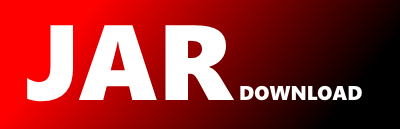
org.ujorm.tools.common.StreamUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ujo-tools Show documentation
Show all versions of ujo-tools Show documentation
Ujorm-tools module is a basic library tools for common use
The newest version!
/*
* Copyright 2012-2012 Pavel Ponec
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ujorm.tools.common;
import java.io.BufferedReader;
import java.io.CharArrayReader;
import java.io.IOException;
import java.net.URL;
import java.util.ArrayDeque;
import java.util.Collections;
import java.util.EnumSet;
import java.util.Iterator;
import java.util.Set;
import java.util.function.Function;
import java.util.stream.Collector;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import org.jetbrains.annotations.NotNull;
/**
* Static methods
* @author Pavel Ponec
*/
public abstract class StreamUtils {
static final Set CH_ID
= Collections.unmodifiableSet(EnumSet.of(Collector.Characteristics.IDENTITY_FINISH));
private StreamUtils() {
}
/** Read a String.
* A line separator can be modifed in the result
* @return The result must be closed.
*/
@NotNull
public Stream rows(@NotNull final String text) {
return new BufferedReader(new CharArrayReader(text.toCharArray())).lines();
}
/** Returns a stream of lines form URL resource
*
* @param url An URL link to a resource
* @return The customer is responsible for closing the stream.
* During closing, an IllegalStateException may occur due to an IOException.
*/
public static Stream rowsOfUrl(@NotNull final URL url) throws IOException {
return StringUtils.readLines(url);
}
/**
* Convert an interator to a Stream
* @param An item type
* @param iterator Source iterator
* @return
*/
public static Stream toStream(@NotNull final Iterator iterator) {
return toStream(iterator, false);
}
/**
* Convert an interator to a Stream
* @param An item type
* @param iterator Source iterator
* @param parallel Parrallell processing is enabled
* @return
*/
public static Stream toStream(@NotNull final Iterator iterator, final boolean parallel) {
final Iterable iterable = () -> iterator;
return StreamSupport.stream(iterable.spliterator(), parallel);
// # For Java 9:
//
//Stream.generate(() -> null)
// .takeWhile(x -> iterator.hasNext())
// .map(n -> iterator.next());
}
/** A stream collecetor to a ArrayDeque type */
@NotNull
public static Collector> collectToDequeue() {
return Collectors.toCollection(ArrayDeque::new);
}
/** Create a joinable function
*
* Usage
*
* Function<Person, String> nameProvider = Joinable
* .of (Person::getBoss)
* .add(Person::getBoss)
* .add(Person::getName);
* String superBossName = nameProvider.apply(getPerson());
*
*
* @param Domain value
* @param Result value
* @param fce An original function
* @return The new object type of Function
*/
@NotNull
public static Joinable toJoinable(@NotNull final Function fce) {
return Joinable.of(fce);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy