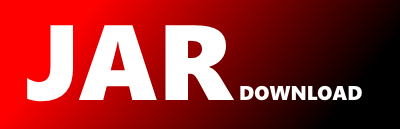
com.google.common.truth.CollectionSubject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truth Show documentation
Show all versions of truth Show documentation
Relocates org.truth0:truth to com.google.truth:truth.
See http://maven.apache.org/guides/mini/guide-relocation.html
The newest version!
/*
* Copyright (c) 2012 Google, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.common.truth;
import static com.google.common.truth.SubjectUtils.accumulate;
import static com.google.common.truth.SubjectUtils.countDuplicates;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import javax.annotation.CheckReturnValue;
public class CollectionSubject, T, C extends Collection>
extends IterableSubject {
@SuppressWarnings({ "unchecked", "rawtypes" })
public static > CollectionSubject extends CollectionSubject, T, C>, T, C> create(
FailureStrategy failureStrategy, Collection collection) {
return new CollectionSubject(failureStrategy, collection);
}
// TODO: Arguably this should even be package private
protected CollectionSubject(FailureStrategy failureStrategy, C collection) {
super(failureStrategy, collection);
}
/**
* Attests that a Collection is empty or fails.
*/
@Override public void isEmpty() {
if (!getSubject().isEmpty()) {
fail("is empty");
}
}
@CheckReturnValue
public Has has() {
return new Has() {
@Override public void item(T item) {
if (!getSubject().contains(item)) {
fail("has item", item);
}
}
@Override public void anyOf(T first) {
anyFrom(accumulate(first));
}
@Override public final void anyOf(T first, T second, T ... rest) {
anyFrom(accumulate(first, second, rest));
}
@Override public void anyFrom(Collection col) {
for (Object item : col) {
if (getSubject().contains(item)) {
return;
}
}
fail("contains", col);
}
@Override public Ordered allOf(T first) {
return allFrom(accumulate(first));
}
@Override public final Ordered allOf(T first, T second, T ... rest) {
return allFrom(accumulate(first, second, rest));
}
@Override public Ordered allFrom(final Collection required) {
Collection toRemove = new ArrayList(required);
// remove each item in the subject, as many times as it occurs in the subject.
for (Object item : getSubject()) {
toRemove.remove(item);
}
if (!toRemove.isEmpty()) {
failWithBadResults("has all of", required, "is missing", countDuplicates(toRemove));
}
return new InOrder("has all in order", required);
}
@Override public Ordered exactly(T first) {
return exactlyAs(accumulate(first));
}
@Override public final Ordered exactly(T first, T second, T ... rest) {
return exactlyAs(accumulate(first, second, rest));
}
@Override public Ordered exactlyAs(Collection required) {
Collection toRemove = new ArrayList(required);
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy