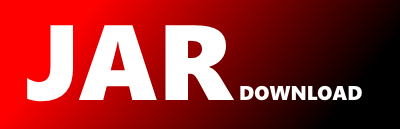
org.trustedanalytics.cloud.cc.api.CcOperationsServices Maven / Gradle / Ivy
/**
* Copyright (c) 2015 Intel Corporation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.trustedanalytics.cloud.cc.api;
import org.trustedanalytics.cloud.cc.api.queries.FilterQuery;
import rx.Observable;
import java.util.UUID;
public interface CcOperationsServices extends CcOperationsCommon {
/**
* Returns services within space identified by given GUID.
* @param spaceGuid GUID
* @return services
*/
Observable getServices(UUID spaceGuid);
/**
* Returns services within organization identified by given GUID
* @param orgGuid GUID
* @return services
*/
Observable getOrganizationServices(UUID orgGuid);
/**
* Returns services
* @return services
*/
Observable getExtendedServices();
/**
* @param filterQuery filter to use when requesting services
* @return filtered services
*/
Observable getExtendedServices(FilterQuery filterQuery);
/**
* Returns service plans within service identified by given GUID
* @return services plans
*/
Observable getExtendedServicePlans(UUID serviceGuid);
/**
* Get visibility of service plan to specific organization
* @param servicePlanGuid
* @return service plan identified by given service plan GUID
*/
Observable getExtendedServicePlan(UUID servicePlanGuid);
/**
* Returns service identified by given GUID.
* @param serviceGuid GUID
* @return service
*/
Observable getService(UUID serviceGuid);
/**
* Creates new service instance using provided configuration.
* @param serviceInstance service instance
* @return new service instance
*/
Observable createServiceInstance(CcNewServiceInstance serviceInstance);
/**
* @return all service instances
*/
Observable getExtendedServiceInstances();
/**
* @param filterQuery filter to use when requesting service instances
* @return filtered service instances
*/
Observable getExtendedServiceInstances(FilterQuery filterQuery);
/**
* @param depth how deep the relations should be resolved
* @return all service instances
*/
Observable getExtendedServiceInstances(int depth);
/**
* @param filterQuery filter to use when requesting service instances
* @param depth how deep the relations should be resolved
* @return filtered service instances
*/
Observable getExtendedServiceInstances(FilterQuery filterQuery, int depth);
/**
* @param instanceGuid GUID
* @return service instance identified by given GUID
*/
Observable getServiceInstance(UUID instanceGuid);
/**
* Deletes service instance identified by given GUID.
* @param instanceGuid GUID
*/
void deleteServiceInstance(UUID instanceGuid);
/**
* Returns list of service bindings matching provided filter
* @param filterQuery filter
* @return matching service bindings
*/
CcServiceBindingList getServiceBindings(FilterQuery filterQuery);
/**
* Returns a list of all service keys acessible to user
* @return list of service keys
*/
Observable getServiceKeys();
/**
* Creates a new service key
* @return Created service key
*/
Observable createServiceKey(CcNewServiceKey serviceKey);
/**
* Deletes given service key
*/
void deleteServiceKey(UUID keyGuid);
Observable getServicesCount();
/**
* Returns total number of services
* @return total number of services
*/
Observable getServiceInstancesCount();
/**
* Returns total number of service instances
* @return total number of service instances
*/
/**
* Set visibility of service plan to specific organization
* @param servicePlanGuid service plan GUID
* @param organizationGuid organization GUID
* @return services plan visibility
*/
Observable setExtendedServicePlanVisibility(UUID servicePlanGuid, UUID organizationGuid);
/**
* Get visibility of service plan to specific organization
* @param filterQuery filter
* @return services plan visibility
*/
Observable getExtendedServicePlanVisibility(FilterQuery filterQuery);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy