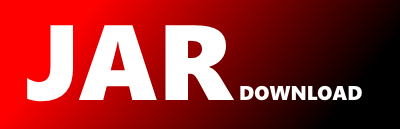
org.unidal.eunit.testfwk.spi.Registry Maven / Gradle / Ivy
package org.unidal.eunit.testfwk.spi;
import java.lang.annotation.ElementType;
import java.lang.annotation.Target;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.unidal.eunit.invocation.IMethodInvoker;
import org.unidal.eunit.invocation.IParameterResolver;
import org.unidal.eunit.testfwk.spi.event.IEventListener;
import org.unidal.eunit.testfwk.spi.task.ITaskExecutor;
import org.unidal.eunit.testfwk.spi.task.ITaskType;
import org.unidal.eunit.testfwk.spi.task.IValve;
import org.unidal.eunit.testfwk.spi.task.Priority;
import org.unidal.eunit.testfwk.spi.task.ValveMap;
public class Registry {
private Class> m_namespace;
private Map>> m_handlers = new HashMap>>();
private Map>> m_metaHandlers = new HashMap>>();
private Map> m_executors = new HashMap>();
private IClassProcessor m_classProcessor;
private List m_listeners = new ArrayList();
private ITestPlanBuilder extends ITestCallback> m_testPlanBuilder;
private ITestCaseBuilder extends ITestCallback> m_testCaseBuilder;
private IMethodInvoker m_methodInvoker;
private ICaseContextFactory m_caseContextFactory;
private List> m_paramResolvers = new ArrayList>();
private ValveMap m_caseValveMap = new ValveMap();
private ValveMap m_classValveMap = new ValveMap();
public Registry(Class> namespace) {
m_namespace = namespace;
}
private void addAnnotationHandler(ElementType type, IAnnotationHandler, ?> handler) {
List> list = m_handlers.get(type);
if (list == null) {
list = new ArrayList>();
m_handlers.put(type, list);
}
if (!list.contains(handler)) {
list.add(handler);
}
}
private void addMetaAnnotationHandler(ElementType type, IMetaAnnotationHandler, ?> handler) {
List> list = m_metaHandlers.get(type);
if (list == null) {
list = new ArrayList>();
m_metaHandlers.put(type, list);
}
if (!list.contains(handler)) {
list.add(handler);
}
}
public List> getAnnotationHandlers(ElementType type) {
List> list = m_handlers.get(type);
if (list == null) {
return Collections.emptyList();
} else {
return list;
}
}
public ICaseContextFactory getCaseContextFactory() {
return m_caseContextFactory;
}
public ValveMap getCaseValveMap() {
return m_caseValveMap;
}
public IClassProcessor getClassProcessor() {
return m_classProcessor;
}
public ValveMap getClassValveMap() {
return m_classValveMap;
}
public List getListeners() {
return m_listeners;
}
public List> getMetaAnnotationHandlers(ElementType type) {
List> list = m_metaHandlers.get(type);
if (list == null) {
return Collections.emptyList();
} else {
return list;
}
}
public IMethodInvoker getMethodInvoker() {
return m_methodInvoker;
}
public Class> getNamespace() {
return m_namespace;
}
public List> getParamResolvers() {
return m_paramResolvers;
}
public ITaskExecutor getTaskExecutor(ITaskType type) {
ITaskExecutor executor = m_executors.get(type);
if (executor == null) {
throw new IllegalStateException(String.format("No task executor registered for task type(%s)!", type));
}
return executor;
}
public ITestCaseBuilder extends ITestCallback> getTestCaseBuilder() {
return m_testCaseBuilder;
}
public ITestPlanBuilder extends ITestCallback> getTestPlanBuilder() {
return m_testPlanBuilder;
}
public void registerAnnotationHandler(IAnnotationHandler, ?> handler) {
Class> annotation = handler.getTargetAnnotation();
Target target = annotation.getAnnotation(Target.class);
if (target == null) {
throw new IllegalArgumentException(String.format("No @Target applied to annotation %s!", annotation));
}
for (ElementType type : target.value()) {
addAnnotationHandler(type, handler);
}
}
public void registerCaseContextFactory(ICaseContextFactory caseContextFactory) {
m_caseContextFactory = caseContextFactory;
}
public void registerCaseValve(Priority priority, IValve extends ICaseContext> valve) {
m_caseValveMap.addValve(priority, valve);
}
public void registerCaseValve(Priority priority, IValve extends ICaseContext> valve, boolean append) {
m_caseValveMap.addValve(priority, valve, append);
}
public void registerClassProcessor(IClassProcessor classProcessor) {
m_classProcessor = classProcessor;
}
public void registerClassValve(IValve extends ICaseContext> valve) {
m_classValveMap.addValve(Priority.LOW, valve);
}
public void registerClassValve(Priority priority, IValve extends ICaseContext> valve) {
m_classValveMap.addValve(priority, valve);
}
public void registerClassValve(Priority priority, IValve extends ICaseContext> valve, boolean append) {
m_classValveMap.addValve(priority, valve, append);
}
public void registerEventListener(IEventListener listener) {
if (!m_listeners.contains(listener)) {
m_listeners.add(listener);
}
}
public void registerMetaAnnotationHandler(IMetaAnnotationHandler, ?> handler) {
Class> annotation = handler.getTargetAnnotation();
Target target = annotation.getAnnotation(Target.class);
if (target == null) {
throw new IllegalArgumentException(String.format("No @Target applied to annotation %s!", annotation));
}
for (ElementType type : target.value()) {
addMetaAnnotationHandler(type, handler);
}
}
public void registerMethodInvoker(IMethodInvoker methodInvoker) {
m_methodInvoker = methodInvoker;
}
@SuppressWarnings("unchecked")
public void registerParamResolver(IParameterResolver extends ICaseContext> resolver) {
if (!m_paramResolvers.contains(resolver)) {
m_paramResolvers.add((IParameterResolver) resolver);
}
}
public void registerTaskExecutors(ITaskExecutor... executors) {
for (ITaskExecutor executor : executors) {
@SuppressWarnings("unchecked")
ITaskExecutor e = (ITaskExecutor) executor;
m_executors.put(e.getTaskType(), e);
}
}
public void registerTestCaseBuilder(ITestCaseBuilder extends ITestCallback> testCaseBuilder) {
m_testCaseBuilder = testCaseBuilder;
}
public void registerTestPlanBuilder(ITestPlanBuilder extends ITestCallback> testPlanBuilder) {
m_testPlanBuilder = testPlanBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy