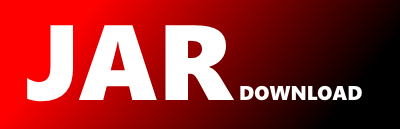
org.unidal.eunit.model.entity.EunitMethod Maven / Gradle / Ivy
/* THIS FILE WAS AUTO GENERATED BY codegen-maven-plugin, DO NOT EDIT IT */
package org.unidal.eunit.model.entity;
import static org.unidal.eunit.model.Constants.ATTR_NAME;
import static org.unidal.eunit.model.Constants.ENTITY_EUNIT_METHOD;
import java.util.ArrayList;
import java.util.List;
import org.unidal.eunit.model.BaseEntity;
import org.unidal.eunit.model.IVisitor;
public class EunitMethod extends BaseEntity {
private String m_name;
private Boolean m_test;
private Long m_timeout;
private Boolean m_ignored;
private Boolean m_beforeAfter;
private Boolean m_static;
private Class> m_returnType;
private transient java.lang.reflect.Method m_method;
private transient EunitClass m_eunitClass;
private transient List m_annotations = new ArrayList();
private List m_groups = new ArrayList();
private List m_parameters = new ArrayList();
private List m_expectedExceptions = new ArrayList();
public EunitMethod() {
}
public EunitMethod(String name) {
m_name = name;
}
@Override
public void accept(IVisitor visitor) {
visitor.visitEunitMethod(this);
}
public EunitMethod addAnnotation(java.lang.annotation.Annotation annotation) {
m_annotations.add(annotation);
return this;
}
public EunitMethod addExpectedException(EunitException expectedException) {
m_expectedExceptions.add(expectedException);
return this;
}
public EunitMethod addGroup(String group) {
m_groups.add(group);
return this;
}
public EunitMethod addParameter(EunitParameter parameter) {
m_parameters.add(parameter);
return this;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof EunitMethod) {
EunitMethod _o = (EunitMethod) obj;
if (!equals(getName(), _o.getName())) {
return false;
}
return true;
}
return false;
}
@SuppressWarnings("unchecked")
public T findAnnotation(Class clazz) {
for (java.lang.annotation.Annotation annotation : m_annotations) {
if (annotation.annotationType() == clazz) {
return (T) annotation;
}
}
return null;
}
public List getAnnotations() {
return m_annotations;
}
public Boolean getBeforeAfter() {
return m_beforeAfter;
}
public EunitClass getEunitClass() {
return m_eunitClass;
}
public List getExpectedExceptions() {
return m_expectedExceptions;
}
public List getGroups() {
return m_groups;
}
public Boolean getIgnored() {
return m_ignored;
}
public java.lang.reflect.Method getMethod() {
return m_method;
}
public String getName() {
return m_name;
}
public List getParameters() {
return m_parameters;
}
public Class> getReturnType() {
return m_returnType;
}
public Boolean getStatic() {
return m_static;
}
public Boolean getTest() {
return m_test;
}
public Long getTimeout() {
return m_timeout;
}
@Override
public int hashCode() {
int hash = 0;
hash = hash * 31 + (m_name == null ? 0 : m_name.hashCode());
return hash;
}
public boolean isAnnotationPresent(Class extends java.lang.annotation.Annotation> clazz) {
return findAnnotation(clazz) != null;
}
public boolean isBeforeAfter() {
return m_beforeAfter != null && m_beforeAfter.booleanValue();
}
public boolean isIgnored() {
return m_ignored != null && m_ignored.booleanValue();
}
public boolean isStatic() {
return m_static != null && m_static.booleanValue();
}
public boolean isTest() {
return m_test != null && m_test.booleanValue();
}
@Override
public void mergeAttributes(EunitMethod other) {
assertAttributeEquals(other, ENTITY_EUNIT_METHOD, ATTR_NAME, m_name, other.getName());
if (other.getTest() != null) {
m_test = other.getTest();
}
if (other.getTimeout() != null) {
m_timeout = other.getTimeout();
}
if (other.getIgnored() != null) {
m_ignored = other.getIgnored();
}
if (other.getBeforeAfter() != null) {
m_beforeAfter = other.getBeforeAfter();
}
if (other.getStatic() != null) {
m_static = other.getStatic();
}
if (other.getReturnType() != null) {
m_returnType = other.getReturnType();
}
if (other.getMethod() != null) {
m_method = other.getMethod();
}
if (other.getEunitClass() != null) {
m_eunitClass = other.getEunitClass();
}
}
public EunitMethod setBeforeAfter(Boolean beforeAfter) {
m_beforeAfter = beforeAfter;
return this;
}
public EunitMethod setEunitClass(EunitClass eunitClass) {
m_eunitClass = eunitClass;
return this;
}
public EunitMethod setIgnored(Boolean ignored) {
m_ignored = ignored;
return this;
}
public EunitMethod setMethod(java.lang.reflect.Method method) {
m_method = method;
return this;
}
public EunitMethod setName(String name) {
m_name = name;
return this;
}
public EunitMethod setReturnType(Class> returnType) {
m_returnType = returnType;
return this;
}
public EunitMethod setStatic(Boolean _static) {
m_static = _static;
return this;
}
public EunitMethod setTest(Boolean test) {
m_test = test;
return this;
}
public EunitMethod setTimeout(Long timeout) {
m_timeout = timeout;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy