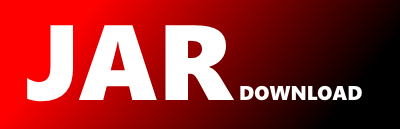
org.umlg.runtime.collection.ocl.OclStdLibSet Maven / Gradle / Ivy
package org.umlg.runtime.collection.ocl;
import org.umlg.runtime.collection.*;
import java.util.Comparator;
public interface OclStdLibSet extends OclStdLibCollection {
/**
* union(s : Set(T)) : Set(T)
*
*
* The union of self and s.
* post: result->forAll(elem | self->includes(elem) or s->includes(elem))
* post: self ->forAll(elem | result->includes(elem))
* post: s ->forAll(elem | result->includes(elem))
*
*
*/
UmlgSet union(UmlgSet extends E> s);
/**
* union(bag : Bag(T)) : Bag(T)
*
*
* The union of self and bag.
* post: result->forAll(elem | result->count(elem) = self->count(elem) + bag->count(elem))
* post: self->forAll(elem | result->includes(elem))
* post: bag ->forAll(elem | result->includes(elem))
*
*/
UmlgBag union(UmlgBag extends E> bag);
/**
* = (s : Set(T)) : Boolean
*
*
* Evaluates to true if self and s contain the same elements.
* post: result = (self->forAll(elem | s->includes(elem)) and
* s->forAll(elem | self->includes(elem)) )
*
*
*/
Boolean equals(UmlgSet s);
/**
* intersection(s : Set(T)) : Set(T)
*
*
* The intersection of self and s (i.e., the set of all elements that are in both self and s).
* post: result->forAll(elem | self->includes(elem) and s->includes(elem))
* post: self->forAll(elem | s ->includes(elem) = result->includes(elem))
* post: s ->forAll(elem | self->includes(elem) = result->includes(elem))
*
*
*/
UmlgSet intersection(UmlgSet s);
/**
* intersection(bag : Bag(T)) : Set(T)
*
*
* The intersection of self and bag.
* post: result = self->intersection( bag->asSet )
*
*/
UmlgSet intersection(UmlgBag bag);
/**
* – (s : Set(T)) : Set(T)
*
*
* The elements of self, which are not in s.
* post: result->forAll(elem | self->includes(elem) and s->excludes(elem))
*
*/
UmlgSet subtract(UmlgSet s);
/**
* including(object : T) : Set(T)
*
*
* The set containing all elements of self plus object.
* post: result->forAll(elem | self->includes(elem) or (elem = object))
* post: self- >forAll(elem | result->includes(elem)) post:
* result->includes(object)
*
*/
UmlgSet including(E e);
/**
* excluding(object : T) : Set(T)
*
*
* The set containing all elements of self without object.
* post:result->forAll(elem | self->includes(elem) and (elem <> object))
* post:self- >forAll(elem | result->includes(elem) = (object <> elem))
* post:result->excludes(object)
*
*/
UmlgSet excluding(E e);
/**
* symmetricDifference(s : Set(T)) : Set(T)
*
*
* The sets containing all the elements that are in self or s, but not in both.
* post: result->forAll(elem | self->includes(elem) xor s->includes(elem))
* post: self->forAll(elem | result->includes(elem) = s ->excludes(elem))
* post: s ->forAll(elem | result->includes(elem) = self->excludes(elem))
*
*
*/
UmlgSet symmetricDifference(UmlgSet s);
/**
* count(object : T) : Integer
*
*
* The number of occurrences of object in self.
* post: result <= 1
*
*/
int count(E e);
// /**
// * asSet() : Set(T)
// *
// *
// * Redefines the Collection operation. A Set identical to self. This operation exists for convenience reasons.
// * post: result = self
// *
// */
// @Override
// UmlgSet asSet();
/**
* asOrderedSet() : OrderedSet(T)
*
*
* Redefines the Collection operation. An OrderedSet that contains all the elements from self, in undefined order.
* post: result->forAll(elem | self->includes(elem))
*
*/
@Override
UmlgOrderedSet asOrderedSet();
/**
* asSequence() : Sequence(T)
*
*
* Redefines the Collection operation. A Sequence that contains all the elements from self, in undefined order.
* post: result->forAll(elem | self->includes(elem))
* post: self->forAll(elem | result->count(elem) = 1)
*
*/
@Override
UmlgSequence asSequence();
/**
* asBag() : Bag(T)
*
* Redefines the Collection operation. The Bag that contains all the elements from self.
* post: result->forAll(elem | self->includes(elem))
* post: self->forAll(elem | result->count(elem) = 1)
*
*/
@Override
UmlgBag asBag();
/***************************************************
* Iterate goodies
***************************************************/
@Override
UmlgSet select(BooleanExpressionEvaluator e);
@Override
UmlgBag collectNested(BodyExpressionEvaluator e);
@Override
UmlgBag collect(BodyExpressionEvaluator e);
/**
* flatten() : Set(T2)
*
*
* Redefines the Collection operation. If the element type is not a collection type, this results in the same set as self. If the
* element type is a collection type, the result is the set containing all the elements of all the recursively flattened elements
* of self.
* post: result = if self.oclType().elementType.oclIsKindOf(CollectionType) then
* self->iterate(c; acc : Set(T2) = Set{} |
* acc->union(c->flatten()->asSet() ) )
* else
* self
* endif
*
*/
@Override
UmlgSet flatten();
//Predefined Iterator Expressions
@Override
UmlgOrderedSet sortedBy(Comparator e);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy