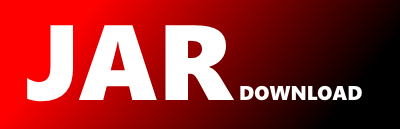
org.unidal.helper.Properties Maven / Gradle / Ivy
The newest version!
package org.unidal.helper;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public class Properties {
public static StringPropertyAccessor forString() {
return new StringPropertyAccessor();
}
public static class MapPropertyProvider implements PropertyProvider {
private String m_name;
private Map m_map;
public MapPropertyProvider(Map map) {
m_map = map;
}
@Override
public Object getProperty(String name) {
T value = null;
if (m_name != null) {
name = m_name;
}
if (value == null && m_map != null) {
value = m_map.get(name);
}
return value;
}
public MapPropertyProvider setName(String name) {
m_name = name;
return this;
}
}
public static abstract class PropertyAccessor {
private List m_providers = new ArrayList();
public PropertyAccessor fromEnv() {
return fromEnv(null);
}
public PropertyAccessor fromEnv(String name) {
m_providers.add(new SystemPropertyProvider(false, true).setName(name));
return this;
}
public PropertyAccessor fromMap(Map map) {
return fromMap(map, null);
}
public PropertyAccessor fromMap(Map map, String name) {
m_providers.add(new MapPropertyProvider(map).setName(name));
return this;
}
public PropertyAccessor fromSystem() {
return fromSystem(null);
}
public PropertyAccessor fromSystem(String name) {
m_providers.add(new SystemPropertyProvider(true, false).setName(name));
return this;
}
protected Object getProperty(String name) {
Object value = null;
for (PropertyProvider provider : m_providers) {
value = provider.getProperty(name);
if (value != null) {
break;
}
}
return value;
}
public abstract T getProperty(String name, T defaultValue);
}
public static interface PropertyProvider {
public Object getProperty(String name);
}
public static class StringPropertyAccessor extends PropertyAccessor {
@Override
public String getProperty(String name, String defaultValue) {
Object value = name == null ? null : getProperty(name);
if (value == null) {
return defaultValue;
} else {
return value.toString();
}
}
}
public static class SystemPropertyProvider implements PropertyProvider {
private boolean m_properties;
private boolean m_env;
private String m_name;
public SystemPropertyProvider(boolean properties, boolean env) {
m_properties = properties;
m_env = env;
}
@Override
public Object getProperty(String name) {
String value = null;
if (m_name != null) {
name = m_name;
}
if (value == null && m_properties) {
value = System.getProperty(name);
}
if (value == null && m_env) {
value = System.getenv(name);
}
return value;
}
public SystemPropertyProvider setName(String name) {
m_name = name;
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy