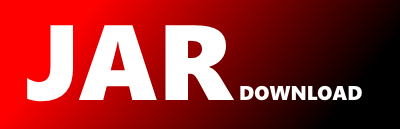
org.unidal.dal.jdbc.BizObjectHelper Maven / Gradle / Ivy
The newest version!
package org.unidal.dal.jdbc;
import java.lang.reflect.Constructor;
import java.util.ArrayList;
import java.util.List;
public class BizObjectHelper {
@SuppressWarnings("unchecked")
private static Constructor getBoConstructor(Class boClass) {
try {
Constructor[] cs = (Constructor[]) boClass.getConstructors();
for (int i = 0; i < cs.length; i++) {
Class>[] paramTypes = cs[i].getParameterTypes();
if (paramTypes.length == 1 && DataObject.class.isAssignableFrom(paramTypes[0])) {
return cs[i];
}
}
} catch (Exception e) {
// ignore it
}
throw new RuntimeException("Can't find a constructor for creating a BO instance from " + boClass);
}
public static List unwrap(List bos, Class doClass) {
List dos = new ArrayList(bos.size());
for (T bo : bos) {
dos.add(unwrap(bo, doClass));
}
return dos;
}
@SuppressWarnings("unchecked")
public static S unwrap(T bo, Class doClass) {
return (S) bo.getDo();
}
public static List wrap(List rows, Class boClass) {
List bos = new ArrayList(rows.size());
for (S row : rows) {
bos.add(wrap(row, boClass));
}
return bos;
}
public static T wrap(S row, Class boClass) {
try {
Constructor c = getBoConstructor(boClass);
return c.newInstance(new Object[] { row });
} catch (Exception e) {
throw new RuntimeException("Can't create a BO instance from " + boClass, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy