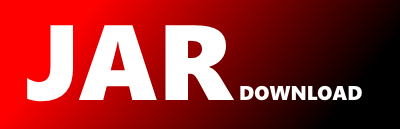
org.unidal.dal.jdbc.entity.DefaultDataObjectAssembly Maven / Gradle / Ivy
The newest version!
package org.unidal.dal.jdbc.entity;
import java.lang.reflect.Method;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.unidal.dal.jdbc.DalRuntimeException;
import org.unidal.dal.jdbc.DataField;
import org.unidal.dal.jdbc.DataObject;
import org.unidal.dal.jdbc.engine.QueryContext;
import org.unidal.dal.jdbc.raw.RawEntity;
import org.unidal.lookup.annotation.Inject;
import org.unidal.lookup.annotation.Named;
@Named(type = DataObjectAssembly.class)
public class DefaultDataObjectAssembly implements DataObjectAssembly {
@Inject
private DataObjectAccessor m_accessor;
@Inject
private DataObjectNaming m_naming;
private Map, Map> m_subObjectsMap = new HashMap, Map>();
private Map m_cache = new HashMap();
@SuppressWarnings("unchecked")
public T newRow(T proto, List subObjectNames, List result) {
Class extends DataObject> masterClass = proto.getClass();
Map subObjects = m_subObjectsMap.get(masterClass);
if (subObjects == null) {
subObjects = new LinkedHashMap();
m_subObjectsMap.put(masterClass, subObjects);
}
T row = (T) m_accessor.newInstance(masterClass);
m_cache.clear();
for (String subObjectName : subObjectNames) {
if (subObjectName != null) {
Method setMethod = subObjects.get(subObjectName);
if (setMethod == null) {
setMethod = m_naming.getSetMethod(masterClass, subObjectName);
subObjects.put(subObjectName, setMethod);
}
DataObject subObject = m_cache.get(setMethod);
if (subObject == null) {
Class subObjectType = (Class) setMethod.getParameterTypes()[0];
subObject = m_accessor.newInstance(subObjectType);
try {
setMethod.invoke(row, new Object[] { subObject });
} catch (Exception e) {
throw new DalRuntimeException("Error when setting SubObject(" + subObject + " to DataObject(" + row
+ ")");
}
m_cache.put(setMethod, subObject);
}
result.add(subObject);
} else {
result.add(row);
}
}
return row;
}
private void prepareOutFields(QueryContext ctx, ResultSet rs) throws SQLException {
ResultSetMetaData metadata = rs.getMetaData();
List outFields = ctx.getOutFields();
int count = metadata.getColumnCount();
for (int i = 1; i <= count; i++) {
String name = metadata.getColumnName(i);
DataField field = new DataField(name);
field.setEntityClass(RawEntity.class);
field.setIndex(i - 1);
outFields.add(field);
}
}
@Override
@SuppressWarnings("unchecked")
public List assemble(QueryContext ctx, ResultSet rs) throws SQLException {
List outFields = ctx.getOutFields();
if (ctx.getQuery().isRaw()) {
prepareOutFields(ctx, rs);
}
List outSubObjectNames = ctx.getOutSubObjectNames();
List subObjects = new ArrayList();
T proto = (T) ctx.getProto();
List rows = new ArrayList();
while (rs.next()) {
subObjects.clear();
T row = newRow(proto, outSubObjectNames, subObjects);
int len = outFields.size();
for (int i = 0; i < len; i++) {
DataField field = outFields.get(i);
if (ctx.getQuery().isRaw()) {
m_accessor.setFieldValue(row, field, rs.getObject(i + 1));
} else {
DataObject subObject = subObjects.get(i);
m_accessor.setFieldValue(subObject, field, rs.getObject(i + 1));
}
}
// call afterLoad() to do some custom data manipulation
row.afterLoad();
rows.add(row);
}
rs.close();
return rows;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy