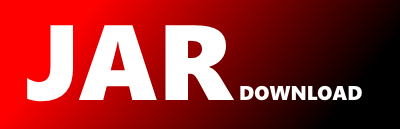
org.unidal.lookup.ContainerHolder Maven / Gradle / Ivy
The newest version!
package org.unidal.lookup;
import java.util.List;
import java.util.Map;
import org.unidal.lookup.extension.Contextualizable;
public abstract class ContainerHolder implements Contextualizable {
private PlexusContainer m_container;
public void contextualize(Map context) {
m_container = (PlexusContainer) context.get("plexus");
}
protected PlexusContainer getContainer() {
return m_container;
}
protected boolean hasComponent(Class role) {
return hasComponent(role, null);
}
protected boolean hasComponent(Class role, String roleHint) {
return getContainer().hasComponent(role, roleHint);
}
protected T lookup(Class role) throws LookupException {
return lookup(role, null);
}
protected T lookup(Class role, String roleHint) throws LookupException {
try {
return (T) getContainer().lookup(role, roleHint == null ? "default" : roleHint);
} catch (ComponentLookupException e) {
String key = role.getName() + ":" + (roleHint == null ? "default" : roleHint);
throw new LookupException("Unable to lookup component(" + key + ").", e);
}
}
protected List lookupList(Class role) throws LookupException {
try {
return (List) getContainer().lookupList(role);
} catch (ComponentLookupException e) {
String key = role.getName();
throw new LookupException("Unable to lookup component list(" + key + ").", e);
}
}
protected Map lookupMap(Class role) throws LookupException {
try {
return (Map) getContainer().lookupMap(role);
} catch (ComponentLookupException e) {
String key = role.getName();
throw new LookupException("Unable to lookup component map(" + key + ").", e);
}
}
protected void release(Object component) throws LookupException {
if (component != null) {
getContainer().release(component);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy