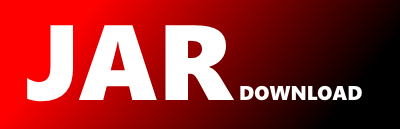
org.umlg.runtime.collection.ocl.OclStdLibCollection Maven / Gradle / Ivy
package org.umlg.runtime.collection.ocl;
import org.umlg.runtime.collection.*;
import org.umlg.runtime.domain.UmlgEnum;
import org.umlg.runtime.domain.ocl.OclAny;
import java.util.Comparator;
/**
* From ocl spec v2.3.1
*/
public interface OclStdLibCollection extends OclAny, Iterable {
default String toJson() {
StringBuilder sb = new StringBuilder();
sb.append("[");
int count = 0;
for (E e : this) {
count++;
if (e instanceof String) {
sb.append("\"");
sb.append(e);
sb.append("\"");
} else if (e instanceof UmlgEnum) {
sb.append("\"");
sb.append(e);
sb.append("\"");
} else {
sb.append(e);
}
if (count < size()) {
sb.append(",");
}
}
sb.append("]");
return sb.toString();
}
/**
* = (c : Collection(T)) : Boolean
*
* True if c is a collection of the same kind as self and contains the same
* elements in the same quantities and in the same order, in the case of an
* ordered collection type.
*/
boolean equals(UmlgCollection c);
/**
* <> (c : Collection(T)) : Boolean
*
* True if c is not equal to self.
* post: result = not (self = c)
*
*/
boolean notEquals(UmlgCollection c);
/**
* size() : Integer
*
* The number of elements in the collection self.
* post: result = self->iterate(elem; acc : Integer = 0 | acc + 1)
*
*
* @return int
*/
int size();
/**
* includes(object : T) : Boolean
*
* True if object is an element of self, false otherwise.
* post: result = (self->count(object) > 0)
*
*/
boolean includes(E t);
/**
* excludes(object : T) : Boolean
*
* True if object is not an element of self, false otherwise.
* post: result = (self->count(object) = 0)
*
*/
boolean excludes(E t);
/**
* count(object : T) : Integer
*
* The number of times that object occurs in the collection self.
* post: result = self->iterate( elem; acc : Integer = 0 |
* if elem = object then acc + 1 else acc endif)
*
*/
int count(E e);
/**
* includesAll(c2 : Collection(T)) : Boolean
*
* Does self contain all the elements of c2 ?
* post: result = c2->forAll(elem | self->includes(elem))
*
*
* @param c
* @return
*/
Boolean includesAll(UmlgCollection c);
/**
* excludesAll(c2 : Collection(T)) : Boolean
*
* Does self contain none of the elements of c2 ?
* post: result = c2->forAll(elem | self->excludes(elem))
*
*
* @param c
* @return
*/
Boolean excludesAll(UmlgCollection c);
/**
* isEmpty() : Boolean
*
* Is self the empty collection?
* post: result = ( self->size() = 0 )
* Note: null->isEmpty() returns 'true' in virtue of the implicit casting from null to Bag{}
*
*
* @return
*/
boolean isEmpty();
/**
* notEmpty() : Boolean
*
* Is self not the empty collection?
* post: result = ( self->size() <> 0 )
* null->notEmpty() returns 'false' in virtue of the implicit casting from null to Bag{}.
*
*
* @return
*/
Boolean notEmpty();
/**
* max() : T
*
* The element with the maximum value of all elements in self. Elements must be of a type supporting the max operation.
* The max operation - supported by the elements - must take one parameter of type T and be both associative and
* commutative. UnlimitedNatural, Integer and Real fulfill this condition.
* post: result = self->iterate( elem; acc : T = self.first() | acc.max(elem) )
*
*
* @return
*/
E max();
/**
* min() : T
*
* The element with the minimum value of all elements in self. Elements must be of a type supporting the min operation.
* The min operation - supported by the elements - must take one parameter of type T and be both associative and
* commutative. UnlimitedNatural, Integer and Real fulfill this condition.
* post: result = self->iterate( elem; acc : T = self.first() | acc.min(elem) )
*
*
* @return
*/
E min();
/**
* sum() : T
*
* The addition of all elements in self. Elements must be of a type supporting the + operation. The + operation must take one
* parameter of type T and be both associative: (a+b)+c = a+(b+c), and commutative: a+b = b+a. UnlimitedNatural, Integer
* and Real fulfill this condition.
* post: result = self->iterate( elem; acc : T = 0 | acc + elem )
* If the + operation is not both associative and commutative, the sum expression is not well-formed, which may result in
* unpredictable results during evaluation. If an implementation is able to detect a lack of associativity or commutativity, the
* implementation may bypass the evaluation and return an invalid result.
*
*
* @return
*/
E sum();
/**
* product(c2: Collection(T2)) : Set( Tuple( first: T, second: T2) )
*
* The cartesian product operation of self and c2.
* post: result = self->iterate (e1; acc: Set(Tuple(first: T, second: T2)) = Set{} |
* c2->iterate (e2; acc2: Set(Tuple(first: T, second: T2)) = acc |
* acc2->including (Tuple{first = e1, second = e2}) ) )
*
*
* @return
*/
// TODO support Tuples
UmlgSet> product(UmlgCollection c);
/**
* asSet() : Set(T)
*
* The Set containing all the elements from self, with duplicates removed.
* post: result->forAll(elem | self ->includes(elem))
* post: self ->forAll(elem | result->includes(elem))
*
*
* @return
*/
UmlgSet asSet();
/**
* asOrderedSet() : OrderedSet(T)
*
* An OrderedSet that contains all the elements from self, with duplicates removed, in an order dependent on the particular
* concrete collection type.
* post: result->forAll(elem | self->includes(elem))
* post: self ->forAll(elem | result->includes(elem))
*
*/
UmlgOrderedSet asOrderedSet();
/**
* asSequence() : Sequence(T)
*
* A Sequence that contains all the elements from self, in an order dependent on the particular concrete collection type.
* post: result->forAll(elem | self->includes(elem))
* post: self ->forAll(elem | result->includes(elem))
*
*/
UmlgSequence asSequence();
/**
* asBag() : Bag(T)
*
* The Bag that contains all the elements from self.
* post: result->forAll(elem | self->includes(elem))
* post: self ->forAll(elem | result->includes(elem))
*
*/
UmlgBag asBag();
/***************************************************
* Iterate goodies
***************************************************/
R iterate(IterateExpressionAccumulator v);
/**
* The subset of set for which expr is true. source->select(iterator | body)
* = source->iterate(iterator; result : Set(T) = Set{} | if body then
* result->including(iterator) else result endif) select may have at most
* one iterator variable.
*/
UmlgCollection select(BooleanExpressionEvaluator e);
/**
* Returns any element in the source collection for which body evaluates to
* true. If there is more than one element for which body is true, one of
* them is returned. There must be at least one element fulfilling body,
* otherwise the result of this IteratorExp is null.
*
* source->any(iterator | body) = source->select(iterator |
* body)->asSequence()->first()
*
* any may have at most one iterator variable.
*
* @param e
* @return
*/
E any(BooleanExpressionEvaluator e);
/**
* Results in invalid if if body evaluates to invalid for any element in the source collection,
* otherwise true if body evaluates to a different, possibly null, value for each element in the source collection;
* otherwise result is false.
*
* source->isUnique (iterator | body) =
* source->collect (iterator | Tuple{iter = Tuple{iterator}, value = body})
* ->forAll (x, y | (x.iter <> y.iter) implies (x.value <> y.value))
*
* isUnique may have at most one iterator variable.
*/
Boolean isUnique(BodyExpressionEvaluator e);
Boolean exists(BooleanExpressionEvaluator e);
Boolean forAll(BooleanExpressionEvaluator e);
/**
* The Collection of elements that results from applying body to every
* member of the source set. The result is flattened. Notice that this is
* based on collectNested, which can be of different type depending on the
* type of source. collectNested is defined individually for each subclass
* of CollectionType.
*
* source->collect (iterator | body) = source->collectNested (iterator |
* body)->flatten()
*
* collect may have at most one iterator variable.
*
* @param e
* @return
*/
UmlgCollection collect(BodyExpressionEvaluator e);
/**
* The Bag of elements which results from applying body to every member of
* the source set. source->collectNested(iterator | body) =
* source->iterate(iterator; result : Bag(body.type) = Bag{} |
* result->including(body ) )
*
* collectNested may have at most one iterator variable.
*
* @param e
* @return
*/
UmlgCollection collectNested(BodyExpressionEvaluator e);
/**
* flatten() : Collection(T2)
*
* If the element type is not a collection type, this results in the same collection as self. If the element type is a collection
* type, the result is a collection containing all the elements of all the recursively flattened elements of self.
* [1] Well-formedness rules
* [2] [1] A collection cannot contain invalid values.
* context Collection
* inv: self->forAll(not oclIsInvalid())
*
*
* @return
*/
UmlgCollection flatten();
//Predefined Iterator Expressions
UmlgCollection sortedBy(Comparator e);
}