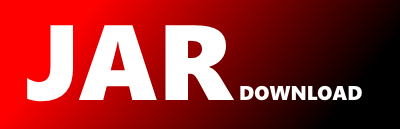
org.unidal.converter.dom.NodeArrayConverter Maven / Gradle / Ivy
The newest version!
package org.unidal.converter.dom;
import java.lang.reflect.Array;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.List;
import org.unidal.converter.Converter;
import org.unidal.converter.ConverterException;
import org.unidal.converter.ConverterManager;
import org.unidal.converter.TypeUtil;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
public class NodeArrayConverter implements Converter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy