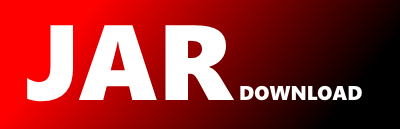
org.ujmp.jung.JungVisualizationViewer Maven / Gradle / Ivy
/*
* Copyright (C) 2008-2015 by Holger Arndt
*
* This file is part of the Universal Java Matrix Package (UJMP).
* See the NOTICE file distributed with this work for additional
* information regarding copyright ownership and licensing.
*
* UJMP is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* UJMP is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with UJMP; if not, write to the
* Free Software Foundation, Inc., 51 Franklin St, Fifth Floor,
* Boston, MA 02110-1301 USA
*/
package org.ujmp.jung;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.event.ComponentEvent;
import java.awt.event.ComponentListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import javax.swing.JPopupMenu;
import javax.swing.event.TableModelEvent;
import javax.swing.event.TableModelListener;
import org.apache.commons.collections15.Transformer;
import org.ujmp.core.graphmatrix.GraphMatrix;
import org.ujmp.gui.MatrixGUIObject;
import org.ujmp.gui.panels.AbstractPanel;
import org.ujmp.gui.util.UIDefaults;
import edu.uci.ics.jung.algorithms.layout.CircleLayout;
import edu.uci.ics.jung.algorithms.layout.FRLayout;
import edu.uci.ics.jung.algorithms.layout.FRLayout2;
import edu.uci.ics.jung.algorithms.layout.ISOMLayout;
import edu.uci.ics.jung.algorithms.layout.KKLayout;
import edu.uci.ics.jung.algorithms.layout.Layout;
import edu.uci.ics.jung.algorithms.layout.SpringLayout;
import edu.uci.ics.jung.algorithms.layout.SpringLayout2;
import edu.uci.ics.jung.graph.Graph;
import edu.uci.ics.jung.visualization.DefaultVisualizationModel;
import edu.uci.ics.jung.visualization.RenderContext;
import edu.uci.ics.jung.visualization.VisualizationModel;
import edu.uci.ics.jung.visualization.VisualizationViewer;
import edu.uci.ics.jung.visualization.control.DefaultModalGraphMouse;
import edu.uci.ics.jung.visualization.control.GraphMouseListener;
import edu.uci.ics.jung.visualization.control.ModalGraphMouse.Mode;
import edu.uci.ics.jung.visualization.decorators.ToStringLabeller;
import edu.uci.ics.jung.visualization.layout.LayoutTransition;
import edu.uci.ics.jung.visualization.renderers.BasicVertexLabelRenderer.InsidePositioner;
import edu.uci.ics.jung.visualization.renderers.DefaultEdgeLabelRenderer;
import edu.uci.ics.jung.visualization.renderers.DefaultVertexLabelRenderer;
import edu.uci.ics.jung.visualization.renderers.Renderer;
import edu.uci.ics.jung.visualization.util.Animator;
public class JungVisualizationViewer extends AbstractPanel implements MouseListener, TableModelListener,
ComponentListener, GraphMouseListener {
private static final long serialVersionUID = 7328433763448698033L;
public static enum GraphLayout {
CircleLayout, FRLayout, FRLayout2, ISOMLayout, KKLayout, SpringLayout, SpringLayout2
};
private final GraphMatrixWrapper graph;
private boolean showNodeLabels;
private boolean showEdgeLabels;
private final Transformer emptyNodeLabelTransformer;
private final Transformer, String> emptyEdgeLabelTransformer;
private final VisualizationViewer> vv;
private final Layout> layout;
private final GraphMatrix graphMatrix;
private final MatrixGUIObject matrixGUIObject;
public JungVisualizationViewer(GraphMatrixWrapper graph, boolean showNodeLabels, boolean showEdgeLabels) {
super(graph.getGraphMatrix().getGUIObject());
this.graph = graph;
this.graphMatrix = graph.getGraphMatrix();
this.matrixGUIObject = (MatrixGUIObject) graphMatrix.getGUIObject();
this.showNodeLabels = showNodeLabels;
this.showEdgeLabels = showEdgeLabels;
if (graph.getVertexCount() < 1000) {
layout = new FRLayout>(graph);
} else {
layout = new ISOMLayout>(graph);
}
VisualizationModel> visualizationModel = new DefaultVisualizationModel>(
layout);
vv = new VisualizationViewer>(visualizationModel);
vv.setForeground(new Color(0, 0, 0, 150));
vv.setBackground(Color.WHITE);
DefaultModalGraphMouse graphMouse = new DefaultModalGraphMouse();
vv.setGraphMouse(graphMouse);
graphMouse.setMode(Mode.PICKING);
RenderContext> rc = vv.getRenderContext();
emptyNodeLabelTransformer = rc.getVertexLabelTransformer();
emptyEdgeLabelTransformer = rc.getEdgeLabelTransformer();
rc.setVertexIconTransformer(new VertexIconTransformer(vv.getPickedVertexState()));
rc.setVertexFillPaintTransformer(new ColorTransformer(vv.getPickedVertexState()));
rc.setVertexLabelRenderer(new DefaultVertexLabelRenderer(UIDefaults.SELECTEDCOLOR));
rc.setEdgeDrawPaintTransformer(new ColorTransformer>(vv.getPickedEdgeState()));
rc.setEdgeLabelRenderer(new DefaultEdgeLabelRenderer(UIDefaults.SELECTEDCOLOR));
rc.setArrowFillPaintTransformer(new ColorTransformer>(vv.getPickedEdgeState()));
rc.setArrowDrawPaintTransformer(new ColorTransformer>(vv.getPickedEdgeState()));
vv.getRenderer().getVertexLabelRenderer().setPositioner(new InsidePositioner());
vv.getRenderer().getVertexLabelRenderer().setPosition(Renderer.VertexLabel.Position.AUTO);
if (showNodeLabels) {
rc.setVertexLabelTransformer(new ToStringLabeller());
}
if (showEdgeLabels) {
rc.setEdgeLabelTransformer(new ToStringLabeller>());
}
vv.setVertexToolTipTransformer(new ToStringLabeller());
setLayout(new BorderLayout());
add(vv, BorderLayout.CENTER);
vv.addMouseListener(this);
addComponentListener(this);
vv.addGraphMouseListener(this);
if (graph instanceof GraphMatrixWrapper) {
((MatrixGUIObject) ((GraphMatrixWrapper) graph).getGraphMatrix().getGUIObject())
.addTableModelListener(this);
}
}
public JungVisualizationViewer(GraphMatrixWrapper graph) {
this(graph, graph.getVertexCount() < 100, graph.getVertexCount() < 100);
}
public JungVisualizationViewer(GraphMatrix graphMatrix) {
this(new GraphMatrixWrapper(graphMatrix));
}
public JungVisualizationViewer(GraphMatrix graphMatrix, boolean showNodeLabels, boolean showEdgeLabels) {
this(new GraphMatrixWrapper(graphMatrix), showNodeLabels, showEdgeLabels);
}
public void switchLayout(GraphLayout type) {
Layout> layout = null;
switch (type) {
case KKLayout:
layout = new KKLayout>(graph);
break;
case FRLayout:
layout = new FRLayout>(graph);
break;
case ISOMLayout:
layout = new ISOMLayout>(graph);
break;
case SpringLayout:
layout = new SpringLayout>(graph);
break;
case CircleLayout:
layout = new CircleLayout>(graph);
break;
case FRLayout2:
layout = new FRLayout2>(graph);
break;
case SpringLayout2:
layout = new SpringLayout2>(graph);
break;
default:
break;
}
if (graph.getVertexCount() < 100) {
layout.setInitializer(vv.getGraphLayout());
layout.setSize(getSize());
LayoutTransition> lt = new LayoutTransition>(vv, vv.getGraphLayout(),
layout);
Animator animator = new Animator(lt);
animator.start();
vv.getRenderContext().getMultiLayerTransformer().setToIdentity();
repaint(500);
} else {
vv.setModel(new DefaultVisualizationModel>(layout));
repaint(500);
}
}
public final void mouseClicked(MouseEvent e) {
switch (e.getButton()) {
case MouseEvent.BUTTON1:
break;
case MouseEvent.BUTTON2:
break;
case MouseEvent.BUTTON3:
JPopupMenu popup = null;
popup = new JungGraphActions(this);
popup.show(e.getComponent(), e.getX(), e.getY());
break;
}
}
public void refreshUI() {
vv.getGraphLayout().setSize(getSize());
vv.setModel(new DefaultVisualizationModel>(vv.getGraphLayout()));
repaint(500);
}
public void mousePressed(MouseEvent e) {
}
public void mouseReleased(MouseEvent e) {
}
public void mouseEntered(MouseEvent e) {
}
public void mouseExited(MouseEvent e) {
}
public boolean isShowNodeLabels() {
return showNodeLabels;
}
public void setShowNodeLabels(boolean showNodeLabels) {
this.showNodeLabels = showNodeLabels;
if (showNodeLabels) {
vv.getRenderContext().setVertexLabelTransformer(new ToStringLabeller());
} else {
vv.getRenderContext().setVertexLabelTransformer(emptyNodeLabelTransformer);
}
repaint(500);
}
public boolean isShowEdgeLabels() {
return showEdgeLabels;
}
public void setShowEdgeLabels(boolean showEdgeLabels) {
this.showEdgeLabels = showEdgeLabels;
if (showEdgeLabels) {
vv.getRenderContext().setEdgeLabelTransformer(new ToStringLabeller>());
} else {
vv.getRenderContext().setEdgeLabelTransformer(emptyEdgeLabelTransformer);
}
repaint(500);
}
public Graph> getGraph() {
return graph;
}
public void tableChanged(TableModelEvent e) {
repaint(500);
}
public void componentResized(ComponentEvent e) {
vv.getGraphLayout().setSize(getSize());
vv.setModel(new DefaultVisualizationModel>(vv.getGraphLayout()));
repaint(500);
}
public void componentMoved(ComponentEvent e) {
}
public void componentShown(ComponentEvent e) {
}
public void componentHidden(ComponentEvent e) {
}
public void graphClicked(N v, MouseEvent me) {
long index = graphMatrix.getIndexOfNode(v);
matrixGUIObject.getRowSelectionModel().setSelectionInterval(index, index);
matrixGUIObject.getColumnSelectionModel().setSelectionInterval(index, index);
}
public void graphPressed(N v, MouseEvent me) {
long index = graphMatrix.getIndexOfNode(v);
matrixGUIObject.getRowSelectionModel().setSelectionInterval(index, index);
matrixGUIObject.getColumnSelectionModel().setSelectionInterval(index, index);
}
public void graphReleased(N v, MouseEvent me) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy