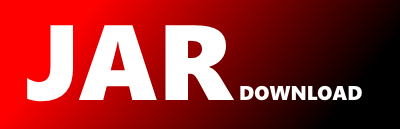
org.uniknow.spring.cqrs.example.ApplicationService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cqrs-eventsourcing Show documentation
Show all versions of cqrs-eventsourcing Show documentation
Tutorial showing how to use CQRS and Event sourcing
The newest version!
/**
* Copyright (C) 2014 uniknow. All rights reserved.
*
* This Java class is subject of the following restrictions:
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* 3. The end-user documentation included with the redistribution, if any, must
* include the following acknowledgment: "This product includes software
* developed by uniknow." Alternately, this acknowledgment may appear in the
* software itself, if and wherever such third-party acknowledgments normally
* appear.
*
* 4. The name ''uniknow'' must not be used to endorse or promote products
* derived from this software without prior written permission.
*
* 5. Products derived from this software may not be called ''UniKnow'', nor may
* ''uniknow'' appear in their name, without prior written permission of
* uniknow.
*
* THIS SOFTWARE IS PROVIDED ''AS IS'' AND ANY EXPRESSED OR IMPLIED WARRANTIES,
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND
* FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL WWS OR ITS
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOS OF USE, DATA, OR PROFITS; OR
* BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER
* IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package org.uniknow.spring.cqrs.example;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.uniknow.spring.cqrs.*;
import org.uniknow.spring.cqrs.example.event.BaseEvent;
import org.uniknow.spring.eventStore.EventState;
import org.uniknow.spring.eventStore.EventStore;
import org.uniknow.spring.eventStore.EventStream;
import java.util.List;
@Component
public class ApplicationService {
/**
* Store in which (domain) events are persisted
*/
@Autowired
private EventStore eventStore;
/**
* Provider by which Command Handlers for specific commands can be found
*/
@Autowired
private CommandHandlerProvider commandHandlerProvider;
/**
* Provider by which Event Handlers for specific event can be found
*/
@Autowired
private EventHandlerProvider eventHandlerProvider;
public void handle(Command command) throws Exception {
EventStream eventStream = eventStore
.loadEventStream(command.aggregateId());
// Process command
List events = (List) commandHandlerProvider
.getHandler(command).handle(command);
// Persist events generated by command within event store
if (events != null && events.size() > 0) {
eventStore.store(command.aggregateId(), eventStream.version(),
events);
// Notify event handlers
notifyAll(events);
} else {
// Command generated no events
}
}
private void notifyAll(List events) {
for (BaseEvent event : events) {
if (event.getState() == EventState.OK) {
// Get handlers for event
List> eventHandlers = eventHandlerProvider
.getHandler(event);
for (EventHandler eventHandler : eventHandlers) {
eventHandler.handle(event);
}
} else {
throw new RuntimeException("Event should have state OK");
}
}
}
// private Object newAggregateInstance(Command command)
// throws InstantiationException, IllegalAccessException {
// return commandHandlerLookup.targetType(command).newInstance();
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy