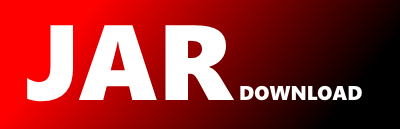
org.umlg.concretetest.Angel Maven / Gradle / Ivy
The newest version!
package org.umlg.concretetest;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import org.apache.commons.text.StringEscapeUtils;
import org.apache.tinkerpop.gremlin.structure.Vertex;
import org.umlg.concretetest.God.GodRuntimePropertyEnum;
import org.umlg.concretetest.Universe.UniverseRuntimePropertyEnum;
import org.umlg.query.BaseUmlgWithQuery;
import org.umlg.runtime.adaptor.UMLG;
import org.umlg.runtime.adaptor.UmlgLabelConverterFactory;
import org.umlg.runtime.adaptor.UmlgTmpIdManager;
import org.umlg.runtime.collection.Filter;
import org.umlg.runtime.collection.Qualifier;
import org.umlg.runtime.collection.UmlgCollection;
import org.umlg.runtime.collection.UmlgRuntimeProperty;
import org.umlg.runtime.collection.UmlgSet;
import org.umlg.runtime.collection.memory.UmlgMemorySet;
import org.umlg.runtime.collection.persistent.PropertyTree;
import org.umlg.runtime.collection.persistent.UmlgSetImpl;
import org.umlg.runtime.domain.CompositionNode;
import org.umlg.runtime.domain.DataTypeEnum;
import org.umlg.runtime.domain.UmlgNode;
import org.umlg.runtime.notification.ChangeHolder;
import org.umlg.runtime.notification.UmlgNotificationManager;
import org.umlg.runtime.util.ObjectMapperFactory;
import org.umlg.runtime.util.UmlgFormatter;
import org.umlg.runtime.validation.DateTimeValidation;
import org.umlg.runtime.validation.UmlgConstraintViolation;
import org.umlg.runtime.validation.UmlgConstraintViolationException;
import org.umlg.runtime.validation.UmlgValidation;
import org.umlg.tag.Tag;
public class Angel extends BaseUmlgWithQuery implements CompositionNode {
static final public long serialVersionUID = 1L;
private UmlgSet name;
private UmlgSet god;
private UmlgSet universe;
/**
* constructor for Angel
*
* @param compositeOwner
*/
public Angel(God compositeOwner) {
super(true);
compositeOwner.addToAngel(this);
}
/**
* constructor for Angel
*
* @param id
*/
public Angel(Object id) {
super(id);
}
/**
* constructor for Angel
*
* @param vertex
*/
public Angel(Vertex vertex) {
super(vertex);
}
/**
* default constructor for Angel
*/
public Angel() {
this(true);
}
/**
* constructor for Angel
*
* @param persistent
*/
public Angel(Boolean persistent) {
super(persistent);
}
public void addToGod(God god) {
if ( god != null ) {
if ( !this.god.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::A__::god is a one and already has a value!");
}
this.god.add(god);
}
}
public void addToGodIgnoreInverse(God god) {
if ( god != null ) {
if ( !this.god.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::A__::god is a one and already has a value!");
}
this.god.addIgnoreInverse(god);
}
}
public void addToName(String name) {
if ( !this.name.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( name != null ) {
List violations = validateName(name);
if ( violations.isEmpty() ) {
this.name.add(name);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToNameIgnoreInverse(String name) {
if ( !this.name.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( name != null ) {
List violations = validateName(name);
if ( violations.isEmpty() ) {
this.name.add(name);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToUniverse(Universe universe) {
if ( universe != null ) {
if ( !this.universe.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::A__::universe is a one and already has a value!");
}
this.universe.add(universe);
}
}
public void addToUniverseIgnoreInverse(Universe universe) {
if ( universe != null ) {
if ( !this.universe.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::A__::universe is a one and already has a value!");
}
this.universe.addIgnoreInverse(universe);
}
}
static public UmlgSet extends Angel> allInstances(Filter filter) {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(Angel.class.getName(), filter));
return result;
}
static public UmlgSet extends Angel> allInstances() {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(Angel.class.getName()));
return result;
}
@Override
public List checkClassConstraints() {
List result = new ArrayList();
result.addAll(super.checkClassConstraints());
return result;
}
public void clearGod() {
this.god.clear();
}
public void clearName() {
this.name.clear();
}
public void clearUniverse() {
this.universe.clear();
}
@Override
public void delete() {
if ( true ) {
UmlgNotificationManager.INSTANCE.notify(
GodRuntimePropertyEnum.angel,
ChangeHolder.of(
this.getGod(),
GodRuntimePropertyEnum.angel,
ChangeHolder.ChangeType.DELETE,
this.toJson()
)
);
}
this.god.clear();
this.universe.clear();
super.delete();
}
@Override
public void fromJson(Map propertyMap) {
fromJsonDataTypeAndComposite(propertyMap);
fromJsonNonCompositeOne(propertyMap);
fromJsonNonCompositeRequiredMany(propertyMap);
}
@Override
public void fromJson(String json) {
ObjectMapper mapper = ObjectMapperFactory.INSTANCE.getObjectMapper();
try {
@SuppressWarnings( "unchecked")
Map propertyMap = mapper.readValue(json, Map.class);
fromJson(propertyMap);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Override
public void fromJsonDataTypeAndComposite(Map propertyMap) {
super.fromJsonDataTypeAndComposite(propertyMap);
if ( propertyMap.containsKey("name") ) {
if ( propertyMap.get("name") != null ) {
String name = (String)propertyMap.get("name");
setName(name);
} else {
setName(null);
}
}
}
@SuppressWarnings( "unchecked")
@Override
public void fromJsonNonCompositeOne(Map propertyMap) {
super.fromJsonNonCompositeOne(propertyMap);
if ( propertyMap.containsKey("universe") ) {
if ( propertyMap.get("universe") != null ) {
Map universeMap = (Map)propertyMap.get("universe");
if ( universeMap.isEmpty() || universeMap.get("id") == null ) {
setUniverse(null);
} else {
setUniverse((Universe)UMLG.get().getEntity((universeMap.get("id"))));
}
} else {
setUniverse(null);
}
}
}
@Override
public void fromJsonNonCompositeRequiredMany(Map propertyMap) {
super.fromJsonNonCompositeRequiredMany(propertyMap);
}
public God getGod() {
UmlgSet tmp = this.god;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
@Override
public String getMetaDataAsJson() {
return Angel.AngelRuntimePropertyEnum.asJson();
}
public String getName() {
UmlgSet tmp = this.name;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
@Override
public UmlgNode getOwningObject() {
UmlgNode result;
if ( getGod() != null ) {
result = getGod();
} else {
result = null;
}
return result;
}
@Override
public String getQualifiedName() {
return "umlgtest::org::umlg::concretetest::Angel";
}
/**
* getQualifiers is called from the collection in order to update the index used to implement the qualifier
*
* @param tumlRuntimeProperty
* @param node
* @param inverse
*/
@Override
public List getQualifiers(UmlgRuntimeProperty tumlRuntimeProperty, UmlgNode node, boolean inverse) {
List result = super.getQualifiers(tumlRuntimeProperty, node, inverse);
AngelRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result.isEmpty() ) {
switch ( runtimeProperty ) {
default:
result = Collections.emptyList();
}
}
return result;
}
/**
* getSize is called from the BaseCollection.addInternal in order to save the size of the inverse collection to update the edge's sequence order
*
* @param inverse
* @param tumlRuntimeProperty
*/
@Override
public int getSize(boolean inverse, UmlgRuntimeProperty tumlRuntimeProperty) {
int result = super.getSize(inverse, tumlRuntimeProperty);
AngelRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result == 0 ) {
switch ( runtimeProperty ) {
case name:
result = name.size();
break;
case god:
result = god.size();
break;
case universe:
result = universe.size();
break;
default:
result = 0;
}
}
return result;
}
public Universe getUniverse() {
UmlgSet tmp = this.universe;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
@Override
public boolean hasOnlyOneCompositeParent() {
int result = 0;
result = result + (getGod() != null ? 1 : 0);
return result == 1;
}
public void initDataTypeVariablesWithDefaultValues() {
super.initDataTypeVariablesWithDefaultValues();
}
public void initVariables() {
super.initVariables();
}
/**
* boolean properties' default values are initialized in the constructor via z_internalBooleanProperties
*
* @param loaded
*/
@Override
public void initialiseProperties(boolean loaded) {
super.initialiseProperties(loaded);
this.name = new UmlgSetImpl(this, PropertyTree.from(AngelRuntimePropertyEnum.name), loaded);
this.god = new UmlgSetImpl(this, PropertyTree.from(AngelRuntimePropertyEnum.god), loaded);
this.universe = new UmlgSetImpl(this, PropertyTree.from(AngelRuntimePropertyEnum.universe), loaded);
}
@Override
public void initialiseProperty(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, boolean loaded) {
AngelRuntimePropertyEnum runtimeProperty;
super.initialiseProperty(tumlRuntimeProperty, inverse, loaded);
if ( !inverse ) {
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
this.name = new UmlgSetImpl(this, PropertyTree.from(AngelRuntimePropertyEnum.name), loaded);
break;
case god:
this.god = new UmlgSetImpl(this, PropertyTree.from(AngelRuntimePropertyEnum.god), loaded);
break;
case universe:
this.universe = new UmlgSetImpl(this, PropertyTree.from(AngelRuntimePropertyEnum.universe), loaded);
break;
}
}
}
@Override
public UmlgRuntimeProperty inverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
UmlgRuntimeProperty fromSuperRuntimeProperty = super.inverseAdder(tumlRuntimeProperty, inverse, umlgNode);
AngelRuntimePropertyEnum runtimeProperty;
if ( fromSuperRuntimeProperty != null ) {
return fromSuperRuntimeProperty;
} else {
if ( !inverse ) {
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case god:
this.god.inverseAdder((God)umlgNode);
break;
case universe:
this.universe.inverseAdder((Universe)umlgNode);
break;
}
return runtimeProperty;
} else {
return null;
}
}
}
@Override
public boolean isTinkerRoot() {
return false;
}
public UmlgSet lookupFor_angel_universe() {
UmlgSet result = new UmlgMemorySet();
Filter filter = new Filter() {
@Override
public boolean filter(Universe entity){
return entity.getAngel() == null;
}
};
result.addAll(org.umlg.concretetest.Universe.allInstances(filter));
return result;
}
public void removeFromGod(God god) {
if ( god != null ) {
this.god.remove(god);
}
}
public void removeFromGod(UmlgSet god) {
if ( !god.isEmpty() ) {
this.god.removeAll(god);
}
}
public void removeFromName(String name) {
if ( name != null ) {
this.name.remove(name);
}
}
public void removeFromName(UmlgSet name) {
if ( !name.isEmpty() ) {
this.name.removeAll(name);
}
}
public void removeFromUniverse(UmlgSet universe) {
if ( !universe.isEmpty() ) {
this.universe.removeAll(universe);
}
}
public void removeFromUniverse(Universe universe) {
if ( universe != null ) {
this.universe.remove(universe);
}
}
public void setGod(God god) {
clearGod();
addToGod(god);
}
public void setName(String name) {
if ( name == null ) {
clearName();
} else {
z_internalClearName();
}
addToName(name);
}
public void setUniverse(Universe universe) {
if ( universe != null ) {
universe.clearAngel();
universe.initialiseProperty(UniverseRuntimePropertyEnum.angel, false, true);
}
clearUniverse();
addToUniverse(universe);
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJson(Boolean deep) {
String result = super.toJson(deep);
result = result.substring(1, result.length() - 1);
StringBuilder sb = new StringBuilder(result);
sb.append(", ");
sb.append("\"name\": " + (getName() != null ? "\"" + StringEscapeUtils.escapeJson(getName()) + "\"" : null ));
sb.append(", ");
if ( getGod() != null ) {
if ( UmlgTmpIdManager.INSTANCE.get(getGod().getId()) != null ) {
sb.append("\"god\": " + "{\"id\": \"" + getGod().getId() + "\", \"tmpId\": \"" + UmlgTmpIdManager.INSTANCE.get(getGod().getId()) + "\",\"displayName\": \"" + getGod().getName() + "\"}" + "");
} else {
sb.append("\"god\": " + "{\"id\": \"" + getGod().getId() + "\", \"displayName\": \"" + getGod().getName() + "\"}" + "");
}
} else {
sb.append("\"god\": " + "{\"id\": " + null + ", \"displayName\": " + null + "}");
}
sb.append(", ");
if ( getUniverse() != null ) {
if ( UmlgTmpIdManager.INSTANCE.get(getUniverse().getId()) != null ) {
sb.append("\"universe\": " + "{\"id\": \"" + getUniverse().getId() + "\", \"tmpId\": \"" + UmlgTmpIdManager.INSTANCE.get(getUniverse().getId()) + "\",\"displayName\": \"" + getUniverse().getName() + "\"}" + "");
} else {
sb.append("\"universe\": " + "{\"id\": \"" + getUniverse().getId() + "\", \"displayName\": \"" + getUniverse().getName() + "\"}" + "");
}
} else {
sb.append("\"universe\": " + "{\"id\": " + null + ", \"displayName\": " + null + "}");
}
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJson() {
return toJson(false);
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJsonWithoutCompositeParent(Boolean deep) {
String result = super.toJsonWithoutCompositeParent(deep);
result = result.substring(1, result.length() - 1);
StringBuilder sb = new StringBuilder(result);
sb.append(", ");
sb.append("\"name\": " + (getName() != null ? "\"" + StringEscapeUtils.escapeJson(getName()) + "\"" : null ));
sb.append(", ");
if ( getUniverse() != null ) {
if ( UmlgTmpIdManager.INSTANCE.get(getUniverse().getId()) != null ) {
sb.append("\"universe\": " + "{\"id\": \"" + getUniverse().getId() + "\", \"tmpId\": \"" + UmlgTmpIdManager.INSTANCE.get(getUniverse().getId()) + "\",\"displayName\": \"" + getUniverse().getName() + "\"}" + "");
} else {
sb.append("\"universe\": " + "{\"id\": \"" + getUniverse().getId() + "\", \"displayName\": \"" + getUniverse().getName() + "\"}" + "");
}
} else {
sb.append("\"universe\": " + "{\"id\": " + null + ", \"displayName\": " + null + "}");
}
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJsonWithoutCompositeParent() {
return toJsonWithoutCompositeParent(false);
}
@Override
public List validateMultiplicities() {
List result = new ArrayList();
if ( getGod() == null ) {
result.add(new UmlgConstraintViolation("multiplicity", "umlgtest::org::umlg::concretetest::A__::god", "lower multiplicity is 1"));
}
if ( getUpdatedOn() == null ) {
result.add(new UmlgConstraintViolation("multiplicity", "umlglib::org::umlg::BaseModelUmlg::updatedOn", "lower multiplicity is 1"));
}
if ( getCreatedOn() == null ) {
result.add(new UmlgConstraintViolation("multiplicity", "umlglib::org::umlg::BaseModelUmlg::createdOn", "lower multiplicity is 1"));
}
return result;
}
public List validateName(String name) {
List result = new ArrayList();
return result;
}
@Override
public void z_internalAddPersistentValueToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
AngelRuntimePropertyEnum runtimeProperty;
super.z_internalAddPersistentValueToCollection(umlgRuntimeProperty, umlgNode);
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
this.name.z_internalAdder((String)umlgNode);
break;
case god:
this.god.z_internalAdder((God)umlgNode);
break;
case universe:
this.universe.z_internalAdder((Universe)umlgNode);
break;
}
}
}
@Override
public void z_internalAddToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
AngelRuntimePropertyEnum runtimeProperty;
super.z_internalAddToCollection(umlgRuntimeProperty, umlgNode);
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
this.name.z_internalAdder((String)umlgNode);
break;
case god:
this.god.z_internalAdder((God)umlgNode);
break;
case universe:
this.universe.z_internalAdder((Universe)umlgNode);
break;
}
}
}
@Override
public Set z_internalBooleanProperties() {
Set result = super.z_internalBooleanProperties();
return result;
}
public void z_internalClearGod() {
this.god.z_internalClear();
}
public void z_internalClearName() {
this.name.z_internalClear();
}
public void z_internalClearUniverse() {
this.universe.z_internalClear();
}
@Override
public Set z_internalDataTypeProperties() {
Set result = super.z_internalDataTypeProperties();
result.add(AngelRuntimePropertyEnum.name);
return result;
}
@Override
public Map z_internalDataTypePropertiesWithDefaultValues() {
Map result = super.z_internalDataTypePropertiesWithDefaultValues();
return result;
}
@Override
public UmlgCollection extends Object> z_internalGetCollectionFor(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse) {
UmlgCollection extends Object> result = null;
result = super.z_internalGetCollectionFor(tumlRuntimeProperty, inverse);
if ( result == null ) {
AngelRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
result = this.name;
break;
case god:
result = this.god;
break;
case universe:
result = this.universe;
break;
}
}
}
return result;
}
@Override
public UmlgRuntimeProperty z_internalInverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
UmlgRuntimeProperty fromSuperRuntimeProperty = super.inverseAdder(tumlRuntimeProperty, inverse, umlgNode);
AngelRuntimePropertyEnum runtimeProperty;
if ( fromSuperRuntimeProperty != null ) {
return fromSuperRuntimeProperty;
} else {
if ( !inverse ) {
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case god:
this.god.z_internalAdder((God)umlgNode);
break;
case universe:
this.universe.z_internalAdder((Universe)umlgNode);
break;
}
return runtimeProperty;
} else {
return null;
}
}
}
@Override
public void z_internalMarkCollectionLoaded(UmlgRuntimeProperty umlgRuntimeProperty, boolean loaded) {
AngelRuntimePropertyEnum runtimeProperty;
super.z_internalMarkCollectionLoaded(umlgRuntimeProperty, loaded);
runtimeProperty = (AngelRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
this.name.setLoaded(loaded);
break;
case god:
this.god.setLoaded(loaded);
break;
case universe:
this.universe.setLoaded(loaded);
break;
}
}
}
static public enum AngelRuntimePropertyEnum implements UmlgRuntimeProperty {
name(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Angel::name",/* persistentName */ "name",/* inverseName */ "inverseOf::name",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Angel::name",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("name"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ String.class,/* json */ "{\"name\": \"name\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Angel::name\", \"persistentName\": \"name\", \"inverseName\": \"inverseOf::name\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::concretetest::Angel::name\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"name\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
god(/* qualifiedName */ "umlgtest::org::umlg::concretetest::A__::god",/* persistentName */ "god",/* inverseName */ "angel",/* inverseQualifiedName */ "umlgtest::org::umlg::concretetest::God::angel",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ false,/* isComposite */ false,/* isInverseComposite */ true,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("A__"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 1,/* inverseUpper */ -1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ God.class,/* json */ "{\"name\": \"god\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::A__::god\", \"persistentName\": \"god\", \"inverseName\": \"angel\", \"inverseQualifiedName\": \"umlgtest::org::umlg::concretetest::God::angel\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": false, \"composite\": false, \"inverseComposite\": true, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 1, \"inverseUpper\": -1, \"label\": \"A__\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
updatedOn(/* qualifiedName */ "umlglib::org::umlg::BaseModelUmlg::updatedOn",/* persistentName */ "updatedOn",/* inverseName */ "inverseOf::updatedOn",/* inverseQualifiedName */ "inverseOf::umlglib::org::umlg::BaseModelUmlg::updatedOn",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ DataTypeEnum.DateTime,/* validations */ Arrays.asList(new DateTimeValidation()),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("updatedOn"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 1,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ LocalDateTime.class,/* json */ "{\"name\": \"updatedOn\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": \"" + DataTypeEnum.DateTime.toString() + "\", \"validations\": {\"dateTime\": {}}, \"qualifiedName\": \"umlglib::org::umlg::BaseModelUmlg::updatedOn\", \"persistentName\": \"updatedOn\", \"inverseName\": \"inverseOf::updatedOn\", \"inverseQualifiedName\": \"inverseOf::umlglib::org::umlg::BaseModelUmlg::updatedOn\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 1, \"inverseUpper\": 1, \"label\": \"updatedOn\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
universe(/* qualifiedName */ "umlgtest::org::umlg::concretetest::A__::universe",/* persistentName */ "universe",/* inverseName */ "angel",/* inverseQualifiedName */ "umlgtest::org::umlg::concretetest::A__::angel",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("A__"),/* isOneToOne */ true,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Universe.class,/* json */ "{\"name\": \"universe\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::A__::universe\", \"persistentName\": \"universe\", \"inverseName\": \"angel\", \"inverseQualifiedName\": \"umlgtest::org::umlg::concretetest::A__::angel\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": true, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"A__\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
tag(/* qualifiedName */ "umlglib::org::umlg::tag::tag_baseUmlg_1::tag",/* persistentName */ "tag",/* inverseName */ "baseUmlg",/* inverseQualifiedName */ "umlglib::org::umlg::tag::Tag::baseUmlg",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ false,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("tag_baseUmlg_1"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ -1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Tag.class,/* json */ "{\"name\": \"tag\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlglib::org::umlg::tag::tag_baseUmlg_1::tag\", \"persistentName\": \"tag\", \"inverseName\": \"baseUmlg\", \"inverseQualifiedName\": \"umlglib::org::umlg::tag::Tag::baseUmlg\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": false, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": -1, \"label\": \"tag_baseUmlg_1\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
createdOn(/* qualifiedName */ "umlglib::org::umlg::BaseModelUmlg::createdOn",/* persistentName */ "createdOn",/* inverseName */ "inverseOf::createdOn",/* inverseQualifiedName */ "inverseOf::umlglib::org::umlg::BaseModelUmlg::createdOn",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ DataTypeEnum.DateTime,/* validations */ Arrays.asList(new DateTimeValidation()),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("createdOn"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 1,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ LocalDateTime.class,/* json */ "{\"name\": \"createdOn\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": \"" + DataTypeEnum.DateTime.toString() + "\", \"validations\": {\"dateTime\": {}}, \"qualifiedName\": \"umlglib::org::umlg::BaseModelUmlg::createdOn\", \"persistentName\": \"createdOn\", \"inverseName\": \"inverseOf::createdOn\", \"inverseQualifiedName\": \"inverseOf::umlglib::org::umlg::BaseModelUmlg::createdOn\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 1, \"inverseUpper\": 1, \"label\": \"createdOn\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false);
private String _qualifiedName;
private String _persistentName;
private String _inverseName;
private String _inverseQualifiedName;
private boolean _associationClassOne;
private boolean _memberEndOfAssociationClass;
private String _associationClassPropertyName;
private String _inverseAssociationClassPropertyName;
private boolean _associationClassProperty;
private boolean _onePrimitivePropertyOfAssociationClass;
private boolean _onePrimitive;
private Boolean _readOnly;
private DataTypeEnum dataTypeEnum;
private List validations;
private boolean _manyPrimitive;
private boolean _oneEnumeration;
private boolean _manyEnumeration;
private boolean _controllingSide;
private boolean _composite;
private boolean _inverseComposite;
private String _label;
private boolean _oneToOne;
private boolean _oneToMany;
private boolean _manyToOne;
private boolean _manyToMany;
private int _upper;
private int _lower;
private int _inverseUpper;
private boolean _qualified;
private boolean _inverseQualified;
private boolean _ordered;
private boolean _inverseOrdered;
private boolean _unique;
private boolean _inverseUnique;
private boolean _derived;
private boolean _navigability;
private Class _propertyType;
private String _json;
private boolean _changeListener;
/**
* constructor for AngelRuntimePropertyEnum
*
* @param _qualifiedName
* @param _persistentName
* @param _inverseName
* @param _inverseQualifiedName
* @param _associationClassOne
* @param _memberEndOfAssociationClass
* @param _associationClassPropertyName
* @param _inverseAssociationClassPropertyName
* @param _associationClassProperty
* @param _onePrimitivePropertyOfAssociationClass
* @param _onePrimitive
* @param _readOnly
* @param dataTypeEnum
* @param validations
* @param _manyPrimitive
* @param _oneEnumeration
* @param _manyEnumeration
* @param _controllingSide
* @param _composite
* @param _inverseComposite
* @param _label
* @param _oneToOne
* @param _oneToMany
* @param _manyToOne
* @param _manyToMany
* @param _upper
* @param _lower
* @param _inverseUpper
* @param _qualified
* @param _inverseQualified
* @param _ordered
* @param _inverseOrdered
* @param _unique
* @param _inverseUnique
* @param _derived
* @param _navigability
* @param _propertyType
* @param _json
* @param _changeListener
*/
private AngelRuntimePropertyEnum(String _qualifiedName, String _persistentName, String _inverseName, String _inverseQualifiedName, boolean _associationClassOne, boolean _memberEndOfAssociationClass, String _associationClassPropertyName, String _inverseAssociationClassPropertyName, boolean _associationClassProperty, boolean _onePrimitivePropertyOfAssociationClass, boolean _onePrimitive, Boolean _readOnly, DataTypeEnum dataTypeEnum, List validations, boolean _manyPrimitive, boolean _oneEnumeration, boolean _manyEnumeration, boolean _controllingSide, boolean _composite, boolean _inverseComposite, String _label, boolean _oneToOne, boolean _oneToMany, boolean _manyToOne, boolean _manyToMany, int _upper, int _lower, int _inverseUpper, boolean _qualified, boolean _inverseQualified, boolean _ordered, boolean _inverseOrdered, boolean _unique, boolean _inverseUnique, boolean _derived, boolean _navigability, Class _propertyType, String _json, boolean _changeListener) {
this._qualifiedName = _qualifiedName;
this._persistentName = _persistentName;
this._inverseName = _inverseName;
this._inverseQualifiedName = _inverseQualifiedName;
this._associationClassOne = _associationClassOne;
this._memberEndOfAssociationClass = _memberEndOfAssociationClass;
this._associationClassPropertyName = _associationClassPropertyName;
this._inverseAssociationClassPropertyName = _inverseAssociationClassPropertyName;
this._associationClassProperty = _associationClassProperty;
this._onePrimitivePropertyOfAssociationClass = _onePrimitivePropertyOfAssociationClass;
this._onePrimitive = _onePrimitive;
this._readOnly = _readOnly;
this.dataTypeEnum = dataTypeEnum;
this.validations = validations;
this._manyPrimitive = _manyPrimitive;
this._oneEnumeration = _oneEnumeration;
this._manyEnumeration = _manyEnumeration;
this._controllingSide = _controllingSide;
this._composite = _composite;
this._inverseComposite = _inverseComposite;
this._label = _label;
this._oneToOne = _oneToOne;
this._oneToMany = _oneToMany;
this._manyToOne = _manyToOne;
this._manyToMany = _manyToMany;
this._upper = _upper;
this._lower = _lower;
this._inverseUpper = _inverseUpper;
this._qualified = _qualified;
this._inverseQualified = _inverseQualified;
this._ordered = _ordered;
this._inverseOrdered = _inverseOrdered;
this._unique = _unique;
this._inverseUnique = _inverseUnique;
this._derived = _derived;
this._navigability = _navigability;
this._propertyType = _propertyType;
this._json = _json;
this._changeListener = _changeListener;
}
static public String asJson() {
int count = 1;
StringBuilder sb = new StringBuilder();;
sb.append("{\"name\": \"Angel\", ");
sb.append("\"qualifiedName\": \"umlgtest::org::umlg::concretetest::Angel\", ");
sb.append("\"uri\": \"TODO\", ");
sb.append("\"properties\": [");
for ( AngelRuntimePropertyEnum l : AngelRuntimePropertyEnum.values() ) {
sb.append(l.toJson());
if ( count < AngelRuntimePropertyEnum.values().length ) {
count++;
sb.append(",");
}
}
sb.append("]}");
return sb.toString();
}
static public AngelRuntimePropertyEnum fromInverseQualifiedName(String inverseQualifiedName) {
if ( createdOn.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return createdOn;
}
if ( tag.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return tag;
}
if ( universe.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return universe;
}
if ( updatedOn.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return updatedOn;
}
if ( god.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return god;
}
if ( name.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return name;
}
return null;
}
static public AngelRuntimePropertyEnum fromLabel(String _label) {
if ( createdOn.getLabel().equals(_label) ) {
return createdOn;
}
if ( tag.getLabel().equals(_label) ) {
return tag;
}
if ( universe.getLabel().equals(_label) ) {
return universe;
}
if ( updatedOn.getLabel().equals(_label) ) {
return updatedOn;
}
if ( god.getLabel().equals(_label) ) {
return god;
}
if ( name.getLabel().equals(_label) ) {
return name;
}
return null;
}
static public AngelRuntimePropertyEnum fromQualifiedName(String qualifiedName) {
if ( createdOn.getQualifiedName().equals(qualifiedName) ) {
return createdOn;
}
if ( tag.getQualifiedName().equals(qualifiedName) ) {
return tag;
}
if ( universe.getQualifiedName().equals(qualifiedName) ) {
return universe;
}
if ( updatedOn.getQualifiedName().equals(qualifiedName) ) {
return updatedOn;
}
if ( god.getQualifiedName().equals(qualifiedName) ) {
return god;
}
if ( name.getQualifiedName().equals(qualifiedName) ) {
return name;
}
return null;
}
public String getAssociationClassPropertyName() {
return this._associationClassPropertyName;
}
public DataTypeEnum getDataTypeEnum() {
return this.dataTypeEnum;
}
public String getInverseAssociationClassPropertyName() {
return this._inverseAssociationClassPropertyName;
}
public String getInverseName() {
return this._inverseName;
}
public String getInverseQualifiedName() {
return this._inverseQualifiedName;
}
public int getInverseUpper() {
return this._inverseUpper;
}
public String getJson() {
return this._json;
}
public String getLabel() {
return this._label;
}
public int getLower() {
return this._lower;
}
public String getPersistentName() {
return this._persistentName;
}
public Class getPropertyType() {
return this._propertyType;
}
public String getQualifiedName() {
return this._qualifiedName;
}
public Boolean getReadOnly() {
return this._readOnly;
}
public int getUpper() {
return this._upper;
}
public List getValidations() {
return this.validations;
}
public boolean isAssociationClassOne() {
return this._associationClassOne;
}
public boolean isAssociationClassProperty() {
return this._associationClassProperty;
}
public boolean isChangeListener() {
return this._changeListener;
}
public boolean isComposite() {
return this._composite;
}
public boolean isControllingSide() {
return this._controllingSide;
}
public boolean isDerived() {
return this._derived;
}
public boolean isInverseComposite() {
return this._inverseComposite;
}
public boolean isInverseOrdered() {
return this._inverseOrdered;
}
public boolean isInverseQualified() {
return this._inverseQualified;
}
public boolean isInverseUnique() {
return this._inverseUnique;
}
public boolean isManyEnumeration() {
return this._manyEnumeration;
}
public boolean isManyPrimitive() {
return this._manyPrimitive;
}
public boolean isManyToMany() {
return this._manyToMany;
}
public boolean isManyToOne() {
return this._manyToOne;
}
public boolean isMemberEndOfAssociationClass() {
return this._memberEndOfAssociationClass;
}
public boolean isNavigability() {
return this._navigability;
}
public boolean isOneEnumeration() {
return this._oneEnumeration;
}
public boolean isOnePrimitive() {
return this._onePrimitive;
}
public boolean isOnePrimitivePropertyOfAssociationClass() {
return this._onePrimitivePropertyOfAssociationClass;
}
public boolean isOneToMany() {
return this._oneToMany;
}
public boolean isOneToOne() {
return this._oneToOne;
}
public boolean isOrdered() {
return this._ordered;
}
public boolean isQualified() {
return this._qualified;
}
public boolean isUnique() {
return this._unique;
}
@Override
public boolean isValid(int elementCount) {
if ( isQualified() ) {
return elementCount >= getLower();
} else {
return (getUpper() == -1 || elementCount <= getUpper()) && elementCount >= getLower();
}
}
@Override
public String toJson() {
return getJson();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy