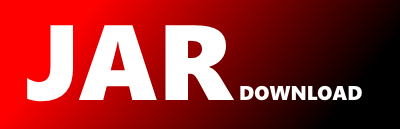
org.umlg.model.IndexCreator Maven / Gradle / Ivy
The newest version!
package org.umlg.model;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import org.apache.tinkerpop.gremlin.structure.Edge;
import org.apache.tinkerpop.gremlin.structure.Vertex;
import org.umlg.associationclass.HalfHourMeasurement;
import org.umlg.associationclass.HourMeasurement;
import org.umlg.collectiontest.Fantasy;
import org.umlg.collectiontest.Foot;
import org.umlg.collectiontest.Nightmare;
import org.umlg.indexing.PTYPE;
import org.umlg.indexing.Product;
import org.umlg.ocl.qualifier.OclQualifierB;
import org.umlg.ocl.qualifier.OclQualifierC;
import org.umlg.qualifiertest.BBQUalifierBBB;
import org.umlg.qualifiertest.BBQualifierBB;
import org.umlg.qualifiertest.BQualifierB;
import org.umlg.qualifiertest.BQualifierC;
import org.umlg.qualifiertest.BQualifierD;
import org.umlg.qualifiertest.ConcreteQ1;
import org.umlg.qualifiertest.ConcreteQ2;
import org.umlg.qualifiertest.ENUM1;
import org.umlg.qualifiertest.Many1;
import org.umlg.qualifiertest.Many2;
import org.umlg.qualifiertest.Nature;
import org.umlg.qualifiertest.QualifierB;
import org.umlg.qualifiertest.QualifierD;
import org.umlg.rootallinstances.BaseRoot;
import org.umlg.rootallinstances.MiddleRoot;
import org.umlg.rootallinstances.TopRoot;
import org.umlg.runtime.adaptor.UMLG;
import org.umlg.runtime.adaptor.UmlgGraph;
import org.umlg.runtime.adaptor.UmlgIndexManager;
import org.umlg.runtime.adaptor.UmlgParameter;
/** This class is responsible to create all keyed indexes.
* It is invoked via reflection the first time a graph is created.
*/
public class IndexCreator implements UmlgIndexManager {
public void createIndexes() {
UMLG.get().createKeyIndex("name2", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Nature"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Foot"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Fantasy"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Nightmare"));
UMLG.get().createKeyIndex("name1", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Nature"));
UMLG.get().createKeyIndex("nameUnique", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Nature"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Many1"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Many2"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Many1"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Many2"));
UMLG.get().createKeyIndex("name1", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "QualifierB"));
UMLG.get().createKeyIndex("name2", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "QualifierB"));
UMLG.get().createKeyIndex("int1", Vertex.class, new UmlgParameter>("unusedIndexValueType", Integer.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "QualifierB"));
UMLG.get().createKeyIndex("int2", Vertex.class, new UmlgParameter>("unusedIndexValueType", Integer.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "QualifierB"));
UMLG.get().createKeyIndex("int3", Vertex.class, new UmlgParameter>("unusedIndexValueType", Integer.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "QualifierB"));
UMLG.get().createKeyIndex("date", Vertex.class, new UmlgParameter>("unusedIndexValueType", LocalDate.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "QualifierD"));
UMLG.get().createKeyIndex("eNUM1", Vertex.class, new UmlgParameter>("unusedIndexValueType", ENUM1.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "QualifierD"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BQualifierB"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BQualifierC"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BQualifierD"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BQualifierB"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BQualifierC"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BQualifierD"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "ConcreteQ1"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "ConcreteQ2"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BBQUalifierBBB"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BBQualifierBB"));
UMLG.get().createKeyIndex("parameterName", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BBQUalifierBBB"));
UMLG.get().createKeyIndex("parameterName", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BBQualifierBB"));
UMLG.get().createKeyIndex("nameNonUnique", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Nightmare"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "OclQualifierB"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "OclQualifierC"));
UMLG.get().createKeyIndex(UmlgGraph.ROOT_VERTEX, Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "ROOT_VERTEX"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "BaseRoot"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "MiddleRoot"));
UMLG.get().createKeyIndex("name", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("nameUnique", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "BaseRoot"));
UMLG.get().createKeyIndex("nameUnique", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "MiddleRoot"));
UMLG.get().createKeyIndex("nameUnique", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexedName", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexedNonUniqueName", Vertex.class, new UmlgParameter>("unusedIndexValueType", String.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexUniqueInteger", Vertex.class, new UmlgParameter>("unusedIndexValueType", Integer.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexNonUniqueInteger", Vertex.class, new UmlgParameter>("unusedIndexValueType", Integer.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexUniqueUnlimitedNatural", Vertex.class, new UmlgParameter>("unusedIndexValueType", Integer.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexNonUniqueUnlimitedNatural", Vertex.class, new UmlgParameter>("unusedIndexValueType", Integer.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexUniqueReal", Vertex.class, new UmlgParameter>("unusedIndexValueType", Double.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexNonUniqueReal", Vertex.class, new UmlgParameter>("unusedIndexValueType", Double.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("indexNonUniqueBoolean", Vertex.class, new UmlgParameter>("unusedIndexValueType", Boolean.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "TopRoot"));
UMLG.get().createKeyIndex("aggregated", Vertex.class, new UmlgParameter>("unusedIndexValueType", Boolean.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "HalfHourMeasurement"));
UMLG.get().createKeyIndex("aggregated", Vertex.class, new UmlgParameter>("unusedIndexValueType", Boolean.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "HourMeasurement"));
UMLG.get().createKeyIndex("created", Vertex.class, new UmlgParameter>("unusedIndexValueType", LocalDate.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Product"));
UMLG.get().createKeyIndex("deleted", Vertex.class, new UmlgParameter>("unusedIndexValueType", LocalDateTime.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "Product"));
UMLG.get().createKeyIndex("time", Vertex.class, new UmlgParameter>("unusedIndexValueType", LocalTime.class), new UmlgParameter("unusedUniqueorNot", true), new UmlgParameter("unusedLabel", "Product"));
UMLG.get().createKeyIndex("type", Vertex.class, new UmlgParameter>("unusedIndexValueType", PTYPE.class), new UmlgParameter("unusedUniqueorNot", false), new UmlgParameter("unusedLabel", "Product"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy