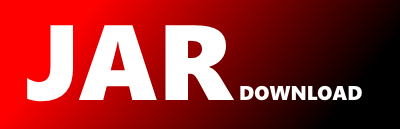
org.truth0.subjects.IterableSubject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truth Show documentation
Show all versions of truth Show documentation
Relocates org.truth0:truth to com.google.truth:truth.
See http://maven.apache.org/guides/mini/guide-relocation.html
/*
* Copyright (C) 2011 Google, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.truth0.subjects;
import com.google.common.annotations.GwtCompatible;
import org.truth0.FailureStrategy;
import java.util.Arrays;
import java.util.Iterator;
/**
* @author Kevin Bourrillion
*/
@GwtCompatible
public class IterableSubject, T, C extends Iterable> extends Subject {
@SuppressWarnings({ "unchecked", "rawtypes" })
public static > IterableSubject extends IterableSubject, T, C>, T, C> create(
FailureStrategy failureStrategy, Iterable list) {
return new IterableSubject(failureStrategy, list);
}
// TODO: Arguably this should even be package private
protected IterableSubject(FailureStrategy failureStrategy, C list) {
super(failureStrategy, list);
}
/**
* Attests that the subject holds no more objects, or fails.
*/
public void isEmpty() {
if (getSubject().iterator().hasNext()) {
fail("is empty");
}
}
/**
* Attests that the subject holds one or more objects, or fails
*/
public void isNotEmpty() {
if (!getSubject().iterator().hasNext()) {
fail("is not empty");
}
}
/**
* Asserts that the items are supplied in the order given by the iterable. If
* the iterable under test and/or the {@code expectedItems} do not provide
* iteration order guarantees (say, {@link Set}s), this method may provide
* unexpected results. Consider using {@link #is(T)} in such cases, or using
* collections and iterables that provide strong order guarantees.
*/
public void iteratesAs(Iterable expectedItems) {
Iterator actualItems = getSubject().iterator();
for (Object expected : expectedItems) {
if (!actualItems.hasNext()) {
fail("iterates through", expectedItems);
} else {
Object actual = actualItems.next();
if (actual == expected || actual != null && actual.equals(expected)) {
continue;
} else {
fail("iterates through", expectedItems);
}
}
}
if (actualItems.hasNext()) {
fail("iterates through", expectedItems);
}
}
/**
* @deprecated use {@link #iteratesAs(T...)}
*/
@Deprecated
public void iteratesOverSequence(T... expectedItems) {
iteratesAs(expectedItems);
}
/**
* Asserts that the items are supplied in the order given by the iterable. If
* the iterable under test does not provide iteration order guarantees (say,
* a {@link Set}), this method is not suitable for asserting that order.
* Consider using {@link #is(T)}
*/
public void iteratesAs(T... expectedItems) {
iteratesAs(Arrays.asList(expectedItems));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy