTY:
state = CSS_PROPERTY;
languageIndex = LANG_INDEX_CSS;
break;
case INTERNAL_CSS_VALUE:
state = CSS_VALUE;
languageIndex = LANG_INDEX_CSS;
break;
case INTERNAL_IN_JS_TEMPLATE_LITERAL_VALID:
state = JS_TEMPLATE_LITERAL;
validJSString = true;
languageIndex = LANG_INDEX_JS;
break;
case INTERNAL_IN_JS_TEMPLATE_LITERAL_INVALID:
state = JS_TEMPLATE_LITERAL;
validJSString = false;
languageIndex = LANG_INDEX_JS;
break;
default:
if (initialTokenType<-1024) {
int main = -(-initialTokenType & 0xffffff00);
switch (main) {
default: // Should never happen
case INTERNAL_CSS_STRING:
state = CSS_STRING;
break;
case INTERNAL_CSS_CHAR:
state = CSS_CHAR_LITERAL;
break;
case INTERNAL_CSS_MLC:
state = CSS_C_STYLE_COMMENT;
break;
}
cssPrevState = -initialTokenType&0xff;
languageIndex = LANG_INDEX_CSS;
}
else {
state = Token.NULL;
}
break;
}
setLanguageIndex(languageIndex);
start = text.offset;
s = text;
try {
yyreset(zzReader);
yybegin(state);
return yylex();
} catch (IOException ioe) {
ioe.printStackTrace();
return new TokenImpl();
}
}
/**
* Sets whether markup close tags should be completed. You might not want
* this to be the case, since some tags in standard HTML aren't usually
* closed.
*
* @param complete Whether closing markup tags are completed.
* @see #getCompleteCloseTags()
*/
public static void setCompleteCloseTags(boolean complete) {
completeCloseTags = complete;
}
/**
* Refills the input buffer.
*
* @return true
if EOF was reached, otherwise
* false
.
*/
private boolean zzRefill() {
return zzCurrentPos>=s.offset+s.count;
}
/**
* Resets the scanner to read from a new input stream.
* Does not close the old reader.
*
* All internal variables are reset, the old input stream
* cannot be reused (internal buffer is discarded and lost).
* Lexical state is set to YY_INITIAL.
*
* @param reader the new input stream
*/
public final void yyreset(java.io.Reader reader) {
// 's' has been updated.
zzBuffer = s.array;
/*
* We replaced the line below with the two below it because zzRefill
* no longer "refills" the buffer (since the way we do it, it's always
* "full" the first time through, since it points to the segment's
* array). So, we assign zzEndRead here.
*/
//zzStartRead = zzEndRead = s.offset;
zzStartRead = s.offset;
zzEndRead = zzStartRead + s.count - 1;
zzCurrentPos = zzMarkedPos = s.offset;
zzLexicalState = YYINITIAL;
zzReader = reader;
zzAtEOF = false;
}
/**
* Creates a new scanner
* There is also a java.io.InputStream version of this constructor.
*
* @param in the java.io.Reader to read input from.
*/
public HTMLTokenMaker(java.io.Reader in) {
this.zzReader = in;
}
/**
* Creates a new scanner.
* There is also java.io.Reader version of this constructor.
*
* @param in the java.io.Inputstream to read input from.
*/
public HTMLTokenMaker(java.io.InputStream in) {
this(new java.io.InputStreamReader(in));
}
/**
* Unpacks the compressed character translation table.
*
* @param packed the packed character translation table
* @return the unpacked character translation table
*/
private static char [] zzUnpackCMap(String packed) {
char [] map = new char[0x10000];
int i = 0; /* index in packed string */
int j = 0; /* index in unpacked array */
while (i < 194) {
int count = packed.charAt(i++);
char value = packed.charAt(i++);
do map[j++] = value; while (--count > 0);
}
return map;
}
/**
* Closes the input stream.
*/
public final void yyclose() throws java.io.IOException {
zzAtEOF = true; /* indicate end of file */
zzEndRead = zzStartRead; /* invalidate buffer */
if (zzReader != null)
zzReader.close();
}
/**
* Returns the current lexical state.
*/
public final int yystate() {
return zzLexicalState;
}
/**
* Enters a new lexical state
*
* @param newState the new lexical state
*/
@Override
public final void yybegin(int newState) {
zzLexicalState = newState;
}
/**
* Returns the text matched by the current regular expression.
*/
public final String yytext() {
return new String( zzBuffer, zzStartRead, zzMarkedPos-zzStartRead );
}
/**
* Returns the character at position pos from the
* matched text.
*
* It is equivalent to yytext().charAt(pos), but faster
*
* @param pos the position of the character to fetch.
* A value from 0 to yylength()-1.
*
* @return the character at position pos
*/
public final char yycharat(int pos) {
return zzBuffer[zzStartRead+pos];
}
/**
* Returns the length of the matched text region.
*/
public final int yylength() {
return zzMarkedPos-zzStartRead;
}
/**
* Reports an error that occured while scanning.
*
* In a wellformed scanner (no or only correct usage of
* yypushback(int) and a match-all fallback rule) this method
* will only be called with things that "Can't Possibly Happen".
* If this method is called, something is seriously wrong
* (e.g. a JFlex bug producing a faulty scanner etc.).
*
* Usual syntax/scanner level error handling should be done
* in error fallback rules.
*
* @param errorCode the code of the errormessage to display
*/
private void zzScanError(int errorCode) {
String message;
try {
message = ZZ_ERROR_MSG[errorCode];
}
catch (ArrayIndexOutOfBoundsException e) {
message = ZZ_ERROR_MSG[ZZ_UNKNOWN_ERROR];
}
throw new Error(message);
}
/**
* Pushes the specified amount of characters back into the input stream.
*
* They will be read again by then next call of the scanning method
*
* @param number the number of characters to be read again.
* This number must not be greater than yylength()!
*/
public void yypushback(int number) {
if ( number > yylength() )
zzScanError(ZZ_PUSHBACK_2BIG);
zzMarkedPos -= number;
}
/**
* Resumes scanning until the next regular expression is matched,
* the end of input is encountered or an I/O-Error occurs.
*
* @return the next token
* @exception java.io.IOException if any I/O-Error occurs
*/
public org.fife.ui.rsyntaxtextarea.Token yylex() throws java.io.IOException {
int zzInput;
int zzAction;
// cached fields:
int zzCurrentPosL;
int zzMarkedPosL;
int zzEndReadL = zzEndRead;
char [] zzBufferL = zzBuffer;
char [] zzCMapL = ZZ_CMAP;
int [] zzTransL = ZZ_TRANS;
int [] zzRowMapL = ZZ_ROWMAP;
int [] zzAttrL = ZZ_ATTRIBUTE;
while (true) {
zzMarkedPosL = zzMarkedPos;
zzAction = -1;
zzCurrentPosL = zzCurrentPos = zzStartRead = zzMarkedPosL;
zzState = zzLexicalState;
zzForAction: {
while (true) {
if (zzCurrentPosL < zzEndReadL)
zzInput = zzBufferL[zzCurrentPosL++];
else if (zzAtEOF) {
zzInput = YYEOF;
break zzForAction;
}
else {
// store back cached positions
zzCurrentPos = zzCurrentPosL;
zzMarkedPos = zzMarkedPosL;
boolean eof = zzRefill();
// get translated positions and possibly new buffer
zzCurrentPosL = zzCurrentPos;
zzMarkedPosL = zzMarkedPos;
zzBufferL = zzBuffer;
zzEndReadL = zzEndRead;
if (eof) {
zzInput = YYEOF;
break zzForAction;
}
else {
zzInput = zzBufferL[zzCurrentPosL++];
}
}
int zzNext = zzTransL[ zzRowMapL[zzState] + zzCMapL[zzInput] ];
if (zzNext == -1) break zzForAction;
zzState = zzNext;
int zzAttributes = zzAttrL[zzState];
if ( (zzAttributes & 1) == 1 ) {
zzAction = zzState;
zzMarkedPosL = zzCurrentPosL;
if ( (zzAttributes & 8) == 8 ) break zzForAction;
}
}
}
// store back cached position
zzMarkedPos = zzMarkedPosL;
switch (zzAction < 0 ? zzAction : ZZ_ACTION[zzAction]) {
case 55:
{ addToken(Token.OPERATOR); yybegin(CSS_VALUE);
}
case 113: break;
case 80:
{ addToken(Token.ERROR_NUMBER_FORMAT);
}
case 114: break;
case 64:
{ addToken(start,zzStartRead-1, Token.LITERAL_CHAR); addEndToken(INTERNAL_CSS_CHAR - cssPrevState); return firstToken;
}
case 115: break;
case 23:
{ start = zzMarkedPos-1; yybegin(INATTR_SINGLE_SCRIPT);
}
case 116: break;
case 10:
{ yybegin(YYINITIAL); addToken(start,zzStartRead, Token.MARKUP_DTD);
}
case 117: break;
case 49:
{ addToken(Token.SEPARATOR); yybegin(CSS_PROPERTY);
}
case 118: break;
case 4:
{ addToken(Token.MARKUP_TAG_DELIMITER); yybegin(INTAG);
}
case 119: break;
case 106:
{ addToken(Token.RESERVED_WORD_2);
}
case 120: break;
case 90:
{ addToken(start,zzStartRead+1, Token.COMMENT_MULTILINE); yybegin(cssPrevState);
}
case 121: break;
case 87:
{ start = zzMarkedPos-2; cssPrevState = zzLexicalState; yybegin(CSS_C_STYLE_COMMENT);
}
case 122: break;
case 40:
{ /* Line ending in '\' => continue to next line. */
if (validJSString) {
addToken(start,zzStartRead, Token.LITERAL_CHAR);
addEndToken(INTERNAL_IN_JS_CHAR_VALID);
}
else {
addToken(start,zzStartRead, Token.ERROR_CHAR);
addEndToken(INTERNAL_IN_JS_CHAR_INVALID);
}
return firstToken;
}
case 123: break;
case 5:
{ addToken(Token.WHITESPACE);
}
case 124: break;
case 107:
{ addToken(zzStartRead,zzStartRead, Token.MARKUP_TAG_DELIMITER);
addToken(zzMarkedPos-6,zzMarkedPos-1, Token.MARKUP_TAG_NAME);
start = zzMarkedPos; yybegin(INTAG_SCRIPT);
}
case 125: break;
case 89:
{ addToken(Token.REGEX);
}
case 126: break;
case 42:
{ addToken(start,zzStartRead-1, Token.COMMENT_MULTILINE); addEndToken(INTERNAL_IN_JS_MLC); return firstToken;
}
case 127: break;
case 111:
{ int temp = zzStartRead;
addToken(start,zzStartRead-1, Token.COMMENT_EOL);
yybegin(YYINITIAL, LANG_INDEX_DEFAULT);
addToken(temp,temp+1, Token.MARKUP_TAG_DELIMITER);
addToken(zzMarkedPos-7,zzMarkedPos-2, Token.MARKUP_TAG_NAME);
addToken(zzMarkedPos-1,zzMarkedPos-1, Token.MARKUP_TAG_DELIMITER);
}
case 128: break;
case 102:
{ addToken(Token.FUNCTION);
}
case 129: break;
case 8:
{ addToken(start,zzStartRead-1, Token.MARKUP_PROCESSING_INSTRUCTION); return firstToken;
}
case 130: break;
case 36:
{ addToken(start,zzStartRead-1, Token.ERROR_STRING_DOUBLE); addEndToken(INTERNAL_IN_JS); return firstToken;
}
case 131: break;
case 46:
{ start = zzMarkedPos-1; cssPrevState = zzLexicalState; yybegin(CSS_STRING);
}
case 132: break;
case 91:
{ addToken(start, zzStartRead - 1, Token.LITERAL_BACKQUOTE);
start = zzMarkedPos-2;
if (varDepths==null) {
varDepths = new Stack();
}
else {
varDepths.clear();
}
varDepths.push(Boolean.TRUE);
yybegin(JS_TEMPLATE_LITERAL_EXPR);
}
case 133: break;
case 72:
{ if (!varDepths.empty()) {
varDepths.pop();
if (varDepths.empty()) {
addToken(start,zzStartRead, Token.VARIABLE);
start = zzMarkedPos;
yybegin(JS_TEMPLATE_LITERAL);
}
}
}
case 134: break;
case 67:
{ if (validJSString) {
addToken(start, zzStartRead - 1, Token.LITERAL_BACKQUOTE);
addEndToken(INTERNAL_IN_JS_TEMPLATE_LITERAL_VALID);
}
else {
addToken(start,zzStartRead - 1, Token.ERROR_STRING_DOUBLE);
addEndToken(INTERNAL_IN_JS_TEMPLATE_LITERAL_INVALID);
}
return firstToken;
}
case 135: break;
case 69:
{ /* Skip valid '$' that is not part of template literal expression start */
}
case 136: break;
case 20:
{ yybegin(INTAG); addToken(start,zzStartRead, Token.MARKUP_TAG_ATTRIBUTE_VALUE);
}
case 137: break;
case 25:
{ start = zzMarkedPos-1; yybegin(INATTR_DOUBLE_STYLE);
}
case 138: break;
case 35:
{ start = zzMarkedPos-1; validJSString = true; yybegin(JS_TEMPLATE_LITERAL);
}
case 139: break;
case 83:
{ /* Skip all escaped chars. */
}
case 140: break;
case 77:
{ addToken(Token.MARKUP_TAG_DELIMITER); yybegin(YYINITIAL);
}
case 141: break;
case 28:
{ yybegin(INTAG_STYLE); addToken(start,zzStartRead, Token.MARKUP_TAG_ATTRIBUTE_VALUE);
}
case 142: break;
case 101:
{ if(JavaScriptTokenMaker.isJavaScriptCompatible("1.6")){ addToken(Token.RESERVED_WORD);} else {addToken(Token.IDENTIFIER);}
}
case 143: break;
case 96:
{ if(JavaScriptTokenMaker.isJavaScriptCompatible("1.7")){ addToken(Token.RESERVED_WORD);} else {addToken(Token.IDENTIFIER);}
}
case 144: break;
case 56:
{ /*System.out.println("css_value: " + yytext());*/ addToken(Token.IDENTIFIER);
}
case 145: break;
case 17:
{ /* A non-recognized HTML tag name */ yypushback(yylength()); yybegin(INTAG);
}
case 146: break;
case 26:
{ addToken(Token.MARKUP_TAG_DELIMITER); yybegin(CSS, LANG_INDEX_CSS);
}
case 147: break;
case 65:
{ addToken(start,zzStartRead, Token.LITERAL_CHAR); yybegin(cssPrevState);
}
case 148: break;
case 92:
{ varDepths.push(Boolean.TRUE);
}
case 149: break;
case 27:
{ start = zzMarkedPos-1; yybegin(INATTR_SINGLE_STYLE);
}
case 150: break;
case 7:
{ addToken(start,zzStartRead-1, Token.MARKUP_COMMENT); return firstToken;
}
case 151: break;
case 85:
{ /* Invalid latin-1 character \xXX */ validJSString = false;
}
case 152: break;
case 24:
{ yybegin(INTAG_SCRIPT); addToken(start,zzStartRead, Token.MARKUP_TAG_ATTRIBUTE_VALUE);
}
case 153: break;
case 37:
{ int type = validJSString ? Token.LITERAL_STRING_DOUBLE_QUOTE : Token.ERROR_STRING_DOUBLE; addToken(start,zzStartRead, type); yybegin(JAVASCRIPT);
}
case 154: break;
case 99:
{ addToken(Token.COMMENT_MULTILINE);
}
case 155: break;
case 75:
{ start = zzMarkedPos-2; yybegin(PI);
}
case 156: break;
case 108:
{ yybegin(YYINITIAL, LANG_INDEX_DEFAULT);
addToken(zzStartRead,zzStartRead+1, Token.MARKUP_TAG_DELIMITER);
addToken(zzMarkedPos-6,zzMarkedPos-2, Token.MARKUP_TAG_NAME);
addToken(zzMarkedPos-1,zzMarkedPos-1, Token.MARKUP_TAG_DELIMITER);
}
case 157: break;
case 51:
{ /*System.out.println("css_property: " + yytext());*/ addToken(Token.IDENTIFIER);
}
case 158: break;
case 9:
{ addToken(start,zzStartRead-1, Token.MARKUP_DTD); return firstToken;
}
case 159: break;
case 73:
{ int count = yylength();
addToken(zzStartRead,zzStartRead, Token.MARKUP_TAG_DELIMITER);
zzMarkedPos -= (count-1); //yypushback(count-1);
yybegin(INTAG_CHECK_TAG_NAME);
}
case 160: break;
case 63:
{ /* Skip escaped chars. */
}
case 161: break;
case 84:
{ /* Invalid Unicode character \\uXXXX */ validJSString = false;
}
case 162: break;
case 105:
{ addToken(zzStartRead,zzStartRead, Token.MARKUP_TAG_DELIMITER);
addToken(zzMarkedPos-5,zzMarkedPos-1, Token.MARKUP_TAG_NAME);
start = zzMarkedPos; cssPrevState = zzLexicalState; yybegin(INTAG_STYLE);
}
case 163: break;
case 76:
{ yybegin(YYINITIAL); addToken(start,zzStartRead+1, Token.MARKUP_PROCESSING_INSTRUCTION);
}
case 164: break;
case 86:
{ yybegin(JAVASCRIPT); addToken(start,zzStartRead+1, Token.COMMENT_MULTILINE);
}
case 165: break;
case 30:
{ addEndToken(INTERNAL_IN_JS); return firstToken;
}
case 166: break;
case 39:
{ addToken(start,zzStartRead-1, Token.ERROR_CHAR); addEndToken(INTERNAL_IN_JS); return firstToken;
}
case 167: break;
case 15:
{ addToken(Token.OPERATOR);
}
case 168: break;
case 58:
{ addToken(Token.OPERATOR); yybegin(CSS_PROPERTY);
}
case 169: break;
case 97:
{ start = zzMarkedPos-4; yybegin(COMMENT);
}
case 170: break;
case 94:
{ yybegin(YYINITIAL); addToken(start,zzStartRead+2, Token.MARKUP_COMMENT);
}
case 171: break;
case 110:
{ yybegin(YYINITIAL, LANG_INDEX_DEFAULT);
int temp = zzStartRead;
addToken(start,zzStartRead-1, Token.COMMENT_MULTILINE);
addToken(temp,temp+1, Token.MARKUP_TAG_DELIMITER);
addToken(zzMarkedPos-7,zzMarkedPos-2, Token.MARKUP_TAG_NAME);
addToken(zzMarkedPos-1,zzMarkedPos-1, Token.MARKUP_TAG_DELIMITER);
}
case 172: break;
case 88:
{ addToken(Token.VARIABLE);
}
case 173: break;
case 44:
{ /*System.out.println("CSS: " + yytext());*/ addToken(Token.IDENTIFIER);
}
case 174: break;
case 70:
{ int type = validJSString ? Token.LITERAL_BACKQUOTE : Token.ERROR_STRING_DOUBLE; addToken(start,zzStartRead, type); yybegin(JAVASCRIPT);
}
case 175: break;
case 2:
{ addToken(Token.IDENTIFIER);
}
case 176: break;
case 103:
{ int temp=zzStartRead; addToken(start,zzStartRead-1, Token.COMMENT_MULTILINE); addHyperlinkToken(temp,zzMarkedPos-1, Token.COMMENT_MULTILINE); start = zzMarkedPos;
}
case 177: break;
case 22:
{ addToken(Token.MARKUP_TAG_DELIMITER); yybegin(JAVASCRIPT, LANG_INDEX_JS);
}
case 178: break;
case 104:
{ int temp=zzStartRead; addToken(start,zzStartRead-1, Token.COMMENT_EOL); addHyperlinkToken(temp,zzMarkedPos-1, Token.COMMENT_EOL); start = zzMarkedPos;
}
case 179: break;
case 54:
{ addToken(Token.SEPARATOR); yybegin(CSS);
}
case 180: break;
case 31:
{ start = zzMarkedPos-1; validJSString = true; yybegin(JS_STRING);
}
case 181: break;
case 62:
{ addToken(start,zzStartRead, Token.LITERAL_STRING_DOUBLE_QUOTE); yybegin(cssPrevState);
}
case 182: break;
case 52:
{ addEndToken(INTERNAL_CSS_PROPERTY); return firstToken;
}
case 183: break;
case 68:
{ if (validJSString) {
addToken(start,zzStartRead, Token.LITERAL_BACKQUOTE);
addEndToken(INTERNAL_IN_JS_TEMPLATE_LITERAL_VALID);
}
else {
addToken(start,zzStartRead, Token.ERROR_STRING_DOUBLE);
addEndToken(INTERNAL_IN_JS_TEMPLATE_LITERAL_INVALID);
}
return firstToken;
}
case 184: break;
case 41:
{ int type = validJSString ? Token.LITERAL_CHAR : Token.ERROR_CHAR; addToken(start,zzStartRead, type); yybegin(JAVASCRIPT);
}
case 185: break;
case 34:
{ start = zzMarkedPos-1; validJSString = true; yybegin(JS_CHAR);
}
case 186: break;
case 79:
{ start = zzMarkedPos-2; yybegin(JS_MLC);
}
case 187: break;
case 82:
{ addToken(Token.LITERAL_NUMBER_HEXADECIMAL);
}
case 188: break;
case 60:
{ int temp = zzMarkedPos - 2;
addToken(zzStartRead, temp, Token.FUNCTION);
addToken(zzMarkedPos-1, zzMarkedPos-1, Token.SEPARATOR);
zzStartRead = zzCurrentPos = zzMarkedPos;
}
case 189: break;
case 112:
{ addToken(Token.ANNOTATION);
}
case 190: break;
case 29:
{ addToken(Token.ERROR_IDENTIFIER);
}
case 191: break;
case 57:
{ addEndToken(INTERNAL_CSS_VALUE); return firstToken;
}
case 192: break;
case 98:
{ int temp=zzStartRead; addToken(start,zzStartRead-1, Token.MARKUP_COMMENT); addHyperlinkToken(temp,zzMarkedPos-1, Token.MARKUP_COMMENT); start = zzMarkedPos;
}
case 193: break;
case 6:
{ addToken(Token.MARKUP_ENTITY_REFERENCE);
}
case 194: break;
case 100:
{ addToken(Token.LITERAL_BOOLEAN);
}
case 195: break;
case 18:
{ /* Shouldn't happen */ yypushback(1); yybegin(INTAG);
}
case 196: break;
case 3:
{ addNullToken(); return firstToken;
}
case 197: break;
case 45:
{ addEndToken(INTERNAL_CSS); return firstToken;
}
case 198: break;
case 109:
{ yybegin(YYINITIAL, LANG_INDEX_DEFAULT);
addToken(zzStartRead,zzStartRead+1, Token.MARKUP_TAG_DELIMITER);
addToken(zzMarkedPos-7,zzMarkedPos-2, Token.MARKUP_TAG_NAME);
addToken(zzMarkedPos-1,zzMarkedPos-1, Token.MARKUP_TAG_DELIMITER);
}
case 199: break;
case 50:
{ start = zzMarkedPos-1; cssPrevState = zzLexicalState; yybegin(CSS_CHAR_LITERAL);
}
case 200: break;
case 53:
{ addToken(Token.RESERVED_WORD);
}
case 201: break;
case 12:
{ start = zzMarkedPos-1; yybegin(INATTR_DOUBLE);
}
case 202: break;
case 14:
{ yybegin(YYINITIAL); addToken(Token.MARKUP_TAG_DELIMITER);
}
case 203: break;
case 47:
{ addToken(Token.DATA_TYPE);
}
case 204: break;
case 33:
{ addToken(Token.SEPARATOR);
}
case 205: break;
case 93:
{ int count = yylength();
addToken(zzStartRead,zzStartRead+1, Token.MARKUP_TAG_DELIMITER);
zzMarkedPos -= (count-2); //yypushback(count-2);
yybegin(INTAG_CHECK_TAG_NAME);
}
case 206: break;
case 61:
{ addToken(start,zzStartRead-1, Token.LITERAL_STRING_DOUBLE_QUOTE); addEndToken(INTERNAL_CSS_STRING - cssPrevState); return firstToken;
}
case 207: break;
case 59:
{ /* End of a function */ addToken(Token.SEPARATOR);
}
case 208: break;
case 19:
{ addToken(Token.MARKUP_TAG_NAME);
}
case 209: break;
case 11:
{ addToken(Token.MARKUP_TAG_ATTRIBUTE);
}
case 210: break;
case 78:
{ start = zzMarkedPos-2; yybegin(JS_EOL_COMMENT);
}
case 211: break;
case 38:
{ /* Line ending in '\' => continue to next line. */
if (validJSString) {
addToken(start,zzStartRead, Token.LITERAL_STRING_DOUBLE_QUOTE);
addEndToken(INTERNAL_IN_JS_STRING_VALID);
}
else {
addToken(start,zzStartRead, Token.ERROR_STRING_DOUBLE);
addEndToken(INTERNAL_IN_JS_STRING_INVALID);
}
return firstToken;
}
case 212: break;
case 81:
{ addToken(Token.LITERAL_NUMBER_FLOAT);
}
case 213: break;
case 16:
{ start = zzMarkedPos-1; yybegin(INATTR_SINGLE);
}
case 214: break;
case 95:
{ boolean highlightedAsRegex = false;
if (firstToken==null) {
addToken(Token.REGEX);
highlightedAsRegex = true;
}
else {
// If this is *likely* to be a regex, based on
// the previous token, highlight it as such.
Token t = firstToken.getLastNonCommentNonWhitespaceToken();
if (RSyntaxUtilities.regexCanFollowInJavaScript(t)) {
addToken(Token.REGEX);
highlightedAsRegex = true;
}
}
// If it doesn't *appear* to be a regex, highlight it as
// individual tokens.
if (!highlightedAsRegex) {
int temp = zzStartRead + 1;
addToken(zzStartRead, zzStartRead, Token.OPERATOR);
zzStartRead = zzCurrentPos = zzMarkedPos = temp;
}
}
case 215: break;
case 13:
{ addToken(Token.MARKUP_TAG_DELIMITER);
}
case 216: break;
case 66:
{ addToken(start,zzStartRead-1, Token.COMMENT_MULTILINE); addEndToken(INTERNAL_CSS_MLC - cssPrevState); return firstToken;
}
case 217: break;
case 32:
{ addToken(Token.LITERAL_NUMBER_DECIMAL_INT);
}
case 218: break;
case 74:
{ start = zzMarkedPos-2; yybegin(DTD);
}
case 219: break;
case 21:
{ start = zzMarkedPos-1; yybegin(INATTR_DOUBLE_SCRIPT);
}
case 220: break;
case 43:
{ addToken(start,zzStartRead-1, Token.COMMENT_EOL); addEndToken(INTERNAL_IN_JS); return firstToken;
}
case 221: break;
case 71:
{ // TODO: This isn't right. The expression and its depth should continue to the next line.
addToken(start,zzStartRead-1, Token.VARIABLE); addEndToken(INTERNAL_IN_JS_TEMPLATE_LITERAL_INVALID); return firstToken;
}
case 222: break;
case 48:
{ /* Unknown pseudo class */ addToken(Token.DATA_TYPE);
}
case 223: break;
case 1:
{
}
case 224: break;
default:
if (zzInput == YYEOF && zzStartRead == zzCurrentPos) {
zzAtEOF = true;
switch (zzLexicalState) {
case INATTR_SINGLE_SCRIPT: {
addToken(start,zzStartRead-1, Token.MARKUP_TAG_ATTRIBUTE_VALUE); addEndToken(INTERNAL_ATTR_SINGLE_QUOTE_SCRIPT); return firstToken;
}
case 820: break;
case JS_CHAR: {
addToken(start,zzStartRead-1, Token.ERROR_CHAR); addEndToken(INTERNAL_IN_JS); return firstToken;
}
case 821: break;
case CSS_STRING: {
addToken(start,zzStartRead-1, Token.LITERAL_STRING_DOUBLE_QUOTE); addEndToken(INTERNAL_CSS_STRING - cssPrevState); return firstToken;
}
case 822: break;
case JS_MLC: {
addToken(start,zzStartRead-1, Token.COMMENT_MULTILINE); addEndToken(INTERNAL_IN_JS_MLC); return firstToken;
}
case 823: break;
case CSS_CHAR_LITERAL: {
addToken(start,zzStartRead-1, Token.LITERAL_CHAR); addEndToken(INTERNAL_CSS_CHAR - cssPrevState); return firstToken;
}
case 824: break;
case INTAG_SCRIPT: {
addToken(zzMarkedPos,zzMarkedPos, INTERNAL_INTAG_SCRIPT); return firstToken;
}
case 825: break;
case JS_TEMPLATE_LITERAL_EXPR: {
// TODO: This isn't right. The expression and its depth should continue to the next line.
addToken(start,zzStartRead-1, Token.VARIABLE); addEndToken(INTERNAL_IN_JS_TEMPLATE_LITERAL_INVALID); return firstToken;
}
case 826: break;
case CSS_PROPERTY: {
addEndToken(INTERNAL_CSS_PROPERTY); return firstToken;
}
case 827: break;
case CSS_C_STYLE_COMMENT: {
addToken(start,zzStartRead-1, Token.COMMENT_MULTILINE); addEndToken(INTERNAL_CSS_MLC - cssPrevState); return firstToken;
}
case 828: break;
case CSS: {
addEndToken(INTERNAL_CSS); return firstToken;
}
case 829: break;
case CSS_VALUE: {
addEndToken(INTERNAL_CSS_VALUE); return firstToken;
}
case 830: break;
case COMMENT: {
addToken(start,zzStartRead-1, Token.MARKUP_COMMENT); return firstToken;
}
case 831: break;
case INATTR_DOUBLE_SCRIPT: {
addToken(start,zzStartRead-1, Token.MARKUP_TAG_ATTRIBUTE_VALUE); addEndToken(INTERNAL_ATTR_DOUBLE_QUOTE_SCRIPT); return firstToken;
}
case 832: break;
case PI: {
addToken(start,zzStartRead-1, Token.MARKUP_PROCESSING_INSTRUCTION); return firstToken;
}
case 833: break;
case JAVASCRIPT: {
addEndToken(INTERNAL_IN_JS); return firstToken;
}
case 834: break;
case INTAG: {
addToken(zzMarkedPos,zzMarkedPos, INTERNAL_INTAG); return firstToken;
}
case 835: break;
case INTAG_CHECK_TAG_NAME: {
addToken(zzMarkedPos,zzMarkedPos, INTERNAL_INTAG); return firstToken;
}
case 836: break;
case INATTR_SINGLE_STYLE: {
addToken(start,zzStartRead-1, Token.MARKUP_TAG_ATTRIBUTE_VALUE); addEndToken(INTERNAL_ATTR_SINGLE_QUOTE_STYLE); return firstToken;
}
case 837: break;
case DTD: {
addToken(start,zzStartRead-1, Token.MARKUP_DTD); return firstToken;
}
case 838: break;
case JS_EOL_COMMENT: {
addToken(start,zzStartRead-1, Token.COMMENT_EOL); addEndToken(INTERNAL_IN_JS); return firstToken;
}
case 839: break;
case INATTR_DOUBLE_STYLE: {
addToken(start,zzStartRead-1, Token.MARKUP_TAG_ATTRIBUTE_VALUE); addEndToken(INTERNAL_ATTR_DOUBLE_QUOTE_STYLE); return firstToken;
}
case 840: break;
case INATTR_SINGLE: {
addToken(start,zzStartRead-1, Token.MARKUP_TAG_ATTRIBUTE_VALUE); addEndToken(INTERNAL_ATTR_SINGLE); return firstToken;
}
case 841: break;
case JS_TEMPLATE_LITERAL: {
if (validJSString) {
addToken(start, zzStartRead - 1, Token.LITERAL_BACKQUOTE);
addEndToken(INTERNAL_IN_JS_TEMPLATE_LITERAL_VALID);
}
else {
addToken(start,zzStartRead - 1, Token.ERROR_STRING_DOUBLE);
addEndToken(INTERNAL_IN_JS_TEMPLATE_LITERAL_INVALID);
}
return firstToken;
}
case 842: break;
case YYINITIAL: {
addNullToken(); return firstToken;
}
case 843: break;
case INATTR_DOUBLE: {
addToken(start,zzStartRead-1, Token.MARKUP_TAG_ATTRIBUTE_VALUE); addEndToken(INTERNAL_ATTR_DOUBLE); return firstToken;
}
case 844: break;
case JS_STRING: {
addToken(start,zzStartRead-1, Token.ERROR_STRING_DOUBLE); addEndToken(INTERNAL_IN_JS); return firstToken;
}
case 845: break;
case INTAG_STYLE: {
addToken(zzMarkedPos,zzMarkedPos, INTERNAL_INTAG_STYLE); return firstToken;
}
case 846: break;
default:
return null;
}
}
else {
zzScanError(ZZ_NO_MATCH);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy