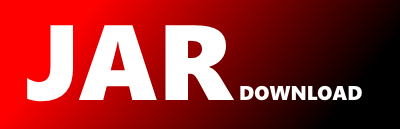
org.uberfire.ext.apps.client.home.AppsHomePresenter Maven / Gradle / Ivy
/*
* Copyright 2015 JBoss, by Red Hat, Inc
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.uberfire.ext.apps.client.home;
import java.util.List;
import javax.annotation.PostConstruct;
import javax.enterprise.context.ApplicationScoped;
import javax.inject.Inject;
import org.jboss.errai.common.client.api.Caller;
import org.jboss.errai.common.client.api.ErrorCallback;
import org.jboss.errai.common.client.api.RemoteCallback;
import org.uberfire.client.annotations.WorkbenchPartTitle;
import org.uberfire.client.annotations.WorkbenchPartView;
import org.uberfire.client.annotations.WorkbenchScreen;
import org.uberfire.client.mvp.PlaceManager;
import org.uberfire.client.mvp.UberView;
import org.uberfire.ext.apps.api.AppsPersistenceAPI;
import org.uberfire.ext.apps.api.Directory;
import org.uberfire.ext.apps.api.DirectoryBreadcrumb;
import org.uberfire.lifecycle.OnOpen;
import org.uberfire.mvp.ParameterizedCommand;
@ApplicationScoped
@WorkbenchScreen(identifier = "AppsHomePresenter")
public class AppsHomePresenter {
@Inject
private View view;
@Inject
private PlaceManager placeManager;
@Inject
private Caller appService;
private Directory currentDirectory;
private Directory root;
@PostConstruct
public void init() {
}
@OnOpen
public void loadContent() {
view.clear();
appService.call(new RemoteCallback() {
public void callback(Directory root_) {
root = root_;
currentDirectory = root_;
setupView();
}
},
new ErrorCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy