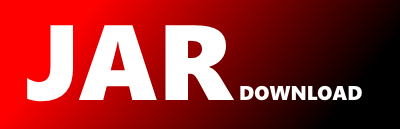
org.umlg.validation.TestValidation Maven / Gradle / Ivy
The newest version!
package org.umlg.validation;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
import org.apache.commons.text.StringEscapeUtils;
import org.apache.tinkerpop.gremlin.structure.Vertex;
import org.umlg.runtime.adaptor.TransactionThreadEntityVar;
import org.umlg.runtime.adaptor.UMLG;
import org.umlg.runtime.adaptor.UmlgLabelConverterFactory;
import org.umlg.runtime.adaptor.UmlgTmpIdManager;
import org.umlg.runtime.collection.Filter;
import org.umlg.runtime.collection.Qualifier;
import org.umlg.runtime.collection.UmlgCollection;
import org.umlg.runtime.collection.UmlgRuntimeProperty;
import org.umlg.runtime.collection.UmlgSet;
import org.umlg.runtime.collection.memory.UmlgMemorySet;
import org.umlg.runtime.collection.persistent.PropertyTree;
import org.umlg.runtime.collection.persistent.UmlgSetImpl;
import org.umlg.runtime.domain.BaseUmlgCompositionNode;
import org.umlg.runtime.domain.CompositionNode;
import org.umlg.runtime.domain.DataTypeEnum;
import org.umlg.runtime.domain.UmlgNode;
import org.umlg.runtime.domain.UmlgRootNode;
import org.umlg.runtime.util.ObjectMapperFactory;
import org.umlg.runtime.util.UmlgFormatter;
import org.umlg.runtime.validation.MaxInteger;
import org.umlg.runtime.validation.MaxReal;
import org.umlg.runtime.validation.MaxUnlimitedNatural;
import org.umlg.runtime.validation.MinInteger;
import org.umlg.runtime.validation.MinReal;
import org.umlg.runtime.validation.MinUnlimitedNatural;
import org.umlg.runtime.validation.RangeInteger;
import org.umlg.runtime.validation.RangeReal;
import org.umlg.runtime.validation.RangeUnlimitedNatural;
import org.umlg.runtime.validation.UmlgConstraintViolation;
import org.umlg.runtime.validation.UmlgConstraintViolationException;
import org.umlg.runtime.validation.UmlgValidation;
import org.umlg.runtime.validation.UmlgValidator;
public class TestValidation extends BaseUmlgCompositionNode implements UmlgRootNode, CompositionNode {
static final public long serialVersionUID = 1L;
private UmlgSet aMaxReal;
private UmlgSet aMinReal;
private UmlgSet aRangeReal;
private UmlgSet aMinUnlimitedNatural;
private UmlgSet aMaxUnlimitedNatural;
private UmlgSet aRangeUnlimitedNatural;
private UmlgSet aMinInteger;
private UmlgSet aMaxInteger;
private UmlgSet aRangeInteger;
private UmlgSet testUnlimitedNatural;
private String tmpId; // tmpId is only used the umlg restlet gui. It is never persisted. Its value is generated by the gui.
/**
* constructor for TestValidation
*
* @param id
*/
public TestValidation(Object id) {
super(id);
}
/**
* constructor for TestValidation
*
* @param vertex
*/
public TestValidation(Vertex vertex) {
super(vertex);
}
/**
* default constructor for TestValidation
*/
public TestValidation() {
this(true);
}
/**
* constructor for TestValidation
*
* @param persistent
*/
public TestValidation(Boolean persistent) {
super(persistent);
}
public void addToAMaxInteger(Integer aMaxInteger) {
if ( !this.aMaxInteger.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMaxInteger != null ) {
List violations = validateAMaxInteger(aMaxInteger);
if ( violations.isEmpty() ) {
this.aMaxInteger.add(aMaxInteger);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxIntegerIgnoreInverse(Integer aMaxInteger) {
if ( !this.aMaxInteger.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMaxInteger != null ) {
List violations = validateAMaxInteger(aMaxInteger);
if ( violations.isEmpty() ) {
this.aMaxInteger.add(aMaxInteger);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxReal(Double aMaxReal) {
if ( !this.aMaxReal.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMaxReal != null ) {
List violations = validateAMaxReal(aMaxReal);
if ( violations.isEmpty() ) {
this.aMaxReal.add(aMaxReal);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxRealIgnoreInverse(Double aMaxReal) {
if ( !this.aMaxReal.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMaxReal != null ) {
List violations = validateAMaxReal(aMaxReal);
if ( violations.isEmpty() ) {
this.aMaxReal.add(aMaxReal);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxUnlimitedNatural(Integer aMaxUnlimitedNatural) {
if ( !this.aMaxUnlimitedNatural.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMaxUnlimitedNatural != null ) {
List violations = validateAMaxUnlimitedNatural(aMaxUnlimitedNatural);
if ( violations.isEmpty() ) {
this.aMaxUnlimitedNatural.add(aMaxUnlimitedNatural);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMaxUnlimitedNaturalIgnoreInverse(Integer aMaxUnlimitedNatural) {
if ( !this.aMaxUnlimitedNatural.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMaxUnlimitedNatural != null ) {
List violations = validateAMaxUnlimitedNatural(aMaxUnlimitedNatural);
if ( violations.isEmpty() ) {
this.aMaxUnlimitedNatural.add(aMaxUnlimitedNatural);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinInteger(Integer aMinInteger) {
if ( !this.aMinInteger.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMinInteger != null ) {
List violations = validateAMinInteger(aMinInteger);
if ( violations.isEmpty() ) {
this.aMinInteger.add(aMinInteger);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinIntegerIgnoreInverse(Integer aMinInteger) {
if ( !this.aMinInteger.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMinInteger != null ) {
List violations = validateAMinInteger(aMinInteger);
if ( violations.isEmpty() ) {
this.aMinInteger.add(aMinInteger);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinReal(Double aMinReal) {
if ( !this.aMinReal.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMinReal != null ) {
List violations = validateAMinReal(aMinReal);
if ( violations.isEmpty() ) {
this.aMinReal.add(aMinReal);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinRealIgnoreInverse(Double aMinReal) {
if ( !this.aMinReal.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMinReal != null ) {
List violations = validateAMinReal(aMinReal);
if ( violations.isEmpty() ) {
this.aMinReal.add(aMinReal);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinUnlimitedNatural(Integer aMinUnlimitedNatural) {
if ( !this.aMinUnlimitedNatural.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMinUnlimitedNatural != null ) {
List violations = validateAMinUnlimitedNatural(aMinUnlimitedNatural);
if ( violations.isEmpty() ) {
this.aMinUnlimitedNatural.add(aMinUnlimitedNatural);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToAMinUnlimitedNaturalIgnoreInverse(Integer aMinUnlimitedNatural) {
if ( !this.aMinUnlimitedNatural.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aMinUnlimitedNatural != null ) {
List violations = validateAMinUnlimitedNatural(aMinUnlimitedNatural);
if ( violations.isEmpty() ) {
this.aMinUnlimitedNatural.add(aMinUnlimitedNatural);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeInteger(Integer aRangeInteger) {
if ( !this.aRangeInteger.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aRangeInteger != null ) {
List violations = validateARangeInteger(aRangeInteger);
if ( violations.isEmpty() ) {
this.aRangeInteger.add(aRangeInteger);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeIntegerIgnoreInverse(Integer aRangeInteger) {
if ( !this.aRangeInteger.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aRangeInteger != null ) {
List violations = validateARangeInteger(aRangeInteger);
if ( violations.isEmpty() ) {
this.aRangeInteger.add(aRangeInteger);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeReal(Double aRangeReal) {
if ( !this.aRangeReal.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aRangeReal != null ) {
List violations = validateARangeReal(aRangeReal);
if ( violations.isEmpty() ) {
this.aRangeReal.add(aRangeReal);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeRealIgnoreInverse(Double aRangeReal) {
if ( !this.aRangeReal.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aRangeReal != null ) {
List violations = validateARangeReal(aRangeReal);
if ( violations.isEmpty() ) {
this.aRangeReal.add(aRangeReal);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeUnlimitedNatural(Integer aRangeUnlimitedNatural) {
if ( !this.aRangeUnlimitedNatural.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aRangeUnlimitedNatural != null ) {
List violations = validateARangeUnlimitedNatural(aRangeUnlimitedNatural);
if ( violations.isEmpty() ) {
this.aRangeUnlimitedNatural.add(aRangeUnlimitedNatural);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToARangeUnlimitedNaturalIgnoreInverse(Integer aRangeUnlimitedNatural) {
if ( !this.aRangeUnlimitedNatural.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( aRangeUnlimitedNatural != null ) {
List violations = validateARangeUnlimitedNatural(aRangeUnlimitedNatural);
if ( violations.isEmpty() ) {
this.aRangeUnlimitedNatural.add(aRangeUnlimitedNatural);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToTestUnlimitedNatural(Integer testUnlimitedNatural) {
if ( !this.testUnlimitedNatural.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( testUnlimitedNatural != null ) {
List violations = validateTestUnlimitedNatural(testUnlimitedNatural);
if ( violations.isEmpty() ) {
this.testUnlimitedNatural.add(testUnlimitedNatural);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
public void addToTestUnlimitedNaturalIgnoreInverse(Integer testUnlimitedNatural) {
if ( !this.testUnlimitedNatural.isEmpty() ) {
throw new RuntimeException("Property is a one and already has value, first clear it before adding!");
}
if ( testUnlimitedNatural != null ) {
List violations = validateTestUnlimitedNatural(testUnlimitedNatural);
if ( violations.isEmpty() ) {
this.testUnlimitedNatural.add(testUnlimitedNatural);
} else {
throw new UmlgConstraintViolationException(violations);
}
}
}
static public UmlgSet extends TestValidation> allInstances(Filter filter) {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(TestValidation.class.getName(), filter));
return result;
}
static public UmlgSet extends TestValidation> allInstances() {
UmlgSet result = new UmlgMemorySet();
result.addAll(UMLG.get().allInstances(TestValidation.class.getName()));
return result;
}
@Override
public List checkClassConstraints() {
List result = new ArrayList();
return result;
}
public void clearAMaxInteger() {
this.aMaxInteger.clear();
}
public void clearAMaxReal() {
this.aMaxReal.clear();
}
public void clearAMaxUnlimitedNatural() {
this.aMaxUnlimitedNatural.clear();
}
public void clearAMinInteger() {
this.aMinInteger.clear();
}
public void clearAMinReal() {
this.aMinReal.clear();
}
public void clearAMinUnlimitedNatural() {
this.aMinUnlimitedNatural.clear();
}
public void clearARangeInteger() {
this.aRangeInteger.clear();
}
public void clearARangeReal() {
this.aRangeReal.clear();
}
public void clearARangeUnlimitedNatural() {
this.aRangeUnlimitedNatural.clear();
}
public void clearTestUnlimitedNatural() {
this.testUnlimitedNatural.clear();
}
@Override
public void delete() {
TransactionThreadEntityVar.remove(this);
this.vertex.remove();
}
@Override
public void fromJson(Map propertyMap) {
fromJsonDataTypeAndComposite(propertyMap);
fromJsonNonCompositeOne(propertyMap);
fromJsonNonCompositeRequiredMany(propertyMap);
}
@Override
public void fromJson(String json) {
ObjectMapper mapper = ObjectMapperFactory.INSTANCE.getObjectMapper();
try {
@SuppressWarnings( "unchecked")
Map propertyMap = mapper.readValue(json, Map.class);
fromJson(propertyMap);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Override
public void fromJsonDataTypeAndComposite(Map propertyMap) {
Number aMinRealAsNumber = (Number)propertyMap.get("aMinReal");
Number aMaxIntegerAsNumber = (Number)propertyMap.get("aMaxInteger");
Number aRangeRealAsNumber = (Number)propertyMap.get("aRangeReal");
Number aRangeUnlimitedNaturalAsNumber = (Number)propertyMap.get("aRangeUnlimitedNatural");
Number aMinIntegerAsNumber = (Number)propertyMap.get("aMinInteger");
Number aMaxRealAsNumber = (Number)propertyMap.get("aMaxReal");
Number aRangeIntegerAsNumber = (Number)propertyMap.get("aRangeInteger");
Number aMinUnlimitedNaturalAsNumber = (Number)propertyMap.get("aMinUnlimitedNatural");
Number aMaxUnlimitedNaturalAsNumber = (Number)propertyMap.get("aMaxUnlimitedNatural");
Number testUnlimitedNaturalAsNumber = (Number)propertyMap.get("testUnlimitedNatural");
if ( propertyMap.containsKey("aMinReal") ) {
if ( propertyMap.get("aMinReal") != null ) {
Double aMinReal = aMinRealAsNumber != null ? aMinRealAsNumber.doubleValue() : null;
setAMinReal(aMinReal);
} else {
setAMinReal(null);
}
}
if ( propertyMap.containsKey("aMaxInteger") ) {
if ( propertyMap.get("aMaxInteger") != null ) {
Integer aMaxInteger = aMaxIntegerAsNumber != null ? aMaxIntegerAsNumber.intValue() : null;
setAMaxInteger(aMaxInteger);
} else {
setAMaxInteger(null);
}
}
if ( propertyMap.containsKey("aRangeReal") ) {
if ( propertyMap.get("aRangeReal") != null ) {
Double aRangeReal = aRangeRealAsNumber != null ? aRangeRealAsNumber.doubleValue() : null;
setARangeReal(aRangeReal);
} else {
setARangeReal(null);
}
}
if ( propertyMap.containsKey("aRangeUnlimitedNatural") ) {
if ( propertyMap.get("aRangeUnlimitedNatural") != null ) {
Integer aRangeUnlimitedNatural = aRangeUnlimitedNaturalAsNumber != null ? aRangeUnlimitedNaturalAsNumber.intValue() : null;
setARangeUnlimitedNatural(aRangeUnlimitedNatural);
} else {
setARangeUnlimitedNatural(null);
}
}
if ( propertyMap.containsKey("aMinInteger") ) {
if ( propertyMap.get("aMinInteger") != null ) {
Integer aMinInteger = aMinIntegerAsNumber != null ? aMinIntegerAsNumber.intValue() : null;
setAMinInteger(aMinInteger);
} else {
setAMinInteger(null);
}
}
if ( propertyMap.containsKey("aMaxReal") ) {
if ( propertyMap.get("aMaxReal") != null ) {
Double aMaxReal = aMaxRealAsNumber != null ? aMaxRealAsNumber.doubleValue() : null;
setAMaxReal(aMaxReal);
} else {
setAMaxReal(null);
}
}
if ( propertyMap.containsKey("aRangeInteger") ) {
if ( propertyMap.get("aRangeInteger") != null ) {
Integer aRangeInteger = aRangeIntegerAsNumber != null ? aRangeIntegerAsNumber.intValue() : null;
setARangeInteger(aRangeInteger);
} else {
setARangeInteger(null);
}
}
if ( propertyMap.containsKey("aMinUnlimitedNatural") ) {
if ( propertyMap.get("aMinUnlimitedNatural") != null ) {
Integer aMinUnlimitedNatural = aMinUnlimitedNaturalAsNumber != null ? aMinUnlimitedNaturalAsNumber.intValue() : null;
setAMinUnlimitedNatural(aMinUnlimitedNatural);
} else {
setAMinUnlimitedNatural(null);
}
}
if ( propertyMap.containsKey("aMaxUnlimitedNatural") ) {
if ( propertyMap.get("aMaxUnlimitedNatural") != null ) {
Integer aMaxUnlimitedNatural = aMaxUnlimitedNaturalAsNumber != null ? aMaxUnlimitedNaturalAsNumber.intValue() : null;
setAMaxUnlimitedNatural(aMaxUnlimitedNatural);
} else {
setAMaxUnlimitedNatural(null);
}
}
if ( propertyMap.containsKey("testUnlimitedNatural") ) {
if ( propertyMap.get("testUnlimitedNatural") != null ) {
Integer testUnlimitedNatural = testUnlimitedNaturalAsNumber != null ? testUnlimitedNaturalAsNumber.intValue() : null;
setTestUnlimitedNatural(testUnlimitedNatural);
} else {
setTestUnlimitedNatural(null);
}
}
if ( propertyMap.containsKey("tmpId") ) {
if ( propertyMap.get("tmpId") != null ) {
this.tmpId = (String)propertyMap.get("tmpId");
UmlgTmpIdManager.INSTANCE.put(this.tmpId, getId());
} else {
this.tmpId = null;
}
}
}
@Override
public void fromJsonNonCompositeOne(Map propertyMap) {
}
@Override
public void fromJsonNonCompositeRequiredMany(Map propertyMap) {
}
public Integer getAMaxInteger() {
UmlgSet tmp = this.aMaxInteger;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Double getAMaxReal() {
UmlgSet tmp = this.aMaxReal;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Integer getAMaxUnlimitedNatural() {
UmlgSet tmp = this.aMaxUnlimitedNatural;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Integer getAMinInteger() {
UmlgSet tmp = this.aMinInteger;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Double getAMinReal() {
UmlgSet tmp = this.aMinReal;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Integer getAMinUnlimitedNatural() {
UmlgSet tmp = this.aMinUnlimitedNatural;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Integer getARangeInteger() {
UmlgSet tmp = this.aRangeInteger;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Double getARangeReal() {
UmlgSet tmp = this.aRangeReal;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
public Integer getARangeUnlimitedNatural() {
UmlgSet tmp = this.aRangeUnlimitedNatural;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
@Override
public String getMetaDataAsJson() {
return TestValidation.TestValidationRuntimePropertyEnum.asJson();
}
@Override
public UmlgNode getOwningObject() {
return null;
}
@Override
public String getQualifiedName() {
return "umlgtest::org::umlg::validation::TestValidation";
}
/**
* getQualifiers is called from the collection in order to update the index used to implement the qualifier
*
* @param tumlRuntimeProperty
* @param node
* @param inverse
*/
@Override
public List getQualifiers(UmlgRuntimeProperty tumlRuntimeProperty, UmlgNode node, boolean inverse) {
List result = Collections.emptyList();
TestValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result.isEmpty() ) {
switch ( runtimeProperty ) {
default:
result = Collections.emptyList();
}
}
return result;
}
/**
* getSize is called from the BaseCollection.addInternal in order to save the size of the inverse collection to update the edge's sequence order
*
* @param inverse
* @param tumlRuntimeProperty
*/
@Override
public int getSize(boolean inverse, UmlgRuntimeProperty tumlRuntimeProperty) {
int result = 0;
TestValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName());
} else {
runtimeProperty = TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName());
}
if ( runtimeProperty != null && result == 0 ) {
switch ( runtimeProperty ) {
case aMinReal:
result = aMinReal.size();
break;
case aMaxInteger:
result = aMaxInteger.size();
break;
case aRangeReal:
result = aRangeReal.size();
break;
case aRangeUnlimitedNatural:
result = aRangeUnlimitedNatural.size();
break;
case aMinInteger:
result = aMinInteger.size();
break;
case aMaxReal:
result = aMaxReal.size();
break;
case aRangeInteger:
result = aRangeInteger.size();
break;
case aMinUnlimitedNatural:
result = aMinUnlimitedNatural.size();
break;
case aMaxUnlimitedNatural:
result = aMaxUnlimitedNatural.size();
break;
case testUnlimitedNatural:
result = testUnlimitedNatural.size();
break;
default:
result = 0;
}
}
return result;
}
public Integer getTestUnlimitedNatural() {
UmlgSet tmp = this.testUnlimitedNatural;
if ( !tmp.isEmpty() ) {
return tmp.iterator().next();
} else {
return null;
}
}
@Override
public String getUid() {
String uid;
if ( !this.vertex.property("uid").isPresent() && this.vertex.property("uid").value() != null ) {
uid=UUID.randomUUID().toString();
this.vertex.property("uid", uid);
} else {
uid=this.vertex.value("uid");
}
return uid;
}
@Override
public boolean hasOnlyOneCompositeParent() {
int result = 0;
return result == 1;
}
public void initDataTypeVariablesWithDefaultValues() {
}
public void initVariables() {
}
/**
* boolean properties' default values are initialized in the constructor via z_internalBooleanProperties
*
* @param loaded
*/
@Override
public void initialiseProperties(boolean loaded) {
this.aMinReal = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMinReal), loaded);
this.aMaxInteger = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMaxInteger), loaded);
this.aRangeReal = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aRangeReal), loaded);
this.aRangeUnlimitedNatural = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aRangeUnlimitedNatural), loaded);
this.aMinInteger = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMinInteger), loaded);
this.aMaxReal = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMaxReal), loaded);
this.aRangeInteger = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aRangeInteger), loaded);
this.aMinUnlimitedNatural = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMinUnlimitedNatural), loaded);
this.aMaxUnlimitedNatural = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMaxUnlimitedNatural), loaded);
this.testUnlimitedNatural = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.testUnlimitedNatural), loaded);
}
@Override
public void initialiseProperty(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, boolean loaded) {
TestValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMinReal:
this.aMinReal = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMinReal), loaded);
break;
case aMaxInteger:
this.aMaxInteger = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMaxInteger), loaded);
break;
case aRangeReal:
this.aRangeReal = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aRangeReal), loaded);
break;
case aRangeUnlimitedNatural:
this.aRangeUnlimitedNatural = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aRangeUnlimitedNatural), loaded);
break;
case aMinInteger:
this.aMinInteger = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMinInteger), loaded);
break;
case aMaxReal:
this.aMaxReal = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMaxReal), loaded);
break;
case aRangeInteger:
this.aRangeInteger = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aRangeInteger), loaded);
break;
case aMinUnlimitedNatural:
this.aMinUnlimitedNatural = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMinUnlimitedNatural), loaded);
break;
case aMaxUnlimitedNatural:
this.aMaxUnlimitedNatural = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.aMaxUnlimitedNatural), loaded);
break;
case testUnlimitedNatural:
this.testUnlimitedNatural = new UmlgSetImpl(this, PropertyTree.from(TestValidationRuntimePropertyEnum.testUnlimitedNatural), loaded);
break;
}
}
}
@Override
public UmlgRuntimeProperty inverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
TestValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
}
return runtimeProperty;
} else {
return null;
}
}
@Override
public boolean isTinkerRoot() {
return true;
}
public void removeFromAMaxInteger(Integer aMaxInteger) {
if ( aMaxInteger != null ) {
this.aMaxInteger.remove(aMaxInteger);
}
}
public void removeFromAMaxInteger(UmlgSet aMaxInteger) {
if ( !aMaxInteger.isEmpty() ) {
this.aMaxInteger.removeAll(aMaxInteger);
}
}
public void removeFromAMaxReal(Double aMaxReal) {
if ( aMaxReal != null ) {
this.aMaxReal.remove(aMaxReal);
}
}
public void removeFromAMaxReal(UmlgSet aMaxReal) {
if ( !aMaxReal.isEmpty() ) {
this.aMaxReal.removeAll(aMaxReal);
}
}
public void removeFromAMaxUnlimitedNatural(Integer aMaxUnlimitedNatural) {
if ( aMaxUnlimitedNatural != null ) {
this.aMaxUnlimitedNatural.remove(aMaxUnlimitedNatural);
}
}
public void removeFromAMaxUnlimitedNatural(UmlgSet aMaxUnlimitedNatural) {
if ( !aMaxUnlimitedNatural.isEmpty() ) {
this.aMaxUnlimitedNatural.removeAll(aMaxUnlimitedNatural);
}
}
public void removeFromAMinInteger(Integer aMinInteger) {
if ( aMinInteger != null ) {
this.aMinInteger.remove(aMinInteger);
}
}
public void removeFromAMinInteger(UmlgSet aMinInteger) {
if ( !aMinInteger.isEmpty() ) {
this.aMinInteger.removeAll(aMinInteger);
}
}
public void removeFromAMinReal(Double aMinReal) {
if ( aMinReal != null ) {
this.aMinReal.remove(aMinReal);
}
}
public void removeFromAMinReal(UmlgSet aMinReal) {
if ( !aMinReal.isEmpty() ) {
this.aMinReal.removeAll(aMinReal);
}
}
public void removeFromAMinUnlimitedNatural(Integer aMinUnlimitedNatural) {
if ( aMinUnlimitedNatural != null ) {
this.aMinUnlimitedNatural.remove(aMinUnlimitedNatural);
}
}
public void removeFromAMinUnlimitedNatural(UmlgSet aMinUnlimitedNatural) {
if ( !aMinUnlimitedNatural.isEmpty() ) {
this.aMinUnlimitedNatural.removeAll(aMinUnlimitedNatural);
}
}
public void removeFromARangeInteger(Integer aRangeInteger) {
if ( aRangeInteger != null ) {
this.aRangeInteger.remove(aRangeInteger);
}
}
public void removeFromARangeInteger(UmlgSet aRangeInteger) {
if ( !aRangeInteger.isEmpty() ) {
this.aRangeInteger.removeAll(aRangeInteger);
}
}
public void removeFromARangeReal(Double aRangeReal) {
if ( aRangeReal != null ) {
this.aRangeReal.remove(aRangeReal);
}
}
public void removeFromARangeReal(UmlgSet aRangeReal) {
if ( !aRangeReal.isEmpty() ) {
this.aRangeReal.removeAll(aRangeReal);
}
}
public void removeFromARangeUnlimitedNatural(Integer aRangeUnlimitedNatural) {
if ( aRangeUnlimitedNatural != null ) {
this.aRangeUnlimitedNatural.remove(aRangeUnlimitedNatural);
}
}
public void removeFromARangeUnlimitedNatural(UmlgSet aRangeUnlimitedNatural) {
if ( !aRangeUnlimitedNatural.isEmpty() ) {
this.aRangeUnlimitedNatural.removeAll(aRangeUnlimitedNatural);
}
}
public void removeFromTestUnlimitedNatural(Integer testUnlimitedNatural) {
if ( testUnlimitedNatural != null ) {
this.testUnlimitedNatural.remove(testUnlimitedNatural);
}
}
public void removeFromTestUnlimitedNatural(UmlgSet testUnlimitedNatural) {
if ( !testUnlimitedNatural.isEmpty() ) {
this.testUnlimitedNatural.removeAll(testUnlimitedNatural);
}
}
public void setAMaxInteger(Integer aMaxInteger) {
if ( aMaxInteger == null ) {
clearAMaxInteger();
} else {
z_internalClearAMaxInteger();
}
addToAMaxInteger(aMaxInteger);
}
public void setAMaxReal(Double aMaxReal) {
if ( aMaxReal == null ) {
clearAMaxReal();
} else {
z_internalClearAMaxReal();
}
addToAMaxReal(aMaxReal);
}
public void setAMaxUnlimitedNatural(Integer aMaxUnlimitedNatural) {
if ( aMaxUnlimitedNatural == null ) {
clearAMaxUnlimitedNatural();
} else {
z_internalClearAMaxUnlimitedNatural();
}
addToAMaxUnlimitedNatural(aMaxUnlimitedNatural);
}
public void setAMinInteger(Integer aMinInteger) {
if ( aMinInteger == null ) {
clearAMinInteger();
} else {
z_internalClearAMinInteger();
}
addToAMinInteger(aMinInteger);
}
public void setAMinReal(Double aMinReal) {
if ( aMinReal == null ) {
clearAMinReal();
} else {
z_internalClearAMinReal();
}
addToAMinReal(aMinReal);
}
public void setAMinUnlimitedNatural(Integer aMinUnlimitedNatural) {
if ( aMinUnlimitedNatural == null ) {
clearAMinUnlimitedNatural();
} else {
z_internalClearAMinUnlimitedNatural();
}
addToAMinUnlimitedNatural(aMinUnlimitedNatural);
}
public void setARangeInteger(Integer aRangeInteger) {
if ( aRangeInteger == null ) {
clearARangeInteger();
} else {
z_internalClearARangeInteger();
}
addToARangeInteger(aRangeInteger);
}
public void setARangeReal(Double aRangeReal) {
if ( aRangeReal == null ) {
clearARangeReal();
} else {
z_internalClearARangeReal();
}
addToARangeReal(aRangeReal);
}
public void setARangeUnlimitedNatural(Integer aRangeUnlimitedNatural) {
if ( aRangeUnlimitedNatural == null ) {
clearARangeUnlimitedNatural();
} else {
z_internalClearARangeUnlimitedNatural();
}
addToARangeUnlimitedNatural(aRangeUnlimitedNatural);
}
public void setTestUnlimitedNatural(Integer testUnlimitedNatural) {
if ( testUnlimitedNatural == null ) {
clearTestUnlimitedNatural();
} else {
z_internalClearTestUnlimitedNatural();
}
addToTestUnlimitedNatural(testUnlimitedNatural);
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJson(Boolean deep) {
StringBuilder sb = new StringBuilder();
if ( this.tmpId != null ) {
sb.append("\"tmpId\": \"" + this.tmpId + "\", ");
}
sb.append("\"id\": \"" + getId() + "\", ");
sb.append("\"aMinReal\": " + getAMinReal() + "");
sb.append(", ");
sb.append("\"aMaxInteger\": " + getAMaxInteger() + "");
sb.append(", ");
sb.append("\"aRangeReal\": " + getARangeReal() + "");
sb.append(", ");
sb.append("\"aRangeUnlimitedNatural\": " + getARangeUnlimitedNatural() + "");
sb.append(", ");
sb.append("\"aMinInteger\": " + getAMinInteger() + "");
sb.append(", ");
sb.append("\"aMaxReal\": " + getAMaxReal() + "");
sb.append(", ");
sb.append("\"aRangeInteger\": " + getARangeInteger() + "");
sb.append(", ");
sb.append("\"aMinUnlimitedNatural\": " + getAMinUnlimitedNatural() + "");
sb.append(", ");
sb.append("\"aMaxUnlimitedNatural\": " + getAMaxUnlimitedNatural() + "");
sb.append(", ");
sb.append("\"testUnlimitedNatural\": " + getTestUnlimitedNatural() + "");
sb.append(", ");
sb.append("\"qualifiedName\": \"" + getQualifiedName() + "\"");
sb.append(", ");
//PlaceHolder for restful
sb.append("\"uri\": {}");
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJson() {
return toJson(false);
}
/**
* deep indicates that components also be serialized.
*
* @param deep
*/
@Override
public String toJsonWithoutCompositeParent(Boolean deep) {
StringBuilder sb = new StringBuilder();
if ( this.tmpId != null ) {
sb.append("\"tmpId\": \"" + this.tmpId + "\", ");
}
sb.append("\"id\": \"" + getId() + "\", ");
sb.append("\"aMinReal\": " + getAMinReal() + "");
sb.append(", ");
sb.append("\"aMaxInteger\": " + getAMaxInteger() + "");
sb.append(", ");
sb.append("\"aRangeReal\": " + getARangeReal() + "");
sb.append(", ");
sb.append("\"aRangeUnlimitedNatural\": " + getARangeUnlimitedNatural() + "");
sb.append(", ");
sb.append("\"aMinInteger\": " + getAMinInteger() + "");
sb.append(", ");
sb.append("\"aMaxReal\": " + getAMaxReal() + "");
sb.append(", ");
sb.append("\"aRangeInteger\": " + getARangeInteger() + "");
sb.append(", ");
sb.append("\"aMinUnlimitedNatural\": " + getAMinUnlimitedNatural() + "");
sb.append(", ");
sb.append("\"aMaxUnlimitedNatural\": " + getAMaxUnlimitedNatural() + "");
sb.append(", ");
sb.append("\"testUnlimitedNatural\": " + getTestUnlimitedNatural() + "");
sb.append(", ");
sb.append("\"qualifiedName\": \"" + getQualifiedName() + "\"");
sb.append(", ");
//PlaceHolder for restful
sb.append("\"uri\": {}");
sb.insert(0, "{");
sb.append("}");
return sb.toString();
}
@Override
public String toJsonWithoutCompositeParent() {
return toJsonWithoutCompositeParent(false);
}
public List validateAMaxInteger(Integer aMaxInteger) {
List result = new ArrayList();
if ( !UmlgValidator.validateMaxInteger(aMaxInteger, 5) ) {
result.add(new UmlgConstraintViolation("MaxInteger", "umlgtest::org::umlg::validation::TestValidation::aMaxInteger", "MaxInteger does not pass validation!"));
}
return result;
}
public List validateAMaxReal(Double aMaxReal) {
List result = new ArrayList();
if ( !UmlgValidator.validateMaxReal(aMaxReal, 5.123) ) {
result.add(new UmlgConstraintViolation("MaxReal", "umlgtest::org::umlg::validation::TestValidation::aMaxReal", "MaxReal does not pass validation!"));
}
return result;
}
public List validateAMaxUnlimitedNatural(Integer aMaxUnlimitedNatural) {
List result = new ArrayList();
if ( !UmlgValidator.validateValidUnlimitedNatural(aMaxUnlimitedNatural) ) {
result.add(new UmlgConstraintViolation("UnlimitedNatural", "umlgtest::org::umlg::validation::TestValidation::aMaxUnlimitedNatural", " must be greater or equal to zero!"));
}
if ( !UmlgValidator.validateMaxUnlimitedNatural(aMaxUnlimitedNatural, 0) ) {
result.add(new UmlgConstraintViolation("MaxUnlimitedNatural", "umlgtest::org::umlg::validation::TestValidation::aMaxUnlimitedNatural", "MaxUnlimitedNatural does not pass validation!"));
}
return result;
}
public List validateAMinInteger(Integer aMinInteger) {
List result = new ArrayList();
if ( !UmlgValidator.validateMinInteger(aMinInteger, 5) ) {
result.add(new UmlgConstraintViolation("MinInteger", "umlgtest::org::umlg::validation::TestValidation::aMinInteger", "MinInteger does not pass validation!"));
}
return result;
}
public List validateAMinReal(Double aMinReal) {
List result = new ArrayList();
if ( !UmlgValidator.validateMinReal(aMinReal, 5.123) ) {
result.add(new UmlgConstraintViolation("MinReal", "umlgtest::org::umlg::validation::TestValidation::aMinReal", "MinReal does not pass validation!"));
}
return result;
}
public List validateAMinUnlimitedNatural(Integer aMinUnlimitedNatural) {
List result = new ArrayList();
if ( !UmlgValidator.validateValidUnlimitedNatural(aMinUnlimitedNatural) ) {
result.add(new UmlgConstraintViolation("UnlimitedNatural", "umlgtest::org::umlg::validation::TestValidation::aMinUnlimitedNatural", " must be greater or equal to zero!"));
}
if ( !UmlgValidator.validateMinUnlimitedNatural(aMinUnlimitedNatural, 2147483646) ) {
result.add(new UmlgConstraintViolation("MinUnlimitedNatural", "umlgtest::org::umlg::validation::TestValidation::aMinUnlimitedNatural", "MinUnlimitedNatural does not pass validation!"));
}
return result;
}
public List validateARangeInteger(Integer aRangeInteger) {
List result = new ArrayList();
if ( !UmlgValidator.validateRangeInteger(aRangeInteger, 1, 5) ) {
result.add(new UmlgConstraintViolation("RangeInteger", "umlgtest::org::umlg::validation::TestValidation::aRangeInteger", "RangeInteger does not pass validation!"));
}
return result;
}
public List validateARangeReal(Double aRangeReal) {
List result = new ArrayList();
if ( !UmlgValidator.validateRangeReal(aRangeReal, 0.0, 5.123) ) {
result.add(new UmlgConstraintViolation("RangeReal", "umlgtest::org::umlg::validation::TestValidation::aRangeReal", "RangeReal does not pass validation!"));
}
return result;
}
public List validateARangeUnlimitedNatural(Integer aRangeUnlimitedNatural) {
List result = new ArrayList();
if ( !UmlgValidator.validateValidUnlimitedNatural(aRangeUnlimitedNatural) ) {
result.add(new UmlgConstraintViolation("UnlimitedNatural", "umlgtest::org::umlg::validation::TestValidation::aRangeUnlimitedNatural", " must be greater or equal to zero!"));
}
if ( !UmlgValidator.validateRangeUnlimitedNatural(aRangeUnlimitedNatural, 1, 2147483645) ) {
result.add(new UmlgConstraintViolation("RangeUnlimitedNatural", "umlgtest::org::umlg::validation::TestValidation::aRangeUnlimitedNatural", "RangeUnlimitedNatural does not pass validation!"));
}
return result;
}
@Override
public List validateMultiplicities() {
List result = new ArrayList();
return result;
}
public List validateTestUnlimitedNatural(Integer testUnlimitedNatural) {
List result = new ArrayList();
if ( !UmlgValidator.validateValidUnlimitedNatural(testUnlimitedNatural) ) {
result.add(new UmlgConstraintViolation("UnlimitedNatural", "umlgtest::org::umlg::validation::TestValidation::testUnlimitedNatural", " must be greater or equal to zero!"));
}
return result;
}
@Override
public void z_internalAddPersistentValueToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
TestValidationRuntimePropertyEnum runtimeProperty;
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMinReal:
this.aMinReal.z_internalAdder((double)umlgNode);
break;
case aMaxInteger:
this.aMaxInteger.z_internalAdder((int)umlgNode);
break;
case aRangeReal:
this.aRangeReal.z_internalAdder((double)umlgNode);
break;
case aRangeUnlimitedNatural:
this.aRangeUnlimitedNatural.z_internalAdder((int)umlgNode);
break;
case aMinInteger:
this.aMinInteger.z_internalAdder((int)umlgNode);
break;
case aMaxReal:
this.aMaxReal.z_internalAdder((double)umlgNode);
break;
case aRangeInteger:
this.aRangeInteger.z_internalAdder((int)umlgNode);
break;
case aMinUnlimitedNatural:
this.aMinUnlimitedNatural.z_internalAdder((int)umlgNode);
break;
case aMaxUnlimitedNatural:
this.aMaxUnlimitedNatural.z_internalAdder((int)umlgNode);
break;
case testUnlimitedNatural:
this.testUnlimitedNatural.z_internalAdder((int)umlgNode);
break;
}
}
}
@Override
public void z_internalAddToCollection(UmlgRuntimeProperty umlgRuntimeProperty, Object umlgNode) {
TestValidationRuntimePropertyEnum runtimeProperty;
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMinReal:
this.aMinReal.z_internalAdder((Double)umlgNode);
break;
case aMaxInteger:
this.aMaxInteger.z_internalAdder((Integer)umlgNode);
break;
case aRangeReal:
this.aRangeReal.z_internalAdder((Double)umlgNode);
break;
case aRangeUnlimitedNatural:
this.aRangeUnlimitedNatural.z_internalAdder((Integer)umlgNode);
break;
case aMinInteger:
this.aMinInteger.z_internalAdder((Integer)umlgNode);
break;
case aMaxReal:
this.aMaxReal.z_internalAdder((Double)umlgNode);
break;
case aRangeInteger:
this.aRangeInteger.z_internalAdder((Integer)umlgNode);
break;
case aMinUnlimitedNatural:
this.aMinUnlimitedNatural.z_internalAdder((Integer)umlgNode);
break;
case aMaxUnlimitedNatural:
this.aMaxUnlimitedNatural.z_internalAdder((Integer)umlgNode);
break;
case testUnlimitedNatural:
this.testUnlimitedNatural.z_internalAdder((Integer)umlgNode);
break;
}
}
}
@Override
public Set z_internalBooleanProperties() {
Set result = new HashSet();
return result;
}
public void z_internalClearAMaxInteger() {
this.aMaxInteger.z_internalClear();
}
public void z_internalClearAMaxReal() {
this.aMaxReal.z_internalClear();
}
public void z_internalClearAMaxUnlimitedNatural() {
this.aMaxUnlimitedNatural.z_internalClear();
}
public void z_internalClearAMinInteger() {
this.aMinInteger.z_internalClear();
}
public void z_internalClearAMinReal() {
this.aMinReal.z_internalClear();
}
public void z_internalClearAMinUnlimitedNatural() {
this.aMinUnlimitedNatural.z_internalClear();
}
public void z_internalClearARangeInteger() {
this.aRangeInteger.z_internalClear();
}
public void z_internalClearARangeReal() {
this.aRangeReal.z_internalClear();
}
public void z_internalClearARangeUnlimitedNatural() {
this.aRangeUnlimitedNatural.z_internalClear();
}
public void z_internalClearTestUnlimitedNatural() {
this.testUnlimitedNatural.z_internalClear();
}
@Override
public Set z_internalDataTypeProperties() {
Set result = new HashSet();
result.add(TestValidationRuntimePropertyEnum.aMinReal);
result.add(TestValidationRuntimePropertyEnum.aMaxInteger);
result.add(TestValidationRuntimePropertyEnum.aRangeReal);
result.add(TestValidationRuntimePropertyEnum.aRangeUnlimitedNatural);
result.add(TestValidationRuntimePropertyEnum.aMinInteger);
result.add(TestValidationRuntimePropertyEnum.aMaxReal);
result.add(TestValidationRuntimePropertyEnum.aRangeInteger);
result.add(TestValidationRuntimePropertyEnum.aMinUnlimitedNatural);
result.add(TestValidationRuntimePropertyEnum.aMaxUnlimitedNatural);
result.add(TestValidationRuntimePropertyEnum.testUnlimitedNatural);
return result;
}
@Override
public Map z_internalDataTypePropertiesWithDefaultValues() {
Map result = new HashMap();
return result;
}
@Override
public UmlgCollection extends Object> z_internalGetCollectionFor(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse) {
UmlgCollection extends Object> result = null;
if ( result == null ) {
TestValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMinReal:
result = this.aMinReal;
break;
case aMaxInteger:
result = this.aMaxInteger;
break;
case aRangeReal:
result = this.aRangeReal;
break;
case aRangeUnlimitedNatural:
result = this.aRangeUnlimitedNatural;
break;
case aMinInteger:
result = this.aMinInteger;
break;
case aMaxReal:
result = this.aMaxReal;
break;
case aRangeInteger:
result = this.aRangeInteger;
break;
case aMinUnlimitedNatural:
result = this.aMinUnlimitedNatural;
break;
case aMaxUnlimitedNatural:
result = this.aMaxUnlimitedNatural;
break;
case testUnlimitedNatural:
result = this.testUnlimitedNatural;
break;
}
}
}
return result;
}
@Override
public UmlgRuntimeProperty z_internalInverseAdder(UmlgRuntimeProperty tumlRuntimeProperty, boolean inverse, UmlgNode umlgNode) {
TestValidationRuntimePropertyEnum runtimeProperty;
if ( !inverse ) {
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getQualifiedName()));
} else {
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(tumlRuntimeProperty.getInverseQualifiedName()));
}
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
}
return runtimeProperty;
} else {
return null;
}
}
@Override
public void z_internalMarkCollectionLoaded(UmlgRuntimeProperty umlgRuntimeProperty, boolean loaded) {
TestValidationRuntimePropertyEnum runtimeProperty;
runtimeProperty = (TestValidationRuntimePropertyEnum.fromQualifiedName(umlgRuntimeProperty.getQualifiedName()));
if ( runtimeProperty != null ) {
switch ( runtimeProperty ) {
case aMinReal:
this.aMinReal.setLoaded(loaded);
break;
case aMaxInteger:
this.aMaxInteger.setLoaded(loaded);
break;
case aRangeReal:
this.aRangeReal.setLoaded(loaded);
break;
case aRangeUnlimitedNatural:
this.aRangeUnlimitedNatural.setLoaded(loaded);
break;
case aMinInteger:
this.aMinInteger.setLoaded(loaded);
break;
case aMaxReal:
this.aMaxReal.setLoaded(loaded);
break;
case aRangeInteger:
this.aRangeInteger.setLoaded(loaded);
break;
case aMinUnlimitedNatural:
this.aMinUnlimitedNatural.setLoaded(loaded);
break;
case aMaxUnlimitedNatural:
this.aMaxUnlimitedNatural.setLoaded(loaded);
break;
case testUnlimitedNatural:
this.testUnlimitedNatural.setLoaded(loaded);
break;
}
}
}
static public enum TestValidationRuntimePropertyEnum implements UmlgRuntimeProperty {
aMinReal(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aMinReal",/* persistentName */ "aMinReal",/* inverseName */ "inverseOf::aMinReal",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aMinReal",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MinReal(5.123)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMinReal"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Double.class,/* json */ "{\"name\": \"aMinReal\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"min\": 5.123}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aMinReal\", \"persistentName\": \"aMinReal\", \"inverseName\": \"inverseOf::aMinReal\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aMinReal\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMinReal\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMaxInteger(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aMaxInteger",/* persistentName */ "aMaxInteger",/* inverseName */ "inverseOf::aMaxInteger",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aMaxInteger",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MaxInteger(5)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMaxInteger"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aMaxInteger\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"max\": 5}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aMaxInteger\", \"persistentName\": \"aMaxInteger\", \"inverseName\": \"inverseOf::aMaxInteger\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aMaxInteger\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMaxInteger\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aRangeReal(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aRangeReal",/* persistentName */ "aRangeReal",/* inverseName */ "inverseOf::aRangeReal",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aRangeReal",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new RangeReal(0.0, 5.123)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aRangeReal"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Double.class,/* json */ "{\"name\": \"aRangeReal\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"range\": {\"min\": 0.0, \"max\": 5.123}}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aRangeReal\", \"persistentName\": \"aRangeReal\", \"inverseName\": \"inverseOf::aRangeReal\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aRangeReal\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aRangeReal\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aRangeUnlimitedNatural(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aRangeUnlimitedNatural",/* persistentName */ "aRangeUnlimitedNatural",/* inverseName */ "inverseOf::aRangeUnlimitedNatural",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aRangeUnlimitedNatural",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new RangeUnlimitedNatural(1, 2147483645)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aRangeUnlimitedNatural"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aRangeUnlimitedNatural\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"range\": {\"min\": 1, \"max\": 2147483645}}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aRangeUnlimitedNatural\", \"persistentName\": \"aRangeUnlimitedNatural\", \"inverseName\": \"inverseOf::aRangeUnlimitedNatural\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aRangeUnlimitedNatural\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aRangeUnlimitedNatural\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMinInteger(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aMinInteger",/* persistentName */ "aMinInteger",/* inverseName */ "inverseOf::aMinInteger",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aMinInteger",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MinInteger(5)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMinInteger"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aMinInteger\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"min\": 5}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aMinInteger\", \"persistentName\": \"aMinInteger\", \"inverseName\": \"inverseOf::aMinInteger\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aMinInteger\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMinInteger\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMaxReal(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aMaxReal",/* persistentName */ "aMaxReal",/* inverseName */ "inverseOf::aMaxReal",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aMaxReal",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MaxReal(5.123)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMaxReal"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Double.class,/* json */ "{\"name\": \"aMaxReal\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"max\": 5.123}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aMaxReal\", \"persistentName\": \"aMaxReal\", \"inverseName\": \"inverseOf::aMaxReal\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aMaxReal\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMaxReal\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aRangeInteger(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aRangeInteger",/* persistentName */ "aRangeInteger",/* inverseName */ "inverseOf::aRangeInteger",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aRangeInteger",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new RangeInteger(1, 5)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aRangeInteger"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aRangeInteger\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"range\": {\"min\": 1, \"max\": 5}}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aRangeInteger\", \"persistentName\": \"aRangeInteger\", \"inverseName\": \"inverseOf::aRangeInteger\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aRangeInteger\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aRangeInteger\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMinUnlimitedNatural(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aMinUnlimitedNatural",/* persistentName */ "aMinUnlimitedNatural",/* inverseName */ "inverseOf::aMinUnlimitedNatural",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aMinUnlimitedNatural",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MinUnlimitedNatural(2147483646)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMinUnlimitedNatural"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aMinUnlimitedNatural\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"min\": 2147483646}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aMinUnlimitedNatural\", \"persistentName\": \"aMinUnlimitedNatural\", \"inverseName\": \"inverseOf::aMinUnlimitedNatural\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aMinUnlimitedNatural\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMinUnlimitedNatural\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
aMaxUnlimitedNatural(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::aMaxUnlimitedNatural",/* persistentName */ "aMaxUnlimitedNatural",/* inverseName */ "inverseOf::aMaxUnlimitedNatural",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::aMaxUnlimitedNatural",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Arrays.asList(new MaxUnlimitedNatural(0)),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("aMaxUnlimitedNatural"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"aMaxUnlimitedNatural\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": {\"max\": 0}, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::aMaxUnlimitedNatural\", \"persistentName\": \"aMaxUnlimitedNatural\", \"inverseName\": \"inverseOf::aMaxUnlimitedNatural\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::aMaxUnlimitedNatural\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"aMaxUnlimitedNatural\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
testUnlimitedNatural(/* qualifiedName */ "umlgtest::org::umlg::validation::TestValidation::testUnlimitedNatural",/* persistentName */ "testUnlimitedNatural",/* inverseName */ "inverseOf::testUnlimitedNatural",/* inverseQualifiedName */ "inverseOf::umlgtest::org::umlg::validation::TestValidation::testUnlimitedNatural",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ true,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ false,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("testUnlimitedNatural"),/* isOneToOne */ false,/* isOneToMany */ false,/* isManyToOne */ true,/* isManyToMany */ false,/* upper */ 1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ true,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ true,/* propertyType */ Integer.class,/* json */ "{\"name\": \"testUnlimitedNatural\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": true, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation::testUnlimitedNatural\", \"persistentName\": \"testUnlimitedNatural\", \"inverseName\": \"inverseOf::testUnlimitedNatural\", \"inverseQualifiedName\": \"inverseOf::umlgtest::org::umlg::validation::TestValidation::testUnlimitedNatural\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": false, \"oneToOne\": false, \"oneToMany\": false, \"manyToOne\": true, \"manyToMany\": false, \"upper\": 1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"testUnlimitedNatural\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": true, \"inverseUnique\": false, \"derived\": false, \"navigable\": true}",/* isChangeListenerAttribute */ false),
umlgtest(/* qualifiedName */ "umlgtest",/* persistentName */ "umlgtest",/* inverseName */ "inverseOfumlgtest",/* inverseQualifiedName */ "inverseOfumlgtest",/* isAssociationClassOne */ false,/* isMemberEndOfAssociationClass */ false,/* associationClassPropertyNameField */ "null",/* inverseAssociationClassPropertyNameField */ "null",/* isAssociationClassProperty */ false,/* isOnePrimitivePropertyOfAssociationClass */ false,/* isOnePrimitive */ false,/* isReadOnly */ false,/* dataTypeEnum */ null,/* validations */ Collections.emptyList(),/* isManyPrimitive */ false,/* oneEnumeration */ false,/* manyEnumeration */ false,/* isControllingSide */ true,/* isComposite */ false,/* isInverseComposite */ true,/* label */ UmlgLabelConverterFactory.getUmlgLabelConverter().convert("rootTestValidation"),/* isOneToOne */ true,/* isOneToMany */ false,/* isManyToOne */ false,/* isManyToMany */ false,/* upper */ -1,/* lower */ 0,/* inverseUpper */ 1,/* isQualified */ false,/* isInverseQualified */ false,/* isOrdered */ false,/* isInverseOrdered */ false,/* isUnique */ false,/* isInverseUnique */ false,/* isDerived */ false,/* isNavigable */ false,/* propertyType */ Object.class,/* json */ "{\"name\": \"umlgtest\", \"associationClassOne\": false, \"memberEndOfAssociationClass\": false, \"associationClassPropertyName\": null, \"inverseAssociationClassPropertyName\": null, \"associationClassProperty\": false, \"onePrimitivePropertyOfAssociationClass\": false, \"onePrimitive\": false, \"readOnly\": false, \"dataTypeEnum\": null, \"validations\": null, \"qualifiedName\": \"umlgtest\", \"persistentName\": \"umlgtest\", \"inverseName\": \"inverseOfumlgtest\", \"inverseQualifiedName\": \"inverseOfumlgtest\", \"manyPrimitive\": false, \"oneEnumeration\": false, \"manyEnumeration\": false, \"controllingSide\": true, \"composite\": false, \"inverseComposite\": true, \"oneToOne\": true, \"oneToMany\": false, \"manyToOne\": false, \"manyToMany\": false, \"upper\": -1, \"lower\": 0, \"inverseUpper\": 1, \"label\": \"rootTestValidation\", \"qualified\": false, \"inverseQualified\": false, \"ordered\": false, \"inverseOrdered\": false, \"unique\": false, \"inverseUnique\": false, \"derived\": false, \"navigable\": false}",/* isChangeListenerAttribute */ false);
private String _qualifiedName;
private String _persistentName;
private String _inverseName;
private String _inverseQualifiedName;
private boolean _associationClassOne;
private boolean _memberEndOfAssociationClass;
private String _associationClassPropertyName;
private String _inverseAssociationClassPropertyName;
private boolean _associationClassProperty;
private boolean _onePrimitivePropertyOfAssociationClass;
private boolean _onePrimitive;
private Boolean _readOnly;
private DataTypeEnum dataTypeEnum;
private List validations;
private boolean _manyPrimitive;
private boolean _oneEnumeration;
private boolean _manyEnumeration;
private boolean _controllingSide;
private boolean _composite;
private boolean _inverseComposite;
private String _label;
private boolean _oneToOne;
private boolean _oneToMany;
private boolean _manyToOne;
private boolean _manyToMany;
private int _upper;
private int _lower;
private int _inverseUpper;
private boolean _qualified;
private boolean _inverseQualified;
private boolean _ordered;
private boolean _inverseOrdered;
private boolean _unique;
private boolean _inverseUnique;
private boolean _derived;
private boolean _navigability;
private Class _propertyType;
private String _json;
private boolean _changeListener;
/**
* constructor for TestValidationRuntimePropertyEnum
*
* @param _qualifiedName
* @param _persistentName
* @param _inverseName
* @param _inverseQualifiedName
* @param _associationClassOne
* @param _memberEndOfAssociationClass
* @param _associationClassPropertyName
* @param _inverseAssociationClassPropertyName
* @param _associationClassProperty
* @param _onePrimitivePropertyOfAssociationClass
* @param _onePrimitive
* @param _readOnly
* @param dataTypeEnum
* @param validations
* @param _manyPrimitive
* @param _oneEnumeration
* @param _manyEnumeration
* @param _controllingSide
* @param _composite
* @param _inverseComposite
* @param _label
* @param _oneToOne
* @param _oneToMany
* @param _manyToOne
* @param _manyToMany
* @param _upper
* @param _lower
* @param _inverseUpper
* @param _qualified
* @param _inverseQualified
* @param _ordered
* @param _inverseOrdered
* @param _unique
* @param _inverseUnique
* @param _derived
* @param _navigability
* @param _propertyType
* @param _json
* @param _changeListener
*/
private TestValidationRuntimePropertyEnum(String _qualifiedName, String _persistentName, String _inverseName, String _inverseQualifiedName, boolean _associationClassOne, boolean _memberEndOfAssociationClass, String _associationClassPropertyName, String _inverseAssociationClassPropertyName, boolean _associationClassProperty, boolean _onePrimitivePropertyOfAssociationClass, boolean _onePrimitive, Boolean _readOnly, DataTypeEnum dataTypeEnum, List validations, boolean _manyPrimitive, boolean _oneEnumeration, boolean _manyEnumeration, boolean _controllingSide, boolean _composite, boolean _inverseComposite, String _label, boolean _oneToOne, boolean _oneToMany, boolean _manyToOne, boolean _manyToMany, int _upper, int _lower, int _inverseUpper, boolean _qualified, boolean _inverseQualified, boolean _ordered, boolean _inverseOrdered, boolean _unique, boolean _inverseUnique, boolean _derived, boolean _navigability, Class _propertyType, String _json, boolean _changeListener) {
this._qualifiedName = _qualifiedName;
this._persistentName = _persistentName;
this._inverseName = _inverseName;
this._inverseQualifiedName = _inverseQualifiedName;
this._associationClassOne = _associationClassOne;
this._memberEndOfAssociationClass = _memberEndOfAssociationClass;
this._associationClassPropertyName = _associationClassPropertyName;
this._inverseAssociationClassPropertyName = _inverseAssociationClassPropertyName;
this._associationClassProperty = _associationClassProperty;
this._onePrimitivePropertyOfAssociationClass = _onePrimitivePropertyOfAssociationClass;
this._onePrimitive = _onePrimitive;
this._readOnly = _readOnly;
this.dataTypeEnum = dataTypeEnum;
this.validations = validations;
this._manyPrimitive = _manyPrimitive;
this._oneEnumeration = _oneEnumeration;
this._manyEnumeration = _manyEnumeration;
this._controllingSide = _controllingSide;
this._composite = _composite;
this._inverseComposite = _inverseComposite;
this._label = _label;
this._oneToOne = _oneToOne;
this._oneToMany = _oneToMany;
this._manyToOne = _manyToOne;
this._manyToMany = _manyToMany;
this._upper = _upper;
this._lower = _lower;
this._inverseUpper = _inverseUpper;
this._qualified = _qualified;
this._inverseQualified = _inverseQualified;
this._ordered = _ordered;
this._inverseOrdered = _inverseOrdered;
this._unique = _unique;
this._inverseUnique = _inverseUnique;
this._derived = _derived;
this._navigability = _navigability;
this._propertyType = _propertyType;
this._json = _json;
this._changeListener = _changeListener;
}
static public String asJson() {
int count = 1;
StringBuilder sb = new StringBuilder();;
sb.append("{\"name\": \"TestValidation\", ");
sb.append("\"qualifiedName\": \"umlgtest::org::umlg::validation::TestValidation\", ");
sb.append("\"uri\": \"TODO\", ");
sb.append("\"properties\": [");
for ( TestValidationRuntimePropertyEnum l : TestValidationRuntimePropertyEnum.values() ) {
sb.append(l.toJson());
if ( count < TestValidationRuntimePropertyEnum.values().length ) {
count++;
sb.append(",");
}
}
sb.append("]}");
return sb.toString();
}
static public TestValidationRuntimePropertyEnum fromInverseQualifiedName(String inverseQualifiedName) {
if ( umlgtest.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return umlgtest;
}
if ( testUnlimitedNatural.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return testUnlimitedNatural;
}
if ( aMaxUnlimitedNatural.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMaxUnlimitedNatural;
}
if ( aMinUnlimitedNatural.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMinUnlimitedNatural;
}
if ( aRangeInteger.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aRangeInteger;
}
if ( aMaxReal.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMaxReal;
}
if ( aMinInteger.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMinInteger;
}
if ( aRangeUnlimitedNatural.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aRangeUnlimitedNatural;
}
if ( aRangeReal.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aRangeReal;
}
if ( aMaxInteger.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMaxInteger;
}
if ( aMinReal.getInverseQualifiedName().equals(inverseQualifiedName) ) {
return aMinReal;
}
return null;
}
static public TestValidationRuntimePropertyEnum fromLabel(String _label) {
if ( umlgtest.getLabel().equals(_label) ) {
return umlgtest;
}
if ( testUnlimitedNatural.getLabel().equals(_label) ) {
return testUnlimitedNatural;
}
if ( aMaxUnlimitedNatural.getLabel().equals(_label) ) {
return aMaxUnlimitedNatural;
}
if ( aMinUnlimitedNatural.getLabel().equals(_label) ) {
return aMinUnlimitedNatural;
}
if ( aRangeInteger.getLabel().equals(_label) ) {
return aRangeInteger;
}
if ( aMaxReal.getLabel().equals(_label) ) {
return aMaxReal;
}
if ( aMinInteger.getLabel().equals(_label) ) {
return aMinInteger;
}
if ( aRangeUnlimitedNatural.getLabel().equals(_label) ) {
return aRangeUnlimitedNatural;
}
if ( aRangeReal.getLabel().equals(_label) ) {
return aRangeReal;
}
if ( aMaxInteger.getLabel().equals(_label) ) {
return aMaxInteger;
}
if ( aMinReal.getLabel().equals(_label) ) {
return aMinReal;
}
return null;
}
static public TestValidationRuntimePropertyEnum fromQualifiedName(String qualifiedName) {
if ( umlgtest.getQualifiedName().equals(qualifiedName) ) {
return umlgtest;
}
if ( testUnlimitedNatural.getQualifiedName().equals(qualifiedName) ) {
return testUnlimitedNatural;
}
if ( aMaxUnlimitedNatural.getQualifiedName().equals(qualifiedName) ) {
return aMaxUnlimitedNatural;
}
if ( aMinUnlimitedNatural.getQualifiedName().equals(qualifiedName) ) {
return aMinUnlimitedNatural;
}
if ( aRangeInteger.getQualifiedName().equals(qualifiedName) ) {
return aRangeInteger;
}
if ( aMaxReal.getQualifiedName().equals(qualifiedName) ) {
return aMaxReal;
}
if ( aMinInteger.getQualifiedName().equals(qualifiedName) ) {
return aMinInteger;
}
if ( aRangeUnlimitedNatural.getQualifiedName().equals(qualifiedName) ) {
return aRangeUnlimitedNatural;
}
if ( aRangeReal.getQualifiedName().equals(qualifiedName) ) {
return aRangeReal;
}
if ( aMaxInteger.getQualifiedName().equals(qualifiedName) ) {
return aMaxInteger;
}
if ( aMinReal.getQualifiedName().equals(qualifiedName) ) {
return aMinReal;
}
return null;
}
public String getAssociationClassPropertyName() {
return this._associationClassPropertyName;
}
public DataTypeEnum getDataTypeEnum() {
return this.dataTypeEnum;
}
public String getInverseAssociationClassPropertyName() {
return this._inverseAssociationClassPropertyName;
}
public String getInverseName() {
return this._inverseName;
}
public String getInverseQualifiedName() {
return this._inverseQualifiedName;
}
public int getInverseUpper() {
return this._inverseUpper;
}
public String getJson() {
return this._json;
}
public String getLabel() {
return this._label;
}
public int getLower() {
return this._lower;
}
public String getPersistentName() {
return this._persistentName;
}
public Class getPropertyType() {
return this._propertyType;
}
public String getQualifiedName() {
return this._qualifiedName;
}
public Boolean getReadOnly() {
return this._readOnly;
}
public int getUpper() {
return this._upper;
}
public List getValidations() {
return this.validations;
}
public boolean isAssociationClassOne() {
return this._associationClassOne;
}
public boolean isAssociationClassProperty() {
return this._associationClassProperty;
}
public boolean isChangeListener() {
return this._changeListener;
}
public boolean isComposite() {
return this._composite;
}
public boolean isControllingSide() {
return this._controllingSide;
}
public boolean isDerived() {
return this._derived;
}
public boolean isInverseComposite() {
return this._inverseComposite;
}
public boolean isInverseOrdered() {
return this._inverseOrdered;
}
public boolean isInverseQualified() {
return this._inverseQualified;
}
public boolean isInverseUnique() {
return this._inverseUnique;
}
public boolean isManyEnumeration() {
return this._manyEnumeration;
}
public boolean isManyPrimitive() {
return this._manyPrimitive;
}
public boolean isManyToMany() {
return this._manyToMany;
}
public boolean isManyToOne() {
return this._manyToOne;
}
public boolean isMemberEndOfAssociationClass() {
return this._memberEndOfAssociationClass;
}
public boolean isNavigability() {
return this._navigability;
}
public boolean isOneEnumeration() {
return this._oneEnumeration;
}
public boolean isOnePrimitive() {
return this._onePrimitive;
}
public boolean isOnePrimitivePropertyOfAssociationClass() {
return this._onePrimitivePropertyOfAssociationClass;
}
public boolean isOneToMany() {
return this._oneToMany;
}
public boolean isOneToOne() {
return this._oneToOne;
}
public boolean isOrdered() {
return this._ordered;
}
public boolean isQualified() {
return this._qualified;
}
public boolean isUnique() {
return this._unique;
}
@Override
public boolean isValid(int elementCount) {
if ( isQualified() ) {
return elementCount >= getLower();
} else {
return (getUpper() == -1 || elementCount <= getUpper()) && elementCount >= getLower();
}
}
@Override
public String toJson() {
return getJson();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy