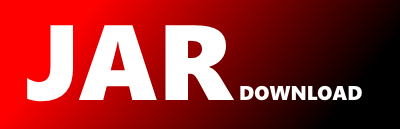
org.umlg.runtime.adaptor.UmlgSchemaCreatorImpl Maven / Gradle / Ivy
package org.umlg.runtime.adaptor;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.umlg.associationclass.Company;
import org.umlg.associationclass.Friendship;
import org.umlg.associationclass.Job;
import org.umlg.associationclass.Person;
import org.umlg.enumeration.TestEnumeration;
import org.umlg.oclassociationrole.Many;
import org.umlg.oclassociationrole.One;
import org.umlg.qualifier.A;
import org.umlg.qualifier.B;
import org.umlg.qualifier.Bank;
import org.umlg.qualifier.C;
import org.umlg.qualifier.Customer;
import org.umlg.qualifier.D;
import org.umlg.qualifier.Depth1;
import org.umlg.qualifier.Depth2;
import org.umlg.qualifier.Employee;
import org.umlg.runtime.domain.UmlgNode;
import org.umlg.testocl.OclTest1;
import org.umlg.testocl.OclTest2;
import org.umlg.testocl.OclTestCollection;
import org.umlg.testocl.OclTestCollection2;
public class UmlgSchemaCreatorImpl implements UmlgSchemaCreator {
static public UmlgSchemaCreatorImpl INSTANCE = new UmlgSchemaCreatorImpl();
private Set> qualifiedNameVertexSchemaSet = new HashSet>();
private Set qualifiedNameEdgeSchemaSet = new HashSet();
/**
* constructor for UmlgSchemaCreatorImpl
*/
private UmlgSchemaCreatorImpl() {
addAllVertexEntries();
addAllEdgeEntries();
}
@Override
public void createEdgeSchemas(EdgeSchemaCreator edgeSchemaCreator) {
for ( String label : qualifiedNameEdgeSchemaSet ) {
edgeSchemaCreator.create(label);
}
}
@Override
public void createVertexSchemas(VertexSchemaCreator vertexSchemaCreator) {
for ( List hierarchy : qualifiedNameVertexSchemaSet ) {
vertexSchemaCreator.create(hierarchy);
}
}
static public UmlgSchemaCreator getInstance() {
return INSTANCE;
}
private void addAllEdgeEntries() {
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("rootOclTest1Meta");
this.qualifiedNameEdgeSchemaSet.add("root_OclTest1");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("rootOclTestCollectionMeta");
this.qualifiedNameEdgeSchemaSet.add("root_OclTestCollection");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("rootOclTestCollection2Meta");
this.qualifiedNameEdgeSchemaSet.add("root_OclTestCollection2");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("rootOclTest2Meta");
this.qualifiedNameEdgeSchemaSet.add("root_OclTest2");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("rootBankMeta");
this.qualifiedNameEdgeSchemaSet.add("root_Bank");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("customer_depth1_1");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("rootCustomerMeta");
this.qualifiedNameEdgeSchemaSet.add("root_Customer");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("A__");
this.qualifiedNameEdgeSchemaSet.add("rootEmployeeMeta");
this.qualifiedNameEdgeSchemaSet.add("root_Employee");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("customer_depth1_1");
this.qualifiedNameEdgeSchemaSet.add("depth1_depth2_1");
this.qualifiedNameEdgeSchemaSet.add("rootDepth1Meta");
this.qualifiedNameEdgeSchemaSet.add("root_Depth1");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("depth1_depth2_1");
this.qualifiedNameEdgeSchemaSet.add("rootDepth2Meta");
this.qualifiedNameEdgeSchemaSet.add("root_Depth2");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("a_b_1");
this.qualifiedNameEdgeSchemaSet.add("rootAMeta");
this.qualifiedNameEdgeSchemaSet.add("root_A");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("a_b_1");
this.qualifiedNameEdgeSchemaSet.add("b_c_1");
this.qualifiedNameEdgeSchemaSet.add("rootBMeta");
this.qualifiedNameEdgeSchemaSet.add("root_B");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("c_d_1");
this.qualifiedNameEdgeSchemaSet.add("b_c_1");
this.qualifiedNameEdgeSchemaSet.add("rootCMeta");
this.qualifiedNameEdgeSchemaSet.add("root_C");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("c_d_1");
this.qualifiedNameEdgeSchemaSet.add("rootDMeta");
this.qualifiedNameEdgeSchemaSet.add("root_D");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("one_many_1");
this.qualifiedNameEdgeSchemaSet.add("rootOneMeta");
this.qualifiedNameEdgeSchemaSet.add("root_One");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("one_many_1");
this.qualifiedNameEdgeSchemaSet.add("rootManyMeta");
this.qualifiedNameEdgeSchemaSet.add("root_Many");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("Friendship");
this.qualifiedNameEdgeSchemaSet.add("Job");
this.qualifiedNameEdgeSchemaSet.add("Friendship");
this.qualifiedNameEdgeSchemaSet.add("rootPersonMeta");
this.qualifiedNameEdgeSchemaSet.add("root_Person");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("Job");
this.qualifiedNameEdgeSchemaSet.add("rootCompanyMeta");
this.qualifiedNameEdgeSchemaSet.add("root_Company");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("rootJobMeta");
this.qualifiedNameEdgeSchemaSet.add("root_Job");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("rootFriendshipMeta");
this.qualifiedNameEdgeSchemaSet.add("root_Friendship");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
this.qualifiedNameEdgeSchemaSet.add("A_testEnumeration_eNUMX_1");
this.qualifiedNameEdgeSchemaSet.add("rootTestEnumerationMeta");
this.qualifiedNameEdgeSchemaSet.add("root_TestEnumeration");
this.qualifiedNameEdgeSchemaSet.add("deletedVertexEdgeToRoot");
this.qualifiedNameEdgeSchemaSet.add("allinstances");
}
private void addAllVertexEntries() {
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::testocl::OclTest1"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.testocl.meta.OclTest1Meta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::testocl::OclTestCollection"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.testocl.meta.OclTestCollectionMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::testocl::OclTestCollection2"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.testocl.meta.OclTestCollection2Meta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::testocl::OclTest2"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.testocl.meta.OclTest2Meta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::Bank"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.BankMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::Customer"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.CustomerMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::Employee"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.EmployeeMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::Depth1"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.Depth1Meta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::Depth2"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.Depth2Meta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::A"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.AMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::B"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.BMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::C"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.CMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::qualifier::D"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.qualifier.meta.DMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::oclassociationrole::One"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.oclassociationrole.meta.OneMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::oclassociationrole::Many"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.oclassociationrole.meta.ManyMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::associationclass::Person"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.associationclass.meta.PersonMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::associationclass::Company"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.associationclass.meta.CompanyMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::associationclass::Job"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.associationclass.meta.JobMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::associationclass::Friendship"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.associationclass.meta.FriendshipMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org::umlg::runtime::domain::BaseUmlgCompositionNode", "org::umlg::enumeration::TestEnumeration"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("org.umlg.enumeration.meta.TestEnumerationMeta"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("rootVertex"));
this.qualifiedNameVertexSchemaSet.add(Arrays.asList("deletionVertex"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy