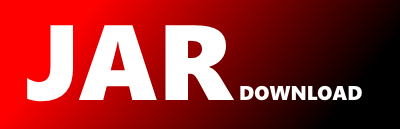
org.unidal.eunit.model.entity.EunitClass Maven / Gradle / Ivy
/* THIS FILE WAS AUTO GENERATED BY codegen-maven-plugin, DO NOT EDIT IT */
package org.unidal.eunit.model.entity;
import java.util.ArrayList;
import java.util.List;
import org.unidal.eunit.model.BaseEntity;
import org.unidal.eunit.model.IVisitor;
public class EunitClass extends BaseEntity {
private Class> m_type;
private Boolean m_ignored;
private transient List m_annotations = new ArrayList();
private List m_groups = new ArrayList();
private List m_fields = new ArrayList();
private List m_methods = new ArrayList();
public EunitClass() {
}
@Override
public void accept(IVisitor visitor) {
visitor.visitEunitClass(this);
}
public EunitClass addAnnotation(java.lang.annotation.Annotation annotation) {
m_annotations.add(annotation);
return this;
}
public EunitClass addField(EunitField field) {
m_fields.add(field);
return this;
}
public EunitClass addGroup(String group) {
m_groups.add(group);
return this;
}
public EunitClass addMethod(EunitMethod method) {
m_methods.add(method);
return this;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof EunitClass) {
EunitClass _o = (EunitClass) obj;
if (!equals(getType(), _o.getType())) {
return false;
}
if (!equals(getIgnored(), _o.getIgnored())) {
return false;
}
if (!equals(getGroups(), _o.getGroups())) {
return false;
}
if (!equals(getFields(), _o.getFields())) {
return false;
}
if (!equals(getMethods(), _o.getMethods())) {
return false;
}
return true;
}
return false;
}
public EunitField findField(String name) {
for (EunitField field : m_fields) {
if (!equals(field.getName(), name)) {
continue;
}
return field;
}
return null;
}
public EunitMethod findMethod(String name) {
for (EunitMethod method : m_methods) {
if (!equals(method.getName(), name)) {
continue;
}
return method;
}
return null;
}
@SuppressWarnings("unchecked")
public T findAnnotation(Class clazz) {
for (java.lang.annotation.Annotation annotation : m_annotations) {
if (annotation.annotationType() == clazz) {
return (T) annotation;
}
}
return null;
}
public List getAnnotations() {
return m_annotations;
}
public List getFields() {
return m_fields;
}
public List getGroups() {
return m_groups;
}
public Boolean getIgnored() {
return m_ignored;
}
public List getMethods() {
return m_methods;
}
public Class> getType() {
return m_type;
}
@Override
public int hashCode() {
int hash = 0;
hash = hash * 31 + (m_type == null ? 0 : m_type.hashCode());
hash = hash * 31 + (m_ignored == null ? 0 : m_ignored.hashCode());
for (String e : m_groups) {
hash = hash * 31 + (e == null ? 0 :e.hashCode());
}
for (EunitField e : m_fields) {
hash = hash * 31 + (e == null ? 0 :e.hashCode());
}
for (EunitMethod e : m_methods) {
hash = hash * 31 + (e == null ? 0 :e.hashCode());
}
return hash;
}
public boolean isAnnotationPresent(Class extends java.lang.annotation.Annotation> clazz) {
return findAnnotation(clazz) != null;
}
public boolean isIgnored() {
return m_ignored != null && m_ignored.booleanValue();
}
@Override
public void mergeAttributes(EunitClass other) {
if (other.getType() != null) {
m_type = other.getType();
}
if (other.getIgnored() != null) {
m_ignored = other.getIgnored();
}
}
public EunitField removeField(String name) {
int len = m_fields.size();
for (int i = 0; i < len; i++) {
EunitField field = m_fields.get(i);
if (!equals(field.getName(), name)) {
continue;
}
return m_fields.remove(i);
}
return null;
}
public EunitMethod removeMethod(String name) {
int len = m_methods.size();
for (int i = 0; i < len; i++) {
EunitMethod method = m_methods.get(i);
if (!equals(method.getName(), name)) {
continue;
}
return m_methods.remove(i);
}
return null;
}
public EunitClass setIgnored(Boolean ignored) {
m_ignored = ignored;
return this;
}
public EunitClass setType(Class> type) {
m_type = type;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy