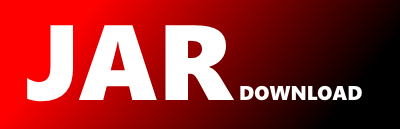
org.unidal.eunit.testfwk.CaseContext Maven / Gradle / Ivy
package org.unidal.eunit.testfwk;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.List;
import org.unidal.eunit.invocation.IMethodInvoker;
import org.unidal.eunit.invocation.IParameterResolver;
import org.unidal.eunit.model.entity.EunitClass;
import org.unidal.eunit.model.entity.EunitMethod;
import org.unidal.eunit.model.entity.EunitParameter;
import org.unidal.eunit.testfwk.spi.ICaseContext;
import org.unidal.eunit.testfwk.spi.IClassContext;
import org.unidal.eunit.testfwk.spi.task.ITask;
import org.unidal.eunit.testfwk.spi.task.ITaskType;
public class CaseContext implements ICaseContext {
private IClassContext m_ctx;
private EunitMethod m_eunitMethod;
private ITask extends ITaskType> m_task;
private Object m_testInstance;
public CaseContext(IClassContext ctx, EunitMethod eunitMethod) {
m_ctx = ctx;
m_eunitMethod = eunitMethod;
Class> testClass = ctx.getTestClass();
try {
Constructor> c = testClass.getDeclaredConstructor();
if (!c.isAccessible()) {
c.setAccessible(true);
}
m_testInstance = c.newInstance();
} catch (Exception e) {
throw new RuntimeException(String.format(
"Unable to create instance of test class(%s), please make sure it has a public zero-argument constructor defined!",
testClass.getName()));
}
}
protected Object checkAndGetFirstAttribute(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy