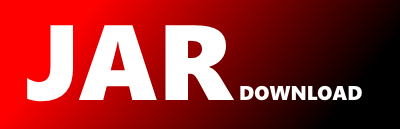
org.umlg.sqlg.test.gremlincompile.TestTraversalPerformance Maven / Gradle / Ivy
package org.umlg.sqlg.test.gremlincompile;
import org.apache.commons.lang3.time.StopWatch;
import org.junit.Assert;
import org.junit.Test;
import org.umlg.sqlg.structure.PropertyType;
import org.umlg.sqlg.structure.SqlgVertex;
import org.umlg.sqlg.structure.topology.VertexLabel;
import org.umlg.sqlg.test.BaseTest;
import java.util.HashMap;
import java.util.Map;
/**
* Date: 2015/02/01
* Time: 11:48 AM
*/
public class TestTraversalPerformance extends BaseTest {
@Test
public void testSpeedWithLargeSchemaFastQuery1() {
StopWatch stopWatch = new StopWatch();
stopWatch.start();
Map columns = new HashMap<>();
for (int i = 0; i < 100; i++) {
columns.put("property_" + i, PropertyType.STRING);
}
//Create a large schema, it slows the maps down
int NUMBER_OF_SCHEMA_ELEMENTS = 1_000;
for (int i = 0; i < NUMBER_OF_SCHEMA_ELEMENTS; i++) {
VertexLabel person = this.sqlgGraph.getTopology().ensureVertexLabelExist("Person_" + i, columns);
VertexLabel dog = this.sqlgGraph.getTopology().ensureVertexLabelExist("Dog_" + i, columns);
person.ensureEdgeLabelExist("pet_" + i, dog, columns);
if (i % 100 == 0) {
this.sqlgGraph.tx().commit();
}
}
this.sqlgGraph.tx().commit();
stopWatch.stop();
System.out.println("done creating schema time taken " + stopWatch.toString());
stopWatch.reset();
stopWatch.start();
Map columnValues = new HashMap<>();
for (int i = 0; i < 100; i++) {
columnValues.put("property_" + i, "asdasdasd");
}
for (int i = 0; i < NUMBER_OF_SCHEMA_ELEMENTS; i++) {
SqlgVertex person = (SqlgVertex) this.sqlgGraph.addVertex("Person_" + i, columnValues);
SqlgVertex dog = (SqlgVertex) this.sqlgGraph.addVertex("Dog_" + i, columnValues);
person.addEdgeWithMap("pet_" + i, dog, columnValues);
}
this.sqlgGraph.tx().commit();
stopWatch.stop();
System.out.println("done inserting data time taken " + stopWatch.toString());
stopWatch.reset();
stopWatch.start();
for (int i = 0; i < 10_000; i++) {
Assert.assertEquals(1, this.sqlgGraph.traversal().V().hasLabel("Person_0").out("pet_0").toList().size());
}
stopWatch.stop();
System.out.println("total query time " + stopWatch.toString());
}
//// @Test
// public void testSpeedWithLargeSchemaFastQuery() {
// StopWatch stopWatch = new StopWatch();
// stopWatch.start();
// Map columns = new HashMap<>();
// for (int i = 0; i < 100; i++) {
// columns.put("property_" + i, PropertyType.STRING);
// }
// //Create a large schema, it slows the maps down
// for (int i = 0; i < 1_000; i++) {
// VertexLabel person = this.sqlgGraph.getTopology().ensureVertexLabelExist("Person_" + i, columns);
// VertexLabel dog = this.sqlgGraph.getTopology().ensureVertexLabelExist("Dog_" + i, columns);
// person.ensureEdgeLabelExist("pet_" + i, dog, columns);
// if (i % 100 == 0) {
// this.sqlgGraph.tx().commit();
// }
// }
// this.sqlgGraph.tx().commit();
// stopWatch.stop();
// System.out.println("done time taken " + stopWatch.toString());
// stopWatch.reset();
// stopWatch.start();
//
// Map columnValues = new HashMap<>();
// for (int i = 0; i < 100; i++) {
// columnValues.put("property_" + i, "asdasdasd");
// }
// for (int i = 0; i < 1_000; i++) {
// SqlgVertex person = (SqlgVertex) this.sqlgGraph.addVertex("Person_" + i, columnValues);
// SqlgVertex dog = (SqlgVertex) this.sqlgGraph.addVertex("Dog_" + i, columnValues);
// person.addEdgeWithMap("pet_" + i, dog, columnValues);
// }
// this.sqlgGraph.tx().commit();
//
// for (int i = 0; i < 100_000; i++) {
// Assert.assertEquals(1, this.sqlgGraph.traversal().V().hasLabel("Person_0").out("pet_0").toList().size());
// stopWatch.stop();
// System.out.println("query time " + stopWatch.toString());
// stopWatch.reset();
// stopWatch.start();
// }
// stopWatch.stop();
// System.out.println("query time " + stopWatch.toString());
//
// }
//
//// @Test
// public void testSpeed() throws InterruptedException {
// StopWatch stopWatch = new StopWatch();
// stopWatch.start();
// Map columns = new HashMap<>();
// for (int i = 0; i < 100; i++) {
// columns.put("property_" + i, "asdasd");
// }
// //Create a large schema, it slows the maps down
// this.sqlgGraph.tx().normalBatchModeOn();
// for (int i = 0; i < 100; i++) {
// if (i % 100 == 0) {
// stopWatch.stop();
// System.out.println("got " + i + " time taken " + stopWatch.toString());
// stopWatch.reset();
// stopWatch.start();
// }
// Vertex person = this.sqlgGraph.addVertex("Person_" + i, columns);
// Vertex dog = this.sqlgGraph.addVertex("Dog_" + i, columns);
// ((SqlgVertex)person).addEdgeWithMap("pet_" + i, dog, columns);
// this.sqlgGraph.tx().commit();
// }
// this.sqlgGraph.tx().commit();
// stopWatch.stop();
// System.out.println("done time taken " + stopWatch.toString());
// stopWatch.reset();
// stopWatch.start();
//
// Thread.sleep(5_000);
//
// Map properties = new HashMap() {{
// put("name", PropertyType.STRING);
// }};
// VertexLabel godVertexLabel = this.sqlgGraph.getTopology().getPublicSchema().ensureVertexLabelExist("God", properties);
// VertexLabel handVertexLabel = this.sqlgGraph.getTopology().getPublicSchema().ensureVertexLabelExist("Hand", properties);
// VertexLabel fingerVertexLabel = this.sqlgGraph.getTopology().getPublicSchema().ensureVertexLabelExist("Finger", properties);
// godVertexLabel.ensureEdgeLabelExist("hand", handVertexLabel);
// handVertexLabel.ensureEdgeLabelExist("finger", fingerVertexLabel);
// this.sqlgGraph.tx().commit();
//
// stopWatch.stop();
// System.out.println("done another time taken " + stopWatch.toString());
// stopWatch.reset();
// stopWatch.start();
//
// StopWatch stopWatchAddOnly = new StopWatch();
// stopWatchAddOnly.start();
//
// this.sqlgGraph.tx().normalBatchModeOn();
// for (int i = 1; i < 1_00_001; i++) {
// Vertex a = this.sqlgGraph.addVertex(T.label, "God", "name", "god" + i);
// for (int j = 0; j < 2; j++) {
// Vertex b = this.sqlgGraph.addVertex(T.label, "Hand", "name", "name_" + j);
// a.addEdge("hand", b);
// for (int k = 0; k < 5; k++) {
// Vertex c = this.sqlgGraph.addVertex(T.label, "Finger", "name", "name_" + k);
// Edge e = b.addEdge("finger", c);
// }
// }
//// if (i % 500_000 == 0) {
//// this.graph.tx().flush();
//// stopWatch.split();
//// System.out.println(stopWatch.toString());
//// stopWatch.unsplit();
//// }
// }
// stopWatchAddOnly.stop();
// System.out.println("Time for add only : " + stopWatchAddOnly.toString());
// this.sqlgGraph.tx().commit();
// stopWatch.stop();
// System.out.println("Time for insert: " + stopWatch.toString());
// stopWatch.reset();
// stopWatch.start();
// for (int i = 0; i < 100; i++) {
// GraphTraversal traversal = sqlgGraph.traversal().V().hasLabel("God").as("god").out("hand").as("hand").out("finger").as("finger").path();
// while (traversal.hasNext()) {
// Path path = traversal.next();
// List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy