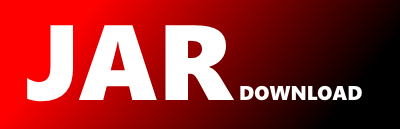
org.umlg.concretetest.Universe Maven / Gradle / Ivy
The newest version!
package org.umlg.concretetest;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.lang.reflect.Constructor;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.text.StringEscapeUtils;
import org.apache.tinkerpop.gremlin.structure.Vertex;
import org.umlg.componenttest.SpaceTime;
import org.umlg.componenttest.SpaceTime.SpaceTimeRuntimePropertyEnum;
import org.umlg.concretetest.Angel.AngelRuntimePropertyEnum;
import org.umlg.concretetest.Demon.DemonRuntimePropertyEnum;
import org.umlg.navigability.NonNavigableMany;
import org.umlg.navigability.NonNavigableMany.NonNavigableManyRuntimePropertyEnum;
import org.umlg.navigability.NonNavigableOne;
import org.umlg.navigability.NonNavigableOne.NonNavigableOneRuntimePropertyEnum;
import org.umlg.query.BaseUmlgWithQuery;
import org.umlg.runtime.adaptor.UMLG;
import org.umlg.runtime.adaptor.UmlgLabelConverterFactory;
import org.umlg.runtime.adaptor.UmlgSchemaFactory;
import org.umlg.runtime.adaptor.UmlgTmpIdManager;
import org.umlg.runtime.collection.Filter;
import org.umlg.runtime.collection.Qualifier;
import org.umlg.runtime.collection.UmlgCollection;
import org.umlg.runtime.collection.UmlgRuntimeProperty;
import org.umlg.runtime.collection.UmlgSet;
import org.umlg.runtime.collection.memory.UmlgMemorySet;
import org.umlg.runtime.collection.persistent.PropertyTree;
import org.umlg.runtime.collection.persistent.UmlgSetImpl;
import org.umlg.runtime.domain.CompositionNode;
import org.umlg.runtime.domain.DataTypeEnum;
import org.umlg.runtime.domain.UmlgNode;
import org.umlg.runtime.domain.json.ToJsonUtil;
import org.umlg.runtime.util.ObjectMapperFactory;
import org.umlg.runtime.util.UmlgFormatter;
import org.umlg.runtime.validation.DateTimeValidation;
import org.umlg.runtime.validation.Email;
import org.umlg.runtime.validation.MaxInteger;
import org.umlg.runtime.validation.MaxLength;
import org.umlg.runtime.validation.MinInteger;
import org.umlg.runtime.validation.MinLength;
import org.umlg.runtime.validation.RangeInteger;
import org.umlg.runtime.validation.RangeLength;
import org.umlg.runtime.validation.UmlgConstraintViolation;
import org.umlg.runtime.validation.UmlgConstraintViolationException;
import org.umlg.runtime.validation.UmlgValidation;
import org.umlg.runtime.validation.UmlgValidator;
import org.umlg.tag.Tag;
public class Universe extends BaseUmlgWithQuery implements CompositionNode {
static final public long serialVersionUID = 1L;
private UmlgSet name;
private UmlgSet spaceTime;
private UmlgSet testMinLength;
private UmlgSet maxLength;
private UmlgSet rangeLength;
private UmlgSet min;
private UmlgSet max;
private UmlgSet range;
private UmlgSet email;
private UmlgSet god;
private UmlgSet angel;
private UmlgSet demon;
private UmlgSet nonNavigableOne;
private UmlgSet nonNavigableMany;
/**
* constructor for Universe
*
* @param compositeOwner
*/
public Universe(God compositeOwner) {
super(true);
compositeOwner.addToUniverse(this);
}
/**
* constructor for Universe
*
* @param id
*/
public Universe(Object id) {
super(id);
}
/**
* constructor for Universe
*
* @param vertex
*/
public Universe(Vertex vertex) {
super(vertex);
}
/**
* default constructor for Universe
*/
public Universe() {
this(true);
}
/**
* constructor for Universe
*
* @param persistent
*/
public Universe(Boolean persistent) {
super(persistent);
}
public void addToAngel(Angel angel) {
if ( angel != null ) {
if ( !this.angel.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::A__::angel is a one and already has a value!");
}
this.angel.add(angel);
}
}
public void addToAngelIgnoreInverse(Angel angel) {
if ( angel != null ) {
if ( !this.angel.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::A__::angel is a one and already has a value!");
}
this.angel.addIgnoreInverse(angel);
}
}
public void addToDemon(Demon demon) {
if ( demon != null ) {
demon.clearUniverse();
demon.initialiseProperty(DemonRuntimePropertyEnum.universe, false, true);
removeFromDemon(demon);
}
if ( demon != null ) {
this.demon.add(demon);
}
}
public void addToDemon(UmlgSet demon) {
if ( !demon.isEmpty() ) {
this.demon.addAll(demon);
}
}
public void addToDemonIgnoreInverse(Demon demon) {
if ( demon != null ) {
demon.clearUniverse();
demon.initialiseProperty(DemonRuntimePropertyEnum.universe, false, true);
removeFromDemon(demon);
}
if ( demon != null ) {
this.demon.addIgnoreInverse(demon);
}
}
public void addToEmail(String email) {
if ( !this.email.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( email != null ) {
List violations = validateEmail(email);
if ( violations.isEmpty() ) {
this.email.add(email);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToEmailIgnoreInverse(String email) {
if ( !this.email.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( email != null ) {
List violations = validateEmail(email);
if ( violations.isEmpty() ) {
this.email.add(email);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToGod(God god) {
if ( god != null ) {
if ( !this.god.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::A__::god is a one and already has a value!");
}
this.god.add(god);
}
}
public void addToGodIgnoreInverse(God god) {
if ( god != null ) {
if ( !this.god.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::A__::god is a one and already has a value!");
}
this.god.addIgnoreInverse(god);
}
}
public void addToMax(Integer max) {
if ( !this.max.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( max != null ) {
List violations = validateMax(max);
if ( violations.isEmpty() ) {
this.max.add(max);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToMaxIgnoreInverse(Integer max) {
if ( !this.max.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( max != null ) {
List violations = validateMax(max);
if ( violations.isEmpty() ) {
this.max.add(max);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToMaxLength(String maxLength) {
if ( !this.maxLength.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( maxLength != null ) {
List violations = validateMaxLength(maxLength);
if ( violations.isEmpty() ) {
this.maxLength.add(maxLength);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToMaxLengthIgnoreInverse(String maxLength) {
if ( !this.maxLength.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( maxLength != null ) {
List violations = validateMaxLength(maxLength);
if ( violations.isEmpty() ) {
this.maxLength.add(maxLength);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToMin(Integer min) {
if ( !this.min.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( min != null ) {
List violations = validateMin(min);
if ( violations.isEmpty() ) {
this.min.add(min);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToMinIgnoreInverse(Integer min) {
if ( !this.min.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( min != null ) {
List violations = validateMin(min);
if ( violations.isEmpty() ) {
this.min.add(min);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToName(String name) {
if ( !this.name.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( name != null ) {
List violations = validateName(name);
if ( violations.isEmpty() ) {
this.name.add(name);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToNameIgnoreInverse(String name) {
if ( !this.name.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( name != null ) {
List violations = validateName(name);
if ( violations.isEmpty() ) {
this.name.add(name);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToNonNavigableMany(NonNavigableMany nonNavigableMany) {
if ( nonNavigableMany != null ) {
nonNavigableMany.clearUniverse();
nonNavigableMany.initialiseProperty(NonNavigableManyRuntimePropertyEnum.universe, false, true);
removeFromNonNavigableMany(nonNavigableMany);
}
if ( nonNavigableMany != null ) {
this.nonNavigableMany.add(nonNavigableMany);
}
}
public void addToNonNavigableMany(UmlgSet nonNavigableMany) {
if ( !nonNavigableMany.isEmpty() ) {
this.nonNavigableMany.addAll(nonNavigableMany);
}
}
public void addToNonNavigableManyIgnoreInverse(NonNavigableMany nonNavigableMany) {
if ( nonNavigableMany != null ) {
nonNavigableMany.clearUniverse();
nonNavigableMany.initialiseProperty(NonNavigableManyRuntimePropertyEnum.universe, false, true);
removeFromNonNavigableMany(nonNavigableMany);
}
if ( nonNavigableMany != null ) {
this.nonNavigableMany.addIgnoreInverse(nonNavigableMany);
}
}
public void addToNonNavigableOne(NonNavigableOne nonNavigableOne) {
if ( nonNavigableOne != null ) {
if ( !this.nonNavigableOne.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::navigability::A__::nonNavigableOne is a one and already has a value!");
}
this.nonNavigableOne.add(nonNavigableOne);
}
}
public void addToNonNavigableOneIgnoreInverse(NonNavigableOne nonNavigableOne) {
if ( nonNavigableOne != null ) {
if ( !this.nonNavigableOne.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::navigability::A__::nonNavigableOne is a one and already has a value!");
}
this.nonNavigableOne.addIgnoreInverse(nonNavigableOne);
}
}
public void addToRange(Integer range) {
if ( !this.range.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( range != null ) {
List violations = validateRange(range);
if ( violations.isEmpty() ) {
this.range.add(range);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToRangeIgnoreInverse(Integer range) {
if ( !this.range.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( range != null ) {
List violations = validateRange(range);
if ( violations.isEmpty() ) {
this.range.add(range);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToRangeLength(String rangeLength) {
if ( !this.rangeLength.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( rangeLength != null ) {
List violations = validateRangeLength(rangeLength);
if ( violations.isEmpty() ) {
this.rangeLength.add(rangeLength);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToRangeLengthIgnoreInverse(String rangeLength) {
if ( !this.rangeLength.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( rangeLength != null ) {
List violations = validateRangeLength(rangeLength);
if ( violations.isEmpty() ) {
this.rangeLength.add(rangeLength);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToSpaceTime(SpaceTime spaceTime) {
if ( spaceTime != null ) {
if ( !this.spaceTime.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::Universe::spaceTime is a one and already has a value!");
}
this.spaceTime.add(spaceTime);
}
}
public void addToSpaceTimeIgnoreInverse(SpaceTime spaceTime) {
if ( spaceTime != null ) {
if ( !this.spaceTime.isEmpty() ) {
throw new RuntimeException("Property umlgtest::org::umlg::concretetest::Universe::spaceTime is a one and already has a value!");
}
this.spaceTime.addIgnoreInverse(spaceTime);
}
}
public void addToTestMinLength(String testMinLength) {
if ( !this.testMinLength.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( testMinLength != null ) {
List violations = validateTestMinLength(testMinLength);
if ( violations.isEmpty() ) {
this.testMinLength.add(testMinLength);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToTestMinLengthIgnoreInverse(String testMinLength) {
if ( !this.testMinLength.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( testMinLength != null ) {
List violations = validateTestMinLength(testMinLength);
if ( violations.isEmpty() ) {
this.testMinLength.add(testMinLength);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
static public UmlgSet extends Universe> allInstances(Filter filter) {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(Universe.class.getName(), filter));
return result;
}
static public UmlgSet extends Universe> allInstances() {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(Universe.class.getName()));
return result;
}
@Override
public List checkClassConstraints() {
List result = new ArrayList();
result.addAll(super.checkClassConstraints());
return result;
}
public void clearAngel() {
this.angel.clear();
}
public void clearDemon() {
this.demon.clear();
}
public void clearEmail() {
this.email.clear();
}
public void clearGod() {
this.god.clear();
}
public void clearMax() {
this.max.clear();
}
public void clearMaxLength() {
this.maxLength.clear();
}
public void clearMin() {
this.min.clear();
}
public void clearName() {
this.name.clear();
}
public void clearNonNavigableMany() {
this.nonNavigableMany.clear();
}
public void clearNonNavigableOne() {
this.nonNavigableOne.clear();
}
public void clearRange() {
this.range.clear();
}
public void clearRangeLength() {
this.rangeLength.clear();
}
public void clearSpaceTime() {
this.spaceTime.clear();
}
public void clearTestMinLength() {
this.testMinLength.clear();
}
@Override
public void delete() {
if ( getSpaceTime() != null ) {
getSpaceTime().delete();
}
this.god.clear();
this.nonNavigableMany.clear();
this.nonNavigableOne.clear();
this.angel.clear();
this.email.clear();
this.demon.clear();
super.delete();
}
@Override
public void fromJson(Map propertyMap) {
fromJsonDataTypeAndComposite(propertyMap);
fromJsonNonCompositeOne(propertyMap);
fromJsonNonCompositeRequiredMany(propertyMap);
}
@Override
public void fromJson(String json) {
ObjectMapper mapper = ObjectMapperFactory.INSTANCE.getObjectMapper();
try {
@SuppressWarnings( "unchecked")
Map propertyMap = mapper.readValue(json, Map.class);
fromJson(propertyMap);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@SuppressWarnings( "unchecked")
@Override
public void fromJsonDataTypeAndComposite(Map propertyMap) {
Number rangeAsNumber = (Number)propertyMap.get("range");
Number minAsNumber = (Number)propertyMap.get("min");
Number maxAsNumber = (Number)propertyMap.get("max");
super.fromJsonDataTypeAndComposite(propertyMap);
if ( propertyMap.containsKey("spaceTime") ) {
if ( propertyMap.get("spaceTime") != null ) {
Map spaceTimeMap = (Map)propertyMap.get("spaceTime");
SpaceTime spaceTime;
String idField = (String)spaceTimeMap.get("id");
if ( idField != null && !idField.startsWith("fake") ) {
spaceTime = UMLG.get().getEntity((spaceTimeMap.get("id")));
} else {
Class baseTumlClass = UmlgSchemaFactory.getUmlgSchemaMap().get((String)spaceTimeMap.get("qualifiedName"));
try {
Constructor constructor = baseTumlClass.getConstructor(Boolean.class);
spaceTime = constructor.newInstance(true);
setSpaceTime(spaceTime);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
spaceTime.fromJsonDataTypeAndComposite(spaceTimeMap);
} else {
setSpaceTime(null);
}
}
if ( propertyMap.containsKey("rangeLength") ) {
if ( propertyMap.get("rangeLength") != null ) {
String rangeLength = (String)propertyMap.get("rangeLength");
setRangeLength(rangeLength);
} else {
setRangeLength(null);
}
}
if ( propertyMap.containsKey("range") ) {
if ( propertyMap.get("range") != null ) {
Integer range = rangeAsNumber != null ? rangeAsNumber.intValue() : null;
setRange(range);
} else {
setRange(null);
}
}
if ( propertyMap.containsKey("name") ) {
if ( propertyMap.get("name") != null ) {
String name = (String)propertyMap.get("name");
setName(name);
} else {
setName(null);
}
}
if ( propertyMap.containsKey("maxLength") ) {
if ( propertyMap.get("maxLength") != null ) {
String maxLength = (String)propertyMap.get("maxLength");
setMaxLength(maxLength);
} else {
setMaxLength(null);
}
}
if ( propertyMap.containsKey("testMinLength") ) {
if ( propertyMap.get("testMinLength") != null ) {
String testMinLength = (String)propertyMap.get("testMinLength");
setTestMinLength(testMinLength);
} else {
setTestMinLength(null);
}
}
if ( propertyMap.containsKey("min") ) {
if ( propertyMap.get("min") != null ) {
Integer min = minAsNumber != null ? minAsNumber.intValue() : null;
setMin(min);
} else {
setMin(null);
}
}
if ( propertyMap.containsKey("email") ) {
if ( propertyMap.get("email") != null ) {
String email = (java.lang.String)propertyMap.get("email");
setEmail(email);
} else {
setEmail(null);
}
}
if ( propertyMap.containsKey("max") ) {
if ( propertyMap.get("max") != null ) {
Integer max = maxAsNumber != null ? maxAsNumber.intValue() : null;
setMax(max);
} else {
setMax(null);
}
}
}
@SuppressWarnings( "unchecked")
@Override
public void fromJsonNonCompositeOne(Map propertyMap) {
super.fromJsonNonCompositeOne(propertyMap);
if ( propertyMap.containsKey("spaceTime") ) {
if ( propertyMap.get("spaceTime") != null ) {
Map spaceTimeMap = (Map)propertyMap.get("spaceTime");
SpaceTime spaceTime;
if ( spaceTimeMap.get("id") != null ) {
spaceTime = UMLG.get().getEntity((spaceTimeMap.get("id")));
} else {
Class baseTumlClass = UmlgSchemaFactory.getUmlgSchemaMap().get((String)spaceTimeMap.get("qualifiedName"));
try {
Constructor constructor = baseTumlClass.getConstructor(Boolean.class);
spaceTime = constructor.newInstance(true);
setSpaceTime(spaceTime);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
spaceTime.fromJsonNonCompositeOne(spaceTimeMap);
} else {
setSpaceTime(null);
}
}
if ( propertyMap.containsKey("nonNavigableOne") ) {
if ( propertyMap.get("nonNavigableOne") != null ) {
Map nonNavigableOneMap = (Map)propertyMap.get("nonNavigableOne");
if ( nonNavigableOneMap.isEmpty() || nonNavigableOneMap.get("id") == null ) {
setNonNavigableOne(null);
} else {
setNonNavigableOne((NonNavigableOne)UMLG.get().getEntity((nonNavigableOneMap.get("id"))));
}
} else {
setNonNavigableOne(null);
}
}
if ( propertyMap.containsKey("angel") ) {
if ( propertyMap.get("angel") != null ) {
Map angelMap = (Map)propertyMap.get("angel");
if ( angelMap.isEmpty() || angelMap.get("id") == null ) {
setAngel(null);
} else {
setAngel((Angel)UMLG.get().getEntity((angelMap.get("id"))));
}
} else {
setAngel(null);
}
}
}
@Override
public void fromJsonNonCompositeRequiredMany(Map propertyMap) {
super.fromJsonNonCompositeRequiredMany(propertyMap);
}
public Angel getAngel() {
UmlgSet tmp = this.angel;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public UmlgSet getDemon() {
return this.demon;
}
public String getEmail() {
UmlgSet tmp = this.email;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public God getGod() {
UmlgSet tmp = this.god;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Integer getMax() {
UmlgSet tmp = this.max;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public String getMaxLength() {
UmlgSet tmp = this.maxLength;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
@Override
public String getMetaDataAsJson() {
return Universe.UniverseRuntimePropertyEnum.asJson();
}
public Integer getMin() {
UmlgSet tmp = this.min;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public String getName() {
UmlgSet tmp = this.name;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public UmlgSet getNonNavigableMany() {
return this.nonNavigableMany;
}
public NonNavigableOne getNonNavigableOne() {
UmlgSet tmp = this.nonNavigableOne;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
@Override
public UmlgNode getOwningObject() {
UmlgNode result;
if ( getGod() != null ) {
result = getGod();
} else {
result = null;
}
return result;
}
@Override
public String getQualifiedName() {
return "umlgtest::org::umlg::concretetest::Universe";
}
/**
* getQualifiers is called from the collection in order to update the index used to implement the qualifier
*
* @param tumlRuntimeProperty
* @param node
* @param inverse
*/
@Override
public List getQualifiers(UmlgRuntimeProperty tumlRuntimeProperty, UmlgNode node, boolean inverse) {
List result = super.getQualifiers(tumlRuntimeProperty, node, inverse);
UniverseRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result.isEmpty() ) {
switch ( runtimeProperty ) {
default:
result = Collections.emptyList();
}
}
return result;
}
public Integer getRange() {
UmlgSet tmp = this.range;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public String getRangeLength() {
UmlgSet tmp = this.rangeLength;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
/**
* getSize is called from the BaseCollection.addInternal in order to save the size of the inverse collection to update the edge's sequence order
*
* @param inverse
* @param tumlRuntimeProperty
*/
@Override
public int getSize(boolean inverse, UmlgRuntimeProperty tumlRuntimeProperty) {
int result = super.getSize(inverse, tumlRuntimeProperty);
UniverseRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result == 0 ) {
switch ( runtimeProperty ) {
case spaceTime:
result = spaceTime.size();
break;
case rangeLength:
result = rangeLength.size();
break;
case range:
result = range.size();
break;
case demon:
result = demon.size();
break;
case maxLength:
result = maxLength.size();
break;
case testMinLength:
result = testMinLength.size();
break;
case god:
result = god.size();
break;
case nonNavigableMany:
result = nonNavigableMany.size();
break;
case min:
result = min.size();
break;
case max:
result = max.size();
break;
case email:
result = email.size();
break;
case name:
result = name.size();
break;
case nonNavigableOne:
result = nonNavigableOne.size();
break;
case angel:
result = angel.size();
break;
default:
result = 0;
}
}
return result;
}
public SpaceTime getSpaceTime() {
UmlgSet tmp = this.spaceTime;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public String getTestMinLength() {
UmlgSet tmp = this.testMinLength;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
@Override
public boolean hasOnlyOneCompositeParent() {
int result = 0;
result = result + (getGod() != null ? 1 : 0);
return result == 1;
}
public void initDataTypeVariablesWithDefaultValues() {
super.initDataTypeVariablesWithDefaultValues();
}
public void initVariables() {
super.initVariables();
}
/**
* boolean properties' default values are initialized in the constructor via z_internalBooleanProperties
*
* @param loaded
*/
@Override
public void initialiseProperties(boolean loaded) {
super.initialiseProperties(loaded);
this.spaceTime = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.spaceTime), loaded);
this.rangeLength = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.rangeLength), loaded);
this.range = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.range), loaded);
this.maxLength = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.maxLength), loaded);
this.testMinLength = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.testMinLength), loaded);
this.god = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.god), loaded);
this.nonNavigableMany = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.nonNavigableMany), loaded);
this.min = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.min), loaded);
this.email = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.email), loaded);
this.name = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.name), loaded);
this.nonNavigableOne = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.nonNavigableOne), loaded);
this.angel = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.angel), loaded);
this.max = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.max), loaded);
this.demon = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.demon), loaded);
}
@Override
public void initialiseProperty(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, boolean loaded) {
UniverseRuntimePropertyEnum runtimeProperty;
super.initialiseProperty(tumlRuntimeProperty, inverse, loaded);
if ( !inverse ) {
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case maxLength:
this.maxLength = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.maxLength), loaded);
break;
case spaceTime:
this.spaceTime = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.spaceTime), loaded);
break;
case rangeLength:
this.rangeLength = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.rangeLength), loaded);
break;
case range:
this.range = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.range), loaded);
break;
case testMinLength:
this.testMinLength = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.testMinLength), loaded);
break;
case demon:
this.demon = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.demon), loaded);
break;
case god:
this.god = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.god), loaded);
break;
case nonNavigableMany:
this.nonNavigableMany = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.nonNavigableMany), loaded);
break;
case min:
this.min = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.min), loaded);
break;
case angel:
this.angel = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.angel), loaded);
break;
case email:
this.email = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.email), loaded);
break;
case name:
this.name = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.name), loaded);
break;
case max:
this.max = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.max), loaded);
break;
case nonNavigableOne:
this.nonNavigableOne = new UmlgSetImpl(this, PropertyTree.from(UniverseRuntimePropertyEnum.nonNavigableOne), loaded);
break;
}
}
}
@Override
public UmlgRuntimeProperty inverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
UmlgRuntimeProperty fromSuperRuntimeProperty = super.inverseAdder(tumlRuntimeProperty, inverse, umlgNode);
UniverseRuntimePropertyEnum runtimeProperty;
if ( fromSuperRuntimeProperty != null ) {
return fromSuperRuntimeProperty;
} else {
if ( !inverse ) {
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case spaceTime:
this.spaceTime.inverseAdder((SpaceTime)umlgNode);
break;
case god:
this.god.inverseAdder((God)umlgNode);
break;
case nonNavigableMany:
this.nonNavigableMany.inverseAdder((NonNavigableMany)umlgNode);
break;
case nonNavigableOne:
this.nonNavigableOne.inverseAdder((NonNavigableOne)umlgNode);
break;
case angel:
this.angel.inverseAdder((Angel)umlgNode);
break;
case demon:
this.demon.inverseAdder((Demon)umlgNode);
break;
}
return runtimeProperty;
} else {
return null;
}
}
}
@Override
public boolean isTinkerRoot() {
return false;
}
public UmlgSet lookupFor_universe_angel() {
UmlgSet result = new UmlgMemorySet();
Filter filter = new Filter() {
@Override
public boolean filter(Angel entity){
return entity.getUniverse() == null;
}
};
result.addAll(org.umlg.concretetest.Angel.allInstances(filter));
return result;
}
public UmlgSet lookupFor_universe_demon() {
UmlgSet result = new UmlgMemorySet();
Filter filter = new Filter() {
@Override
public boolean filter(Demon entity){
return entity.getUniverse() == null;
}
};
result.addAll(org.umlg.concretetest.Demon.allInstances(filter));
return result;
}
public UmlgSet lookupFor_universe_nonNavigableMany() {
UmlgSet result = new UmlgMemorySet();
Filter filter = new Filter() {
@Override
public boolean filter(NonNavigableMany entity){
return entity.getUniverse() == null;
}
};
result.addAll(org.umlg.navigability.NonNavigableMany.allInstances(filter));
return result;
}
public UmlgSet lookupFor_universe_nonNavigableOne() {
UmlgSet result = new UmlgMemorySet();
Filter filter = new Filter() {
@Override
public boolean filter(NonNavigableOne entity){
return entity.z_internal_getUniverse() == null;
}
};
result.addAll(org.umlg.navigability.NonNavigableOne.allInstances(filter));
return result;
}
public void removeFromAngel(Angel angel) {
if ( angel != null ) {
this.angel.remove(angel);
}
}
public void removeFromAngel(UmlgSet angel) {
if ( !angel.isEmpty() ) {
this.angel.removeAll(angel);
}
}
public void removeFromDemon(Demon demon) {
if ( demon != null ) {
this.demon.remove(demon);
}
}
public void removeFromDemon(UmlgSet demon) {
if ( !demon.isEmpty() ) {
this.demon.removeAll(demon);
}
}
public void removeFromEmail(String email) {
if ( email != null ) {
this.email.remove(email);
}
}
public void removeFromEmail(UmlgSet email) {
if ( !email.isEmpty() ) {
this.email.removeAll(email);
}
}
public void removeFromGod(God god) {
if ( god != null ) {
this.god.remove(god);
}
}
public void removeFromGod(UmlgSet god) {
if ( !god.isEmpty() ) {
this.god.removeAll(god);
}
}
public void removeFromMax(Integer max) {
if ( max != null ) {
this.max.remove(max);
}
}
public void removeFromMax(UmlgSet max) {
if ( !max.isEmpty() ) {
this.max.removeAll(max);
}
}
public void removeFromMaxLength(String maxLength) {
if ( maxLength != null ) {
this.maxLength.remove(maxLength);
}
}
public void removeFromMaxLength(UmlgSet maxLength) {
if ( !maxLength.isEmpty() ) {
this.maxLength.removeAll(maxLength);
}
}
public void removeFromMin(Integer min) {
if ( min != null ) {
this.min.remove(min);
}
}
public void removeFromMin(UmlgSet min) {
if ( !min.isEmpty() ) {
this.min.removeAll(min);
}
}
public void removeFromName(String name) {
if ( name != null ) {
this.name.remove(name);
}
}
public void removeFromName(UmlgSet name) {
if ( !name.isEmpty() ) {
this.name.removeAll(name);
}
}
public void removeFromNonNavigableMany(NonNavigableMany nonNavigableMany) {
if ( nonNavigableMany != null ) {
this.nonNavigableMany.remove(nonNavigableMany);
}
}
public void removeFromNonNavigableMany(UmlgSet nonNavigableMany) {
if ( !nonNavigableMany.isEmpty() ) {
this.nonNavigableMany.removeAll(nonNavigableMany);
}
}
public void removeFromNonNavigableOne(NonNavigableOne nonNavigableOne) {
if ( nonNavigableOne != null ) {
this.nonNavigableOne.remove(nonNavigableOne);
}
}
public void removeFromNonNavigableOne(UmlgSet nonNavigableOne) {
if ( !nonNavigableOne.isEmpty() ) {
this.nonNavigableOne.removeAll(nonNavigableOne);
}
}
public void removeFromRange(Integer range) {
if ( range != null ) {
this.range.remove(range);
}
}
public void removeFromRange(UmlgSet range) {
if ( !range.isEmpty() ) {
this.range.removeAll(range);
}
}
public void removeFromRangeLength(String rangeLength) {
if ( rangeLength != null ) {
this.rangeLength.remove(rangeLength);
}
}
public void removeFromRangeLength(UmlgSet rangeLength) {
if ( !rangeLength.isEmpty() ) {
this.rangeLength.removeAll(rangeLength);
}
}
public void removeFromSpaceTime(SpaceTime spaceTime) {
if ( spaceTime != null ) {
this.spaceTime.remove(spaceTime);
}
}
public void removeFromSpaceTime(UmlgSet spaceTime) {
if ( !spaceTime.isEmpty() ) {
this.spaceTime.removeAll(spaceTime);
}
}
public void removeFromTestMinLength(String testMinLength) {
if ( testMinLength != null ) {
this.testMinLength.remove(testMinLength);
}
}
public void removeFromTestMinLength(UmlgSet testMinLength) {
if ( !testMinLength.isEmpty() ) {
this.testMinLength.removeAll(testMinLength);
}
}
public void setAngel(Angel angel) {
if ( angel != null ) {
angel.clearUniverse();
angel.initialiseProperty(AngelRuntimePropertyEnum.universe, false, true);
}
clearAngel();
addToAngel(angel);
}
public void setDemon(UmlgSet demon) {
clearDemon();
if ( demon != null ) {
addToDemon(demon);
}
}
public void setEmail(String email) {
if ( email == null ) {
clearEmail();
} else {
z_internalClearEmail();
}
addToEmail(email);
}
public void setGod(God god) {
clearGod();
addToGod(god);
}
public void setMax(Integer max) {
if ( max == null ) {
clearMax();
} else {
z_internalClearMax();
}
addToMax(max);
}
public void setMaxLength(String maxLength) {
if ( maxLength == null ) {
clearMaxLength();
} else {
z_internalClearMaxLength();
}
addToMaxLength(maxLength);
}
public void setMin(Integer min) {
if ( min == null ) {
clearMin();
} else {
z_internalClearMin();
}
addToMin(min);
}
public void setName(String name) {
if ( name == null ) {
clearName();
} else {
z_internalClearName();
}
addToName(name);
}
public void setNonNavigableMany(UmlgSet nonNavigableMany) {
clearNonNavigableMany();
if ( nonNavigableMany != null ) {
addToNonNavigableMany(nonNavigableMany);
}
}
public void setNonNavigableOne(NonNavigableOne nonNavigableOne) {
if ( nonNavigableOne != null ) {
nonNavigableOne.clearUniverse();
nonNavigableOne.initialiseProperty(NonNavigableOneRuntimePropertyEnum.universe, false, true);
}
clearNonNavigableOne();
addToNonNavigableOne(nonNavigableOne);
}
public void setRange(Integer range) {
if ( range == null ) {
clearRange();
} else {
z_internalClearRange();
}
addToRange(range);
}
public void setRangeLength(String rangeLength) {
if ( rangeLength == null ) {
clearRangeLength();
} else {
z_internalClearRangeLength();
}
addToRangeLength(rangeLength);
}
public void setSpaceTime(SpaceTime spaceTime) {
if ( spaceTime != null ) {
spaceTime.clearUniverse();
spaceTime.initialiseProperty(SpaceTimeRuntimePropertyEnum.universe, false, true);
}
clearSpaceTime();
addToSpaceTime(spaceTime);
}
public void setTestMinLength(String testMinLength) {
if ( testMinLength == null ) {
clearTestMinLength();
} else {
z_internalClearTestMinLength();
}
addToTestMinLength(testMinLength);
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJson(Boolean deep) {
String result = super.toJson(deep);
result = result.substring(1, result.length() - 1);
StringBuilder sb = new StringBuilder(result);
sb.append(", ");
if ( deep ) {
sb.append("\"spaceTime\": " + (getSpaceTime() == null ? "null" : getSpaceTime().toJson(true)) + "");
} else {
sb.delete(sb.length() - 2, sb.length());
}
sb.append(", ");
sb.append("\"rangeLength\": " + (getRangeLength() != null ? "\"" + StringEscapeUtils.escapeJson(getRangeLength()) + "\"" : null ));
sb.append(", ");
sb.append("\"range\": " + getRange() + "");
sb.append(", ");
sb.append("\"name\": " + (getName() != null ? "\"" + StringEscapeUtils.escapeJson(getName()) + "\"" : null ));
sb.append(", ");
sb.append("\"maxLength\": " + (getMaxLength() != null ? "\"" + StringEscapeUtils.escapeJson(getMaxLength()) + "\"" : null ));
sb.append(", ");
sb.append("\"testMinLength\": " + (getTestMinLength() != null ? "\"" + StringEscapeUtils.escapeJson(getTestMinLength()) + "\"" : null ));
sb.append(", ");
if ( getGod() != null ) {
if ( UmlgTmpIdManager.INSTANCE.get(getGod().getId()) != null ) {
sb.append("\"god\": " + "{\"id\": \"" + getGod().getId() + "\", \"tmpId\": \"" + UmlgTmpIdManager.INSTANCE.get(getGod().getId()) + "\",\"displayName\": \"" + getGod().getName() + "\"}" + "");
} else {
sb.append("\"god\": " + "{\"id\": \"" + getGod().getId() + "\", \"displayName\": \"" + getGod().getName() + "\"}" + "");
}
} else {
sb.append("\"god\": " + "{\"id\": " + null + ", \"displayName\": " + null + "}");
}
sb.append(", ");
if ( getNonNavigableOne() != null ) {
if ( UmlgTmpIdManager.INSTANCE.get(getNonNavigableOne().getId()) != null ) {
sb.append("\"nonNavigableOne\": " + "{\"id\": \"" + getNonNavigableOne().getId() + "\", \"tmpId\": \"" + UmlgTmpIdManager.INSTANCE.get(getNonNavigableOne().getId()) + "\",\"displayName\": \"" + getNonNavigableOne().getName() + "\"}" + "");
} else {
sb.append("\"nonNavigableOne\": " + "{\"id\": \"" + getNonNavigableOne().getId() + "\", \"displayName\": \"" + getNonNavigableOne().getName() + "\"}" + "");
}
} else {
sb.append("\"nonNavigableOne\": " + "{\"id\": " + null + ", \"displayName\": " + null + "}");
}
sb.append(", ");
if ( getAngel() != null ) {
if ( UmlgTmpIdManager.INSTANCE.get(getAngel().getId()) != null ) {
sb.append("\"angel\": " + "{\"id\": \"" + getAngel().getId() + "\", \"tmpId\": \"" + UmlgTmpIdManager.INSTANCE.get(getAngel().getId()) + "\",\"displayName\": \"" + getAngel().getName() + "\"}" + "");
} else {
sb.append("\"angel\": " + "{\"id\": \"" + getAngel().getId() + "\", \"displayName\": \"" + getAngel().getName() + "\"}" + "");
}
} else {
sb.append("\"angel\": " + "{\"id\": " + null + ", \"displayName\": " + null + "}");
}
sb.append(", ");
sb.append("\"min\": " + getMin() + "");
sb.append(", ");
sb.append("\"email\": " + (getEmail() != null ? "\"" + getEmail() + "\"" : null ));
sb.append(", ");
sb.append("\"max\": " + getMax() + "");
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJson() {
return toJson(false);
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJsonWithoutCompositeParent(Boolean deep) {
String result = super.toJsonWithoutCompositeParent(deep);
result = result.substring(1, result.length() - 1);
StringBuilder sb = new StringBuilder(result);
sb.append(", ");
if ( deep ) {
sb.append("\"spaceTime\": " + (getSpaceTime() == null ? "null" : getSpaceTime().toJsonWithoutCompositeParent(true)) + "");
} else {
sb.delete(sb.length() - 2, sb.length());
}
sb.append(", ");
sb.append("\"rangeLength\": " + (getRangeLength() != null ? "\"" + StringEscapeUtils.escapeJson(getRangeLength()) + "\"" : null ));
sb.append(", ");
sb.append("\"range\": " + getRange() + "");
sb.append(", ");
sb.append("\"name\": " + (getName() != null ? "\"" + StringEscapeUtils.escapeJson(getName()) + "\"" : null ));
sb.append(", ");
sb.append("\"maxLength\": " + (getMaxLength() != null ? "\"" + StringEscapeUtils.escapeJson(getMaxLength()) + "\"" : null ));
sb.append(", ");
sb.append("\"testMinLength\": " + (getTestMinLength() != null ? "\"" + StringEscapeUtils.escapeJson(getTestMinLength()) + "\"" : null ));
sb.append(", ");
if ( getNonNavigableOne() != null ) {
if ( UmlgTmpIdManager.INSTANCE.get(getNonNavigableOne().getId()) != null ) {
sb.append("\"nonNavigableOne\": " + "{\"id\": \"" + getNonNavigableOne().getId() + "\", \"tmpId\": \"" + UmlgTmpIdManager.INSTANCE.get(getNonNavigableOne().getId()) + "\",\"displayName\": \"" + getNonNavigableOne().getName() + "\"}" + "");
} else {
sb.append("\"nonNavigableOne\": " + "{\"id\": \"" + getNonNavigableOne().getId() + "\", \"displayName\": \"" + getNonNavigableOne().getName() + "\"}" + "");
}
} else {
sb.append("\"nonNavigableOne\": " + "{\"id\": " + null + ", \"displayName\": " + null + "}");
}
sb.append(", ");
if ( getAngel() != null ) {
if ( UmlgTmpIdManager.INSTANCE.get(getAngel().getId()) != null ) {
sb.append("\"angel\": " + "{\"id\": \"" + getAngel().getId() + "\", \"tmpId\": \"" + UmlgTmpIdManager.INSTANCE.get(getAngel().getId()) + "\",\"displayName\": \"" + getAngel().getName() + "\"}" + "");
} else {
sb.append("\"angel\": " + "{\"id\": \"" + getAngel().getId() + "\", \"displayName\": \"" + getAngel().getName() + "\"}" + "");
}
} else {
sb.append("\"angel\": " + "{\"id\": " + null + ", \"displayName\": " + null + "}");
}
sb.append(", ");
sb.append("\"min\": " + getMin() + "");
sb.append(", ");
sb.append("\"email\": " + (getEmail() != null ? "\"" + getEmail() + "\"" : null ));
sb.append(", ");
sb.append("\"max\": " + getMax() + "");
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJsonWithoutCompositeParent() {
return toJsonWithoutCompositeParent(false);
}
public List validateEmail(String email) {
List result = new ArrayList();
if ( !UmlgValidator.validateEmail(email) ) {
result.add(new UmlgConstraintViolation("Email", "umlgtest::org::umlg::concretetest::Universe::email", "Email does not pass validation!"));
}
return result;
}
public List validateMax(Integer max) {
List result = new ArrayList();
if ( !UmlgValidator.validateMaxInteger(max, 100) ) {
result.add(new UmlgConstraintViolation("MaxInteger", "umlgtest::org::umlg::concretetest::Universe::max", "MaxInteger does not pass validation!"));
}
return result;
}
public List validateMaxLength(String maxLength) {
List result = new ArrayList();
if ( !UmlgValidator.validateMaxLength(maxLength, 10) ) {
result.add(new UmlgConstraintViolation("MaxLength", "umlgtest::org::umlg::concretetest::Universe::maxLength", "MaxLength does not pass validation!"));
}
return result;
}
public List validateMin(Integer min) {
List result = new ArrayList();
if ( !UmlgValidator.validateMinInteger(min, 10) ) {
result.add(new UmlgConstraintViolation("MinInteger", "umlgtest::org::umlg::concretetest::Universe::min", "MinInteger does not pass validation!"));
}
return result;
}
@Override
public List validateMultiplicities() {
List result = new ArrayList();
if ( getSpaceTime() == null ) {
result.add(new UmlgConstraintViolation("multiplicity", "umlgtest::org::umlg::concretetest::Universe::spaceTime", "lower multiplicity is 1"));
}
if ( getUpdatedOn() == null ) {
result.add(new UmlgConstraintViolation("multiplicity", "umlglib::org::umlg::BaseModelUmlg::updatedOn", "lower multiplicity is 1"));
}
if ( getGod() == null ) {
result.add(new UmlgConstraintViolation("multiplicity", "umlgtest::org::umlg::concretetest::A__::god", "lower multiplicity is 1"));
}
if ( getCreatedOn() == null ) {
result.add(new UmlgConstraintViolation("multiplicity", "umlglib::org::umlg::BaseModelUmlg::createdOn", "lower multiplicity is 1"));
}
return result;
}
public List validateName(String name) {
List result = new ArrayList();
return result;
}
public List validateRange(Integer range) {
List result = new ArrayList();
if ( !UmlgValidator.validateRangeInteger(range, 2, 4) ) {
result.add(new UmlgConstraintViolation("RangeInteger", "umlgtest::org::umlg::concretetest::Universe::range", "RangeInteger does not pass validation!"));
}
return result;
}
public List validateRangeLength(String rangeLength) {
List result = new ArrayList();
if ( !UmlgValidator.validateRangeLength(rangeLength, 5, 10) ) {
result.add(new UmlgConstraintViolation("RangeLength", "umlgtest::org::umlg::concretetest::Universe::rangeLength", "RangeLength does not pass validation!"));
}
return result;
}
public List validateTestMinLength(String testMinLength) {
List result = new ArrayList();
if ( !UmlgValidator.validateMinLength(testMinLength, 5) ) {
result.add(new UmlgConstraintViolation("MinLength", "umlgtest::org::umlg::concretetest::Universe::testMinLength", "MinLength does not pass validation!"));
}
return result;
}
@Override
public void z_internalAddPersistentValueToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
UniverseRuntimePropertyEnum runtimeProperty;
super.z_internalAddPersistentValueToCollection(umlgRuntimeProperty, umlgNode);
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case testMinLength:
this.testMinLength.z_internalAdder((String)umlgNode);
break;
case spaceTime:
this.spaceTime.z_internalAdder((SpaceTime)umlgNode);
break;
case rangeLength:
this.rangeLength.z_internalAdder((String)umlgNode);
break;
case max:
this.max.z_internalAdder((int)umlgNode);
break;
case demon:
this.demon.z_internalAdder((Demon)umlgNode);
break;
case angel:
this.angel.z_internalAdder((Angel)umlgNode);
break;
case range:
this.range.z_internalAdder((int)umlgNode);
break;
case maxLength:
this.maxLength.z_internalAdder((String)umlgNode);
break;
case god:
this.god.z_internalAdder((God)umlgNode);
break;
case nonNavigableMany:
this.nonNavigableMany.z_internalAdder((NonNavigableMany)umlgNode);
break;
case min:
this.min.z_internalAdder((int)umlgNode);
break;
case email:
this.email.z_internalAdder(UmlgFormatter.parse(UniverseRuntimePropertyEnum.email.getDataTypeEnum(), umlgNode));
break;
case name:
this.name.z_internalAdder((String)umlgNode);
break;
case nonNavigableOne:
this.nonNavigableOne.z_internalAdder((NonNavigableOne)umlgNode);
break;
}
}
}
@Override
public void z_internalAddToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
UniverseRuntimePropertyEnum runtimeProperty;
super.z_internalAddToCollection(umlgRuntimeProperty, umlgNode);
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case range:
this.range.z_internalAdder((Integer)umlgNode);
break;
case spaceTime:
this.spaceTime.z_internalAdder((SpaceTime)umlgNode);
break;
case rangeLength:
this.rangeLength.z_internalAdder((String)umlgNode);
break;
case maxLength:
this.maxLength.z_internalAdder((String)umlgNode);
break;
case testMinLength:
this.testMinLength.z_internalAdder((String)umlgNode);
break;
case god:
this.god.z_internalAdder((God)umlgNode);
break;
case nonNavigableMany:
this.nonNavigableMany.z_internalAdder((NonNavigableMany)umlgNode);
break;
case max:
this.max.z_internalAdder((Integer)umlgNode);
break;
case min:
this.min.z_internalAdder((Integer)umlgNode);
break;
case email:
this.email.z_internalAdder((String)umlgNode);
break;
case demon:
this.demon.z_internalAdder((Demon)umlgNode);
break;
case name:
this.name.z_internalAdder((String)umlgNode);
break;
case nonNavigableOne:
this.nonNavigableOne.z_internalAdder((NonNavigableOne)umlgNode);
break;
case angel:
this.angel.z_internalAdder((Angel)umlgNode);
break;
}
}
}
@Override
public Set z_internalBooleanProperties() {
Set result = super.z_internalBooleanProperties();
return result;
}
public void z_internalClearAngel() {
this.angel.z_internalClear();
}
public void z_internalClearDemon() {
this.demon.z_internalClear();
}
public void z_internalClearEmail() {
this.email.z_internalClear();
}
public void z_internalClearGod() {
this.god.z_internalClear();
}
public void z_internalClearMax() {
this.max.z_internalClear();
}
public void z_internalClearMaxLength() {
this.maxLength.z_internalClear();
}
public void z_internalClearMin() {
this.min.z_internalClear();
}
public void z_internalClearName() {
this.name.z_internalClear();
}
public void z_internalClearNonNavigableMany() {
this.nonNavigableMany.z_internalClear();
}
public void z_internalClearNonNavigableOne() {
this.nonNavigableOne.z_internalClear();
}
public void z_internalClearRange() {
this.range.z_internalClear();
}
public void z_internalClearRangeLength() {
this.rangeLength.z_internalClear();
}
public void z_internalClearSpaceTime() {
this.spaceTime.z_internalClear();
}
public void z_internalClearTestMinLength() {
this.testMinLength.z_internalClear();
}
@Override
public Set z_internalDataTypeProperties() {
Set result = super.z_internalDataTypeProperties();
result.add(UniverseRuntimePropertyEnum.rangeLength);
result.add(UniverseRuntimePropertyEnum.range);
result.add(UniverseRuntimePropertyEnum.maxLength);
result.add(UniverseRuntimePropertyEnum.testMinLength);
result.add(UniverseRuntimePropertyEnum.min);
result.add(UniverseRuntimePropertyEnum.email);
result.add(UniverseRuntimePropertyEnum.name);
result.add(UniverseRuntimePropertyEnum.max);
return result;
}
@Override
public Map z_internalDataTypePropertiesWithDefaultValues() {
Map result = super.z_internalDataTypePropertiesWithDefaultValues();
return result;
}
@Override
public UmlgCollection extends Object> z_internalGetCollectionFor(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse) {
UmlgCollection extends Object> result = null;
result = super.z_internalGetCollectionFor(tumlRuntimeProperty, inverse);
if ( result == null ) {
UniverseRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case range:
result = this.range;
break;
case spaceTime:
result = this.spaceTime;
break;
case rangeLength:
result = this.rangeLength;
break;
case maxLength:
result = this.maxLength;
break;
case angel:
result = this.angel;
break;
case testMinLength:
result = this.testMinLength;
break;
case god:
result = this.god;
break;
case nonNavigableMany:
result = this.nonNavigableMany;
break;
case demon:
result = this.demon;
break;
case min:
result = this.min;
break;
case email:
result = this.email;
break;
case max:
result = this.max;
break;
case name:
result = this.name;
break;
case nonNavigableOne:
result = this.nonNavigableOne;
break;
}
}
}
return result;
}
@Override
public UmlgRuntimeProperty z_internalInverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
UmlgRuntimeProperty fromSuperRuntimeProperty = super.inverseAdder(tumlRuntimeProperty, inverse, umlgNode);
UniverseRuntimePropertyEnum runtimeProperty;
if ( fromSuperRuntimeProperty != null ) {
return fromSuperRuntimeProperty;
} else {
if ( !inverse ) {
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case spaceTime:
this.spaceTime.z_internalAdder((SpaceTime)umlgNode);
break;
case god:
this.god.z_internalAdder((God)umlgNode);
break;
case nonNavigableMany:
this.nonNavigableMany.z_internalAdder((NonNavigableMany)umlgNode);
break;
case nonNavigableOne:
this.nonNavigableOne.z_internalAdder((NonNavigableOne)umlgNode);
break;
case angel:
this.angel.z_internalAdder((Angel)umlgNode);
break;
case demon:
this.demon.z_internalAdder((Demon)umlgNode);
break;
}
return runtimeProperty;
} else {
return null;
}
}
}
@Override
public void z_internalMarkCollectionLoaded(UmlgRuntimeProperty umlgRuntimeProperty, boolean loaded) {
UniverseRuntimePropertyEnum runtimeProperty;
super.z_internalMarkCollectionLoaded(umlgRuntimeProperty, loaded);
runtimeProperty = (UniverseRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case testMinLength:
this.testMinLength.setLoaded(loaded);
break;
case spaceTime:
this.spaceTime.setLoaded(loaded);
break;
case rangeLength:
this.rangeLength.setLoaded(loaded);
break;
case demon:
this.demon.setLoaded(loaded);
break;
case range:
this.range.setLoaded(loaded);
break;
case maxLength:
this.maxLength.setLoaded(loaded);
break;
case angel:
this.angel.setLoaded(loaded);
break;
case god:
this.god.setLoaded(loaded);
break;
case nonNavigableMany:
this.nonNavigableMany.setLoaded(loaded);
break;
case max:
this.max.setLoaded(loaded);
break;
case min:
this.min.setLoaded(loaded);
break;
case email:
this.email.setLoaded(loaded);
break;
case name:
this.name.setLoaded(loaded);
break;
case nonNavigableOne:
this.nonNavigableOne.setLoaded(loaded);
break;
}
}
}
static public enum UniverseRuntimePropertyEnum implements UmlgRuntimeProperty {
spaceTime(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::spaceTime",/* persistentName */ "spaceTime",/* inverseName */ "universe",/* inverseQualifiedName */ "umlgtest::org::umlg::componenttest::A__::universe",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ true,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("A__"),/* isOneToOne */ true,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ 1,/* lower */ 1,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ SpaceTime.class,/* json */ "{\"name\": \"spaceTime\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Universe::spaceTime\", \"persistentName\": \"spaceTime\", \"inverseName\": \"universe\", \"inverseQualifiedName\": \"umlgtest::org::umlg::componenttest::A__::universe\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": true, \"inverseComposite\": false, \"oneToOne\": true, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": false, \"upper\": 1, \"lower\": 1, \"inverseUpper\": 1, \"label\": \"A__\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
rangeLength(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::rangeLength",/* persistentName */ "rangeLength",/* inverseName */ "inverseOf::rangeLength",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Universe::rangeLength",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new RangeLength(5, 10)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("rangeLength"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ String.class,/* json */ "{\"name\": \"rangeLength\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"rangeLength\": {\"min\": 5, \"max\": 10}}, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Universe::rangeLength\", \"persistentName\": \"rangeLength\", \"inverseName\": \"inverseOf::rangeLength\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::concretetest::Universe::rangeLength\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"rangeLength\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
range(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::range",/* persistentName */ "range",/* inverseName */ "inverseOf::range",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Universe::range",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new RangeInteger(2, 4)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("range"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"range\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"range\": {\"min\": 2, \"max\": 4}}, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Universe::range\", \"persistentName\": \"range\", \"inverseName\": \"inverseOf::range\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::concretetest::Universe::range\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"range\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
maxLength(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::maxLength",/* persistentName */ "maxLength",/* inverseName */ "inverseOf::maxLength",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Universe::maxLength",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MaxLength(10)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("maxLength"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ String.class,/* json */ "{\"name\": \"maxLength\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"maxLength\": 10}, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Universe::maxLength\", \"persistentName\": \"maxLength\", \"inverseName\": \"inverseOf::maxLength\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::concretetest::Universe::maxLength\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"maxLength\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
testMinLength(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::testMinLength",/* persistentName */ "testMinLength",/* inverseName */ "inverseOf::testMinLength",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Universe::testMinLength",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MinLength(5)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("testMinLength"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ String.class,/* json */ "{\"name\": \"testMinLength\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"minLength\": 5}, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Universe::testMinLength\", \"persistentName\": \"testMinLength\", \"inverseName\": \"inverseOf::testMinLength\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::concretetest::Universe::testMinLength\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"testMinLength\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
updatedOn(/* qualifiedName */ "umlglib::org::umlg::BaseModelUmlg::updatedOn",/* persistentName */ "updatedOn",/* inverseName */ "inverseOf::updatedOn",/* inverseQualifiedName */ "inverseOf::umlglib::org::umlg::BaseModelUmlg::updatedOn",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ DataTypeEnum.DateTime,/* validations */ Arrays.asList(new DateTimeValidation()),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("updatedOn"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 1,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ LocalDateTime.class,/* json */ "{\"name\": \"updatedOn\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": \"" + DataTypeEnum.DateTime.toString() + "\", \"validations\": {\"dateTime\": {}}, \"qualifiedName\": \"umlglib::org::umlg::BaseModelUmlg::updatedOn\", \"persistentName\": \"updatedOn\", \"inverseName\": \"inverseOf::updatedOn\", \"inverseQualifiedName\": \"inverseOf::umlglib::org::umlg::BaseModelUmlg::updatedOn\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 1, \"inverseUpper\": 1, \"label\": \"updatedOn\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
god(/* qualifiedName */ "umlgtest::org::umlg::concretetest::A__::god",/* persistentName */ "god",/* inverseName */ "universe",/* inverseQualifiedName */ "umlgtest::org::umlg::concretetest::God::universe",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ false,/* isComposite */ false,/* isInverseComposite */ true,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("A__"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 1,/* inverseUpper */ -1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ God.class,/* json */ "{\"name\": \"god\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::A__::god\", \"persistentName\": \"god\", \"inverseName\": \"universe\", \"inverseQualifiedName\": \"umlgtest::org::umlg::concretetest::God::universe\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": false, \"composite\": false, \"inverseComposite\": true, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 1, \"inverseUpper\": -1, \"label\": \"A__\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
nonNavigableMany(/* qualifiedName */ "umlgtest::org::umlg::navigability::A__::nonNavigableMany",/* persistentName */ "nonNavigableMany",/* inverseName */ "universe",/* inverseQualifiedName */ "umlgtest::org::umlg::navigability::A__::universe",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("A__"),/* isOneToOne */ false,/* isOneToMany */ true,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ NonNavigableMany.class,/* json */ "{\"name\": \"nonNavigableMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::navigability::A__::nonNavigableMany\", \"persistentName\": \"nonNavigableMany\", \"inverseName\": \"universe\", \"inverseQualifiedName\": \"umlgtest::org::umlg::navigability::A__::universe\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": true, \"manyToOne\": false, \"manyToMany\": false, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"A__\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
min(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::min",/* persistentName */ "min",/* inverseName */ "inverseOf::min",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Universe::min",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MinInteger(10)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("min"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"min\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"min\": 10}, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Universe::min\", \"persistentName\": \"min\", \"inverseName\": \"inverseOf::min\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::concretetest::Universe::min\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"min\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
email(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::email",/* persistentName */ "email",/* inverseName */ "inverseOf::email",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Universe::email",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ DataTypeEnum.Email,/* validations */ Arrays.asList(new Email()),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("email"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ String.class,/* json */ "{\"name\": \"email\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": \"" + DataTypeEnum.Email.toString() + "\", \"validations\": {\"email\": {}}, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Universe::email\", \"persistentName\": \"email\", \"inverseName\": \"inverseOf::email\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::concretetest::Universe::email\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"email\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
createdOn(/* qualifiedName */ "umlglib::org::umlg::BaseModelUmlg::createdOn",/* persistentName */ "createdOn",/* inverseName */ "inverseOf::createdOn",/* inverseQualifiedName */ "inverseOf::umlglib::org::umlg::BaseModelUmlg::createdOn",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ DataTypeEnum.DateTime,/* validations */ Arrays.asList(new DateTimeValidation()),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("createdOn"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 1,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ LocalDateTime.class,/* json */ "{\"name\": \"createdOn\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": \"" + DataTypeEnum.DateTime.toString() + "\", \"validations\": {\"dateTime\": {}}, \"qualifiedName\": \"umlglib::org::umlg::BaseModelUmlg::createdOn\", \"persistentName\": \"createdOn\", \"inverseName\": \"inverseOf::createdOn\", \"inverseQualifiedName\": \"inverseOf::umlglib::org::umlg::BaseModelUmlg::createdOn\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 1, \"inverseUpper\": 1, \"label\": \"createdOn\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
name(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::name",/* persistentName */ "name",/* inverseName */ "inverseOf::name",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Universe::name",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("name"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ String.class,/* json */ "{\"name\": \"name\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::Universe::name\", \"persistentName\": \"name\", \"inverseName\": \"inverseOf::name\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::concretetest::Universe::name\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"name\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
nonNavigableOne(/* qualifiedName */ "umlgtest::org::umlg::navigability::A__::nonNavigableOne",/* persistentName */ "nonNavigableOne",/* inverseName */ "universe",/* inverseQualifiedName */ "umlgtest::org::umlg::navigability::A__::universe",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ false,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("A__"),/* isOneToOne */ true,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ NonNavigableOne.class,/* json */ "{\"name\": \"nonNavigableOne\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::navigability::A__::nonNavigableOne\", \"persistentName\": \"nonNavigableOne\", \"inverseName\": \"universe\", \"inverseQualifiedName\": \"umlgtest::org::umlg::navigability::A__::universe\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": false, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": true, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"A__\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
angel(/* qualifiedName */ "umlgtest::org::umlg::concretetest::A__::angel",/* persistentName */ "angel",/* inverseName */ "universe",/* inverseQualifiedName */ "umlgtest::org::umlg::concretetest::A__::universe",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ false,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("A__"),/* isOneToOne */ true,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Angel.class,/* json */ "{\"name\": \"angel\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::concretetest::A__::angel\", \"persistentName\": \"angel\", \"inverseName\": \"universe\", \"inverseQualifiedName\": \"umlgtest::org::umlg::concretetest::A__::universe\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": false, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": true, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"A__\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
tag(/* qualifiedName */ "umlglib::org::umlg::tag::tag_baseUmlg_1::tag",/* persistentName */ "tag",/* inverseName */ "baseUmlg",/* inverseQualifiedName */ "umlglib::org::umlg::tag::Tag::baseUmlg",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ false,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("tag_baseUmlg_1"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ -1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Tag.class,/* json */ "{\"name\": \"tag\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlglib::org::umlg::tag::tag_baseUmlg_1::tag\", \"persistentName\": \"tag\", \"inverseName\": \"baseUmlg\", \"inverseQualifiedName\": \"umlglib::org::umlg::tag::Tag::baseUmlg\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": false, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": -1, \"label\": \"tag_baseUmlg_1\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
max(/* qualifiedName */ "umlgtest::org::umlg::concretetest::Universe::max",/* persistentName */ "max",/* inverseName */ "inverseOf::max",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::concretetest::Universe::max",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.