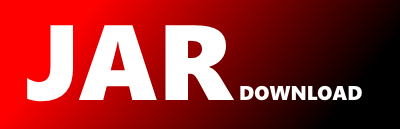
org.umlg.ocl.ocloperator.OclExists1 Maven / Gradle / Ivy
The newest version!
package org.umlg.ocl.ocloperator;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
import org.apache.commons.text.StringEscapeUtils;
import org.apache.tinkerpop.gremlin.structure.Vertex;
import org.umlg.ocl.ocloperator.OclExists2.OclExists2RuntimePropertyEnum;
import org.umlg.runtime.adaptor.TransactionThreadEntityVar;
import org.umlg.runtime.adaptor.UMLG;
import org.umlg.runtime.adaptor.UmlgLabelConverterFactory;
import org.umlg.runtime.adaptor.UmlgTmpIdManager;
import org.umlg.runtime.collection.Filter;
import org.umlg.runtime.collection.Qualifier;
import org.umlg.runtime.collection.UmlgCollection;
import org.umlg.runtime.collection.UmlgRuntimeProperty;
import org.umlg.runtime.collection.UmlgSet;
import org.umlg.runtime.collection.memory.UmlgMemorySet;
import org.umlg.runtime.collection.ocl.*;
import org.umlg.runtime.collection.persistent.PropertyTree;
import org.umlg.runtime.collection.persistent.UmlgSetImpl;
import org.umlg.runtime.domain.BaseUmlgCompositionNode;
import org.umlg.runtime.domain.CompositionNode;
import org.umlg.runtime.domain.DataTypeEnum;
import org.umlg.runtime.domain.UmlgNode;
import org.umlg.runtime.domain.UmlgRootNode;
import org.umlg.runtime.util.ObjectMapperFactory;
import org.umlg.runtime.util.UmlgFormatter;
import org.umlg.runtime.validation.UmlgConstraintViolation;
import org.umlg.runtime.validation.UmlgConstraintViolationException;
import org.umlg.runtime.validation.UmlgValidation;
public class OclExists1 extends BaseUmlgCompositionNode implements UmlgRootNode, CompositionNode {
static final public long serialVersionUID = 1L;
private UmlgSet oclExists2;
private UmlgSet name;
private String tmpId; // tmpId is only used the umlg restlet gui. It is never persisted. Its value is generated by the gui.
/**
* constructor for OclExists1
*
* @param id
*/
public OclExists1(Object id) {
super(id);
}
/**
* constructor for OclExists1
*
* @param vertex
*/
public OclExists1(Vertex vertex) {
super(vertex);
}
/**
* default constructor for OclExists1
*/
public OclExists1() {
this(true);
}
/**
* constructor for OclExists1
*
* @param persistent
*/
public OclExists1(Boolean persistent) {
super(persistent);
}
public void addToName(String name) {
if ( !this.name.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( name != null ) {
List violations = validateName(name);
if ( violations.isEmpty() ) {
this.name.add(name);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToNameIgnoreInverse(String name) {
if ( !this.name.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( name != null ) {
List violations = validateName(name);
if ( violations.isEmpty() ) {
this.name.add(name);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToOclExists2(OclExists2 oclExists2) {
if ( oclExists2 != null ) {
oclExists2.clearOclExists1();
oclExists2.initialiseProperty(OclExists2RuntimePropertyEnum.oclExists1, false, true);
removeFromOclExists2(oclExists2);
}
if ( oclExists2 != null ) {
this.oclExists2.add(oclExists2);
}
}
public void addToOclExists2(UmlgSet oclExists2) {
if ( !oclExists2.isEmpty() ) {
this.oclExists2.addAll(oclExists2);
}
}
public void addToOclExists2IgnoreInverse(OclExists2 oclExists2) {
if ( oclExists2 != null ) {
oclExists2.clearOclExists1();
oclExists2.initialiseProperty(OclExists2RuntimePropertyEnum.oclExists1, false, true);
removeFromOclExists2(oclExists2);
}
if ( oclExists2 != null ) {
this.oclExists2.addIgnoreInverse(oclExists2);
}
}
static public UmlgSet extends OclExists1> allInstances(Filter filter) {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(OclExists1.class.getName(), filter));
return result;
}
static public UmlgSet extends OclExists1> allInstances() {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(OclExists1.class.getName()));
return result;
}
/**
* Implements the ocl statement for constraint 'ExistsConstraint'
*
* package umlgtest::org::umlg::ocl::ocloperator
* context OclExists1 inv:
* self.oclExists2->exists(name='joe')
* endpackage
*
*/
public List checkClassConstraintExistsConstraint() {
List result = new ArrayList();
if ( (getOclExists2().exists(temp1 -> temp1.getName().equals("joe"))) == false ) {
result.add(new UmlgConstraintViolation("ExistsConstraint", "umlgtest::org::umlg::ocl::ocloperator::OclExists1", "ocl\npackage umlgtest::org::umlg::ocl::ocloperator\n context OclExists1 inv:\n self.oclExists2->exists(name='joe')\nendpackage\nfails!"));
}
return result;
}
@Override
public List checkClassConstraints() {
List result = new ArrayList();
result.addAll(checkClassConstraintExistsConstraint());
return result;
}
public void clearName() {
this.name.clear();
}
public void clearOclExists2() {
this.oclExists2.clear();
}
@Override
public void delete() {
for ( OclExists2 child : getOclExists2() ) {
child.delete();
}
TransactionThreadEntityVar.remove(this);
this.vertex.remove();
}
@Override
public void fromJson(Map propertyMap) {
fromJsonDataTypeAndComposite(propertyMap);
fromJsonNonCompositeOne(propertyMap);
fromJsonNonCompositeRequiredMany(propertyMap);
}
@Override
public void fromJson(String json) {
ObjectMapper mapper = ObjectMapperFactory.INSTANCE.getObjectMapper();
try {
@SuppressWarnings( "unchecked")
Map propertyMap = mapper.readValue(json, Map.class);
fromJson(propertyMap);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Override
public void fromJsonDataTypeAndComposite(Map propertyMap) {
if ( propertyMap.containsKey("name") ) {
if ( propertyMap.get("name") != null ) {
String name = (String)propertyMap.get("name");
setName(name);
} else {
setName(null);
}
}
if ( propertyMap.containsKey("tmpId") ) {
if ( propertyMap.get("tmpId") != null ) {
this.tmpId = (String)propertyMap.get("tmpId");
UmlgTmpIdManager.INSTANCE.put(this.tmpId, getId());
} else {
this.tmpId = null;
}
}
}
@Override
public void fromJsonNonCompositeOne(Map propertyMap) {
}
@Override
public void fromJsonNonCompositeRequiredMany(Map propertyMap) {
}
@Override
public String getMetaDataAsJson() {
return OclExists1.OclExists1RuntimePropertyEnum.asJson();
}
public String getName() {
UmlgSet tmp = this.name;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public UmlgSet getOclExists2() {
return this.oclExists2;
}
@Override
public UmlgNode getOwningObject() {
return null;
}
@Override
public String getQualifiedName() {
return "umlgtest::org::umlg::ocl::ocloperator::OclExists1";
}
/**
* getQualifiers is called from the collection in order to update the index used to implement the qualifier
*
* @param tumlRuntimeProperty
* @param node
* @param inverse
*/
@Override
public List getQualifiers(UmlgRuntimeProperty tumlRuntimeProperty, UmlgNode node, boolean inverse) {
List result = Collections.emptyList();
OclExists1RuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result.isEmpty() ) {
switch ( runtimeProperty ) {
default:
result = Collections.emptyList();
}
}
return result;
}
/**
* getSize is called from the BaseCollection.addInternal in order to save the size of the inverse collection to update the edge's sequence order
*
* @param inverse
* @param tumlRuntimeProperty
*/
@Override
public int getSize(boolean inverse, UmlgRuntimeProperty tumlRuntimeProperty) {
int result = 0;
OclExists1RuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result == 0 ) {
switch ( runtimeProperty ) {
case name:
result = name.size();
break;
case oclExists2:
result = oclExists2.size();
break;
default:
result = 0;
}
}
return result;
}
@Override
public String getUid() {
String uid;
if ( !this.vertex.property("uid").isPresent() && this.vertex.property("uid").value() != null ) {
uid=UUID.randomUUID().toString();
this.vertex.property("uid", uid);
} else {
uid=this.vertex.value("uid");
}
return uid;
}
@Override
public boolean hasOnlyOneCompositeParent() {
int result = 0;
return result == 1;
}
public void initDataTypeVariablesWithDefaultValues() {
}
public void initVariables() {
}
/**
* boolean properties' default values are initialized in the constructor via z_internalBooleanProperties
*
* @param loaded
*/
@Override
public void initialiseProperties(boolean loaded) {
this.name = new UmlgSetImpl(this, PropertyTree.from(OclExists1RuntimePropertyEnum.name), loaded);
this.oclExists2 = new UmlgSetImpl(this, PropertyTree.from(OclExists1RuntimePropertyEnum.oclExists2), loaded);
}
@Override
public void initialiseProperty(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, boolean loaded) {
OclExists1RuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
this.name = new UmlgSetImpl(this, PropertyTree.from(OclExists1RuntimePropertyEnum.name), loaded);
break;
case oclExists2:
this.oclExists2 = new UmlgSetImpl(this, PropertyTree.from(OclExists1RuntimePropertyEnum.oclExists2), loaded);
break;
}
}
}
@Override
public UmlgRuntimeProperty inverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
OclExists1RuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case oclExists2:
this.oclExists2.inverseAdder((OclExists2)umlgNode);
break;
}
return runtimeProperty;
} else {
return null;
}
}
@Override
public boolean isTinkerRoot() {
return true;
}
public void removeFromName(String name) {
if ( name != null ) {
this.name.remove(name);
}
}
public void removeFromName(UmlgSet name) {
if ( !name.isEmpty() ) {
this.name.removeAll(name);
}
}
public void removeFromOclExists2(OclExists2 oclExists2) {
if ( oclExists2 != null ) {
this.oclExists2.remove(oclExists2);
}
}
public void removeFromOclExists2(UmlgSet oclExists2) {
if ( !oclExists2.isEmpty() ) {
this.oclExists2.removeAll(oclExists2);
}
}
public void setName(String name) {
if ( name == null ) {
clearName();
} else {
z_internalClearName();
}
addToName(name);
}
public void setOclExists2(UmlgSet oclExists2) {
clearOclExists2();
if ( oclExists2 != null ) {
addToOclExists2(oclExists2);
}
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJson(Boolean deep) {
StringBuilder sb = new StringBuilder();
if ( this.tmpId != null ) {
sb.append("\"tmpId\": \"" + this.tmpId + "\", ");
}
sb.append("\"id\": \"" + getId() + "\", ");
sb.append("\"name\": " + (getName() != null ? "\"" + StringEscapeUtils.escapeJson(getName()) + "\"" : null ));
sb.append(", ");
sb.append("\"qualifiedName\": \"" + getQualifiedName() + "\"");
sb.append(", ");
//PlaceHolder for restful
sb.append("\"uri\": {}");
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJson() {
return toJson(false);
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJsonWithoutCompositeParent(Boolean deep) {
StringBuilder sb = new StringBuilder();
if ( this.tmpId != null ) {
sb.append("\"tmpId\": \"" + this.tmpId + "\", ");
}
sb.append("\"id\": \"" + getId() + "\", ");
sb.append("\"name\": " + (getName() != null ? "\"" + StringEscapeUtils.escapeJson(getName()) + "\"" : null ));
sb.append(", ");
sb.append("\"qualifiedName\": \"" + getQualifiedName() + "\"");
sb.append(", ");
//PlaceHolder for restful
sb.append("\"uri\": {}");
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJsonWithoutCompositeParent() {
return toJsonWithoutCompositeParent(false);
}
@Override
public List validateMultiplicities() {
List result = new ArrayList();
if ( getName() == null ) {
result.add(new UmlgConstraintViolation("multiplicity", "umlgtest::org::umlg::ocl::ocloperator::OclExists1::name", "lower multiplicity is 1"));
}
return result;
}
public List validateName(String name) {
List result = new ArrayList();
return result;
}
@Override
public void z_internalAddPersistentValueToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
OclExists1RuntimePropertyEnum runtimeProperty;
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
this.name.z_internalAdder((String)umlgNode);
break;
case oclExists2:
this.oclExists2.z_internalAdder((OclExists2)umlgNode);
break;
}
}
}
@Override
public void z_internalAddToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
OclExists1RuntimePropertyEnum runtimeProperty;
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
this.name.z_internalAdder((String)umlgNode);
break;
case oclExists2:
this.oclExists2.z_internalAdder((OclExists2)umlgNode);
break;
}
}
}
@Override
public Set z_internalBooleanProperties() {
Set result = new HashSet();
return result;
}
public void z_internalClearName() {
this.name.z_internalClear();
}
public void z_internalClearOclExists2() {
this.oclExists2.z_internalClear();
}
@Override
public Set z_internalDataTypeProperties() {
Set result = new HashSet();
result.add(OclExists1RuntimePropertyEnum.name);
return result;
}
@Override
public Map z_internalDataTypePropertiesWithDefaultValues() {
Map result = new HashMap();
return result;
}
@Override
public UmlgCollection extends Object> z_internalGetCollectionFor(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse) {
UmlgCollection extends Object> result = null;
if ( result == null ) {
OclExists1RuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
result = this.name;
break;
case oclExists2:
result = this.oclExists2;
break;
}
}
}
return result;
}
@Override
public UmlgRuntimeProperty z_internalInverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
OclExists1RuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case oclExists2:
this.oclExists2.z_internalAdder((OclExists2)umlgNode);
break;
}
return runtimeProperty;
} else {
return null;
}
}
@Override
public void z_internalMarkCollectionLoaded(UmlgRuntimeProperty umlgRuntimeProperty, boolean loaded) {
OclExists1RuntimePropertyEnum runtimeProperty;
runtimeProperty = (OclExists1RuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case name:
this.name.setLoaded(loaded);
break;
case oclExists2:
this.oclExists2.setLoaded(loaded);
break;
}
}
}
static public enum OclExists1RuntimePropertyEnum implements UmlgRuntimeProperty {
name(/* qualifiedName */ "umlgtest::org::umlg::ocl::ocloperator::OclExists1::name",/* persistentName */ "name",/* inverseName */ "inverseOf::name",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::ocl::ocloperator::OclExists1::name",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("name"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 1,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ String.class,/* json */ "{\"name\": \"name\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::ocl::ocloperator::OclExists1::name\", \"persistentName\": \"name\", \"inverseName\": \"inverseOf::name\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::ocl::ocloperator::OclExists1::name\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 1, \"inverseUpper\": 1, \"label\": \"name\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
oclExists2(/* qualifiedName */ "umlgtest::org::umlg::ocl::ocloperator::OclExists1::oclExists2",/* persistentName */ "oclExists2",/* inverseName */ "oclExists1",/* inverseQualifiedName */ "umlgtest::org::umlg::ocl::ocloperator::A_oclExists1_oclExists2_1::oclExists1",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ true,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("A_oclExists1_oclExists2_1"),/* isOneToOne */ false,/* isOneToMany */ true,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ true,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ OclExists2.class,/* json */ "{\"name\": \"oclExists2\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::ocl::ocloperator::OclExists1::oclExists2\", \"persistentName\": \"oclExists2\", \"inverseName\": \"oclExists1\", \"inverseQualifiedName\": \"umlgtest::org::umlg::ocl::ocloperator::A_oclExists1_oclExists2_1::oclExists1\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": true, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": true, \"manyToOne\": false, \"manyToMany\": false, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"A_oclExists1_oclExists2_1\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": true, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
umlgtest(/* qualifiedName */ "umlgtest",/* persistentName */ "umlgtest",/* inverseName */ "inverseOfumlgtest",/* inverseQualifiedName */ "inverseOfumlgtest",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ true,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("rootOclExists1"),/* isOneToOne */ true,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ false,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ false,/* propertyType */ Object.class,/* json */ "{\"name\": \"umlgtest\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest\", \"persistentName\": \"umlgtest\", \"inverseName\": \"inverseOfumlgtest\", \"inverseQualifiedName\": \"inverseOfumlgtest\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": true, \"oneToOne\": true, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": false, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"rootOclExists1\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": false, \"inverseUnique\": false, \"derived\": false, \"navigable\": false}",/* isChangeListenerAttribute */ false);
private String _qualifiedName;
private String _persistentName;
private String _inverseName;
private String _inverseQualifiedName;
private boolean _associationClassOne;
private boolean _memberEndOfAssociationClass;
private String _associationClassPropertyName;
private String _inverseAssociationClassPropertyName;
private boolean _associationClassProperty;
private boolean _onePrimitivePropertyOfAssociationClass;
private boolean _onePrimitive;
private Boolean _readOnly;
private DataTypeEnum dataTypeEnum;
private List validations;
private boolean _manyPrimitive;
private boolean _oneEnumeration;
private boolean _manyEnumeration;
private boolean _controllingSide;
private boolean _composite;
private boolean _inverseComposite;
private String _label;
private boolean _oneToOne;
private boolean _oneToMany;
private boolean _manyToOne;
private boolean _manyToMany;
private int _upper;
private int _lower;
private int _inverseUpper;
private boolean _qualified;
private boolean _inverseQualified;
private boolean _ordered;
private boolean _inverseOrdered;
private boolean _unique;
private boolean _inverseUnique;
private boolean _derived;
private boolean _navigability;
private Class _propertyType;
private String _json;
private boolean _changeListener;
/**
* constructor for OclExists1RuntimePropertyEnum
*
* @param _qualifiedName
* @param _persistentName
* @param _inverseName
* @param _inverseQualifiedName
* @param _associationClassOne
* @param _memberEndOfAssociationClass
* @param _associationClassPropertyName
* @param _inverseAssociationClassPropertyName
* @param _associationClassProperty
* @param _onePrimitivePropertyOfAssociationClass
* @param _onePrimitive
* @param _readOnly
* @param dataTypeEnum
* @param validations
* @param _manyPrimitive
* @param _oneEnumeration
* @param _manyEnumeration
* @param _controllingSide
* @param _composite
* @param _inverseComposite
* @param _label
* @param _oneToOne
* @param _oneToMany
* @param _manyToOne
* @param _manyToMany
* @param _upper
* @param _lower
* @param _inverseUpper
* @param _qualified
* @param _inverseQualified
* @param _ordered
* @param _inverseOrdered
* @param _unique
* @param _inverseUnique
* @param _derived
* @param _navigability
* @param _propertyType
* @param _json
* @param _changeListener
*/
private OclExists1RuntimePropertyEnum(String _qualifiedName, String _persistentName, String _inverseName, String _inverseQualifiedName, boolean _associationClassOne, boolean _memberEndOfAssociationClass, String _associationClassPropertyName, String _inverseAssociationClassPropertyName, boolean _associationClassProperty, boolean _onePrimitivePropertyOfAssociationClass, boolean _onePrimitive, Boolean _readOnly, DataTypeEnum dataTypeEnum, List validations, boolean _manyPrimitive, boolean _oneEnumeration, boolean _manyEnumeration, boolean _controllingSide, boolean _composite, boolean _inverseComposite, String _label, boolean _oneToOne, boolean _oneToMany, boolean _manyToOne, boolean _manyToMany, int _upper, int _lower, int _inverseUpper, boolean _qualified, boolean _inverseQualified, boolean _ordered, boolean _inverseOrdered, boolean _unique, boolean _inverseUnique, boolean _derived, boolean _navigability, Class _propertyType, String _json, boolean _changeListener) {
this._qualifiedName = _qualifiedName;
this._persistentName = _persistentName;
this._inverseName = _inverseName;
this._inverseQualifiedName = _inverseQualifiedName;
this._associationClassOne = _associationClassOne;
this._memberEndOfAssociationClass = _memberEndOfAssociationClass;
this._associationClassPropertyName = _associationClassPropertyName;
this._inverseAssociationClassPropertyName = _inverseAssociationClassPropertyName;
this._associationClassProperty = _associationClassProperty;
this._onePrimitivePropertyOfAssociationClass = _onePrimitivePropertyOfAssociationClass;
this._onePrimitive = _onePrimitive;
this._readOnly = _readOnly;
this.dataTypeEnum = dataTypeEnum;
this.validations = validations;
this._manyPrimitive = _manyPrimitive;
this._oneEnumeration = _oneEnumeration;
this._manyEnumeration = _manyEnumeration;
this._controllingSide = _controllingSide;
this._composite = _composite;
this._inverseComposite = _inverseComposite;
this._label = _label;
this._oneToOne = _oneToOne;
this._oneToMany = _oneToMany;
this._manyToOne = _manyToOne;
this._manyToMany = _manyToMany;
this._upper = _upper;
this._lower = _lower;
this._inverseUpper = _inverseUpper;
this._qualified = _qualified;
this._inverseQualified = _inverseQualified;
this._ordered = _ordered;
this._inverseOrdered = _inverseOrdered;
this._unique = _unique;
this._inverseUnique = _inverseUnique;
this._derived = _derived;
this._navigability = _navigability;
this._propertyType = _propertyType;
this._json = _json;
this._changeListener = _changeListener;
}
static public String asJson() {
int count = 1;
StringBuilder sb = new StringBuilder();;
sb.append("{\"name\": \"OclExists1\", ");
sb.append("\"qualifiedName\": \"umlgtest::org::umlg::ocl::ocloperator::OclExists1\", ");
sb.append("\"uri\": \"TODO\", ");
sb.append("\"properties\": [");
for ( OclExists1RuntimePropertyEnum l : OclExists1RuntimePropertyEnum.values() ) {
sb.append(l.toJson());
if ( count < OclExists1RuntimePropertyEnum.values().length ) {
count++;
sb.append(",");
}
}
sb.append("]}");
return sb.toString();
}
static public OclExists1RuntimePropertyEnum fromInverseQualifiedName(String inverseQualifiedName) {
if ( umlgtest.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return umlgtest;
}
if ( oclExists2.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return oclExists2;
}
if ( name.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return name;
}
return null;
}
static public OclExists1RuntimePropertyEnum fromLabel(String _label) {
if ( umlgtest.getLabel().equals(_label) ) {
return umlgtest;
}
if ( oclExists2.getLabel().equals(_label) ) {
return oclExists2;
}
if ( name.getLabel().equals(_label) ) {
return name;
}
return null;
}
static public OclExists1RuntimePropertyEnum fromQualifiedName(String qualifiedName) {
if ( umlgtest.getQualifiedName().equals(qualifiedName) ) {
return umlgtest;
}
if ( oclExists2.getQualifiedName().equals(qualifiedName) ) {
return oclExists2;
}
if ( name.getQualifiedName().equals(qualifiedName) ) {
return name;
}
return null;
}
public String getAssociationClassPropertyName() {
return this._associationClassPropertyName;
}
public DataTypeEnum getDataTypeEnum() {
return this.dataTypeEnum;
}
public String getInverseAssociationClassPropertyName() {
return this._inverseAssociationClassPropertyName;
}
public String getInverseName() {
return this._inverseName;
}
public String getInverseQualifiedName() {
return this._inverseQualifiedName;
}
public int getInverseUpper() {
return this._inverseUpper;
}
public String getJson() {
return this._json;
}
public String getLabel() {
return this._label;
}
public int getLower() {
return this._lower;
}
public String getPersistentName() {
return this._persistentName;
}
public Class getPropertyType() {
return this._propertyType;
}
public String getQualifiedName() {
return this._qualifiedName;
}
public Boolean getReadOnly() {
return this._readOnly;
}
public int getUpper() {
return this._upper;
}
public List getValidations() {
return this.validations;
}
public boolean isAssociationClassOne() {
return this._associationClassOne;
}
public boolean isAssociationClassProperty() {
return this._associationClassProperty;
}
public boolean isChangeListener() {
return this._changeListener;
}
public boolean isComposite() {
return this._composite;
}
public boolean isControllingSide() {
return this._controllingSide;
}
public boolean isDerived() {
return this._derived;
}
public boolean isInverseComposite() {
return this._inverseComposite;
}
public boolean isInverseOrdered() {
return this._inverseOrdered;
}
public boolean isInverseQualified() {
return this._inverseQualified;
}
public boolean isInverseUnique() {
return this._inverseUnique;
}
public boolean isManyEnumeration() {
return this._manyEnumeration;
}
public boolean isManyPrimitive() {
return this._manyPrimitive;
}
public boolean isManyToMany() {
return this._manyToMany;
}
public boolean isManyToOne() {
return this._manyToOne;
}
public boolean isMemberEndOfAssociationClass() {
return this._memberEndOfAssociationClass;
}
public boolean isNavigability() {
return this._navigability;
}
public boolean isOneEnumeration() {
return this._oneEnumeration;
}
public boolean isOnePrimitive() {
return this._onePrimitive;
}
public boolean isOnePrimitivePropertyOfAssociationClass() {
return this._onePrimitivePropertyOfAssociationClass;
}
public boolean isOneToMany() {
return this._oneToMany;
}
public boolean isOneToOne() {
return this._oneToOne;
}
public boolean isOrdered() {
return this._ordered;
}
public boolean isQualified() {
return this._qualified;
}
public boolean isUnique() {
return this._unique;
}
@Override
public boolean isValid(int elementCount) {
if ( isQualified() ) {
return elementCount >= getLower();
} else {
return (getUpper() == -1 || elementCount <= getUpper()) && elementCount >= getLower();
}
}
@Override
public String toJson() {
return getJson();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy