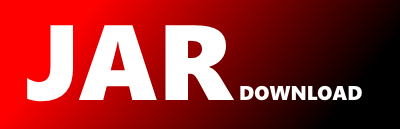
org.ujmp.jung.GraphMatrixWrapper Maven / Gradle / Ivy
/*
* Copyright (C) 2008-2015 by Holger Arndt
*
* This file is part of the Universal Java Matrix Package (UJMP).
* See the NOTICE file distributed with this work for additional
* information regarding copyright ownership and licensing.
*
* UJMP is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* UJMP is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with UJMP; if not, write to the
* Free Software Foundation, Inc., 51 Franklin St, Fifth Floor,
* Boston, MA 02110-1301 USA
*/
package org.ujmp.jung;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.ujmp.core.Matrix;
import org.ujmp.core.graphmatrix.GraphMatrix;
import edu.uci.ics.jung.graph.AbstractTypedGraph;
import edu.uci.ics.jung.graph.DirectedGraph;
import edu.uci.ics.jung.graph.util.EdgeType;
import edu.uci.ics.jung.graph.util.Pair;
public class GraphMatrixWrapper extends AbstractTypedGraph> implements
DirectedGraph> {
private static final long serialVersionUID = -3871581250021217530L;
private final GraphMatrix graphMatrix;
public GraphMatrixWrapper(GraphMatrix graphMatrix) {
super(EdgeType.DIRECTED);
this.graphMatrix = graphMatrix;
}
public Collection> getInEdges(V vertex) {
long childIndex = graphMatrix.getIndexOfNode(vertex);
List parentIndices = graphMatrix.getParentIndices(vertex);
List> edges = new ArrayList>();
for (long parentIndex : parentIndices) {
E edge = graphMatrix.getEdge(parentIndex, childIndex);
EdgeWrapper edgeWrapper = new EdgeWrapper(parentIndex, childIndex, edge);
edges.add(edgeWrapper);
}
return edges;
}
public Collection> getOutEdges(V vertex) {
long parentIndex = graphMatrix.getIndexOfNode(vertex);
List childIndices = graphMatrix.getChildIndices(vertex);
List> edges = new ArrayList>();
for (long childIndex : childIndices) {
E edge = graphMatrix.getEdge(parentIndex, childIndex);
EdgeWrapper edgeWrapper = new EdgeWrapper(parentIndex, childIndex, edge);
edges.add(edgeWrapper);
}
return edges;
}
public Collection getPredecessors(V vertex) {
return graphMatrix.getParents(vertex);
}
public Collection getSuccessors(V vertex) {
return graphMatrix.getChildren(vertex);
}
public V getSource(EdgeWrapper directed_edge) {
return graphMatrix.getNode(directed_edge.getCoordinates().getLongCoordinates()[Matrix.ROW]);
}
public V getDest(EdgeWrapper directed_edge) {
return graphMatrix.getNode(directed_edge.getCoordinates().getLongCoordinates()[Matrix.COLUMN]);
}
public boolean isSource(V vertex, EdgeWrapper edge) {
V source = getSource(edge);
return vertex == source;
}
public boolean isDest(V vertex, EdgeWrapper edge) {
V dest = getDest(edge);
return vertex == dest;
}
public Pair getEndpoints(EdgeWrapper edge) {
long[] c = edge.getCoordinates().getLongCoordinates();
return new Pair(graphMatrix.getNode(c[0]), graphMatrix.getNode(c[1]));
}
public Collection> getEdges() {
return new EdgeWrapperCollection(graphMatrix);
}
public Collection getVertices() {
return graphMatrix.getNodeList();
}
public boolean containsVertex(V vertex) {
return graphMatrix.getNodeList().contains(vertex);
}
public boolean containsEdge(EdgeWrapper edge) {
for (EdgeWrapper edgeWrapper : getEdges()) {
if (edgeWrapper.equals(edge)) {
return true;
}
}
return false;
}
public int getEdgeCount() {
return graphMatrix.getEdgeCount();
}
public int getVertexCount() {
return graphMatrix.getNodeCount();
}
public Collection getNeighbors(V vertex) {
List neighbors = new ArrayList();
neighbors.addAll(graphMatrix.getParents(vertex));
neighbors.addAll(graphMatrix.getChildren(vertex));
return neighbors;
}
public Collection> getIncidentEdges(V vertex) {
List> edges = new ArrayList>();
edges.addAll(getInEdges(vertex));
edges.addAll(getOutEdges(vertex));
return edges;
}
public boolean addVertex(V vertex) {
graphMatrix.addNode(vertex);
return true;
}
public boolean removeVertex(V vertex) {
graphMatrix.removeNode(vertex);
return true;
}
public boolean removeEdge(EdgeWrapper edge) {
long[] coordinates = edge.getCoordinates().getLongCoordinates();
graphMatrix.removeEdge(coordinates[Matrix.ROW], coordinates[Matrix.COLUMN]);
return true;
}
@Override
public boolean addEdge(EdgeWrapper edge, Pair extends V> endpoints, EdgeType edgeType) {
graphMatrix.setEdge(edge.getEdge(), endpoints.getFirst(), endpoints.getSecond());
if (edgeType == EdgeType.UNDIRECTED) {
graphMatrix.setEdge(edge.getEdge(), endpoints.getSecond(), endpoints.getFirst());
}
return true;
}
public GraphMatrix getGraphMatrix() {
return graphMatrix;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy