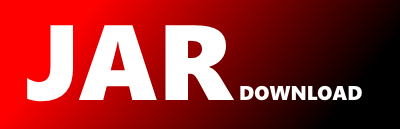
twitter4j.TwitterListener Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twitter4j-async Show documentation
Show all versions of twitter4j-async Show documentation
A Java library for the Twitter API
/*
* Copyright (C) 2007 Yusuke Yamamoto
* Copyright (C) 2011 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package twitter4j;
import twitter4j.api.HelpResources;
import twitter4j.auth.AccessToken;
import twitter4j.auth.OAuth2Token;
import twitter4j.auth.RequestToken;
import java.util.Map;
/**
* A listener for receiving asynchronous responses from Twitter Async APIs.
*
* @author Yusuke Yamamoto - yusuke at mac.com
*/
public interface TwitterListener {
/* Timelines Resources */
void gotMentions(ResponseList statuses);
void gotHomeTimeline(ResponseList statuses);
void gotUserTimeline(ResponseList statuses);
void gotRetweetsOfMe(ResponseList statuses);
/* Tweets Resources */
void gotRetweets(ResponseList retweets);
void gotShowStatus(Status status);
void destroyedStatus(Status destroyedStatus);
void updatedStatus(Status status);
void retweetedStatus(Status retweetedStatus);
void gotOEmbed(OEmbed oembed);
void lookedup(ResponseList statuses);
/* Search Resources */
void searched(QueryResult queryResult);
/* Direct Messages Resources */
void gotDirectMessages(ResponseList messages);
void gotSentDirectMessages(ResponseList messages);
void gotDirectMessage(DirectMessage message);
void destroyedDirectMessage(DirectMessage message);
void sentDirectMessage(DirectMessage message);
/* Friends & Followers Resources */
void gotFriendsIDs(IDs ids);
void gotFollowersIDs(IDs ids);
void lookedUpFriendships(ResponseList friendships);
void gotIncomingFriendships(IDs ids);
void gotOutgoingFriendships(IDs ids);
void createdFriendship(User user);
void destroyedFriendship(User user);
void updatedFriendship(Relationship relationship);
void gotShowFriendship(Relationship relationship);
void gotFriendsList(PagableResponseList users);
void gotFollowersList(PagableResponseList users);
/* Users Resources */
void gotAccountSettings(AccountSettings settings);
void verifiedCredentials(User user);
void updatedAccountSettings(AccountSettings settings);
// updatedDeliveryDevice
void updatedProfile(User user);
void updatedProfileBackgroundImage(User user);
void updatedProfileColors(User user);
void updatedProfileImage(User user);
void gotBlocksList(ResponseList blockingUsers);
void gotBlockIDs(IDs blockingUsersIDs);
void createdBlock(User user);
void destroyedBlock(User user);
void lookedupUsers(ResponseList users);
void gotUserDetail(User user);
void searchedUser(ResponseList userList);
void gotContributees(ResponseList users);
void gotContributors(ResponseList users);
void removedProfileBanner();
void updatedProfileBanner();
void gotMutesList(ResponseList blockingUsers);
void gotMuteIDs(IDs blockingUsersIDs);
void createdMute(User user);
void destroyedMute(User user);
/* Suggested Users Resources */
void gotUserSuggestions(ResponseList users);
void gotSuggestedUserCategories(ResponseList category);
void gotMemberSuggestions(ResponseList users);
/* Favorites Resources */
void gotFavorites(ResponseList statuses);
void createdFavorite(Status status);
void destroyedFavorite(Status status);
/* Lists Resources */
void gotUserLists(ResponseList userLists);
void gotUserListStatuses(ResponseList statuses);
void destroyedUserListMember(UserList userList);
void gotUserListMemberships(PagableResponseList userLists);
void gotUserListSubscribers(PagableResponseList users);
void subscribedUserList(UserList userList);
void checkedUserListSubscription(User user);
void unsubscribedUserList(UserList userList);
void createdUserListMembers(UserList userList);
void checkedUserListMembership(User users);
void createdUserListMember(UserList userList);
void destroyedUserList(UserList userList);
void updatedUserList(UserList userList);
void createdUserList(UserList userList);
void gotShowUserList(UserList userList);
void gotUserListSubscriptions(PagableResponseList userLists);
void gotUserListMembers(PagableResponseList users);
/* Saved Searches Resources */
void gotSavedSearches(ResponseList savedSearches);
void gotSavedSearch(SavedSearch savedSearch);
void createdSavedSearch(SavedSearch savedSearch);
void destroyedSavedSearch(SavedSearch savedSearch);
/* Places & Geo Resources */
void gotGeoDetails(Place place);
void gotReverseGeoCode(ResponseList places);
void searchedPlaces(ResponseList places);
void gotSimilarPlaces(ResponseList places);
/* Trends Resources */
void gotPlaceTrends(Trends trends);
void gotAvailableTrends(ResponseList locations);
void gotClosestTrends(ResponseList locations);
/* Spam Reporting Resources */
void reportedSpam(User reportedSpammer);
/* OAuth Resources */
void gotOAuthRequestToken(RequestToken token);
void gotOAuthAccessToken(AccessToken token);
/* OAuth2 Resources */
void gotOAuth2Token(OAuth2Token token);
/* Help Resources */
void gotAPIConfiguration(TwitterAPIConfiguration conf);
void gotLanguages(ResponseList languages);
void gotPrivacyPolicy(String privacyPolicy);
void gotTermsOfService(String tof);
void gotRateLimitStatus(Map rateLimitStatus);
void onException(TwitterException te, TwitterMethod method);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy