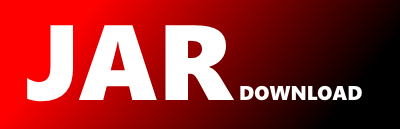
org.umlg.validation.TestManyValidation Maven / Gradle / Ivy
The newest version!
package org.umlg.validation;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
import org.apache.commons.text.StringEscapeUtils;
import org.apache.tinkerpop.gremlin.structure.Vertex;
import org.umlg.runtime.adaptor.TransactionThreadEntityVar;
import org.umlg.runtime.adaptor.UMLG;
import org.umlg.runtime.adaptor.UmlgLabelConverterFactory;
import org.umlg.runtime.adaptor.UmlgTmpIdManager;
import org.umlg.runtime.collection.Filter;
import org.umlg.runtime.collection.Qualifier;
import org.umlg.runtime.collection.UmlgCollection;
import org.umlg.runtime.collection.UmlgRuntimeProperty;
import org.umlg.runtime.collection.UmlgSet;
import org.umlg.runtime.collection.memory.UmlgMemorySet;
import org.umlg.runtime.collection.persistent.PropertyTree;
import org.umlg.runtime.collection.persistent.UmlgSetImpl;
import org.umlg.runtime.domain.BaseUmlgCompositionNode;
import org.umlg.runtime.domain.CompositionNode;
import org.umlg.runtime.domain.DataTypeEnum;
import org.umlg.runtime.domain.UmlgNode;
import org.umlg.runtime.domain.UmlgRootNode;
import org.umlg.runtime.domain.json.ToJsonUtil;
import org.umlg.runtime.util.ObjectMapperFactory;
import org.umlg.runtime.util.UmlgFormatter;
import org.umlg.runtime.validation.MaxInteger;
import org.umlg.runtime.validation.MaxReal;
import org.umlg.runtime.validation.MaxUnlimitedNatural;
import org.umlg.runtime.validation.MinInteger;
import org.umlg.runtime.validation.MinReal;
import org.umlg.runtime.validation.MinUnlimitedNatural;
import org.umlg.runtime.validation.RangeInteger;
import org.umlg.runtime.validation.RangeReal;
import org.umlg.runtime.validation.RangeUnlimitedNatural;
import org.umlg.runtime.validation.UmlgConstraintViolation;
import org.umlg.runtime.validation.UmlgConstraintViolationException;
import org.umlg.runtime.validation.UmlgValidation;
import org.umlg.runtime.validation.UmlgValidator;
public class TestManyValidation extends BaseUmlgCompositionNode implements UmlgRootNode, CompositionNode {
static final public long serialVersionUID = 1L;
private UmlgSet aMaxRealMany;
private UmlgSet aMinRealMany;
private UmlgSet aRangeRealMany;
private UmlgSet aMaxUnlimitedNaturalMany;
private UmlgSet aMinUnlimitedNaturalMany;
private UmlgSet aRangeUnlimitedNaturalMany;
private UmlgSet aMaxIntegerMany;
private UmlgSet aMinIntegerMany;
private UmlgSet aRangeIntegerMany;
private String tmpId; // tmpId is only used the umlg restlet gui. It is never persisted. Its value is generated by the gui.
/**
* constructor for TestManyValidation
*
* @param id
*/
public TestManyValidation(Object id) {
super(id);
}
/**
* constructor for TestManyValidation
*
* @param vertex
*/
public TestManyValidation(Vertex vertex) {
super(vertex);
}
/**
* default constructor for TestManyValidation
*/
public TestManyValidation() {
this(true);
}
/**
* constructor for TestManyValidation
*
* @param persistent
*/
public TestManyValidation(Boolean persistent) {
super(persistent);
}
public void addToAMaxIntegerMany(Integer aMaxIntegerMany) {
if ( aMaxIntegerMany != null ) {
List violations = validateAMaxIntegerMany(aMaxIntegerMany);
if ( violations.isEmpty() ) {
this.aMaxIntegerMany.add(aMaxIntegerMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxIntegerMany(UmlgSet aMaxIntegerMany) {
if ( !aMaxIntegerMany.isEmpty() ) {
this.aMaxIntegerMany.addAll(aMaxIntegerMany);
}
}
public void addToAMaxIntegerManyIgnoreInverse(Integer aMaxIntegerMany) {
if ( aMaxIntegerMany != null ) {
List violations = validateAMaxIntegerMany(aMaxIntegerMany);
if ( violations.isEmpty() ) {
this.aMaxIntegerMany.addIgnoreInverse(aMaxIntegerMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxRealMany(Double aMaxRealMany) {
if ( aMaxRealMany != null ) {
List violations = validateAMaxRealMany(aMaxRealMany);
if ( violations.isEmpty() ) {
this.aMaxRealMany.add(aMaxRealMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxRealMany(UmlgSet aMaxRealMany) {
if ( !aMaxRealMany.isEmpty() ) {
this.aMaxRealMany.addAll(aMaxRealMany);
}
}
public void addToAMaxRealManyIgnoreInverse(Double aMaxRealMany) {
if ( aMaxRealMany != null ) {
List violations = validateAMaxRealMany(aMaxRealMany);
if ( violations.isEmpty() ) {
this.aMaxRealMany.addIgnoreInverse(aMaxRealMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxUnlimitedNaturalMany(Integer aMaxUnlimitedNaturalMany) {
if ( aMaxUnlimitedNaturalMany != null ) {
List violations = validateAMaxUnlimitedNaturalMany(aMaxUnlimitedNaturalMany);
if ( violations.isEmpty() ) {
this.aMaxUnlimitedNaturalMany.add(aMaxUnlimitedNaturalMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxUnlimitedNaturalMany(UmlgSet aMaxUnlimitedNaturalMany) {
if ( !aMaxUnlimitedNaturalMany.isEmpty() ) {
this.aMaxUnlimitedNaturalMany.addAll(aMaxUnlimitedNaturalMany);
}
}
public void addToAMaxUnlimitedNaturalManyIgnoreInverse(Integer aMaxUnlimitedNaturalMany) {
if ( aMaxUnlimitedNaturalMany != null ) {
List violations = validateAMaxUnlimitedNaturalMany(aMaxUnlimitedNaturalMany);
if ( violations.isEmpty() ) {
this.aMaxUnlimitedNaturalMany.addIgnoreInverse(aMaxUnlimitedNaturalMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinIntegerMany(Integer aMinIntegerMany) {
if ( aMinIntegerMany != null ) {
List violations = validateAMinIntegerMany(aMinIntegerMany);
if ( violations.isEmpty() ) {
this.aMinIntegerMany.add(aMinIntegerMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinIntegerMany(UmlgSet aMinIntegerMany) {
if ( !aMinIntegerMany.isEmpty() ) {
this.aMinIntegerMany.addAll(aMinIntegerMany);
}
}
public void addToAMinIntegerManyIgnoreInverse(Integer aMinIntegerMany) {
if ( aMinIntegerMany != null ) {
List violations = validateAMinIntegerMany(aMinIntegerMany);
if ( violations.isEmpty() ) {
this.aMinIntegerMany.addIgnoreInverse(aMinIntegerMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinRealMany(Double aMinRealMany) {
if ( aMinRealMany != null ) {
List violations = validateAMinRealMany(aMinRealMany);
if ( violations.isEmpty() ) {
this.aMinRealMany.add(aMinRealMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinRealMany(UmlgSet aMinRealMany) {
if ( !aMinRealMany.isEmpty() ) {
this.aMinRealMany.addAll(aMinRealMany);
}
}
public void addToAMinRealManyIgnoreInverse(Double aMinRealMany) {
if ( aMinRealMany != null ) {
List violations = validateAMinRealMany(aMinRealMany);
if ( violations.isEmpty() ) {
this.aMinRealMany.addIgnoreInverse(aMinRealMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinUnlimitedNaturalMany(Integer aMinUnlimitedNaturalMany) {
if ( aMinUnlimitedNaturalMany != null ) {
List violations = validateAMinUnlimitedNaturalMany(aMinUnlimitedNaturalMany);
if ( violations.isEmpty() ) {
this.aMinUnlimitedNaturalMany.add(aMinUnlimitedNaturalMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinUnlimitedNaturalMany(UmlgSet aMinUnlimitedNaturalMany) {
if ( !aMinUnlimitedNaturalMany.isEmpty() ) {
this.aMinUnlimitedNaturalMany.addAll(aMinUnlimitedNaturalMany);
}
}
public void addToAMinUnlimitedNaturalManyIgnoreInverse(Integer aMinUnlimitedNaturalMany) {
if ( aMinUnlimitedNaturalMany != null ) {
List violations = validateAMinUnlimitedNaturalMany(aMinUnlimitedNaturalMany);
if ( violations.isEmpty() ) {
this.aMinUnlimitedNaturalMany.addIgnoreInverse(aMinUnlimitedNaturalMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeIntegerMany(Integer aRangeIntegerMany) {
if ( aRangeIntegerMany != null ) {
List violations = validateARangeIntegerMany(aRangeIntegerMany);
if ( violations.isEmpty() ) {
this.aRangeIntegerMany.add(aRangeIntegerMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeIntegerMany(UmlgSet aRangeIntegerMany) {
if ( !aRangeIntegerMany.isEmpty() ) {
this.aRangeIntegerMany.addAll(aRangeIntegerMany);
}
}
public void addToARangeIntegerManyIgnoreInverse(Integer aRangeIntegerMany) {
if ( aRangeIntegerMany != null ) {
List violations = validateARangeIntegerMany(aRangeIntegerMany);
if ( violations.isEmpty() ) {
this.aRangeIntegerMany.addIgnoreInverse(aRangeIntegerMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeRealMany(Double aRangeRealMany) {
if ( aRangeRealMany != null ) {
List violations = validateARangeRealMany(aRangeRealMany);
if ( violations.isEmpty() ) {
this.aRangeRealMany.add(aRangeRealMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeRealMany(UmlgSet aRangeRealMany) {
if ( !aRangeRealMany.isEmpty() ) {
this.aRangeRealMany.addAll(aRangeRealMany);
}
}
public void addToARangeRealManyIgnoreInverse(Double aRangeRealMany) {
if ( aRangeRealMany != null ) {
List violations = validateARangeRealMany(aRangeRealMany);
if ( violations.isEmpty() ) {
this.aRangeRealMany.addIgnoreInverse(aRangeRealMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeUnlimitedNaturalMany(Integer aRangeUnlimitedNaturalMany) {
if ( aRangeUnlimitedNaturalMany != null ) {
List violations = validateARangeUnlimitedNaturalMany(aRangeUnlimitedNaturalMany);
if ( violations.isEmpty() ) {
this.aRangeUnlimitedNaturalMany.add(aRangeUnlimitedNaturalMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeUnlimitedNaturalMany(UmlgSet aRangeUnlimitedNaturalMany) {
if ( !aRangeUnlimitedNaturalMany.isEmpty() ) {
this.aRangeUnlimitedNaturalMany.addAll(aRangeUnlimitedNaturalMany);
}
}
public void addToARangeUnlimitedNaturalManyIgnoreInverse(Integer aRangeUnlimitedNaturalMany) {
if ( aRangeUnlimitedNaturalMany != null ) {
List violations = validateARangeUnlimitedNaturalMany(aRangeUnlimitedNaturalMany);
if ( violations.isEmpty() ) {
this.aRangeUnlimitedNaturalMany.addIgnoreInverse(aRangeUnlimitedNaturalMany);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
static public UmlgSet extends TestManyValidation> allInstances(Filter filter) {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(TestManyValidation.class.getName(), filter));
return result;
}
static public UmlgSet extends TestManyValidation> allInstances() {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(TestManyValidation.class.getName()));
return result;
}
@Override
public List checkClassConstraints() {
List result = new ArrayList();
return result;
}
public void clearAMaxIntegerMany() {
this.aMaxIntegerMany.clear();
}
public void clearAMaxRealMany() {
this.aMaxRealMany.clear();
}
public void clearAMaxUnlimitedNaturalMany() {
this.aMaxUnlimitedNaturalMany.clear();
}
public void clearAMinIntegerMany() {
this.aMinIntegerMany.clear();
}
public void clearAMinRealMany() {
this.aMinRealMany.clear();
}
public void clearAMinUnlimitedNaturalMany() {
this.aMinUnlimitedNaturalMany.clear();
}
public void clearARangeIntegerMany() {
this.aRangeIntegerMany.clear();
}
public void clearARangeRealMany() {
this.aRangeRealMany.clear();
}
public void clearARangeUnlimitedNaturalMany() {
this.aRangeUnlimitedNaturalMany.clear();
}
@Override
public void delete() {
TransactionThreadEntityVar.remove(this);
this.vertex.remove();
}
@Override
public void fromJson(Map propertyMap) {
fromJsonDataTypeAndComposite(propertyMap);
fromJsonNonCompositeOne(propertyMap);
fromJsonNonCompositeRequiredMany(propertyMap);
}
@Override
public void fromJson(String json) {
ObjectMapper mapper = ObjectMapperFactory.INSTANCE.getObjectMapper();
try {
@SuppressWarnings( "unchecked")
Map propertyMap = mapper.readValue(json, Map.class);
fromJson(propertyMap);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Override
public void fromJsonDataTypeAndComposite(Map propertyMap) {
if ( propertyMap.containsKey("aMaxRealMany") ) {
if ( propertyMap.get("aMaxRealMany") != null ) {
UmlgSet aMaxRealMany = new UmlgMemorySet((Collection)propertyMap.get("aMaxRealMany"));
clearAMaxRealMany();
for ( Number value : aMaxRealMany ) {
addToAMaxRealMany(value.doubleValue());
}
} else {
setAMaxRealMany(null);
}
}
if ( propertyMap.containsKey("aMaxUnlimitedNaturalMany") ) {
if ( propertyMap.get("aMaxUnlimitedNaturalMany") != null ) {
UmlgSet aMaxUnlimitedNaturalMany = new UmlgMemorySet((Collection)propertyMap.get("aMaxUnlimitedNaturalMany"));
clearAMaxUnlimitedNaturalMany();
for ( Number value : aMaxUnlimitedNaturalMany ) {
addToAMaxUnlimitedNaturalMany(value.intValue());
}
} else {
setAMaxUnlimitedNaturalMany(null);
}
}
if ( propertyMap.containsKey("aMinUnlimitedNaturalMany") ) {
if ( propertyMap.get("aMinUnlimitedNaturalMany") != null ) {
UmlgSet aMinUnlimitedNaturalMany = new UmlgMemorySet((Collection)propertyMap.get("aMinUnlimitedNaturalMany"));
clearAMinUnlimitedNaturalMany();
for ( Number value : aMinUnlimitedNaturalMany ) {
addToAMinUnlimitedNaturalMany(value.intValue());
}
} else {
setAMinUnlimitedNaturalMany(null);
}
}
if ( propertyMap.containsKey("aRangeUnlimitedNaturalMany") ) {
if ( propertyMap.get("aRangeUnlimitedNaturalMany") != null ) {
UmlgSet aRangeUnlimitedNaturalMany = new UmlgMemorySet((Collection)propertyMap.get("aRangeUnlimitedNaturalMany"));
clearARangeUnlimitedNaturalMany();
for ( Number value : aRangeUnlimitedNaturalMany ) {
addToARangeUnlimitedNaturalMany(value.intValue());
}
} else {
setARangeUnlimitedNaturalMany(null);
}
}
if ( propertyMap.containsKey("aMaxIntegerMany") ) {
if ( propertyMap.get("aMaxIntegerMany") != null ) {
UmlgSet aMaxIntegerMany = new UmlgMemorySet((Collection)propertyMap.get("aMaxIntegerMany"));
clearAMaxIntegerMany();
for ( Number value : aMaxIntegerMany ) {
addToAMaxIntegerMany(value.intValue());
}
} else {
setAMaxIntegerMany(null);
}
}
if ( propertyMap.containsKey("aRangeRealMany") ) {
if ( propertyMap.get("aRangeRealMany") != null ) {
UmlgSet aRangeRealMany = new UmlgMemorySet((Collection)propertyMap.get("aRangeRealMany"));
clearARangeRealMany();
for ( Number value : aRangeRealMany ) {
addToARangeRealMany(value.doubleValue());
}
} else {
setARangeRealMany(null);
}
}
if ( propertyMap.containsKey("aRangeIntegerMany") ) {
if ( propertyMap.get("aRangeIntegerMany") != null ) {
UmlgSet aRangeIntegerMany = new UmlgMemorySet((Collection)propertyMap.get("aRangeIntegerMany"));
clearARangeIntegerMany();
for ( Number value : aRangeIntegerMany ) {
addToARangeIntegerMany(value.intValue());
}
} else {
setARangeIntegerMany(null);
}
}
if ( propertyMap.containsKey("aMinRealMany") ) {
if ( propertyMap.get("aMinRealMany") != null ) {
UmlgSet aMinRealMany = new UmlgMemorySet((Collection)propertyMap.get("aMinRealMany"));
clearAMinRealMany();
for ( Number value : aMinRealMany ) {
addToAMinRealMany(value.doubleValue());
}
} else {
setAMinRealMany(null);
}
}
if ( propertyMap.containsKey("aMinIntegerMany") ) {
if ( propertyMap.get("aMinIntegerMany") != null ) {
UmlgSet aMinIntegerMany = new UmlgMemorySet((Collection)propertyMap.get("aMinIntegerMany"));
clearAMinIntegerMany();
for ( Number value : aMinIntegerMany ) {
addToAMinIntegerMany(value.intValue());
}
} else {
setAMinIntegerMany(null);
}
}
if ( propertyMap.containsKey("tmpId") ) {
if ( propertyMap.get("tmpId") != null ) {
this.tmpId = (String)propertyMap.get("tmpId");
UmlgTmpIdManager.INSTANCE.put(this.tmpId, getId());
} else {
this.tmpId = null;
}
}
}
@Override
public void fromJsonNonCompositeOne(Map propertyMap) {
}
@Override
public void fromJsonNonCompositeRequiredMany(Map propertyMap) {
}
public UmlgSet getAMaxIntegerMany() {
return this.aMaxIntegerMany;
}
public UmlgSet getAMaxRealMany() {
return this.aMaxRealMany;
}
public UmlgSet getAMaxUnlimitedNaturalMany() {
return this.aMaxUnlimitedNaturalMany;
}
public UmlgSet getAMinIntegerMany() {
return this.aMinIntegerMany;
}
public UmlgSet getAMinRealMany() {
return this.aMinRealMany;
}
public UmlgSet getAMinUnlimitedNaturalMany() {
return this.aMinUnlimitedNaturalMany;
}
public UmlgSet getARangeIntegerMany() {
return this.aRangeIntegerMany;
}
public UmlgSet getARangeRealMany() {
return this.aRangeRealMany;
}
public UmlgSet getARangeUnlimitedNaturalMany() {
return this.aRangeUnlimitedNaturalMany;
}
@Override
public String getMetaDataAsJson() {
return TestManyValidation.TestManyValidationRuntimePropertyEnum.asJson();
}
@Override
public UmlgNode getOwningObject() {
return null;
}
@Override
public String getQualifiedName() {
return "umlgtest::org::umlg::validation::TestManyValidation";
}
/**
* getQualifiers is called from the collection in order to update the index used to implement the qualifier
*
* @param tumlRuntimeProperty
* @param node
* @param inverse
*/
@Override
public List getQualifiers(UmlgRuntimeProperty tumlRuntimeProperty, UmlgNode node, boolean inverse) {
List result = Collections.emptyList();
TestManyValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result.isEmpty() ) {
switch ( runtimeProperty ) {
default:
result = Collections.emptyList();
}
}
return result;
}
/**
* getSize is called from the BaseCollection.addInternal in order to save the size of the inverse collection to update the edge's sequence order
*
* @param inverse
* @param tumlRuntimeProperty
*/
@Override
public int getSize(boolean inverse, UmlgRuntimeProperty tumlRuntimeProperty) {
int result = 0;
TestManyValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result == 0 ) {
switch ( runtimeProperty ) {
case aMaxRealMany:
result = aMaxRealMany.size();
break;
case aMaxUnlimitedNaturalMany:
result = aMaxUnlimitedNaturalMany.size();
break;
case aMinUnlimitedNaturalMany:
result = aMinUnlimitedNaturalMany.size();
break;
case aRangeUnlimitedNaturalMany:
result = aRangeUnlimitedNaturalMany.size();
break;
case aMaxIntegerMany:
result = aMaxIntegerMany.size();
break;
case aRangeRealMany:
result = aRangeRealMany.size();
break;
case aRangeIntegerMany:
result = aRangeIntegerMany.size();
break;
case aMinRealMany:
result = aMinRealMany.size();
break;
case aMinIntegerMany:
result = aMinIntegerMany.size();
break;
default:
result = 0;
}
}
return result;
}
@Override
public String getUid() {
String uid;
if ( !this.vertex.property("uid").isPresent() && this.vertex.property("uid").value() != null ) {
uid=UUID.randomUUID().toString();
this.vertex.property("uid", uid);
} else {
uid=this.vertex.value("uid");
}
return uid;
}
@Override
public boolean hasOnlyOneCompositeParent() {
int result = 0;
return result == 1;
}
public void initDataTypeVariablesWithDefaultValues() {
}
public void initVariables() {
}
/**
* boolean properties' default values are initialized in the constructor via z_internalBooleanProperties
*
* @param loaded
*/
@Override
public void initialiseProperties(boolean loaded) {
this.aMaxRealMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMaxRealMany), loaded);
this.aMaxUnlimitedNaturalMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMaxUnlimitedNaturalMany), loaded);
this.aMinUnlimitedNaturalMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMinUnlimitedNaturalMany), loaded);
this.aRangeUnlimitedNaturalMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aRangeUnlimitedNaturalMany), loaded);
this.aMaxIntegerMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMaxIntegerMany), loaded);
this.aRangeRealMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aRangeRealMany), loaded);
this.aRangeIntegerMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aRangeIntegerMany), loaded);
this.aMinRealMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMinRealMany), loaded);
this.aMinIntegerMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMinIntegerMany), loaded);
}
@Override
public void initialiseProperty(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, boolean loaded) {
TestManyValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMaxRealMany:
this.aMaxRealMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMaxRealMany), loaded);
break;
case aMaxUnlimitedNaturalMany:
this.aMaxUnlimitedNaturalMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMaxUnlimitedNaturalMany), loaded);
break;
case aMinUnlimitedNaturalMany:
this.aMinUnlimitedNaturalMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMinUnlimitedNaturalMany), loaded);
break;
case aRangeUnlimitedNaturalMany:
this.aRangeUnlimitedNaturalMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aRangeUnlimitedNaturalMany), loaded);
break;
case aMaxIntegerMany:
this.aMaxIntegerMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMaxIntegerMany), loaded);
break;
case aRangeRealMany:
this.aRangeRealMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aRangeRealMany), loaded);
break;
case aRangeIntegerMany:
this.aRangeIntegerMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aRangeIntegerMany), loaded);
break;
case aMinRealMany:
this.aMinRealMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMinRealMany), loaded);
break;
case aMinIntegerMany:
this.aMinIntegerMany = new UmlgSetImpl(this, PropertyTree.from(TestManyValidationRuntimePropertyEnum.aMinIntegerMany), loaded);
break;
}
}
}
@Override
public UmlgRuntimeProperty inverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
TestManyValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
}
return runtimeProperty;
} else {
return null;
}
}
@Override
public boolean isTinkerRoot() {
return true;
}
public void removeFromAMaxIntegerMany(Integer aMaxIntegerMany) {
if ( aMaxIntegerMany != null ) {
this.aMaxIntegerMany.remove(aMaxIntegerMany);
}
}
public void removeFromAMaxIntegerMany(UmlgSet aMaxIntegerMany) {
if ( !aMaxIntegerMany.isEmpty() ) {
this.aMaxIntegerMany.removeAll(aMaxIntegerMany);
}
}
public void removeFromAMaxRealMany(Double aMaxRealMany) {
if ( aMaxRealMany != null ) {
this.aMaxRealMany.remove(aMaxRealMany);
}
}
public void removeFromAMaxRealMany(UmlgSet aMaxRealMany) {
if ( !aMaxRealMany.isEmpty() ) {
this.aMaxRealMany.removeAll(aMaxRealMany);
}
}
public void removeFromAMaxUnlimitedNaturalMany(Integer aMaxUnlimitedNaturalMany) {
if ( aMaxUnlimitedNaturalMany != null ) {
this.aMaxUnlimitedNaturalMany.remove(aMaxUnlimitedNaturalMany);
}
}
public void removeFromAMaxUnlimitedNaturalMany(UmlgSet aMaxUnlimitedNaturalMany) {
if ( !aMaxUnlimitedNaturalMany.isEmpty() ) {
this.aMaxUnlimitedNaturalMany.removeAll(aMaxUnlimitedNaturalMany);
}
}
public void removeFromAMinIntegerMany(Integer aMinIntegerMany) {
if ( aMinIntegerMany != null ) {
this.aMinIntegerMany.remove(aMinIntegerMany);
}
}
public void removeFromAMinIntegerMany(UmlgSet aMinIntegerMany) {
if ( !aMinIntegerMany.isEmpty() ) {
this.aMinIntegerMany.removeAll(aMinIntegerMany);
}
}
public void removeFromAMinRealMany(Double aMinRealMany) {
if ( aMinRealMany != null ) {
this.aMinRealMany.remove(aMinRealMany);
}
}
public void removeFromAMinRealMany(UmlgSet aMinRealMany) {
if ( !aMinRealMany.isEmpty() ) {
this.aMinRealMany.removeAll(aMinRealMany);
}
}
public void removeFromAMinUnlimitedNaturalMany(Integer aMinUnlimitedNaturalMany) {
if ( aMinUnlimitedNaturalMany != null ) {
this.aMinUnlimitedNaturalMany.remove(aMinUnlimitedNaturalMany);
}
}
public void removeFromAMinUnlimitedNaturalMany(UmlgSet aMinUnlimitedNaturalMany) {
if ( !aMinUnlimitedNaturalMany.isEmpty() ) {
this.aMinUnlimitedNaturalMany.removeAll(aMinUnlimitedNaturalMany);
}
}
public void removeFromARangeIntegerMany(Integer aRangeIntegerMany) {
if ( aRangeIntegerMany != null ) {
this.aRangeIntegerMany.remove(aRangeIntegerMany);
}
}
public void removeFromARangeIntegerMany(UmlgSet aRangeIntegerMany) {
if ( !aRangeIntegerMany.isEmpty() ) {
this.aRangeIntegerMany.removeAll(aRangeIntegerMany);
}
}
public void removeFromARangeRealMany(Double aRangeRealMany) {
if ( aRangeRealMany != null ) {
this.aRangeRealMany.remove(aRangeRealMany);
}
}
public void removeFromARangeRealMany(UmlgSet aRangeRealMany) {
if ( !aRangeRealMany.isEmpty() ) {
this.aRangeRealMany.removeAll(aRangeRealMany);
}
}
public void removeFromARangeUnlimitedNaturalMany(Integer aRangeUnlimitedNaturalMany) {
if ( aRangeUnlimitedNaturalMany != null ) {
this.aRangeUnlimitedNaturalMany.remove(aRangeUnlimitedNaturalMany);
}
}
public void removeFromARangeUnlimitedNaturalMany(UmlgSet aRangeUnlimitedNaturalMany) {
if ( !aRangeUnlimitedNaturalMany.isEmpty() ) {
this.aRangeUnlimitedNaturalMany.removeAll(aRangeUnlimitedNaturalMany);
}
}
public void setAMaxIntegerMany(UmlgSet aMaxIntegerMany) {
clearAMaxIntegerMany();
if ( aMaxIntegerMany != null ) {
addToAMaxIntegerMany(aMaxIntegerMany);
}
}
public void setAMaxRealMany(UmlgSet aMaxRealMany) {
clearAMaxRealMany();
if ( aMaxRealMany != null ) {
addToAMaxRealMany(aMaxRealMany);
}
}
public void setAMaxUnlimitedNaturalMany(UmlgSet aMaxUnlimitedNaturalMany) {
clearAMaxUnlimitedNaturalMany();
if ( aMaxUnlimitedNaturalMany != null ) {
addToAMaxUnlimitedNaturalMany(aMaxUnlimitedNaturalMany);
}
}
public void setAMinIntegerMany(UmlgSet aMinIntegerMany) {
clearAMinIntegerMany();
if ( aMinIntegerMany != null ) {
addToAMinIntegerMany(aMinIntegerMany);
}
}
public void setAMinRealMany(UmlgSet aMinRealMany) {
clearAMinRealMany();
if ( aMinRealMany != null ) {
addToAMinRealMany(aMinRealMany);
}
}
public void setAMinUnlimitedNaturalMany(UmlgSet aMinUnlimitedNaturalMany) {
clearAMinUnlimitedNaturalMany();
if ( aMinUnlimitedNaturalMany != null ) {
addToAMinUnlimitedNaturalMany(aMinUnlimitedNaturalMany);
}
}
public void setARangeIntegerMany(UmlgSet aRangeIntegerMany) {
clearARangeIntegerMany();
if ( aRangeIntegerMany != null ) {
addToARangeIntegerMany(aRangeIntegerMany);
}
}
public void setARangeRealMany(UmlgSet aRangeRealMany) {
clearARangeRealMany();
if ( aRangeRealMany != null ) {
addToARangeRealMany(aRangeRealMany);
}
}
public void setARangeUnlimitedNaturalMany(UmlgSet aRangeUnlimitedNaturalMany) {
clearARangeUnlimitedNaturalMany();
if ( aRangeUnlimitedNaturalMany != null ) {
addToARangeUnlimitedNaturalMany(aRangeUnlimitedNaturalMany);
}
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJson(Boolean deep) {
StringBuilder sb = new StringBuilder();
if ( this.tmpId != null ) {
sb.append("\"tmpId\": \"" + this.tmpId + "\", ");
}
sb.append("\"id\": \"" + getId() + "\", ");
sb.append("\"aMaxRealMany\": " + ToJsonUtil.primitivesToJson(getAMaxRealMany()) + "");
sb.append(", ");
sb.append("\"aMaxUnlimitedNaturalMany\": " + ToJsonUtil.primitivesToJson(getAMaxUnlimitedNaturalMany()) + "");
sb.append(", ");
sb.append("\"aMinUnlimitedNaturalMany\": " + ToJsonUtil.primitivesToJson(getAMinUnlimitedNaturalMany()) + "");
sb.append(", ");
sb.append("\"aRangeUnlimitedNaturalMany\": " + ToJsonUtil.primitivesToJson(getARangeUnlimitedNaturalMany()) + "");
sb.append(", ");
sb.append("\"aMaxIntegerMany\": " + ToJsonUtil.primitivesToJson(getAMaxIntegerMany()) + "");
sb.append(", ");
sb.append("\"aRangeRealMany\": " + ToJsonUtil.primitivesToJson(getARangeRealMany()) + "");
sb.append(", ");
sb.append("\"aRangeIntegerMany\": " + ToJsonUtil.primitivesToJson(getARangeIntegerMany()) + "");
sb.append(", ");
sb.append("\"aMinRealMany\": " + ToJsonUtil.primitivesToJson(getAMinRealMany()) + "");
sb.append(", ");
sb.append("\"aMinIntegerMany\": " + ToJsonUtil.primitivesToJson(getAMinIntegerMany()) + "");
sb.append(", ");
sb.append("\"qualifiedName\": \"" + getQualifiedName() + "\"");
sb.append(", ");
//PlaceHolder for restful
sb.append("\"uri\": {}");
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJson() {
return toJson(false);
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJsonWithoutCompositeParent(Boolean deep) {
StringBuilder sb = new StringBuilder();
if ( this.tmpId != null ) {
sb.append("\"tmpId\": \"" + this.tmpId + "\", ");
}
sb.append("\"id\": \"" + getId() + "\", ");
sb.append("\"aMaxRealMany\": " + ToJsonUtil.primitivesToJson(getAMaxRealMany()) + "");
sb.append(", ");
sb.append("\"aMaxUnlimitedNaturalMany\": " + ToJsonUtil.primitivesToJson(getAMaxUnlimitedNaturalMany()) + "");
sb.append(", ");
sb.append("\"aMinUnlimitedNaturalMany\": " + ToJsonUtil.primitivesToJson(getAMinUnlimitedNaturalMany()) + "");
sb.append(", ");
sb.append("\"aRangeUnlimitedNaturalMany\": " + ToJsonUtil.primitivesToJson(getARangeUnlimitedNaturalMany()) + "");
sb.append(", ");
sb.append("\"aMaxIntegerMany\": " + ToJsonUtil.primitivesToJson(getAMaxIntegerMany()) + "");
sb.append(", ");
sb.append("\"aRangeRealMany\": " + ToJsonUtil.primitivesToJson(getARangeRealMany()) + "");
sb.append(", ");
sb.append("\"aRangeIntegerMany\": " + ToJsonUtil.primitivesToJson(getARangeIntegerMany()) + "");
sb.append(", ");
sb.append("\"aMinRealMany\": " + ToJsonUtil.primitivesToJson(getAMinRealMany()) + "");
sb.append(", ");
sb.append("\"aMinIntegerMany\": " + ToJsonUtil.primitivesToJson(getAMinIntegerMany()) + "");
sb.append(", ");
sb.append("\"qualifiedName\": \"" + getQualifiedName() + "\"");
sb.append(", ");
//PlaceHolder for restful
sb.append("\"uri\": {}");
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJsonWithoutCompositeParent() {
return toJsonWithoutCompositeParent(false);
}
public List validateAMaxIntegerMany(Integer aMaxIntegerMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateMaxInteger(aMaxIntegerMany, 5) ) {
result.add(new UmlgConstraintViolation("MaxInteger", "umlgtest::org::umlg::validation::TestManyValidation::aMaxIntegerMany", "MaxInteger does not pass validation!"));
}
return result;
}
public List validateAMaxRealMany(Double aMaxRealMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateMaxReal(aMaxRealMany, 5.123) ) {
result.add(new UmlgConstraintViolation("MaxReal", "umlgtest::org::umlg::validation::TestManyValidation::aMaxRealMany", "MaxReal does not pass validation!"));
}
return result;
}
public List validateAMaxUnlimitedNaturalMany(Integer aMaxUnlimitedNaturalMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateValidUnlimitedNatural(aMaxUnlimitedNaturalMany) ) {
result.add(new UmlgConstraintViolation("UnlimitedNatural", "umlgtest::org::umlg::validation::TestManyValidation::aMaxUnlimitedNaturalMany", " must be greater or equal to zero!"));
}
if ( !UmlgValidator.validateMaxUnlimitedNatural(aMaxUnlimitedNaturalMany, 5) ) {
result.add(new UmlgConstraintViolation("MaxUnlimitedNatural", "umlgtest::org::umlg::validation::TestManyValidation::aMaxUnlimitedNaturalMany", "MaxUnlimitedNatural does not pass validation!"));
}
return result;
}
public List validateAMinIntegerMany(Integer aMinIntegerMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateMinInteger(aMinIntegerMany, 5) ) {
result.add(new UmlgConstraintViolation("MinInteger", "umlgtest::org::umlg::validation::TestManyValidation::aMinIntegerMany", "MinInteger does not pass validation!"));
}
return result;
}
public List validateAMinRealMany(Double aMinRealMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateMinReal(aMinRealMany, 5.123) ) {
result.add(new UmlgConstraintViolation("MinReal", "umlgtest::org::umlg::validation::TestManyValidation::aMinRealMany", "MinReal does not pass validation!"));
}
return result;
}
public List validateAMinUnlimitedNaturalMany(Integer aMinUnlimitedNaturalMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateValidUnlimitedNatural(aMinUnlimitedNaturalMany) ) {
result.add(new UmlgConstraintViolation("UnlimitedNatural", "umlgtest::org::umlg::validation::TestManyValidation::aMinUnlimitedNaturalMany", " must be greater or equal to zero!"));
}
if ( !UmlgValidator.validateMinUnlimitedNatural(aMinUnlimitedNaturalMany, 5) ) {
result.add(new UmlgConstraintViolation("MinUnlimitedNatural", "umlgtest::org::umlg::validation::TestManyValidation::aMinUnlimitedNaturalMany", "MinUnlimitedNatural does not pass validation!"));
}
return result;
}
public List validateARangeIntegerMany(Integer aRangeIntegerMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateRangeInteger(aRangeIntegerMany, -5, 5) ) {
result.add(new UmlgConstraintViolation("RangeInteger", "umlgtest::org::umlg::validation::TestManyValidation::aRangeIntegerMany", "RangeInteger does not pass validation!"));
}
return result;
}
public List validateARangeRealMany(Double aRangeRealMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateRangeReal(aRangeRealMany, -5.123, 5.123) ) {
result.add(new UmlgConstraintViolation("RangeReal", "umlgtest::org::umlg::validation::TestManyValidation::aRangeRealMany", "RangeReal does not pass validation!"));
}
return result;
}
public List validateARangeUnlimitedNaturalMany(Integer aRangeUnlimitedNaturalMany) {
List result = new ArrayList();
if ( !UmlgValidator.validateValidUnlimitedNatural(aRangeUnlimitedNaturalMany) ) {
result.add(new UmlgConstraintViolation("UnlimitedNatural", "umlgtest::org::umlg::validation::TestManyValidation::aRangeUnlimitedNaturalMany", " must be greater or equal to zero!"));
}
if ( !UmlgValidator.validateRangeUnlimitedNatural(aRangeUnlimitedNaturalMany, 1, 5) ) {
result.add(new UmlgConstraintViolation("RangeUnlimitedNatural", "umlgtest::org::umlg::validation::TestManyValidation::aRangeUnlimitedNaturalMany", "RangeUnlimitedNatural does not pass validation!"));
}
return result;
}
@Override
public List validateMultiplicities() {
List result = new ArrayList();
return result;
}
@Override
public void z_internalAddPersistentValueToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
TestManyValidationRuntimePropertyEnum runtimeProperty;
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMaxRealMany:
for ( double s : (double[])umlgNode ) {
this.aMaxRealMany.z_internalAdder((double)s);
}
break;
case aMaxUnlimitedNaturalMany:
for ( int s : (int[])umlgNode ) {
this.aMaxUnlimitedNaturalMany.z_internalAdder((int)s);
}
break;
case aMinUnlimitedNaturalMany:
for ( int s : (int[])umlgNode ) {
this.aMinUnlimitedNaturalMany.z_internalAdder((int)s);
}
break;
case aRangeUnlimitedNaturalMany:
for ( int s : (int[])umlgNode ) {
this.aRangeUnlimitedNaturalMany.z_internalAdder((int)s);
}
break;
case aMaxIntegerMany:
for ( int s : (int[])umlgNode ) {
this.aMaxIntegerMany.z_internalAdder((int)s);
}
break;
case aRangeRealMany:
for ( double s : (double[])umlgNode ) {
this.aRangeRealMany.z_internalAdder((double)s);
}
break;
case aRangeIntegerMany:
for ( int s : (int[])umlgNode ) {
this.aRangeIntegerMany.z_internalAdder((int)s);
}
break;
case aMinRealMany:
for ( double s : (double[])umlgNode ) {
this.aMinRealMany.z_internalAdder((double)s);
}
break;
case aMinIntegerMany:
for ( int s : (int[])umlgNode ) {
this.aMinIntegerMany.z_internalAdder((int)s);
}
break;
}
}
}
@Override
public void z_internalAddToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
TestManyValidationRuntimePropertyEnum runtimeProperty;
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMaxRealMany:
for ( Double s : (Double[])umlgNode ) {
this.aMaxRealMany.z_internalAdder(s);
}
break;
case aMaxUnlimitedNaturalMany:
for ( Integer s : (Integer[])umlgNode ) {
this.aMaxUnlimitedNaturalMany.z_internalAdder(s);
}
break;
case aMinUnlimitedNaturalMany:
for ( Integer s : (Integer[])umlgNode ) {
this.aMinUnlimitedNaturalMany.z_internalAdder(s);
}
break;
case aRangeUnlimitedNaturalMany:
for ( Integer s : (Integer[])umlgNode ) {
this.aRangeUnlimitedNaturalMany.z_internalAdder(s);
}
break;
case aMaxIntegerMany:
for ( Integer s : (Integer[])umlgNode ) {
this.aMaxIntegerMany.z_internalAdder(s);
}
break;
case aRangeRealMany:
for ( Double s : (Double[])umlgNode ) {
this.aRangeRealMany.z_internalAdder(s);
}
break;
case aRangeIntegerMany:
for ( Integer s : (Integer[])umlgNode ) {
this.aRangeIntegerMany.z_internalAdder(s);
}
break;
case aMinRealMany:
for ( Double s : (Double[])umlgNode ) {
this.aMinRealMany.z_internalAdder(s);
}
break;
case aMinIntegerMany:
for ( Integer s : (Integer[])umlgNode ) {
this.aMinIntegerMany.z_internalAdder(s);
}
break;
}
}
}
@Override
public Set z_internalBooleanProperties() {
Set result = new HashSet();
return result;
}
public void z_internalClearAMaxIntegerMany() {
this.aMaxIntegerMany.z_internalClear();
}
public void z_internalClearAMaxRealMany() {
this.aMaxRealMany.z_internalClear();
}
public void z_internalClearAMaxUnlimitedNaturalMany() {
this.aMaxUnlimitedNaturalMany.z_internalClear();
}
public void z_internalClearAMinIntegerMany() {
this.aMinIntegerMany.z_internalClear();
}
public void z_internalClearAMinRealMany() {
this.aMinRealMany.z_internalClear();
}
public void z_internalClearAMinUnlimitedNaturalMany() {
this.aMinUnlimitedNaturalMany.z_internalClear();
}
public void z_internalClearARangeIntegerMany() {
this.aRangeIntegerMany.z_internalClear();
}
public void z_internalClearARangeRealMany() {
this.aRangeRealMany.z_internalClear();
}
public void z_internalClearARangeUnlimitedNaturalMany() {
this.aRangeUnlimitedNaturalMany.z_internalClear();
}
@Override
public Set z_internalDataTypeProperties() {
Set result = new HashSet();
result.add(TestManyValidationRuntimePropertyEnum.aMaxRealMany);
result.add(TestManyValidationRuntimePropertyEnum.aMaxUnlimitedNaturalMany);
result.add(TestManyValidationRuntimePropertyEnum.aMinUnlimitedNaturalMany);
result.add(TestManyValidationRuntimePropertyEnum.aRangeUnlimitedNaturalMany);
result.add(TestManyValidationRuntimePropertyEnum.aMaxIntegerMany);
result.add(TestManyValidationRuntimePropertyEnum.aRangeRealMany);
result.add(TestManyValidationRuntimePropertyEnum.aRangeIntegerMany);
result.add(TestManyValidationRuntimePropertyEnum.aMinRealMany);
result.add(TestManyValidationRuntimePropertyEnum.aMinIntegerMany);
return result;
}
@Override
public Map z_internalDataTypePropertiesWithDefaultValues() {
Map result = new HashMap();
return result;
}
@Override
public UmlgCollection extends Object> z_internalGetCollectionFor(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse) {
UmlgCollection extends Object> result = null;
if ( result == null ) {
TestManyValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMaxRealMany:
result = this.aMaxRealMany;
break;
case aMaxUnlimitedNaturalMany:
result = this.aMaxUnlimitedNaturalMany;
break;
case aMinUnlimitedNaturalMany:
result = this.aMinUnlimitedNaturalMany;
break;
case aRangeUnlimitedNaturalMany:
result = this.aRangeUnlimitedNaturalMany;
break;
case aMaxIntegerMany:
result = this.aMaxIntegerMany;
break;
case aRangeRealMany:
result = this.aRangeRealMany;
break;
case aRangeIntegerMany:
result = this.aRangeIntegerMany;
break;
case aMinRealMany:
result = this.aMinRealMany;
break;
case aMinIntegerMany:
result = this.aMinIntegerMany;
break;
}
}
}
return result;
}
@Override
public UmlgRuntimeProperty z_internalInverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
TestManyValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
}
return runtimeProperty;
} else {
return null;
}
}
@Override
public void z_internalMarkCollectionLoaded(UmlgRuntimeProperty umlgRuntimeProperty, boolean loaded) {
TestManyValidationRuntimePropertyEnum runtimeProperty;
runtimeProperty = (TestManyValidationRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMaxRealMany:
this.aMaxRealMany.setLoaded(loaded);
break;
case aMaxUnlimitedNaturalMany:
this.aMaxUnlimitedNaturalMany.setLoaded(loaded);
break;
case aMinUnlimitedNaturalMany:
this.aMinUnlimitedNaturalMany.setLoaded(loaded);
break;
case aRangeUnlimitedNaturalMany:
this.aRangeUnlimitedNaturalMany.setLoaded(loaded);
break;
case aMaxIntegerMany:
this.aMaxIntegerMany.setLoaded(loaded);
break;
case aRangeRealMany:
this.aRangeRealMany.setLoaded(loaded);
break;
case aRangeIntegerMany:
this.aRangeIntegerMany.setLoaded(loaded);
break;
case aMinRealMany:
this.aMinRealMany.setLoaded(loaded);
break;
case aMinIntegerMany:
this.aMinIntegerMany.setLoaded(loaded);
break;
}
}
}
static public enum TestManyValidationRuntimePropertyEnum implements UmlgRuntimeProperty {
aMaxRealMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aMaxRealMany",/* persistentName */ "aMaxRealMany",/* inverseName */ "inverseOf::aMaxRealMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMaxRealMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MaxReal(5.123)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMaxRealMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Double.class,/* json */ "{\"name\": \"aMaxRealMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"max\": 5.123}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aMaxRealMany\", \"persistentName\": \"aMaxRealMany\", \"inverseName\": \"inverseOf::aMaxRealMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMaxRealMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMaxRealMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMaxUnlimitedNaturalMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aMaxUnlimitedNaturalMany",/* persistentName */ "aMaxUnlimitedNaturalMany",/* inverseName */ "inverseOf::aMaxUnlimitedNaturalMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMaxUnlimitedNaturalMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MaxUnlimitedNatural(5)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMaxUnlimitedNaturalMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aMaxUnlimitedNaturalMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"max\": 5}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aMaxUnlimitedNaturalMany\", \"persistentName\": \"aMaxUnlimitedNaturalMany\", \"inverseName\": \"inverseOf::aMaxUnlimitedNaturalMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMaxUnlimitedNaturalMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMaxUnlimitedNaturalMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMinUnlimitedNaturalMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aMinUnlimitedNaturalMany",/* persistentName */ "aMinUnlimitedNaturalMany",/* inverseName */ "inverseOf::aMinUnlimitedNaturalMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMinUnlimitedNaturalMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MinUnlimitedNatural(5)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMinUnlimitedNaturalMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aMinUnlimitedNaturalMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"min\": 5}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aMinUnlimitedNaturalMany\", \"persistentName\": \"aMinUnlimitedNaturalMany\", \"inverseName\": \"inverseOf::aMinUnlimitedNaturalMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMinUnlimitedNaturalMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMinUnlimitedNaturalMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aRangeUnlimitedNaturalMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aRangeUnlimitedNaturalMany",/* persistentName */ "aRangeUnlimitedNaturalMany",/* inverseName */ "inverseOf::aRangeUnlimitedNaturalMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aRangeUnlimitedNaturalMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new RangeUnlimitedNatural(1, 5)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aRangeUnlimitedNaturalMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aRangeUnlimitedNaturalMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"range\": {\"min\": 1, \"max\": 5}}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aRangeUnlimitedNaturalMany\", \"persistentName\": \"aRangeUnlimitedNaturalMany\", \"inverseName\": \"inverseOf::aRangeUnlimitedNaturalMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aRangeUnlimitedNaturalMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aRangeUnlimitedNaturalMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMaxIntegerMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aMaxIntegerMany",/* persistentName */ "aMaxIntegerMany",/* inverseName */ "inverseOf::aMaxIntegerMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMaxIntegerMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MaxInteger(5)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMaxIntegerMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aMaxIntegerMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"max\": 5}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aMaxIntegerMany\", \"persistentName\": \"aMaxIntegerMany\", \"inverseName\": \"inverseOf::aMaxIntegerMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMaxIntegerMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMaxIntegerMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aRangeRealMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aRangeRealMany",/* persistentName */ "aRangeRealMany",/* inverseName */ "inverseOf::aRangeRealMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aRangeRealMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new RangeReal(-5.123, 5.123)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aRangeRealMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Double.class,/* json */ "{\"name\": \"aRangeRealMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"range\": {\"min\": -5.123, \"max\": 5.123}}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aRangeRealMany\", \"persistentName\": \"aRangeRealMany\", \"inverseName\": \"inverseOf::aRangeRealMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aRangeRealMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aRangeRealMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aRangeIntegerMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aRangeIntegerMany",/* persistentName */ "aRangeIntegerMany",/* inverseName */ "inverseOf::aRangeIntegerMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aRangeIntegerMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new RangeInteger(-5, 5)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aRangeIntegerMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aRangeIntegerMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"range\": {\"min\": -5, \"max\": 5}}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aRangeIntegerMany\", \"persistentName\": \"aRangeIntegerMany\", \"inverseName\": \"inverseOf::aRangeIntegerMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aRangeIntegerMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aRangeIntegerMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMinRealMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aMinRealMany",/* persistentName */ "aMinRealMany",/* inverseName */ "inverseOf::aMinRealMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMinRealMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MinReal(5.123)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMinRealMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Double.class,/* json */ "{\"name\": \"aMinRealMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"min\": 5.123}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aMinRealMany\", \"persistentName\": \"aMinRealMany\", \"inverseName\": \"inverseOf::aMinRealMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMinRealMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMinRealMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMinIntegerMany(/* qualifiedName */ "umlgtest::org::umlg::validation::TestManyValidation::aMinIntegerMany",/* persistentName */ "aMinIntegerMany",/* inverseName */ "inverseOf::aMinIntegerMany",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMinIntegerMany",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MinInteger(5)),/* isManyPrimitive */ true,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMinIntegerMany"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ true,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aMinIntegerMany\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"min\": 5}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation::aMinIntegerMany\", \"persistentName\": \"aMinIntegerMany\", \"inverseName\": \"inverseOf::aMinIntegerMany\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestManyValidation::aMinIntegerMany\", \"manyPrimitive\": true, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": true, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMinIntegerMany\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
umlgtest(/* qualifiedName */ "umlgtest",/* persistentName */ "umlgtest",/* inverseName */ "inverseOfumlgtest",/* inverseQualifiedName */ "inverseOfumlgtest",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ true,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("rootTestManyValidation"),/* isOneToOne */ true,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ false,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ false,/* propertyType */ Object.class,/* json */ "{\"name\": \"umlgtest\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest\", \"persistentName\": \"umlgtest\", \"inverseName\": \"inverseOfumlgtest\", \"inverseQualifiedName\": \"inverseOfumlgtest\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": true, \"oneToOne\": true, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": false, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"rootTestManyValidation\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": false, \"inverseUnique\": false, \"derived\": false, \"navigable\": false}",/* isChangeListenerAttribute */ false);
private String _qualifiedName;
private String _persistentName;
private String _inverseName;
private String _inverseQualifiedName;
private boolean _associationClassOne;
private boolean _memberEndOfAssociationClass;
private String _associationClassPropertyName;
private String _inverseAssociationClassPropertyName;
private boolean _associationClassProperty;
private boolean _onePrimitivePropertyOfAssociationClass;
private boolean _onePrimitive;
private Boolean _readOnly;
private DataTypeEnum dataTypeEnum;
private List validations;
private boolean _manyPrimitive;
private boolean _oneEnumeration;
private boolean _manyEnumeration;
private boolean _controllingSide;
private boolean _composite;
private boolean _inverseComposite;
private String _label;
private boolean _oneToOne;
private boolean _oneToMany;
private boolean _manyToOne;
private boolean _manyToMany;
private int _upper;
private int _lower;
private int _inverseUpper;
private boolean _qualified;
private boolean _inverseQualified;
private boolean _ordered;
private boolean _inverseOrdered;
private boolean _unique;
private boolean _inverseUnique;
private boolean _derived;
private boolean _navigability;
private Class _propertyType;
private String _json;
private boolean _changeListener;
/**
* constructor for TestManyValidationRuntimePropertyEnum
*
* @param _qualifiedName
* @param _persistentName
* @param _inverseName
* @param _inverseQualifiedName
* @param _associationClassOne
* @param _memberEndOfAssociationClass
* @param _associationClassPropertyName
* @param _inverseAssociationClassPropertyName
* @param _associationClassProperty
* @param _onePrimitivePropertyOfAssociationClass
* @param _onePrimitive
* @param _readOnly
* @param dataTypeEnum
* @param validations
* @param _manyPrimitive
* @param _oneEnumeration
* @param _manyEnumeration
* @param _controllingSide
* @param _composite
* @param _inverseComposite
* @param _label
* @param _oneToOne
* @param _oneToMany
* @param _manyToOne
* @param _manyToMany
* @param _upper
* @param _lower
* @param _inverseUpper
* @param _qualified
* @param _inverseQualified
* @param _ordered
* @param _inverseOrdered
* @param _unique
* @param _inverseUnique
* @param _derived
* @param _navigability
* @param _propertyType
* @param _json
* @param _changeListener
*/
private TestManyValidationRuntimePropertyEnum(String _qualifiedName, String _persistentName, String _inverseName, String _inverseQualifiedName, boolean _associationClassOne, boolean _memberEndOfAssociationClass, String _associationClassPropertyName, String _inverseAssociationClassPropertyName, boolean _associationClassProperty, boolean _onePrimitivePropertyOfAssociationClass, boolean _onePrimitive, Boolean _readOnly, DataTypeEnum dataTypeEnum, List validations, boolean _manyPrimitive, boolean _oneEnumeration, boolean _manyEnumeration, boolean _controllingSide, boolean _composite, boolean _inverseComposite, String _label, boolean _oneToOne, boolean _oneToMany, boolean _manyToOne, boolean _manyToMany, int _upper, int _lower, int _inverseUpper, boolean _qualified, boolean _inverseQualified, boolean _ordered, boolean _inverseOrdered, boolean _unique, boolean _inverseUnique, boolean _derived, boolean _navigability, Class _propertyType, String _json, boolean _changeListener) {
this._qualifiedName = _qualifiedName;
this._persistentName = _persistentName;
this._inverseName = _inverseName;
this._inverseQualifiedName = _inverseQualifiedName;
this._associationClassOne = _associationClassOne;
this._memberEndOfAssociationClass = _memberEndOfAssociationClass;
this._associationClassPropertyName = _associationClassPropertyName;
this._inverseAssociationClassPropertyName = _inverseAssociationClassPropertyName;
this._associationClassProperty = _associationClassProperty;
this._onePrimitivePropertyOfAssociationClass = _onePrimitivePropertyOfAssociationClass;
this._onePrimitive = _onePrimitive;
this._readOnly = _readOnly;
this.dataTypeEnum = dataTypeEnum;
this.validations = validations;
this._manyPrimitive = _manyPrimitive;
this._oneEnumeration = _oneEnumeration;
this._manyEnumeration = _manyEnumeration;
this._controllingSide = _controllingSide;
this._composite = _composite;
this._inverseComposite = _inverseComposite;
this._label = _label;
this._oneToOne = _oneToOne;
this._oneToMany = _oneToMany;
this._manyToOne = _manyToOne;
this._manyToMany = _manyToMany;
this._upper = _upper;
this._lower = _lower;
this._inverseUpper = _inverseUpper;
this._qualified = _qualified;
this._inverseQualified = _inverseQualified;
this._ordered = _ordered;
this._inverseOrdered = _inverseOrdered;
this._unique = _unique;
this._inverseUnique = _inverseUnique;
this._derived = _derived;
this._navigability = _navigability;
this._propertyType = _propertyType;
this._json = _json;
this._changeListener = _changeListener;
}
static public String asJson() {
int count = 1;
StringBuilder sb = new StringBuilder();;
sb.append("{\"name\": \"TestManyValidation\", ");
sb.append("\"qualifiedName\": \"umlgtest::org::umlg::validation::TestManyValidation\", ");
sb.append("\"uri\": \"TODO\", ");
sb.append("\"properties\": [");
for ( TestManyValidationRuntimePropertyEnum l : TestManyValidationRuntimePropertyEnum.values() ) {
sb.append(l.toJson());
if ( count < TestManyValidationRuntimePropertyEnum.values().length ) {
count++;
sb.append(",");
}
}
sb.append("]}");
return sb.toString();
}
static public TestManyValidationRuntimePropertyEnum fromInverseQualifiedName(String inverseQualifiedName) {
if ( umlgtest.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return umlgtest;
}
if ( aMinIntegerMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMinIntegerMany;
}
if ( aMinRealMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMinRealMany;
}
if ( aRangeIntegerMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aRangeIntegerMany;
}
if ( aRangeRealMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aRangeRealMany;
}
if ( aMaxIntegerMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMaxIntegerMany;
}
if ( aRangeUnlimitedNaturalMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aRangeUnlimitedNaturalMany;
}
if ( aMinUnlimitedNaturalMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMinUnlimitedNaturalMany;
}
if ( aMaxUnlimitedNaturalMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMaxUnlimitedNaturalMany;
}
if ( aMaxRealMany.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMaxRealMany;
}
return null;
}
static public TestManyValidationRuntimePropertyEnum fromLabel(String _label) {
if ( umlgtest.getLabel().equals(_label) ) {
return umlgtest;
}
if ( aMinIntegerMany.getLabel().equals(_label) ) {
return aMinIntegerMany;
}
if ( aMinRealMany.getLabel().equals(_label) ) {
return aMinRealMany;
}
if ( aRangeIntegerMany.getLabel().equals(_label) ) {
return aRangeIntegerMany;
}
if ( aRangeRealMany.getLabel().equals(_label) ) {
return aRangeRealMany;
}
if ( aMaxIntegerMany.getLabel().equals(_label) ) {
return aMaxIntegerMany;
}
if ( aRangeUnlimitedNaturalMany.getLabel().equals(_label) ) {
return aRangeUnlimitedNaturalMany;
}
if ( aMinUnlimitedNaturalMany.getLabel().equals(_label) ) {
return aMinUnlimitedNaturalMany;
}
if ( aMaxUnlimitedNaturalMany.getLabel().equals(_label) ) {
return aMaxUnlimitedNaturalMany;
}
if ( aMaxRealMany.getLabel().equals(_label) ) {
return aMaxRealMany;
}
return null;
}
static public TestManyValidationRuntimePropertyEnum fromQualifiedName(String qualifiedName) {
if ( umlgtest.getQualifiedName().equals(qualifiedName) ) {
return umlgtest;
}
if ( aMinIntegerMany.getQualifiedName().equals(qualifiedName) ) {
return aMinIntegerMany;
}
if ( aMinRealMany.getQualifiedName().equals(qualifiedName) ) {
return aMinRealMany;
}
if ( aRangeIntegerMany.getQualifiedName().equals(qualifiedName) ) {
return aRangeIntegerMany;
}
if ( aRangeRealMany.getQualifiedName().equals(qualifiedName) ) {
return aRangeRealMany;
}
if ( aMaxIntegerMany.getQualifiedName().equals(qualifiedName) ) {
return aMaxIntegerMany;
}
if ( aRangeUnlimitedNaturalMany.getQualifiedName().equals(qualifiedName) ) {
return aRangeUnlimitedNaturalMany;
}
if ( aMinUnlimitedNaturalMany.getQualifiedName().equals(qualifiedName) ) {
return aMinUnlimitedNaturalMany;
}
if ( aMaxUnlimitedNaturalMany.getQualifiedName().equals(qualifiedName) ) {
return aMaxUnlimitedNaturalMany;
}
if ( aMaxRealMany.getQualifiedName().equals(qualifiedName) ) {
return aMaxRealMany;
}
return null;
}
public String getAssociationClassPropertyName() {
return this._associationClassPropertyName;
}
public DataTypeEnum getDataTypeEnum() {
return this.dataTypeEnum;
}
public String getInverseAssociationClassPropertyName() {
return this._inverseAssociationClassPropertyName;
}
public String getInverseName() {
return this._inverseName;
}
public String getInverseQualifiedName() {
return this._inverseQualifiedName;
}
public int getInverseUpper() {
return this._inverseUpper;
}
public String getJson() {
return this._json;
}
public String getLabel() {
return this._label;
}
public int getLower() {
return this._lower;
}
public String getPersistentName() {
return this._persistentName;
}
public Class getPropertyType() {
return this._propertyType;
}
public String getQualifiedName() {
return this._qualifiedName;
}
public Boolean getReadOnly() {
return this._readOnly;
}
public int getUpper() {
return this._upper;
}
public List getValidations() {
return this.validations;
}
public boolean isAssociationClassOne() {
return this._associationClassOne;
}
public boolean isAssociationClassProperty() {
return this._associationClassProperty;
}
public boolean isChangeListener() {
return this._changeListener;
}
public boolean isComposite() {
return this._composite;
}
public boolean isControllingSide() {
return this._controllingSide;
}
public boolean isDerived() {
return this._derived;
}
public boolean isInverseComposite() {
return this._inverseComposite;
}
public boolean isInverseOrdered() {
return this._inverseOrdered;
}
public boolean isInverseQualified() {
return this._inverseQualified;
}
public boolean isInverseUnique() {
return this._inverseUnique;
}
public boolean isManyEnumeration() {
return this._manyEnumeration;
}
public boolean isManyPrimitive() {
return this._manyPrimitive;
}
public boolean isManyToMany() {
return this._manyToMany;
}
public boolean isManyToOne() {
return this._manyToOne;
}
public boolean isMemberEndOfAssociationClass() {
return this._memberEndOfAssociationClass;
}
public boolean isNavigability() {
return this._navigability;
}
public boolean isOneEnumeration() {
return this._oneEnumeration;
}
public boolean isOnePrimitive() {
return this._onePrimitive;
}
public boolean isOnePrimitivePropertyOfAssociationClass() {
return this._onePrimitivePropertyOfAssociationClass;
}
public boolean isOneToMany() {
return this._oneToMany;
}
public boolean isOneToOne() {
return this._oneToOne;
}
public boolean isOrdered() {
return this._ordered;
}
public boolean isQualified() {
return this._qualified;
}
public boolean isUnique() {
return this._unique;
}
@Override
public boolean isValid(int elementCount) {
if ( isQualified() ) {
return elementCount >= getLower();
} else {
return (getUpper() == -1 || elementCount <= getUpper()) && elementCount >= getLower();
}
}
@Override
public String toJson() {
return getJson();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy