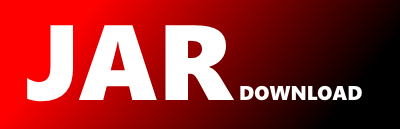
twitter4j.RelationshipJSONImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twitter4j-core Show documentation
Show all versions of twitter4j-core Show documentation
A Java library for the Twitter API
/*
* Copyright 2007 Yusuke Yamamoto
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package twitter4j;
import twitter4j.conf.Configuration;
/**
* A data class that has detailed information about a relationship between two users
*
* @author Perry Sakkaris - psakkaris at gmail.com
* @see GET friendships/show | Twitter Developers
* @since Twitter4J 2.1.0
*/
/*package*/ class RelationshipJSONImpl extends TwitterResponseImpl implements Relationship, java.io.Serializable {
private static final long serialVersionUID = -2001484553401916448L;
private final long targetUserId;
private final String targetUserScreenName;
private final boolean sourceBlockingTarget;
private final boolean sourceNotificationsEnabled;
private final boolean sourceFollowingTarget;
private final boolean sourceFollowedByTarget;
private final boolean sourceCanDm;
private final long sourceUserId;
private final String sourceUserScreenName;
private boolean wantRetweets;
/*package*/ RelationshipJSONImpl(HttpResponse res, Configuration conf) throws TwitterException {
this(res, res.asJSONObject());
if (conf.isJSONStoreEnabled()) {
TwitterObjectFactory.clearThreadLocalMap();
TwitterObjectFactory.registerJSONObject(this, res.asJSONObject());
}
}
/*package*/ RelationshipJSONImpl(JSONObject json) throws TwitterException {
this(null, json);
}
/*package*/ RelationshipJSONImpl(HttpResponse res, JSONObject json) throws TwitterException {
super(res);
try {
JSONObject relationship = json.getJSONObject("relationship");
JSONObject sourceJson = relationship.getJSONObject("source");
JSONObject targetJson = relationship.getJSONObject("target");
sourceUserId = ParseUtil.getLong("id", sourceJson);
targetUserId = ParseUtil.getLong("id", targetJson);
sourceUserScreenName = ParseUtil.getUnescapedString("screen_name", sourceJson);
targetUserScreenName = ParseUtil.getUnescapedString("screen_name", targetJson);
sourceBlockingTarget = ParseUtil.getBoolean("blocking", sourceJson);
sourceFollowingTarget = ParseUtil.getBoolean("following", sourceJson);
sourceFollowedByTarget = ParseUtil.getBoolean("followed_by", sourceJson);
sourceCanDm = ParseUtil.getBoolean("can_dm", sourceJson);
sourceNotificationsEnabled = ParseUtil.getBoolean("notifications_enabled", sourceJson);
wantRetweets = ParseUtil.getBoolean("want_retweets", sourceJson);
} catch (JSONException jsone) {
throw new TwitterException(jsone.getMessage() + ":" + json.toString(), jsone);
}
}
/*package*/
static ResponseList createRelationshipList(HttpResponse res, Configuration conf) throws TwitterException {
try {
if (conf.isJSONStoreEnabled()) {
TwitterObjectFactory.clearThreadLocalMap();
}
JSONArray list = res.asJSONArray();
int size = list.length();
ResponseList relationships = new ResponseListImpl(size, res);
for (int i = 0; i < size; i++) {
JSONObject json = list.getJSONObject(i);
Relationship relationship = new RelationshipJSONImpl(json);
if (conf.isJSONStoreEnabled()) {
TwitterObjectFactory.registerJSONObject(relationship, json);
}
relationships.add(relationship);
}
if (conf.isJSONStoreEnabled()) {
TwitterObjectFactory.registerJSONObject(relationships, list);
}
return relationships;
} catch (JSONException jsone) {
throw new TwitterException(jsone);
}
}
/**
* {@inheritDoc}
*/
@Override
public long getSourceUserId() {
return sourceUserId;
}
/**
* {@inheritDoc}
*/
@Override
public long getTargetUserId() {
return targetUserId;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isSourceBlockingTarget() {
return sourceBlockingTarget;
}
/**
* {@inheritDoc}
*/
@Override
public String getSourceUserScreenName() {
return sourceUserScreenName;
}
/**
* {@inheritDoc}
*/
@Override
public String getTargetUserScreenName() {
return targetUserScreenName;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isSourceFollowingTarget() {
return sourceFollowingTarget;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isTargetFollowingSource() {
return sourceFollowedByTarget;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isSourceFollowedByTarget() {
return sourceFollowedByTarget;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isTargetFollowedBySource() {
return sourceFollowingTarget;
}
/**
* {@inheritDoc}
*/
@Override
public boolean canSourceDm() {
return sourceCanDm;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isSourceNotificationsEnabled() {
return sourceNotificationsEnabled;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isSourceWantRetweets() {
return wantRetweets;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Relationship)) return false;
Relationship that = (Relationship) o;
if (sourceUserId != that.getSourceUserId()) return false;
if (targetUserId != that.getTargetUserId()) return false;
if (!sourceUserScreenName.equals(that.getSourceUserScreenName()))
return false;
if (!targetUserScreenName.equals(that.getTargetUserScreenName()))
return false;
return true;
}
@Override
public int hashCode() {
int result = (int) (targetUserId ^ (targetUserId >>> 32));
result = 31 * result + (targetUserScreenName != null ? targetUserScreenName.hashCode() : 0);
result = 31 * result + (sourceBlockingTarget ? 1 : 0);
result = 31 * result + (sourceNotificationsEnabled ? 1 : 0);
result = 31 * result + (sourceFollowingTarget ? 1 : 0);
result = 31 * result + (sourceFollowedByTarget ? 1 : 0);
result = 31 * result + (sourceCanDm ? 1 : 0);
result = 31 * result + (int) (sourceUserId ^ (sourceUserId >>> 32));
result = 31 * result + (sourceUserScreenName != null ? sourceUserScreenName.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "RelationshipJSONImpl{" +
"sourceUserId=" + sourceUserId +
", targetUserId=" + targetUserId +
", sourceUserScreenName='" + sourceUserScreenName + '\'' +
", targetUserScreenName='" + targetUserScreenName + '\'' +
", sourceFollowingTarget=" + sourceFollowingTarget +
", sourceFollowedByTarget=" + sourceFollowedByTarget +
", sourceCanDm=" + sourceCanDm +
", sourceNotificationsEnabled=" + sourceNotificationsEnabled +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy